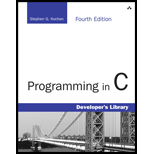
Type in and run the

Program Plan:
- Include the required headers.
- Define the structure
- Define the main method
- Declare the required variables.
- Condition to validate the arguments that are passed.
- Based on the condition that are is specified, proper resultant is being displayed.
The program is used to copy the contents of the text file 1 to the text file 2 by passing the arguments as the commands.
Explanation of Solution
//include the necessary headers
#include<stdio.h>
#include<stdlib.h>
//main method
int main(int argc,char *argv[])
{
//declare the required variables
FILE *in,*out;
int c;
//condition to validate the arguments that are passed
if(argc!=3)
{
//prompt user to enter the files
fprintf(stderr,"Need two files names\n");
//return the value
return 1;
}
//condition to validate the arguments that are passed
if((in=fopen (argv[1],"r"))==NULL)
{
//prompts the user that the file cannot be read
fprintf(stderr,"can't read %s.\n",argv[1]);
//return
return 2;
}
//condition to validate the arguments present
if((out = fopen(argv[2],"w"))==NULL)
{
//prompts user about the write error
fprintf(stderr,"can't write %s.\n",argv[2]);
//return value
return 3;
}
//loop that iterates for the end of file
while((c=getc(in))!=EOF)
//write the contents
putc(c,out);
//display file has been copied
printf("File has been copied.\n");
//close the file
fclose(in);
//close the file
fclose(out);
//return 0
return 0;
}
File has been copied.
- The above code is executed by passing the test file in the form of commands.
- Type the following for making the code to run:
Main.c test.txt test1.txt
test.txt:
Programming is fun
test1.txt:
Programming is fun
After executing bove commands the contents of the “test.txt” is being copied to “test1.txt” as shown above.
Want to see more full solutions like this?
Chapter 16 Solutions
Programming in C
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Digital Fundamentals (11th Edition)
Problem Solving with C++ (9th Edition)
- What is a file’s read position? Initially, where is the read position when an input file is opened?arrow_forwardSince the Master file contains long-term data, is it necessary?arrow_forwardExplain the difference between Sequential Files and Random Files? Why is it important to close the File objects after using them in the code?arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
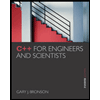