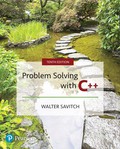
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134521176
Author: SAVITCH
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 16, Problem 5PP
Program Plan Intro
- Include required library files.
- Define a class named “StackOverflowException”.
- Inside the access specifier “public”,
- Define a constructor to assign the message
- Declare a parameterized constructor to assign “msg” to message.
- Define a function “display” to return message.
- Inside the access specifier “private”.
- Declare a string variable “message”.
- Define a class named “StackEmptyException”.
- Inside the access specifier “public”,
- Define a constructor to assign the message
- Declare a parameterized constructor to assign “msg” to message.
- Define a function “display” to return message.
- Inside the access specifier “private”.
- Declare a string variable “message”.
- Define a class named “Stack”.
- Declare an integer array and variable.
- Inside the access specifier “public”,
- Define a constructor to assign “-1” to “top”.
- Define a “push()” function.
- “try” block to check the top is equal to “3”.
- The condition is true, throw exception.
- Otherwise increment the top and the value is assigned to stack.
- “catch” block to display the error message.
- Define a “pop()” function.
- “try” block to check the top is equal to “-1”.
- The condition is true, throw exception.
- Otherwise decrement the top and return the value.
- “catch” block to display the error message.
- Define a “main()” function.
- Create an object for class “Stack”.
- Then check the “push()” and “pop()” function.
- Inside the access specifier “public”,
- Define a constructor to assign the message
- Declare a parameterized constructor to assign “msg” to message.
- Define a function “display” to return message.
- Inside the access specifier “private”.
- Declare a string variable “message”.
- Inside the access specifier “public”,
- Define a constructor to assign the message
- Declare a parameterized constructor to assign “msg” to message.
- Define a function “display” to return message.
- Inside the access specifier “private”.
- Declare a string variable “message”.
- Declare an integer array and variable.
- Inside the access specifier “public”,
- Define a constructor to assign “-1” to “top”.
- Define a “push()” function.
- “try” block to check the top is equal to “3”.
- The condition is true, throw exception.
- Otherwise increment the top and the value is assigned to stack.
- “catch” block to display the error message.
- “try” block to check the top is equal to “3”.
- Define a “pop()” function.
- “try” block to check the top is equal to “-1”.
- The condition is true, throw exception.
- Otherwise decrement the top and return the value.
- “catch” block to display the error message.
- “try” block to check the top is equal to “-1”.
- Create an object for class “Stack”.
- Then check the “push()” and “pop()” function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
ADT stack lets you peek at its top entry without removing it. For some applications of stacks, youalso need to peek at the entry beneath the top entry without removing it. We will call such an operationpeekNxt. If the stack has more than one entry, peekNxt returns the second entry from the top without altering the stack. If the stack has fewer than two entries, peekNxt throws an exception. Write a linkedimplementation of a stack class call LinkedStack.java that includes a method peekNxt.
Please make sure that this is included in peekNxt: If the stack has fewer than two entries, peekNxt throws an exception and if you could please make a main to execute peekNxt, thank you.There are 2 class, 1 with the peekNxt, and 1 for implementation. You only need to work on the first one with the peekNxt in it
import java.util.EmptyStackException;import java.util.NoSuchElementException;public final class LinkedStack<T> implements StackInterface<T>{private Node topNode;…
Create a TestStack project. Add a Stack class with all of the methods implemented and commented (constructor, push, pop, is empty, clear, peek, toString).
Create a TestStack class with main(). main() should use your Stack class to do one of the following:
OPTION A) Use your stack class to reverse a user inputted string. A palindrome is a word that reads the same backwards as it does forwards. For example, madam is a palindrome, hello is not. Use your stack class to test if an input string is a palindrone or not.
OPTION B)
Use your new Stack to add a function that checks for balanced parenthese in an equation. Use these three equations, plus one of your own to test your code.
((3^2 + 8)*(5/2))/(2+6)
((3^2 + 8)*(5/2))/(2+6))
(((3^2 + 8)*(5/2)/(2+6)
When encountering a left parenthese, push it on the stack. Pop one off when you encounter a right parenthese. Return true or false depending if parentheses are balanced or not (stack is empty after last right parenthese causes a…
Stack:#ifndef STACKTYPE_H_INCLUDED#define STACKTYPE_H_INCLUDEDconst int MAX_ITEMS = 5;class FullStack// Exception class thrown// by Push when stack is full.{};class EmptyStack// Exception class thrown// by Pop and Top when stack is emtpy.{};template <class ItemType>class StackType{public:StackType();bool IsFull();bool IsEmpty();void Push(ItemType);void Pop();ItemType Top();private:int top;ItemType items[MAX_ITEMS];};#endif // STACKTYPE_H_INCLUDED
Queue:#ifndef QUETYPE_H_INCLUDED#define QUETYPE_H_INCLUDEDtemplate<class T>class QueType{public:QueType();QueType(int);~QueType();void MakeEmpty();bool IsEmpty();bool IsFull();void Enqueue(T);void Dequeue(T&);T Front();private:int front;int rear;T *info;int maxQue;};#endif // QUETYPE_H_INCLUDED
Chapter 16 Solutions
Problem Solving with C++ (10th Edition)
Ch. 16.1 - Prob. 1STECh. 16.1 - What would be the output produced by the code in...Ch. 16.1 - Prob. 3STECh. 16.1 - What happens when a throw statement is executed?...Ch. 16.1 - In the code given in Self-Test Exercise 1, what is...Ch. 16.1 - Prob. 6STECh. 16.1 - Prob. 7STECh. 16.1 - What is the output produced by the following...Ch. 16.1 - What is the output produced by the program in...Ch. 16.2 - Prob. 10STE
Knowledge Booster
Similar questions
- In the lecture we studied IntStack, a class that implements a static stack of integers. Write a template that will create a static stack of any data type. Demonstrate the class with a driver program. #pragma once class IntStack{private: int *stackArray; int stackSize; int top; public: IntStack(int size); void push(int num); void pop(int &num); bool isFull(); bool isEmpty();}; Queue Exceptions: Modify the static queue class provided in our lecture as follows. Make the isFull and isEmpty member functions private. Define a queue overflow exception and modify enqueue so that it throws this exception when the queue runs out of space. Define a queue underflow exception and modify dequeue so that it throws this exception when the queue is empty. Rewrite the main program so that it catches overflow exceptions when they occur. The exception handler for queue overflow should print an appropriate error message and then terminate the program. #include…arrow_forwardIn C++, Write a program which is menudriven ( i) Push, ii) POP, iii) Peek iv) Print). It will create a stack ofinteger type data. You have to implement the following operations.a. Push (Insert a data to the stack)b. Pop (Print the data at the TOP and remove thedata)c. Peek (Print the data at the TOP)d. Print (Print the whole stack)arrow_forwardA Stack is a data structure for storing a list of elements in a LIFO (last in, first out) fashion. Design a class called Stack with three methods. void Push(object obj)object Pop()void Clear() We should be able to use this stack class as follows:var stack = new Stack();stack.Push(1);stack.Push(2);stack.Push(3);Console.WriteLine(stack.Pop());Console.WriteLine(stack.Pop());Console.WriteLine(stack.Pop()); The output should be 3, 2, 1arrow_forward
- 3. Define a function named stackToQueue. This function expects a stack as an argument. The function builds and returns an instance of LinkedQueue that contains the items in the stack. The function assumes that the stack has the interface described in Chapter 7, "Stacks." The function's postconditions are that the stack is left in the same state as it was before the function was called, and that the queue's front item is the one at the top of the stack. Use this Python template: class Queue:'''TODO: Remove the "pass" statements and implement each methodAdd any methods if necesssaryDON'T use any builtin queue class to store your items'''def __init__(self): # Constructor functionpassdef isEmpty(self): # Returns True if the queue is empty or False otherwisepassdef len(self): # Returns the number of items in the queuepassdef peek(self): # Returns the item at the front of the queuepassdef add(self, item): # Adds item to the rear of the queuepassdef pop(self): # Removes and returns the item…arrow_forwardThe CopyTo method copies the contents of a stack into an array. The arraymust be of type Object since that is the data type of all stack objects. Themethod takes two arguments: an array and the starting array index to beginplacing stack elements. The elements are copied in LIFO order, as if they werepopped from the stack. write a short code fragment demonstrating a CopyTomethod call:arrow_forwardThe ADT stack lets you peek at its top entry without removing it. For some applications of stacks, you also need to peek at the entry beneath the top entry without removing it. We will call such an operation peekNxt. If the stack has more than one entry, peekNxt returns the second entry from the top without altering the stack. If the stack has fewer than two entries, peekNxt throws an exception. Write a linked implementation of a stack class call LinkedStack.java that includes a method peekNxt. Implement pimport java.util.EmptyStackException;import java.util.NoSuchElementException;public final class LinkedStack<T> implements StackInterface<T>{private Node topNode; public YourFirst_YourLast_LinkedStack(){topNode = null;}public void push(T newEntry){Node newNode = new Node(newEntry, topNode);topNode = newNode;} // end pushpublic T peek(){if (isEmpty())throw new EmptyStackException();elsereturn topNode.getData();} // end peekpublic T peekNxt() // Code here{ }…arrow_forward
- Suppose the following operations were performed on an empty stack:pus h(8);pus h(?);pop();push (19);pus h(21);pop();Insert numbers in the following diagram to show what will be stored in the staticstack after the operations have executedarrow_forwardjava /* Practice Stacks and ourVector Write a java program that creates a stack of integers. Fill the stack with 30 random numbers between -300 and +300. A)- With the help of one ourVector Object and an additional stack, reorganize the numbers in the stack so that numbers smaller than -100 go to the bottom of the stack, numbers between -100 and +100 in the middle and numbers larger than +100 in the top (order does not matter) B)- (a little harder) Solve the same problem using only one ourVector object for help C)- (harder) Solve the same problem using only one additional stack as a helper */ public class HWStacks { public static void main(String[] args) { // TODO Auto-generated method stub } }arrow_forwardImplement a program in C++ that has the following three parts and each does the following: Implements in C++ a Stack ADT (save the file as StackADT.h) Implements all the necessary methods (behavior) of a Stack (save the file as StackADT.cpp) Implements in C++ a main function (the client or application program) that creates and uses Stack objects (save the file as StackMain.cpp). This main function should be able to do the following: It should be able to read a given text file as input, It should be able to get each input value from the input file (one word at a time) and store it (push) on the Stack object until it stores all the words from the input file. It should be able to retrieve an item from the stack and display it on the screen, and then delete (pop) that item from the stack, and repeat until it retrieves all the items from the stack object. NOTE: Please make sure you debug, run, test and see the program working correctly before submitting. Submit the files…arrow_forward
- For the following problems, you need to submit a python code that performs the required functions. Q1: (Stack & Queue) 1. Write a program that utilizes the LinkedStack class and LinkedQueue class to perform the following function. Input :empty stack and a Queue Required: reverse the order of the queue. // you must use the methods of the stack and queue class // Q2) Write a member functions to the class ( CircularQueue ) that performs the followings: 1. Sum-even(CQ) : Print the sum of all even numbers stored in CircularQueue. 2. Perform the followings: a. Create two circular linked lists ( CQ1, CQ2). b. Merge the two circular linked lists in one →CQ1. //Remember: you need to use the class ( CircularQueue ) methods.//arrow_forwardImplement a method transfer in class LinkedStack. This method should transfer all elements of a stack sourceS to another stack targetS so that the element that starts at the top of sourceS is the first one to be inserted in targetS, and the element at the bottom of sourceS ends up at the top of targetS. The operation should result in sourceS being an empty stack. Test this method in the main method of LinkedStack. package stacks;public class LinkedStack<E> implements Stack<E> {/** The primary storage for elements of the stack */private SinglyLinkedList<E> list = new SinglyLinkedList<>(); // an empty list/** Constructs an initially empty stack. */public LinkedStack() { } // new stack relies on the initially empty list@Overridepublic int size() { return list.size(); }@Overridepublic boolean isEmpty() { return list.isEmpty(); }@Overridepublic void push(E element) { list.addFirst(element); }@Overridepublic E top() { return list.first(); }@Overridepublic E pop() {…arrow_forwardThe ADT stack lets you peek at its top entry without removing it. For some applications of stacks, you also need to peek at the entry beneath the top entry without removing it. We will call such an operation peek2. If the stack has more than one entry, peek2 returns the second entry from the top without altering the stack. If the stack has fewer than two entries, peek2 throws an exception. Write a linked implementation of a stack class call First_Last_LinkStack.java that includes a method peek2arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
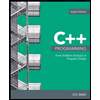
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning