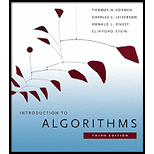
(a)
To show that the insertion sort can sort the
(a)

Explanation of Solution
The procedure of insertion sort in non-increasing order is as below:
INSERTION-SORT(A)
For
while
The j loop is running from 2 to the length of the array and then value in the index of array is stored in the key variable. After that the variable j is decreased by 1 and stored in the variable i.
Now, while loop is used in which two conditions are given: first condition is variable i is greater than 0 and the value of the array at index i must be less than key value.
The value at index i is swapped at the index
Analysis:
The worst case running time of the insertion sort to sort a list of length n is
sub lists of length k , the time taken by insertion sort is calculated as follows:
Hence, the worst case running time of insertion sort to sort the
(b)
To show that the worst case running time to merge the sub lists is
(b)

Explanation of Solution
There are
For this, take two sublists and merge them simultaneously. The time taken by this process is
Hence, the worst case running time to merge the sub listsis
(c)
To find the largest value of k as a function of n so that the modified
(c)

Explanation of Solution
Merge sort is a sorting algorithm that uses divide and conquer method. In merge sort, divide the input sequence in two halves and then sort them, Finally, merge the sorted halves.
The modified algorithm has same running time as standard merge sort if
Hence, the running time of the modified algorithm has same as standard merge sort if
(d)
To choose the value of k in practice.
(d)

Explanation of Solution
Merge sort is a sorting algorithm that uses the divide and conquer method. In merge sort, divide the input sequence in two halves and then sort them. Finally, merge the sorted halves.
For considering the value of k, choose the largest length of sub list as kso that insertion sort becomes faster than merge sort.
Want to see more full solutions like this?
Chapter 2 Solutions
Introduction to Algorithms
- Consider a hybrid sorting algorithm that combines Mergesort with Insertion Sort.It uses Mergesort until the number of elements in the input becomes smaller than or equal to 8, after which it switches to Insertion Sort. What is the number of key comparisons performed by this hybrid sorting algorithm in the best case when running on an input array of size n? Briefly justify your answer. You could assume n = 2k, k is more than 3arrow_forwardModify the FindBestPlan(S) function to create a function FindBestPlan(S, O),where O is a desired sort order for S, and which considers interesting sortorders. A null order indicates that the order is not relevant. Hints: An algorithmA may give the desired order O; if not a sort operation may need to be added to get the desired order. If A is a merge-join, FindBestPlan must be invoked on the two inputs with the desired orders for the inputs.arrow_forwardsolution should have O(l1.length + l2.length) time complexity, since this is what you will be asked to accomplish in an interview. Given two singly linked lists sorted in non-decreasing order, your task is to merge them. In other words, return a singly linked list, also sorted in non-decreasing order, that contains the elements from both original lists.arrow_forward
- Professor JAson uses the following algorithm for merging k sorted lists, each having n/k elements. He takes the first list and merges it with the second list using a linear-time algorithm for merging two sorted lists, such as the merging algorithm used in mergesort. Then, he merges the resulting list of 2n/k elements with the third list, merges the list of 3n/k elements that results with the fourth list, and so forth, until he ends up with a single sorted list of all n elements. Analyze the worst-case running time of the professor’s algorithm in terms of n and k.arrow_forwardIn this problem, consider a non-standard sorting algorithm called the Slow Sort. Given anarray A[1 : n] of n integers, the algorithm is as follows: Slow-Sort(A[1 : n]):1. If n < 100, run merge sort (or selection sort or insertion sort) on A.2. Otherwise, run Slow-Sort(A[1 : n −1]), Slow-Sort(A[2 : n]), and Slow-Sort(A[1 : n −1]) again. Question: Prove the correctness of Slow-Sort.arrow_forward1. (a)The objective is to shuffle a given array of n different elements. You would like to compare various shuffling algorithms and select the best of them. What does it mean: “the best shuffling algorithm”? What is a requirement for the perfect shuffling algorithm? (b) Is the Fisher-Yates Shuffle the perfect shuffling algorithm? Present a formal mathematical proof that this algorithm meets (or does not meet) the requirement given in (a). (c) Evaluate empirically performance of the Fisher-Yates Shuffle for selected numerical values of n. Propose your evaluation criteria and explain your evaluation methodology. Report your observations and conclusions.arrow_forward
- Explain the time and space complexity analysis on merge sort! Quick Sort has the worst complexity of O(n2). Please EXPLAIN what kind of sequence that can trigger the worst-case complexity, and how can we improve the implementation, so that on average-case the quick sort will perform in O(n lg n)arrow_forwardWhat is the time complexity of deleting an element from a sorted array? Does it have better time complexity than deleting a node from an unsorted array? Why? What is the time complexity of append operation of an unsorted array of size n? why? What is the time complexity (in big-O notation) of binary search in a sorted array containing m (m >0) numbers? Why is the insertion not supported for unsorted array? What are the fundamental operations of an unsorted array?arrow_forwardFor this given code, please help me create a recursive case for transpose of matrix using the cache oblivious algorithm. for ( size_t i=0; i<n; i += block ) { for ( size_t j=0; j<n; j++ ) { for ( size_t b = 0; b < block && i + b < n; ++b){ b[ j*n + i ] = a[ i*n + j ]; } } else { recursMatTranspose ( n, n, a, 0, 0, b, 0, 0 ); //recursive case using cache-oblivious algo } }arrow_forward
- Consider the problem of sorting elements in an array of n numbers. Design two algorithms to implement the quicksort as follows: Recursive algorithm, and Non-recursive algorithm Analyze the efficiency of your algorithms as follows: For the recursive algorithm, figure out the recurrence relation and use the Master Theorem to find out the time efficiency in Big-O notation. For the non-recursive algorithm, use counting method to count the number of comparisons in Big-O notation.arrow_forwardIn this problem, consider a non-standard sorting algorithm called the Slow Sort. Given anarray A[1 : n] of n integers, the algorithm is as follows: Slow-Sort(A[1 : n]):1. If n < 100, run merge sort (or selection sort or insertion sort) on A.2. Otherwise, run Slow-Sort(A[1 : n −1]), Slow-Sort(A[2 : n]), and Slow-Sort(A[1 : n −1]) again. Question: Write a recurrence for Slow-Sort and use the recursion tree method to solve this recurrenceand find the tightest asymptotic upper bound on the runtime of Slow-Sort.arrow_forwardConsider the following array of numbers A = {15, 4, 21, 12, 10, 0, 21, 12, 3, 14}. Show the result of the various passes needed to sort A : Using Insertion sort Using Bubble sort Using Selection sort Using RadixSort (r = 5) Use a binary tree to show the different recursive calls resulting from using MergeSort to sort Aarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
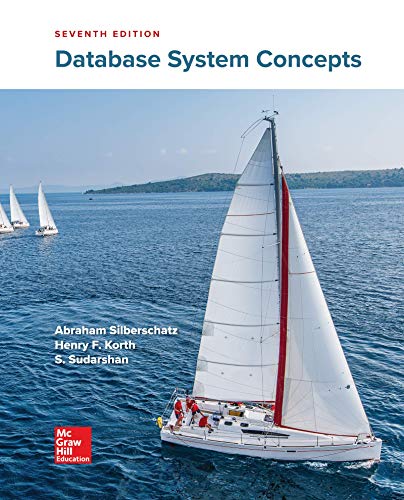
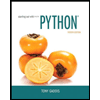
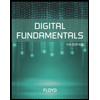
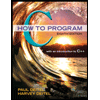
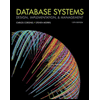
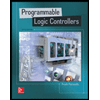