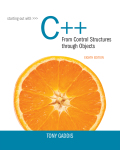
EBK STARTING OUT WITH C++
8th Edition
ISBN: 8220100794438
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 11PC
Program Plan Intro
File Compare
Program Plan:
Main.cpp:
- Include required header files
- Inside “main ()” function,
- Create an object “ifile” for input file stream
- Create an object “ofile” for output file stream.
- Create two class templates “q1” and “q2” to hold characters
- Declare variables “char1” and “char2”
- Till the end of file,
- Enqueue a character from file1.
- Till the end of file,
- Enqueue a character from file2.
- Close both the files.
- Assign “true” to a Boolean variable “status”.
- Do until both the queue becomes empty,
- Dequeue a character from 1st queue “q1”.
- Dequeue a character from 2nd queue “q2”.
- Check if both the characters are not equal.
- If the condition is true then, assign “false” to the Boolean variable.
- Check if the status is true,
- If the condition is true then, print “The files are identical”.
- If the condition is not true then, print “The files are not identical”.
Dynqueue.h:
- Include required header files.
- Create template class
- Declare a class named “Dynqueue”. Inside the class,
- Inside the “private” access specifier,
- Create a structure named “QueueNode”.
- Create an object for the template
- Create a pointer named “next”.
- Create two pointers named “front” and “rear”.
- Declare a variable.
- Create a structure named “QueueNode”.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Declare the functions “enqueue ()”, “dequeue ()”, “isEmpty ()”, “isFull ()”, and “clear ()”.
- Inside the “private” access specifier,
- Declare template class.
- Give definition for the constructor.
- Assign the values.
- Declare template class.
- Give definition for the destructor.
- Call the function “clear ()”.
- Declare template class.
- Give function definition for “enqueue ()”.
- Make the pointer “newNode” as null.
- Assign “num” to newNode->value.
- Make newNode->next as null.
- Check whether the queue is empty using “isEmpty ()” function.
- If the condition is true then, assign newNode to “front” and “rear”.
- If the condition is not true then,
- Assign newNode to rear->next
- Assign newNode to “rear”.
- Increment the variable “numItems”.
- Declare template class.
- Give function definition for “dequeue ()”.
- Assign temp pointer as null.
- Check if the queue is empty using “isEmpty ()” function.
- If the condition is true then print “The queue is empty”.
- If the condition is not true then,
- Assign the value of front to the variable “num”.
- Make front->next as “temp”.
- Delete the front value
- Make temp as front.
- Decrement the variable “numItems”.
- Declare template class.
- Give function definition for “isEmpty ()”.
- Assign “true” to a Boolean variable
- Check if “numItems” is true.
- If the condition is true then assign “false” to the variable.
- Return the Boolean variable.
- Declare template class.
- Give function definition for “clear ()”.
- Create an object for template.
- Dequeue values from queue till the queue becomes empty using “while” condition.
- Create an object for template.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Program Description:in C language
The project is a student management system which maintains student records in a simple text file.
Your task is to write a program to save a list of students records in a FILE and then perform several operations on this FILE.
Your program will ask user to choose the option from a menu.
The 3 major function in menu are: ADD STUDENT
FIND STUDENT
PRINT LIST
Exit
How it Works
The menu is handled by do while and switch statement.
Sample output
The Methods to be implemented are as follows:
1. ADDSTUDENT
This method will add a new student to the file.
It takes 3 parameters ( student name, id, and GPA).
Your program must ask the user to enter the details of new students: Name, ID and GPA
2. FINDSTUDENT
This method will search for a student with his/her id in the file.
If the student is found it will print her/his record to the output.
If the student is not found it will print “There is no record of this student in this system”
3. PRINTLIST
This method will…
File System: It is highly useful in file system handling where for example
the file allocation table contains a sequential list of locations where the files
is split up and stored on a disk. Remember that overtime it is hard for an
OS to find disk space to cover the entire file so it usually splits these up into
chunks across the physical hard drive and stores a sequential list of links
together as a linked list.
Write an algorithm for the above problem and analyse the efficiency of
the algorithm.
C Programming Language
Task: Deviation
Write a program that prompts the user to enter N numbers and calculates which of the numbers has
the largest deviation from the average of all numbers.
You program should first prompt the user to enter how many numbers that will specify. The program
should then scan for each number, separated by a newline. You should calculate the average value
and return the number from the list which is furthest away from this average (to 2dp).
Try using dynamic memory functions to store the incoming array of numbers on the heap.
Code to build from:
+
1 #include
2 #include
3
4 int main(void) {
5
6}
7
Output Example:
deviation.c
How many numbers?
5
Enter them:
1.0
2.0
6.0
3.0
4.0
Average: 3.20
Largest deviation from average: 6.00
Chapter 18 Solutions
EBK STARTING OUT WITH C++
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - The STL stack is considered a container adapter....
Ch. 18 - What types may the STL stack be based on? By...Ch. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - The STL stack container is an adapter for the...Ch. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - Prob. 26RQECh. 18 - Write two different code segments that may be used...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Dynamic String Stack Design a class that stores...Ch. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Inventory Bin Stack Design an inventory class that...Ch. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- Program Specification For this assignment you will write a program to help people record the events of their day by supplying prompts and then saving their responses along with the question and the date to a file. Functional Requirements This program must contain the following features: Write a new entry - Show the user a random prompt (from a list that you create), and save their response, the prompt, and the date as an Entry. Display the journal - Iterate through all entries in the journal and display them to the screen. Save the journal to a file - Prompt the user for a filename and then save the current journal (the complete list of entries) to that file location. Load the journal from a file - Prompt the user for a filename and then load the journal (a complete list of entries) from that file. This should replace any entries currently stored the journal. Provide a menu that allows the user choose these options Your list of prompts must contain at least five different prompts.…arrow_forward# Method: Load levelsList using the data in levelsFile def readLevelsFromFile(self): try: # Set levelsList to an empty list pass # Open the file pass # Use a loop to read through the file line by line pass # This code is inside the loop: # Convert the line to a float and then append it to levelsList pass # Close the file pass except: returnarrow_forwardComputer Science JAVA #7 - program that reads the file named randomPeople.txt sort all the names alphabetically by last name write all the unique names to a file named namesList.txt , there should be no repeatsarrow_forward
- Lab Exercise #6: Product Price List In this activity, you are to create a program that will maintain a list of products and their prices. The program is a menu- driven program that has choices for adding a new product and its price, listing all products with corresponding prices. updating the price of a product, and removing a product. A sample execution is given below: [U]pdate | [L]ist | [E]xit Menu: [A]dd| [R]emove Option: A Product: Notebook Price (Php): 15.00 Option: A Product: Pen Price (Php): 10.00 Option: A Product: notebook *The product already exists* Option: U Product: notebook Price (Php): 18.20 Option: A Product: Pencil Price (Php): 8.50 Option: L NOTEBOOK PEN PENCIL Option: R Product: Pen 18.20 10.00 8.50 Option: R Product: PEN *The product does not exist* When adding an item, the product name and its price will be given. Product names are unique, case-insensitive, and with leading and trailing spaces removed. Therefore, adding the same product more than once is not…arrow_forwardPython: 2048 games Board = list[list[str]]# Checks whether a given board has any# possible move left. If no more moves,# return True. Otherwise return False.def isGameOver(board: Board) -> bool:return False# Returns a tuple (changed, new_board)# where:# changed - a boolean indicating if# the board has changed.# new_board - the board after the user# presses the 'Up' key.def doKeyUp(board: Board) -> tuple[bool, Board]:return False, board# Returns a tuple (changed, new_board)# where:# changed - a boolean indicating if# the board has changed.# new_board - the board after the user# presses the 'Down' key.def doKeyDown(board: Board) -> tuple[bool, Board]:return False, board# Returns a tuple (changed, new_board)# where:# changed - a boolean indicating if# the board has changed.# new_board - the board after the user# presses the 'Left' key.def doKeyLeft(board: Board) -> tuple[bool, Board]:return False, board# Returns a tuple (changed, new_board)# where:# changed - a boolean…arrow_forwardCreate a pseudocode for the Queue Operations. The pseudocode should accept N values, checks if the queue is full or empty and display messages indicating if the Queue is empty or full. It should also print the final contents.arrow_forward
- CENGAGE MINDTAP Programming Exercise 9-2A Instructions The mean of a list of numbers is its arithmetic average. The median of a list is its middle value when the values are placed in order. For example, if an ordered list contains 1, 2, 3, 4, 5, 6, 10, 11, and 12, then the mean is 6, and their median is 5. Write an application that allows you to enter nine integers and displays the values, their mean, and their median. An example of the program is shown below: Enter number 6 12 Enter number 7 14 Enter number 8 16 Enter number 9 18 You entered: 2, 4, 6, 8, 10, 12, 14, 16, 18 The mean is 10.0 and the median is 10 !!arrow_forwardCharge Account Validation Write a program that lets the user enter a charge account number. The program should determine if the number is valid by checking for it in the following list: 5658845 4520125 7895122 8777541 8451277 1302850 8080152 4562555 5552012 5050552 7825877 1250255 1005231 6545231 3852085 7576651 7881200 4581002 The list of numbers above should be initialized in a single-dimensional array. A simple binary search should be used to locate the number entered by the user. If the user enters a number that is in the array, the program should display a message saying that the number is valid along with index. If the user enters a number that is not in the array, the program should display a message indicating that the number is invalid. Note: values should be positive integers.arrow_forwardII. Create a pseudocode for the Queue Operations. The pseudocode should accept N values, checks if the queue is full or empty and display messages indicating if the Queue is empty or full. It should also print the final contents.arrow_forward
- A for statement is a loop that iterates across a list of items. As a result, it continues to operate as long as there are items to process. Is this statement true or false?arrow_forwardsubject: microprocesor and assembly language Write a program that displays a string in all possible combinations of foreground and background colors (16 x 16 =256). The colors are numbered from 0 to 15, so you can use a nested loop to generate all possiblecombinations.arrow_forwardPYTHON Language Programming Write a program that asks user for state name in a text document "state.txt" Please eneter a state: Georgia Georgia has been added to the list Enter another name(Y/N): Y Please enter a state: California New York is already in the list Enter another name (Y/N): N Here are the states in the file: California and Georgiaarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage