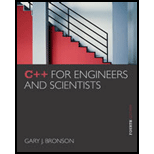
(General math) a. Design, write, compile, and run a C++
b. Manually check the values computed by your program. After verifying that your program is working correctly, modify it to determine the area of a two-dimensional triangle with a base of 3.5 in and a height of 1.45 in.
(a)

Program plan:
In the coding window, write the necessary code:
- To Declarethree float variable base=1, height=1.5 and area.
- To calculate the area of triangle.
- To display the calculated area of triangleto user.
Program description:
The main purpose of the program is to calculate the area of triangle with the given height and radius.In the main method,calculating the area of triangle with the given base and height and finally, displaying the output of area of triangle to user.
Explanation of Solution
Given information:
Program:
Program code to calculate the area of triangle:
//importing essential header files #include<iostream> //using namespace standards usingnamespace std; //main method int main() { //declaring variables float base=1; float height=1.5; float area; //calculating area area=height*base/2; //displaying area to user cout<<"area of triangle with base "<<base<<" inch and height "<<height <<" inch is "<<area<<"sq. inch"; return0; }
Explanation:
In the main method, three float variable base=1, height=1.5 and area are declared. After that the area of triangle is calculated by using the below given formula:
And the finally, calculated results are displayed to user.
Output:
Output of the above given program code:
(b)

To verify the results produced by above program and then use the above program to calculate area of two-dimensional triangle whose base in 3.5in and height 1.5 in.
Explanation of Solution
Given information:
Explanation:
Firstly, verifying the results calculate by the above program.
Putting the given values in the above formula,
Thus, the calculated results are correct.
Now, modifying the program code to calculate the area of triangle with the given height =1.45 inch and base =3.5 inch.
Program code:
//importing essential header files #include<iostream> //using namespace standards usingnamespace std; //main method int main() { //declaring variables float base=3.5; float height=1.45; float area; //calculating area area=height*base/2; //displaying area to user cout<<"area of triangle with base "<<base<<" inch and height "<<height <<" inch is "<<area<<"sq. inch"; return0; }
Explanation:
In this program, in the main method, three float variable base=3.5, height=1.45 and area are declared. After that the area of triangle is calculated by using the below given formula:
And the finally, calculated results are displayed to user.
Output:
Want to see more full solutions like this?
Chapter 2 Solutions
C++ for Engineers and Scientists
- (General math) The volume of oil stored in an underground 200-foot deep cylindrical tank is determined by measuring the distance from the top of the tank to the surface of the oil. Knowing this distance and the radius of the tank, the volume of oil in the tank can be determined by using this formula: volume=radius2(200distance) Using this information, write, compile, and run a C++ program that accepts the radius and distance measurements, calculates the volume of oil in the tank, and displays the two input values and the calculated volume. Verify the results of your program by doing a hand calculation using the following test data: radius=10feetanddistance=12feet.arrow_forward(Physics) a. Design, write, compile, and run a C++ program to calculate the elapsed time it takes to make a 183.67-mile trip. This is the formula for computing elapsed time: elapsedtime=totaldistance/averagespeed The average speed during the trip is 58 mph. b. Manually check the values computed by your program. After verifying that your program is working correctly, modify it to determine the elapsed time it takes to make a 372-mile trip at an average speed of 67 mph.arrow_forward(Physics) a. The weight of an object on Earth is a measurement of the downward force onth e object caused by Earth’s gravity. The formula for this force is determined by using Newton’s Second Law: F=MAeFistheobjectsweight.Mistheobjectsmass.AeistheaccelerationcausedbyEarthsgravity( 32.2ft/se c 2 =9.82m/ s 2 ). Given this information, design, write, compile, and run a C++ program to calculate the weight in lbf of a person having a mass of 4 lbm. Verify the result produced by your program with a hand calculation. b. After verifying that your program is working correctly, use it to determine the weight, on Earth, of a person having a mass of 3.2 lbm.arrow_forward
- (General math) a. Design, write, compile, and run a C++ program to calculate the volume of a sphere with a radius, r, of 2 in. The volume is given by this formula: Volume=4r33 b. Manually check the values computed by your program. After verifying that your program is working correctly, modify it to determine the volume of a cube with a radius of 1.67 in.arrow_forward(Practice) Determine the values of the following integer expressions: a.3+46f.202/( 6+3)b.34/6+6g.( 202)/6+3c.23/128/4h.( 202)/( 6+3)d.10( 1+73)i.5020e.202/6+3j.( 10+3)4arrow_forward(Practice) State whether the following variable names are valid. If they are invalid, state the reason. prod_a c1234 abcd _c3 12345 newamp watts $total new$al a1b2c3d4 9ab6 sum.of average volts1 finvoltarrow_forward
- (General math) a. Write a C++ program to calculate and display the value of the slope of the line connecting two points with the coordinates (3,7) and (8,12). Use the fact that the slope between two points with the coordinates (x1,y1)and(x2,y2)is(y2y1)/(x2x1). b. How do you know the result your program produced is correct? c. After verifying the output your program produces, modify it to determine the slope of the line connecting the points (2,10) and (12,6). d. What do you think will happen if you use the points (2,3) and (2,4), which results in a division by zero? How do you think this situation can be handled? e. If your program doesn’t already do so, change its output to this: The value of the slope is xxx.xx The xxx.xx denotes placing the calculated value in a field wide enough for three places to the left of the decimal point and two places to the right of it.arrow_forward(General math) If a 20-foot ladder is placed on the side of a building at an 85-degree angle, as shown in Figure 3.11, the height at which the ladder touches the building can be calculated as height=20sin85. Calculate this height by hand, and then write, compile, and run a C++ program that determines and displays the value of the height. After verifying that your program works correctly, use it to determine the height of a 25-foot ladder placed at an angle of 85 degrees.arrow_forward(Practice) Run Program 7.10 to determine the average and standard deviation of the following list of 15 grades: 68, 72, 78, 69, 85, 98, 95, 75, 77, 82, 84, 91, 89, 65, and 74.arrow_forward
- (Electrical eng.) a. Write, compile, and run a C++ program that calculates and displays the value of the current flowing through an RC circuit (see Figure 3.19). The circuit consists of a battery connected in a series to a switch, a resistor, and a capacitor. When the switch is closed, the current, i, flowing through the circuit is given by this formula: i=(EIR)et/RC Eisthevoltageofthebatteryinvolts.Risthevalueoftheresistorinohms.Cisthevalueofthecapacitorinfarads.tisthetimeinsecondsaftertheswitchisclosed.eisEulersnumber,whichis2.71828( roundedtofivedecimalplaces). Using this formula, write, compile, and run a C++ program to determine the voltage across the capacitor shown in Figure 3.19 when t is 0.31 seconds. (Note: The value of RC is referred to as the system’s time constant.) The program should prompt the user to enter appropriate values and use input statements to accept the data. In constructing the prompts, use statements such as “Enter the voltage of the battery.” Verify your program’s operation by calculating by hand the current for the following test data: Testdataset1:Voltage=20volts,R=10ohms,RC=0.044,t=0.023secondsTestdataset2:Voltage=35volts,R=10ohms,RC=0.16,t=0.067seconds b. Check the value computed by your program by hand. After verifying that your program is working correctly, use it to complete the following chart:arrow_forward(Automotive) a. An automobile engine’s performance can be determined by monitoring its rotations per minute (rpm). Determine the conversion factors that can be used to convert rpm to frequency in hertz (Hz), given that 1rotation=1cycle,1minute=60seconds,and1Hz=1cycle/sec. b. Using the conversion factors you determined in Exercise 7a, convert 2000 rpm into hertz.arrow_forward(Electrical eng.) The amplification of electronic circuits is measured in units of decibels, which is calculated as the following: 10LOG(Po/Pi) Po is the power of the output signal, and Pi is the power of the input signal. Using this formula, write, compile, and run a C++ program to calculate and display the decibel amplification, in which the output power is 50 times the input power. Verify your program’s result by doing a hand calculation. After verifying that your program is working correctly, use it to determine the amplification of a circuit, where output power is 4.639 watts and input power is 1 watt.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
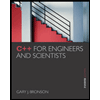