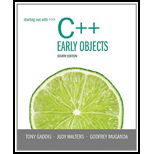
Starting Out with C++: Early Objects
8th Edition
ISBN: 9780133360929
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 7PC
Program Plan Intro
Queue Exceptions
“main.cpp”:
- This program features queues that can throw exceptions and print an appropriate error message and should terminate the program.
- Include the required header files.
- Prompt the user to enqueue 5 items.
- Use of try – catch exceptional handling that returns error message and terminates the program if the queue overflows.
- Prompt the user and allow user option to overflow the queue and dequeue the queue and print the values present in the queue.
“IntQueue.h”:
- Include all the required header files.
- Declare all the variables present in the queue along with their data types.
- Declare all the function definition.
- Declare the class underflow and overflow to check for exceptional handling in queue.
“IntQueue.cpp”:
- Include all the required header files.
- Call a constructor to create a queue and initialize its elements.
- Initialize front and rear each to -1 and the number of elements initially present to 0.
- A destructor is called to delete the queue array.
- Define a queue function enqueue that inserts the value in variable num at the rear of the queue.
- Define a queue function dequeue that deletes the value at the front of the queue, and copies it into variable.
- Define a queue function isEmpty returns true if the queue is empty, and false if elements are present in the queue.
- Define a Queue function is Full return true if queue is full and false if queue is not full.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C# Programing Language
Given the code below
1.Change all of the low-level arrays in your program to use the appropriate C# collection class instead. Be sure to implement Deck as a Stack
2. Make sure that you use the correct type-safe generic designations throughout your program.
3.Write code that watches for that incorrect data and throws an appropriate exception if bad data is found.4. Write Properties instead of Getter and Setter methods.
no lamdas, no delegates, no LINQ, no eventsGo Fish
using System;
namespace GoFish{public enum Suit { Clubs, Diamonds, Hearts, Spades };public enum Rank { Ace, Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack, Queen, King };
// -----------------------------------------------------------------------------------------------------
public class Card{public Rank Rank { get; private set; }public Suit Suit { get; private set; }
public Card(Suit suit, Rank rank){this.Suit = suit;this.Rank = rank;}
public override string ToString(){return ("[" +…
stacks and queues.
program must be able to handle all test cases without causing an exception
Note that this problem does not require recursion to solve (though you can use that if you wish).
Queue Exceptions: Modify the static queue class provided in our lecture as follows.
Make the isFull and isEmpty member functions private.
Define a queue overflow exception and modify enqueue so that it throws this exception when the queue runs out of space.
Define a queue underflow exception and modify dequeue so that it throws this exception when the queue is empty.
Rewrite the main program so that it catches overflow exceptions when they occur. The exception handler for queue overflow should print an appropriate error message and then terminate the program.Here's the code so far:data.h:
#pragma once
class IntQueue{private:int *queueArray;int queueSize;int front;int rear;int numItems;public:IntQueue(int);~IntQueue();void enqueue(int);void dequeue(int &);bool isEmpty();bool isFull();void clear();};implementation:
#include <iostream>#include "Data.h"using namespace std;
//*************************// Constructor *//*************************IntQueue::IntQueue(int s){queueArray = new…
Chapter 18 Solutions
Starting Out with C++: Early Objects
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - The STL stack is considered a container adapter....Ch. 18 - What types may the STL stack be based on? By...
Ch. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PC
Knowledge Booster
Similar questions
- C++ Code for a QueueThe program should features a Queue class with insert(), remove(), peek(),isFull(), isEmpty(), and size() member functions.The main() program creates a queue of five cells, inserts four items, removes threeitems, and inserts four more. The sixth insertion invokes the wraparound feature. All the items are then removed and displayed. The output looks like this:40 50 60 70 80arrow_forwardFor the following problems, you need to submit a python code that performs the required functions. Q1: (Stack & Queue) 1. Write a program that utilizes the LinkedStack class and LinkedQueue class to perform the following function. Input :empty stack and a Queue Required: reverse the order of the queue. // you must use the methods of the stack and queue class // Q2) Write a member functions to the class ( CircularQueue ) that performs the followings: 1. Sum-even(CQ) : Print the sum of all even numbers stored in CircularQueue. 2. Perform the followings: a. Create two circular linked lists ( CQ1, CQ2). b. Merge the two circular linked lists in one →CQ1. //Remember: you need to use the class ( CircularQueue ) methods.//arrow_forwardThis file has syntax and/or logical errors. Determine the problem and fix the program. // Employee's salary should not be negative // Include stack trace when exception occurs using System; using static System.Console; class FDebugEleven03 { static void Main() { Employee emp = new Employee(); try { emp.IdNum = 234; emp.Salary = -12; } catch (NegativeSalaryException e) { WriteLine(e.Message); WriteLine(e.StackTrace); } } } public class NegativeSalaryException : { private static string msg = "Employee salary is negative."; public NegativeSalaryException() : base(msg) { } } public class Employee { private int idNum; private double salary; public int IdNum { get return idNum; set idNum = value; } public double Salary { get {…arrow_forward
- Language: C++ Note: Write both parts separately. Part a: Complete the above Priority Queue class. Write a driver to test it. Part b: Modify the above class to handle ‘N’ different priority levelsarrow_forwardWrite a C++ program to perform the Queue operation. Note:Use the following class template for Queue creation. template <class T>class Queue{ private: int front,rear; T *queue; int maxsize;}; Define the following function in the class Queue class Method name Description int isFull() The method is used to check whether the queue is full or not. void insert(T) The method is used to display the rear element in the queue (if the queue is stored with the element(s)). void deletion() The method is used to delete the front element from the queue. void atFront() The method is used to display the front element in the queue (if the queue is stored with the element(s)). void atRear() The method is used to add data to the rear end of the queue. void display() The method is used to display all the data in the queue. int isEmpty() The method is used to check whether the queue is empty or not. Input and Output Format:The first line of input…arrow_forwardC++ A queue is essentially a waiting list. It’s a sequence of elements with a front and a back. Elements can only be added to the back of the queue and they can only be removed from the front of the queue. Elements are kept in order so that the first element to enter the queue is the first one to leave it.arrow_forward
- c++ Write a client function that returns the back of a queue while leaving the queue unchanged. This function can call any of the methods of the ADT queue. It can also create new queues. The return type is ITemType, and it accepts a queue as a parameter.arrow_forwardIn C, members (fields) of different structures can have the same name. True Falsearrow_forwardfor c++ thank you Error Testing The DynIntStack and Dyn IntQueue classes shown in this chapter are abstract data types using a dynamic stack and dynamic queue, respectively. The classes do not cur- rently test for memory allocation errors. Modify the classes so they determine whether new nodes cannot be created by handling the bad_alloc exception. here is the extention.h file please use it #ifndef DYNINTQUEUE_H #define DYNINTQUEUE_H class DynIntQueue { private: // Structure for the queue nodes struct QueueNode { int value; // Value in a node QueueNode *next; // Pointer to the next node }; QueueNode *front; // The front of the queue QueueNode *rear; // The rear of the queue int numItems; // Number of items in the queue public: // Constructor DynIntQueue(); // Destructor ~DynIntQueue(); // Queue operations void enqueue(int); void dequeue(int &); bool isEmpty() const; bool isFull() const; void…arrow_forward
- Q1: Write a java application for the stack operations with Linked List. Q2: Write java program to take the order from the customer and display the ordered items and total payment. If customer is entering the wrong name or not following the order to enter the menu it has to display error messages. (Find in the sample code) Sample Output:arrow_forwardc++ a Queue Class that allows users to push strings or integer values into it. For example, when running the following code: SpecialQueue Q; Q.push("Albert"); Q.push("Jose"); Q.push(123); Q.pop(); Q.pop(); Q.pop(); The output should be: Albert Jose 123 Submit a TEXT file that contains your class. By class, I mean everything EXCEPT your main function. DO NOT SUBMIT YOUR MAIN().arrow_forwardConcurrent Server Programming TITLE: Quiz Game In this assignment you are asked to write a phyton system to support an online math contest. The contest consists of answering the maximum quantity of sums in 30 seconds. This is done in a concurrent way, which means, that there are three participants answering at the same time. The participant with most correct answers WINS. The jury is responsible in starting up the server, after that they wait for the clients. When the three clients are connected, they must send the message: READY to the server. When the server receives the three READY from the clients, the contest starts. At the end of the program, the server must show the results .Execution (SERVER) - Please provide me the complete code and solution to arrive to the results below.python3 MathinikServer 192.168.1.2 Connected192.168.1.2 is Anne192.168.1.4 Connected192.168.1.4 is Billy192.168.1.3 Connected192.168.1.3 is MarkMark is READYAnne is READYBilly is READYStarting Contest…Contest…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
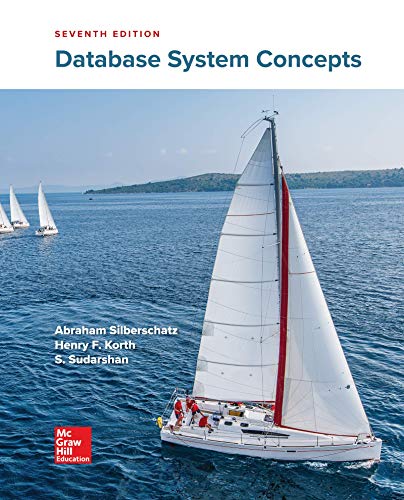
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
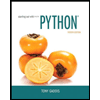
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
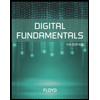
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
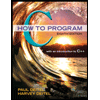
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
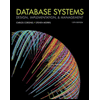
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
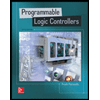
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education