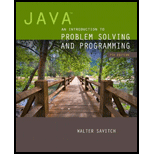
Many sports have constants embedded in their rules. For example, baseball has 9 innings, 3 outs per inning, 3 strikes in an out, and 4 balls per walk. We might encode the constants for a program involving baseball as follows:
public static final int INNINGS = 9;
public static final int OUTS_PER_INNING = 3;
public static final int SIRIKES_PER_OUT = 3;
public static final int BALLS_PBR_WALK = 4;
For each of the following popular sports, give Java named constants that could be used in a program involving that sport:
■ Basketball
■ American football
■ Soccer
■ Cricket
■ Bowling

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Computer Science: An Overview (12th Edition)
Starting Out with Python (4th Edition)
Absolute Java (6th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
- Please solve in JAVA only You are in charge of the cake for a child's birthday. You have decided the cake will have one candle for each year of their total age. They will only be able to blow out the tallest of the candles. Count how many candles are tallest. ExampleCandles = [4,4,1,3]The maximum height candles are 4 units high. There are 2 of them, so return 2. Function DescriptionComplete the function birthdayCakeCandles in the editor below. birthdayCakeCandles has the following parameter(s):• int candles[n]: the candle heights Returns• int: the number of candles that are tallest Input FormatThe first line contains a single integer n, the size of candles[].The second line contains n space-separated integers, where each integer describes the height of candles[i]. Constraints• 1 ≤ n ≤ 105• 1 ≤ candles[i] ≤ 107 Sample Input 043 2 1 3 Sample Output 02 Explanation 0Candle heights are [3,2,1,3]. The tallest candles are 3 units, and there are 2 of them. import java.io.*;import…arrow_forwardYou are working for a lumber company, and your employer would like a program that calculates the cost of lumber for an order. The company sells pine, fir, cedar, maple, and oak lumber. Lumber is priced by board feet. One board foot equals one square foot that is one inch thick. Theprice per board foot is given in the following table: Pine 0.89Fir 1.09Cedar 2.26Maple 4.50Oak 3.10 The lumber is sold in different dimensions (specified in inches of width and height, and feet of length) that need to be converted to board feet. For example, a 2 x 4 x 8 piece is 2 inches wide, 4 inches high, and 8 feet long, and is equivalent to 5.333 board feet (2 * 4 * 8 = 64, which when divided by 12 = 5.333 board feet). An entry from the user will be in the form of a letter and four integer numbers. The integers are the number of pieces, width, height, and length. The letter will be one of P, F, C, M, O (corresponding to the five kinds of wood) or T, meaning total. When the letter is T, there are no…arrow_forwardNancy wants to hang a photo frame of her graduation photo. The ladder is placed at 2 feet away from the wall. The angle that is formed by the wall and the ground is 105 degrees and the angle formed by the center mark and the ladder will be 15 degrees. In Java programming context, use the predefined method(s) in Math class, find the length of the ladder (in 2 decimal format) that Nancy needed in order to put up her graduation photo neatlyarrow_forward
- #this is a python program #topic: OOP Design a class Joker with parameterized constructor so that the following line of code prints the result shown in the output box. [You are not allowed to change the code below] #Write your class code here j1 = Joker('Heath Ledger', 'Mind Game', False) print(j1.name) print(j1.power) print(j1.is_he_psycho) print(“=====================”) j2 = Joker('Joaquin Phoenix', 'Laughing out Loud', True) print(j2.name) print(j2.power) print(j2.is_he_psycho) print(“=====================”) if j1 == j2: print('same') else: print('different') j2.name = 'Heath Ledger' if j1.name == j2.name: print('same') else: print('different') #Write your code for 2,3 here Output: Heath Ledger Mind Game False ===================== Joaquin Phoenix Laughing out Loud True ===================== different same Subtask: 1) Design the class using a parameterized constructor. 2) The first if/else block prints the output as ‘different’, but why?…arrow_forwardUse java Language Write the function parrotTrouble.** We have a loud talking parrot. The "hour" parameter* is the current hour time in the range 0..23. We are* in trouble if the parrot is talking and the hour* is before 7 or after 20. Return true if we are* in trouble.** Some Examples:* parrotTrouble(true, 6) returns true* parrotTrouble(true, 7) returns false* parrotTrouble(false, 6) returns false** @param isTalking true if the parrot is talking.* @param hour the time of day from 0..23.* @return true if you're in trouble with your neighbors.arrow_forwardThe Body Mass Index – BMI is a parameter used to measure the health status of an individual. Various BMI values depicts how healthy or otherwise a person is. Mathematically BMI = weight over height times height Write a java program using either JOptionPane or Scanner to collect the following values of an individual: a) i. Name ii. Weight iii. Height b) Compute the BMI of that individual c) Make the following decisions if: i. BMI < 18.5 is under weight. The Body Mass Index – BMI is a parameter used to measure the health status of an individual. Various BMI values depicts how healthy or otherwise a person is. Mathematically BMI = Write a java program using either JOptionPane or Scanner to collect the following values of an individual: a) i. Name ii. Weight iii. Height b) Compute the BMI of that individual The Body Mass Index – BMI is a parameter used to measure the health status of an individual. Various BMI values depicts how healthy or otherwise a person is. Mathematically BMI =…arrow_forward
- A company wants a program that will calculate the weekly paycheck for an employee based on how many hours they worked. For this company, an employee earns $20 an hour for the first 40 hours that they work. The employee earns overtime, $30 an hour, for each hour they work above 40 hours. Example: If an employee works 60 hours in a week, they would earn $20/hr for the first 40 hours. Then they would earn $30/hr for the 20 hours they worked overtime. Therefore, they earned: ($20/hr * 40hrs) + ($30/hr * 20 hrs) = $800 + $600 = $1400 total.arrow_forwardThe Body Mass Index – BMI is a parameter used to measure the health status of an individual. Various BMI values depict how healthy or otherwise a person is. Mathematically BMI = weight ÷hight×hight Write a java program using either JOptionPane or Scanner to collect the following values of an individual: i. Name ii. Weight iii. Height Major Topic Getting user inputs B.) Compute the BMI of that individual Make the following decisions if: i. BMI < 18.5 is under weight ii. BMI >=18.5 but =25 but = 30 but = 40 Morbid Obesity d)Display the results of the individualarrow_forwardPlease help me in fixing the errors in this java program. Then proceed in putting graphics like in the photos below Program is below // A simple program to demonstrate // Tic-Tac-Toe Game. import java.util.*; public class Main { static String[] board; static String turn; // CheckWinner method will // decide the combination // of three box given below. static String checkWinner() { for (int a = 0; a < 8; a++) { String line = null; switch (a) { case 0: line = board[0] + board[1] + board[2]; break; case 1: line = board[3] + board[4] + board[5]; break; case 2: line = board[6] + board[7] + board[8]; break; case 3: line = board[0] + board[3] + board[6]; break; case 4: line = board[1] + board[4] + board[7]; break; case 5: line = board[2] + board[5] + board[8]; break; case 6: line = board[0] + board[4] + board[8]; break; case 7: line = board[2] + board[4] + board[6]; break; } //For X winner if (line.equals("XXX")) { return "X"; } // For O winner else if (line.equals("OOO")) {…arrow_forward
- JAVACreate a class called Quadratic for representing a one-variable quadratic expression of the form: ax2 + bx + c a,b and c here are the coefficients. The class should contain the following methods: * A constructor that accepts values for a, b, and c. * public double getA() * public double getB() * public double getC() * public double evaluate (int x) * will return the value of the expression at point x * public double discriminant() - that will return (b2 - 4ac) * public boolean isImaginaryRoots() - roots are imaginary if (b2 - 4ac) < 0 * public boolean isRealRoots() - roots are real if (b2 - 4ac) >= 0 // these methods can only be invoked if the roots are not imaginary * public float firstRoot() * public float secondRoot() * public boolean isPerfectSquare(); // If the first and second roots are equal * Try to override the toString methods * Write a sample main program that will work as shown below. Example output: coefficient a: 4 coefficient b: 4 coefficient c: 1…arrow_forwardWrite a program to calculate total ordering cost. Jacob is a retailer for ice. Normally a bag of ice costs $2.5, but the price varies with the amount that he purchases. The following table shows the discount that Jacob receives. Order Volume Discount Rate >= 200 bags 25% off 120 - 199 bags 20% off 60 - 119 bags 15% off 40 -59 bags 10% off 20 – 39 bags 5.5% off 0-19 bags No discount (1) Inyourprogram,definevariableswithmeaningfulnamestoacceptuserinputonordervolume, and calculate total ordering cost based on the order volume inputted. Use if-else statements to handle the calculations for different order volumes as shown in the above table. (2) Yourprogramshouldprintoutuserordervolume,discountrate,andtotalorderingcostinconsole (similar to the following sample outputs)arrow_forwardDebug this program to create the desired result attached in the picture. public class Library { // Add the missing implementation to this class public static void main(String[] args) { // Create two libraries Library firstLibrary = new Library("10 Main St."); Library secondLibrary = new Library("228 Liberty St."); // Add four books to the first library firstLibrary.addBook(new Book("A Tale of Two Cities ")); firstLibrary.addBook(new Book("Le Petit Prince")); firstLibrary.addBook(new Book("The DaVinci Code")); firstLibrary.addBook(new Book("The Lord of the Rings")); // Print opening hours and the addresses System.out.println("Library hours:"); printOpeningHours(); System.out.println(); System.out.println("Library addresses:"); firstLibrary.printAddress(); secondLibrary.printAddress(); System.out.println(); // Try to borrow The Lords of the Rings from both libraries System.out.println("Borrowing The Lord of the Rings:"); firstLibrary.borrowBook("The Lord of the Rings");…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
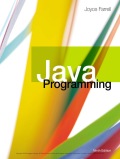
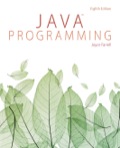