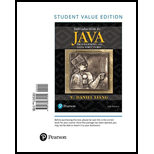
(Create a text file) Write a

Create a text file
Program Plan:
- Declare the class “writeText”.
- Definition for main class.
- Create an object to “PrintWriter” to create a new file named “Exercise17_01.txt”.
- For loop to iterate till 100.
- Write the 100 randomly generated integers to the file.
Program to create a file named “Exercise17_01.txt” to write 100 integers randomly to the created text file.
Explanation of Solution
Program:
//Import required packages
import java.io.*;
import java.util.*;
//Definition for class "writeText"
public class WriteText {
// Definition for main class
public static void main(String[] args) throws IOException {
// Try block
try (
/* Create an object for printWriter to write output to the text file */
PrintWriter out = new PrintWriter(new FileOutputStream(
"Exercise17_01.txt", true));)
{
// Loop to iterate
for (int j = 0; j < 100; j++)
// Generate the random number
out.print((int) (Math.random() * 100000) + " ");
}
}
}
Screenshot of the file “Exercise17_01.txt”
Want to see more full solutions like this?
Chapter 17 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version, Student Value Edition (11th Edition)
Additional Engineering Textbook Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
C++ How to Program (10th Edition)
Artificial Intelligence: A Modern Approach
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Java How To Program (Early Objects)
- Show two different ways to reset the file position pointer to the beginning of the filearrow_forwardHow do you check for end of file when reading an input file using INT 21h Function 3Fh?arrow_forwarda. What happens if you try to move the file marker past the end of a file? b. What is returned if subsequent calls are made to read the file?arrow_forward
- What classes do you use to write output to a binary file? What classes do you use to read from a binary file?arrow_forwardWrite code that does the following: opens an output file with the filename number_list.txt, uses a loop to write the numbers 1 through 100 to the file, and then closes the file.arrow_forwardHow do you parse through this input file? C++arrow_forward
- What is the import file suposed to be?arrow_forwardUsing C++, write a fragment of code to open a file called random.txt. If the file does not exist, create and open it for both input and output. Then, write 10 consecutive random numbers in the range of 1 to 10 into the filearrow_forwardWhen you say a file is indexed, do you mean it has an index directory that allows for random access?arrow_forward
- State which of the following are true and which are false. If false, explain why.d) If the file position pointer points to a location in a sequential file other than the beginningof the file, the file must be closed and reopened to read from the beginning of the file.arrow_forwardHow do I seperate the parts of the code into different files properly?arrow_forwardHow do I write a code that will open the text file for reading, (test.txt), and that prints the first line of the file?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
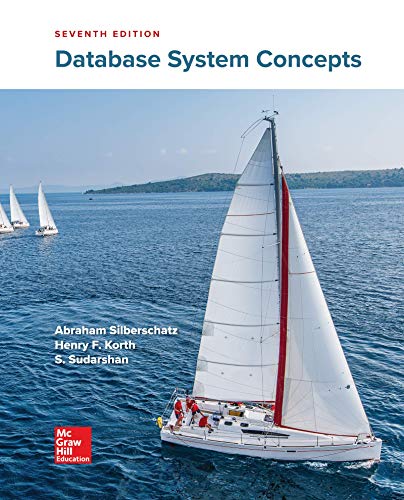
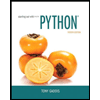
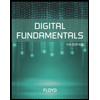
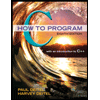
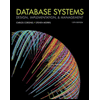
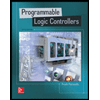