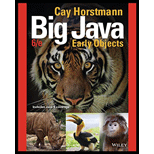
Big Java, Binder Ready Version: Early Objects
6th Edition
ISBN: 9781119056447
Author: Cay S. Horstmann
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 9PE
Program Plan Intro
HashSet
Program plan:
Filename: “HashSet.java”
This code snippet creates a class “HashSet”. In the code,
- Import the required packages.
- Define a class “HashSet”.
- Declare an array list “buckets”.
- Define an integer “size”.
- Define the constructor “HashSet()”.
- Define the array list “buckets”.
- Iterate a “for” loop,
- Add “null” to the “bucket”.
- Set “size” equal to “0”.
- Define a method “contains”.
- Define a variable “h” by calling “hashCode()” using “x”.
- If the value of “h” is less than 0,
- Set “h” equal to “-h”.
- Find the reminder of “h” and size of the “buckets”.
- Create a node “current” by getting element from “bucket”.
- Iterate a “while” loop,
- If the “data” part of node “current” is not equal to “x”.
- Return “true”.
-
- Set “current” equal to link of “current”.
- If the “data” part of node “current” is not equal to “x”.
- Return the value “false”.
- Define the method “add()”.
- Define a variable “h” by calling “hashCode()” using “x”.
- If the value of “h” is less than 0,
- Set “h” equal to “-h”.
- Find the reminder of “h” and size of the “buckets”.
- Create a node “current” by getting element from “bucket”.
- Iterate a “while” loop,
- If the “data” part of node “current” is not equal to “x”.
- Return “true”.
-
- Set “current” equal to link of “current”.
- If the “data” part of node “current” is not equal to “x”.
- Return the value “false”.
- Create a new node “newNode”.
- Set “data” of “newNode” equal to “x”.
- Set “next” of “newNode” equal to element at “h” of “bucket”.
- Set “newNode” at position “h” of the bucket.
- Increment the “size” by 1.
- Return “true”.
- Define the method “remove()”.
- Define a variable “h” by calling “hashCode()” using “x”.
- If the value of “h” is less than 0,
- Set “h” equal to “-h”.
- Find the reminder of “h” and size of the “buckets”.
- Create a node “previous”.
- Create a node “current” by getting element from “bucket”.
- Iterate a “while” loop,
- If the “data” part of node “current” is not equal to “x”.
- If “previous” equal to “null”,
-
- Set “next” of “current” to “h”.
- Else,
-
- Set the “next” of “current” to “next” of “previous”.
- Decrement “size” by 1.
- Return “true”.
-
-
- Set “previous” equal to “current”.
- Set “current.next” to “current”.
-
- If the “data” part of node “current” is not equal to “x”.
- Return “false”.
- Define the method “Iterator”.
- Create new “HashSetIterator” and return the value.
- Define the method “size()”.
- Return “size”.
- Define a class “Node”.
- Create a variable “data” of type “E”.
- Create a variable “next”.
- Define a class “HashSetIterator”.
- Declare the class members.
- Define the constructor “HashSetIterator()”.
- Set the values of class members.
- Define a method “hasNext()”.
- If “current” and “current.next” are not equal to null,
- Return true.
-
-
- Iterate a “for” loop,
- If element at “b” of “buckets” is not equal to “null”,
- Return “true”.
- If element at “b” of “buckets” is not equal to “null”,
- Return “false”.
- Iterate a “for” loop,
-
- If “current” and “current.next” are not equal to null,
- Define a method “next()” with return type “E”.
- Set “previous” equal to “current”.
- Set “previousBucket” equal to “bucket”.
- If “current” and “current.next” are not equal to null,
- Increment “bucket” by 1.
- Iterate a “while” loop,
-
- Increment “bucket” by 1.
-
- If “bucket” less than size of the “bucket”.
- Set the value of “current”.
- Else,
- Throw an exception
- If “bucket” less than size of the “bucket”.
- Else,
- Set “current” equal to “current.next”.
- Return “data” of “current”.
-
- Increment “bucket” by 1.
- Define a method “remove()”.
- If “previous” and “previous.next” are not equal to null,
- Set “previous.next” equal to “current.next”.
-
-
- If “previousBucket” is less than “bucket”,
- Set “bucket” equal to “current.next”.
- Else,
- Throw an exception.
- Set “current” equal to “previous”.
- Set “bucket” equal to “previousBucket”.
- If “previousBucket” is less than “bucket”,
-
- If “previous” and “previous.next” are not equal to null,
Filename: “HashSetDemo.java”
This code snippet creates a class “HashSetDemo”. In the code,
- Import the required packages.
- Define a class “HashSetDemo”.
- Define the “main” method.
- Define a “HashSet” “names”.
- Add values to “names” using “add()”.
- Create an “Iterator” named “iter”.
- Iterate a “while” loop,
- Print values of “names”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Chapter 18 Solutions
Big Java, Binder Ready Version: Early Objects
Ch. 18.1 - Prob. 1SCCh. 18.1 - Prob. 2SCCh. 18.1 - Prob. 3SCCh. 18.1 - Prob. 4SCCh. 18.1 - Prob. 5SCCh. 18.2 - Prob. 6SCCh. 18.2 - Prob. 7SCCh. 18.2 - Prob. 8SCCh. 18.2 - Prob. 9SCCh. 18.2 - Prob. 10SC
Ch. 18.3 - Prob. 11SCCh. 18.3 - Prob. 12SCCh. 18.3 - Prob. 13SCCh. 18.3 - Prob. 14SCCh. 18.3 - Prob. 15SCCh. 18.4 - Prob. 16SCCh. 18.4 - Prob. 17SCCh. 18.4 - Prob. 18SCCh. 18.4 - Prob. 19SCCh. 18.4 - Prob. 20SCCh. 18.4 - Prob. 21SCCh. 18.5 - Prob. 22SCCh. 18.5 - Prob. 23SCCh. 18.5 - Prob. 24SCCh. 18.5 - Prob. 25SCCh. 18.5 - Prob. 26SCCh. 18.5 - Prob. 27SCCh. 18 - Prob. 1RECh. 18 - Prob. 2RECh. 18 - Prob. 3RECh. 18 - Prob. 4RECh. 18 - Prob. 5RECh. 18 - Prob. 6RECh. 18 - Prob. 7RECh. 18 - Prob. 8RECh. 18 - Prob. 9RECh. 18 - Prob. 10RECh. 18 - Prob. 11RECh. 18 - Prob. 12RECh. 18 - Prob. 13RECh. 18 - Prob. 14RECh. 18 - Prob. 1PECh. 18 - Prob. 2PECh. 18 - Prob. 3PECh. 18 - Prob. 4PECh. 18 - Prob. 5PECh. 18 - Prob. 6PECh. 18 - Prob. 7PECh. 18 - Prob. 8PECh. 18 - Prob. 9PECh. 18 - Prob. 10PECh. 18 - Prob. 11PECh. 18 - Prob. 12PECh. 18 - Prob. 13PECh. 18 - Prob. 14PECh. 18 - Prob. 15PECh. 18 - Prob. 16PECh. 18 - Prob. 17PECh. 18 - Prob. 18PECh. 18 - Prob. 19PECh. 18 - Prob. 20PECh. 18 - Prob. 21PECh. 18 - Prob. 22PECh. 18 - Prob. 1PPCh. 18 - Prob. 2PPCh. 18 - Prob. 3PPCh. 18 - Prob. 4PPCh. 18 - Prob. 5PPCh. 18 - Prob. 6PPCh. 18 - Prob. 7PPCh. 18 - Prob. 8PP
Knowledge Booster
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
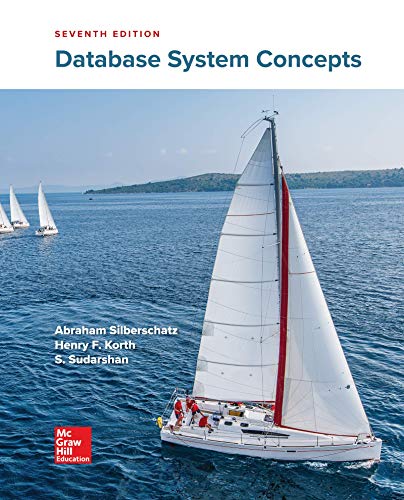
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
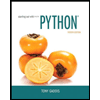
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
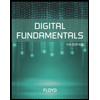
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
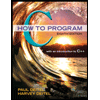
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
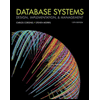
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
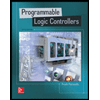
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education