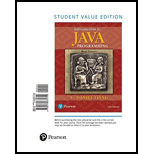
SetOperation.java
Program Plan:
- Include the class name named “SetOperation”.
- Import java array class from util package.
- Import java linkedhash set class from util package.
- Import the set interface.
- Define class.
- Declare the main()method.
- Create linked list Hash set set1 and assign name of person as values.
- Create another linked list Hash set set2 and assign name of person as values.
- Display the union of the two sets set1 and set2 using addAll() function and print the result.
- Display the difference of the two sets by calling the removeAll function and print the result.
- Display the intersection of the two sets and print the result.
- Close the main method.
- Close the class “SetOperation”.

The below java code to create two sets and assign person’s name to them and then perform set union, difference and intersection of the two sets.
Explanation of Solution
Program:
//import java array class from util package
import java.util.Arrays;
//import java linkedlist hash set class from util package
import java.util.LinkedHashSet;
//import the set interface
import java.util.Set;
//class Definition
public class SetOperation {
// main method
public static void main(String[] args) {
/* create linked list hash set Set1 and assign name of person as values */
Set<String> set1 = new LinkedHashSet<>(Arrays.asList("George", "Jim",
"John", "Blake", "Kevin", "Michael"));
/*Create another linked list hash set Set2 and assign name of person as values */
Set<String> set2 = new LinkedHashSet<>(Arrays.asList("George", "Katie","Kevin", "Michelle", "Ryan"));
/* Display the union of the two sets set1 and set2 using addAll function */
Set<String> union = new LinkedHashSet<>(set1);
union.addAll(set2);
// print the union results
System.out.println("Union of the two sets: " + union);
/* Display the difference of the two sets by calling the removeAll function
*/
Set<String> difference = new LinkedHashSet<>(set1);
difference.removeAll(set2);
// print the difference of two sets
System.out.println("Difference of the two sets: " + difference);
// Display the intersection of the two sets
Set<String> intersection = new LinkedHashSet<>();
/*
Check for the elements that are present in set 2 and also present in
* set 1
*/
for (String e : set2) {
if (set1.contains(e))
intersection.add(e);
}
// print the intersection of two sets
System.out.println("Intersection of the two sets: " + intersection);
}
}
Union of the two sets: [George, Jim, John, Blake, Kevin, Michael, Katie, Michelle, Ryan]
Difference of the two sets: [Jim, John, Blake, Michael]
Intersection of the two sets: [George, Kevin]
Want to see more full solutions like this?
Chapter 21 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
- Hashing is a technique that is used to uniquely identify a specific object from a group of similar objects. Can you use one or two real-world example(s) to explain what a "hash" really is? How can a programmer implement it? Pls, give a thorough ans.arrow_forwardDevelop a procedure that will allow you to remove from a linked list all of the nodes that share a key?arrow_forwardPROBLEM 4-Hashing and Collision. Complete the table below. Follow-up Question: From the table above, how many synonyms are there?arrow_forward
- We use LinkedLists to store all of our hash map data due to the sloppy manner in which the map was originally constructed. This substantially reduces the efficacy of a hash map.arrow_forwardPlease draw the hashtablearrow_forwardFind any two practical applications of hashing, investigate them exhaustively, and then describe them in detail. a. Do you also include references from your own research?arrow_forward
- Java - A good guideline for hash tables is that the size should be based on a product of two primes. True or False?arrow_forwardWrite a code in python, You will implement Hashtable using two techniques: Separate Chaining and Linear Probing. Implement Hashtable using Separate Chaining Implement hashtable using Linear Probing Test your both Hashtable classes with instances of Student class; you can have three (3) data members and appropriate functions, including hash() function. Test your both Hashtable classes with instances of Employee class; you can have three (3) data members and appropriate functions, including hash() function.arrow_forwardL is a list of data elements that is linear. Use the list as follows: I a linear open addressed hash table with the appropriate hash function; and (ii) a prioritised list.Use a) hashing and b) search to find a list of keys on the representations I and ii). Binary search is defined as (b). Compare how well the two methods performed over the list L.arrow_forward
- PYTHON Why am I getting an error and it doesn't show the right output? Problem 1# Implement a hashtable using an array. Your implementation should include public methods for insertion, deletion, and# search, as well as helper methods for resizing. The hash table is resized when the loadfactor becomes greater than 0.6# during insertion of a new item. You will be using linear probing technique for collision resolution. Assume the key to# be an integer and use the hash function h(k) = k mod m where m is the size of the hashtable. class HashTableProb: def __init__(self, size=10): # Initialize the hashtable with the given size and an empty array to hold the key-value pairs. self.__size = size # size of the hashtable self.__hashtable = [None for _ in range(size)] self.__itemcount = 0 # Keeps track of the number of items in the current hashtable def __contains__(self, key): return self.__searchkey(key) def __next_prime(self, x): def…arrow_forwardThere seems to be no distinction between Array lists and Hash tables. .arrow_forwardthis is the MD5 hashing for js, how do i do something similar for C++? <!------ MD5 Hashing Function ----->function hashCode(str){ md5(str);var hash = md5.create();hash.update(str);hash.hex();var hashed = hash.array();var ka ='';for(i=0;i<hashed.length;i++){ka+=String.fromCharCode(hashed[i]);}return ka;}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
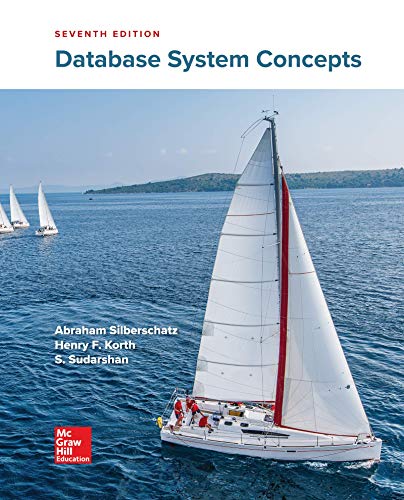
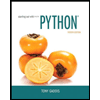
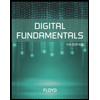
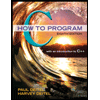
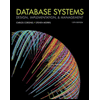
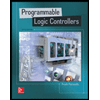