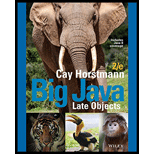
Big Java Late Objects
2nd Edition
ISBN: 9781119330455
Author: Horstmann
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 17, Problem 7PE
Program Plan Intro
Adding methods to Binary Tree
Program plan:
BinaryTree.java:
- Define the class “BinaryTree.java”.
- Declare the private variable root from class “Node”.
- Defining the constructor “BinaryTree” to generate an empty tree.
- Assign root to null.
- Define the parameterized constructor “BinaryTree(object rootData)” to generate a tree one root element.
- Generate root node.
- Define the parameterized constructor “public BinaryTree(Object rootData, BinaryTree left, BinaryTree right)”.
- Generates root and children nodes.
- Define the “preorder()” method for both tree with many nodes and a tree with one node.
- For a tree with one node and no children,
- “preorder(root,v)” is called.
- For a tree with multiple nodes,
- If (node == null) returns v.visit(node.data);
- If (node.left != null) then, preorder(node.left, v);
- If (node.right != null) then, preorder(node.right, v);
- For a tree with multiple nodes,
- “preorder(root,v)” is called.
- For a tree with one node and no children,
- Define the “inorder()” method for both tree with many nodes and a tree with one node.
- For a tree with one node and no children,
- “inorder(root,v)” is called.
- For a tree with multiple nodes,
- If (node.left != null) then, inorder(node.left, v) and v.visit(node.data);
- If (node.right != null) then, inorder(node.right, v);
- For a tree with multiple nodes,
- “inorder(root,v)” is called.
- For a tree with one node and no children,
- Define the “postorder()” method for both tree with many nodes and a tree with one node.
- For a tree with one node and no children,
- “postorder(root,v)” is called.
- For a tree with multiple nodes,
- If (node.left != null) then, postorder(v, node.left);
- If (node.right != null) then, postorder(v, node.right) and v.visit(node.data);
- For a tree with multiple nodes,
- “postorder(root,v)” is called.
- For a tree with one node and no children,
- Define the class “Node”.
- Declare “object data”.
- Declare “Node left”.
- Declare “Node right”.
- Define the function static “height()”
- If (n == null) returns the null value.
- Otherwise returns the value 1 + Math.max(height(n.left), height(n.right)).
- Define the function “height()”
- Returns the height of this tree.
- Define “isEmpty()” function:
- It checks whether this tree is empty.
- Return true value if this tree is empty.
- Define the “data()” function.
- Gets the data at the root of this tree.
- Returns the root data.
- Define “left()” and “right()” function.
- “left()” function return the left child of the root.
- “right()” function return the right child of the root.
Visitor.java:
- Define interface “Visitor”
- Calls the “visit(object data)”
BinaryTreeTester.java:
- Import the required header files.
- Define “BinaryTreeTester” class.
- Define the “main()” function.
- Create an object “names” for “BinaryTree” with tree values.
- Create an object “visit” for “Visitor” object and print the data.
- Call “inorder()”, “preoder()” and “postorder()” function.
- Define the “main()” function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
Big Java Late Objects
Ch. 17.1 - Prob. 1SCCh. 17.1 - Prob. 2SCCh. 17.1 - Prob. 3SCCh. 17.1 - Prob. 4SCCh. 17.1 - Prob. 5SCCh. 17.1 - Prob. 6SCCh. 17.1 - Prob. 7SCCh. 17.2 - Prob. 8SCCh. 17.2 - Prob. 9SCCh. 17.2 - Prob. 10SC
Ch. 17.2 - Prob. 11SCCh. 17.2 - Prob. 12SCCh. 17.3 - Prob. 13SCCh. 17.3 - Prob. 14SCCh. 17.3 - Prob. 15SCCh. 17.3 - Prob. 16SCCh. 17.3 - Prob. 17SCCh. 17.3 - Prob. 18SCCh. 17.4 - Prob. 19SCCh. 17.4 - Prob. 20SCCh. 17.4 - Prob. 21SCCh. 17.4 - Prob. 22SCCh. 17.4 - Prob. 23SCCh. 17.4 - Prob. 24SCCh. 17.5 - Prob. 25SCCh. 17.5 - Prob. 26SCCh. 17.5 - Prob. 27SCCh. 17.5 - Prob. 28SCCh. 17.5 - Prob. 29SCCh. 17.5 - Prob. 30SCCh. 17.6 - Prob. 31SCCh. 17.6 - Prob. 32SCCh. 17.6 - Prob. 33SCCh. 17.6 - Prob. 34SCCh. 17.6 - Prob. 35SCCh. 17.7 - Prob. 36SCCh. 17.7 - Prob. 37SCCh. 17.7 - Prob. 38SCCh. 17.7 - Prob. 39SCCh. 17.7 - Prob. 40SCCh. 17 - Prob. 1RECh. 17 - Prob. 2RECh. 17 - Prob. 3RECh. 17 - Prob. 4RECh. 17 - Prob. 5RECh. 17 - Prob. 6RECh. 17 - Prob. 7RECh. 17 - Prob. 8RECh. 17 - Prob. 9RECh. 17 - Prob. 10RECh. 17 - Prob. 11RECh. 17 - Prob. 12RECh. 17 - Prob. 13RECh. 17 - Prob. 14RECh. 17 - Prob. 16RECh. 17 - Prob. 18RECh. 17 - Prob. 19RECh. 17 - Prob. 20RECh. 17 - Prob. 21RECh. 17 - Prob. 22RECh. 17 - Prob. 23RECh. 17 - Prob. 24RECh. 17 - Prob. 25RECh. 17 - Prob. 26RECh. 17 - Prob. 27RECh. 17 - Prob. 28RECh. 17 - Prob. 1PECh. 17 - Prob. 2PECh. 17 - Prob. 3PECh. 17 - Prob. 4PECh. 17 - Prob. 5PECh. 17 - Prob. 6PECh. 17 - Prob. 7PECh. 17 - Prob. 8PECh. 17 - Prob. 9PECh. 17 - Prob. 10PECh. 17 - Prob. 11PECh. 17 - Prob. 12PECh. 17 - Prob. 13PECh. 17 - Prob. 1PPCh. 17 - Prob. 2PPCh. 17 - Prob. 3PPCh. 17 - Prob. 4PPCh. 17 - Prob. 5PPCh. 17 - Prob. 6PPCh. 17 - Prob. 7PPCh. 17 - Prob. 8PPCh. 17 - Prob. 9PPCh. 17 - Prob. 10PPCh. 17 - Prob. 11PP
Knowledge Booster
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
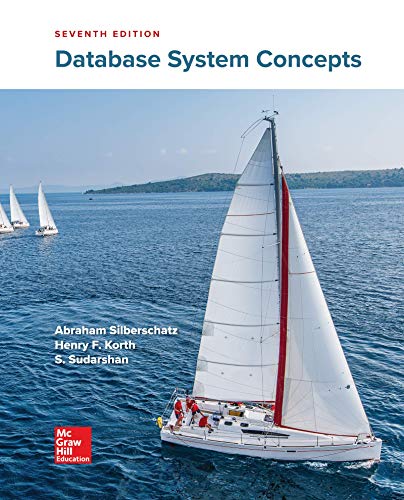
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
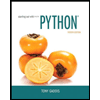
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
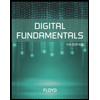
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
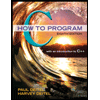
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
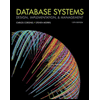
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
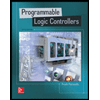
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education