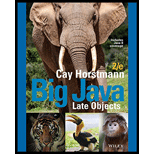
Big Java Late Objects
2nd Edition
ISBN: 9781119330455
Author: Horstmann
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 13PE
Program Plan Intro
Find the minimum in a Heap
Program plan:
Filename: “MinHeap.java”
This code snippet creates a class “MinHeap”. In the code,
- Import the required packages.
- Define a class “MinHeap”.
- Create an array list “elements”.
- Define the constructor “MinHeap()”.
- Define the array list “elements”.
- Initialize “elements” with “null”.
- Define a method “add()”.
- Add a new leaf using “add()”.
- Declare a variable “index” and set the value to it.
- Iterate a “while” loop,
- Set elements to “elements” using method “set()”.
- Get the parent index of “index”.
- Set the “newElement” to “index” using “set()”.
- Define a method “peak()” of type “E”.
- Get the first element of “elements” and return the value.
- Define a method “remove()”.
- Get the first element of “elements” to “minimum”.
- Define the “lastIndex”.
- Get the element at “lastIndex” to “last”.
- If “lastIndex” is greater than 1,
- Add element “last” at index 1.
- Call the method “fixHeap()”.
- Return “minimum”.
- Define a method “fixHeap()”,
- Get the first element to “root”.
- Define the variable “lastIndext” by setting last index of “elements”.
- Set “index” equal to 1.
- Declare a Boolean variable “true”.
- Iterate a “while” loop,
- Call the method “getLeftChildIndex()” on “index” and set the value to “childIndex”.
- If “childIndex” is less than or equal to “lastIndex”,
- Call “getIndexChild()” of “index” to “child”.
- If the condition is true,
-
- Call “getRightChildIndex()” on “index” and set the result value to “childIndex”.
- Call the method “getRightChild()” on “index” and set the value to “child”.
- If the condition is true,
-
- Set “child” at “index”.
- Set “childIndex” to “index”.
- Else,
-
- Set “false” to “more”.
- Else,
- Set “false” to “more”.
-
-
- Set “root” to “index” using “set”.
-
- Define a method “empty()”,
- Return “true” if “size” of “element” equal to 1,
- Define the method “getLeftChildIndex()”,
- Return the index.
- Define the method “getRightChildIndex()”,
- Return the index.
- Define the method “getParentIndex()”,
- Return the index.
- Define the method “getLeftChild()”,
- Return the element.
- Define the method “getRightChild()”,
- Return the element.
- Define the method “getParent()”,
- Return the element.
Filename: “MinHeapTester.java”
This code snippet creates a class “MinHeapTester”. In the code,
- Import the required packages.
- Define a class “MinHeapTester”.
- Define the “main” method.
- Declare a “MinHeap” named “heap”.
- Add elements to “heap” using “add()” method.
- Remove an element from “heap” and store it in a string variable “top”.
- Print the value of “top”.
- Print the expected value of “top”.
- Remove an element from “heap” and store it in a string variable “top”.
- Print the value of “top”.
- Print the expected value of “top”.
- Remove an element from “heap” and store it in a string variable “top”.
- Print the value of “top”.
- Print the expected value of “top”.
- Define the “main” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Chapter 18 Solutions
Big Java Late Objects
Ch. 18.1 - Prob. 1SCCh. 18.1 - Prob. 2SCCh. 18.1 - Prob. 3SCCh. 18.1 - Prob. 4SCCh. 18.1 - Prob. 5SCCh. 18.2 - Prob. 6SCCh. 18.2 - Prob. 7SCCh. 18.2 - Prob. 8SCCh. 18.2 - Prob. 9SCCh. 18.2 - Prob. 10SC
Ch. 18.3 - Prob. 11SCCh. 18.3 - Prob. 12SCCh. 18.3 - Prob. 13SCCh. 18.3 - Prob. 14SCCh. 18.3 - Prob. 15SCCh. 18.4 - Prob. 16SCCh. 18.4 - Prob. 17SCCh. 18.4 - Prob. 18SCCh. 18.4 - Prob. 19SCCh. 18.4 - Prob. 20SCCh. 18.4 - Prob. 21SCCh. 18.5 - Prob. 22SCCh. 18.5 - Prob. 23SCCh. 18.5 - Prob. 24SCCh. 18.5 - Prob. 25SCCh. 18.5 - Prob. 26SCCh. 18.5 - Prob. 27SCCh. 18 - Prob. 1RECh. 18 - Prob. 2RECh. 18 - Prob. 3RECh. 18 - Prob. 4RECh. 18 - Prob. 5RECh. 18 - Prob. 6RECh. 18 - Prob. 7RECh. 18 - Prob. 8RECh. 18 - Prob. 9RECh. 18 - Prob. 10RECh. 18 - Prob. 11RECh. 18 - Prob. 12RECh. 18 - Prob. 13RECh. 18 - Prob. 14RECh. 18 - Prob. 1PECh. 18 - Prob. 2PECh. 18 - Prob. 3PECh. 18 - Prob. 4PECh. 18 - Prob. 5PECh. 18 - Prob. 6PECh. 18 - Prob. 7PECh. 18 - Prob. 8PECh. 18 - Prob. 9PECh. 18 - Prob. 10PECh. 18 - Prob. 11PECh. 18 - Prob. 12PECh. 18 - Prob. 13PECh. 18 - Prob. 14PECh. 18 - Prob. 15PECh. 18 - Prob. 16PECh. 18 - Prob. 17PECh. 18 - Prob. 18PECh. 18 - Prob. 19PECh. 18 - Prob. 20PECh. 18 - Prob. 21PECh. 18 - Prob. 22PECh. 18 - Prob. 1PPCh. 18 - Prob. 2PPCh. 18 - Prob. 3PPCh. 18 - Prob. 4PPCh. 18 - Prob. 5PPCh. 18 - Prob. 6PPCh. 18 - Prob. 7PPCh. 18 - Prob. 8PP
Knowledge Booster
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
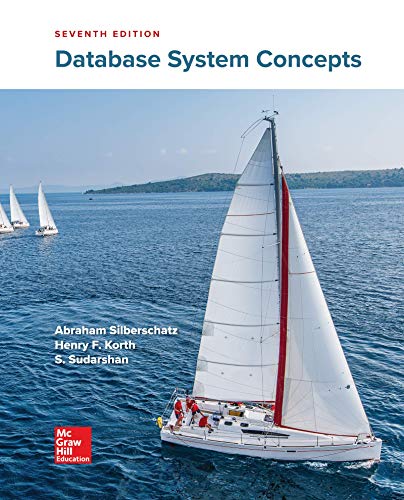
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
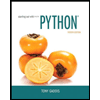
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
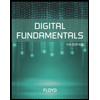
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
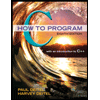
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
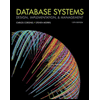
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
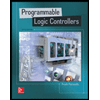
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education