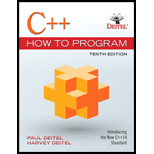
Concept explainers
22.41E a) Program Description:
The program declares an array of characters and integer to store the alphabets and corresponding counts initialized to 0;
It takes multi line inputs from the user and stores those into a
22.41E a) Program
/* * 22_41a_charactercount.cpp string handling and total alphabet counts table */ #include<string> #include<iostream> #include<cstring> #include<vector> usingnamespace std; intmain() { char alphaBets[] = { 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T','U', 'V', 'W', 'X', 'Y', 'Z','a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z'}; int counts[]={ 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,0}; vector<string> input; string str, strInput; //take input from user cout<<"Please enter any text. Enter ZZZ to finish typing:\n\n"; getline(cin, str); while ( str!= "ZZZ") { input.push_back(str); getline(cin, str); } //process the input string for (constauto& em: input){ strInput = em; for(int i=0; i < strInput.length();i++){ char test = strInput.at(i); for(int j=0;j <sizeof(alphaBets); j++){ if( test == alphaBets[j]){ counts[j]+=1; } } } } cout<<"The Alphabets counts in the supplied text are as below :\n\n\n"; for(int n=0;n <sizeof(alphaBets); n++){ cout<<"Count of "<<alphaBets[n]<<" = "<<counts[n]<<endl; } return 0; }//end main
22.41E a) Sample Output
Sample Output 22.41 a)
Please enter any text. Enter ZZZ to finish typing:
This is another test, of a justification program
for 65 chars long, with so many inputs and ! and commas,
Therefore, I have to admit this is just
a very basic program which does nothing but keeps testing
the input provided for length and applies justification logic
to the right & left parts of 65th position.
There can be many possible improvements
to this very very basic program. Thanks !
ZZZ
The Alphabets counts in the supplied text are as below :
Count of A = 0
Count of B = 0
Count of C = 0
Count of D = 0
Count of E = 0
Count of F = 0
Count of G = 0
Count of H = 0
Count of I = 1
Count of J = 0
Count of K = 0
Count of L = 0
Count of M = 0
Count of N = 0
Count of O = 0
Count of P = 0
Count of Q = 0
Count of R = 0
Count of S = 0
Count of T = 3
Count of U = 0
Count of V = 0
Count of W = 0
Count of X = 0
Count of Y = 0
Count of Z = 0
Count of a = 23
Count of b = 5
Count of c = 9
Count of d = 7
Count of e = 26
Count of f = 8
Count of g = 9
Count of h = 18
Count of i = 28
Count of j = 3
Count of k = 2
Count of l = 6
Count of m = 10
Count of n = 19
Count of o = 25
Count of p = 13
Count of q = 0
Count of r = 22
Count of s = 25
Count of t = 31
Count of u = 7
Count of v = 7
Count of w = 2
Count of x = 0
Count of y = 6
Count of z = 0
22.41E b) Program Description
The program stores lines of text input into a vector<string> and then iterates over each element, tokenizing strings into words using strtok and keeps a count of the words in an array which is finally printed out. The max word length assumed is 45 as that's the longest word in English dictionary (pneumonoultramicroscopicsilicovolcanoconiosis ).
22.41E b) Program
/* * 22_41b_wordslengthcpp string handling and total words & their length counts table */ #include<string> #include<iostream> #include<cstring> #include<vector> usingnamespace std; intmain() { //Assume the maximum word length = 45 ( as per modern dictionary //keep counts lengthwise , index 0 for length one char int counts[45]={0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}; vectorinput; string str, strInput; //take input from user cout<<"Please enter any text. Enter ZZZ to finish typing:\n\n"; getline(cin, str); while ( str!= "ZZZ") { input.push_back(str); getline(cin, str); } constchar space[2]= " "; //process the input string for (constauto& em: input){ char * temp = newchar[em.length() + 1]; strcpy(temp, em.c_str()); char * word = strtok(temp, space); while(word != 0){ int wordLen = strlen(word); if( wordLen >0) counts[wordLen-1]+=1; word =strtok(NULL, space); } } cout<<"The Words Length and their counts in the supplied text are as below :\n\n" <<"\tWord Length\t\t\t\t"<<"Total count\n" <<"\t------------\t\t\t\t------------\n"<<endl; for(intn=0;n< 45; n++){ cout<<"\t "<<n+1<<"\t\t\t\t "<<counts[n]<<endl; } return 0; }//end main
22.41E b) Sample Output
pneumonoultramicroscopicsilicovolcanokoniosis is a disease caused by exposure to volcanic silica, also termed as silicosis.
ZZZ
The Words Length and their counts in the supplied text are as below :
Word Length Total count
------------ ------------
1 2
2 4
3 0
4 1
5 0
6 3
7 1
8 2
9 1
10 0
11 0
12 0
13 0
14 0
15 0
16 0
17 0
18 0
19 0
20 0
21 0
22 0
23 0
24 0
25 0
26 0
27 0
28 0
29 0
30 0
31 0
32 0
33 0
34 0
35 0
36 0
37 0
38 0
39 0
40 0
41 0
42 0
43 0
44 0
45 1
22.41E c) Program Description
The program will use map
Finally, using the vector, all words will be iterated and their respective counts will be fetched from the map.
Explanation:
22.41E c)Program
/* * 22_41c_wordscount.cpp string handling and unique words & their length counts table */ #include<string> #include<iostream> #include<cstring> #include<vector> #include<map> #include<algorithm>// to convert whole string to uppercase or lowercase using STL. usingnamespace std; intmain() { map
22.41E c) Sample Output
Please enter any text. Enter ZZZ to finish typing:
To be or not To be is an old proverb yet interests many in the modern world
To understand this better a course in modern literature has to be pursued and that too in a deep sense
ZZZ
The unique Words and their counts in the supplied text are as below :
Word Total count -------------------- ------------ to 4 be 3 or 1 not 1 is 1 an 1 old 1 proverb 1 yet 1 interests 1 many 1 in 3 the 1 modern 2 world 1 understand 1 this 1 better 1 a 2 course 1 litratire 1 has 1 pursued 1 and 1 that 1 too 1 deep 1 sense 1

Want to see the full answer?
Check out a sample textbook solution
Chapter 22 Solutions
C++ How To Program Plus Mylab Programming With Pearson Etext -- Access Card Package (10th Edition)
- 13.16.1: Overload salutation printing. Complete the second PrintSalutation function to print the following given personName "Holly" and customSalutation "Welcome":Welcome, Holly End with a newline. #include <iostream>#include <string>using namespace std; void PrintSalutation(string personName) { cout << "Hello, " << personName << endl;} // Define void PrintSalutation(string personName, string customSalutation)... /* Your solution goes here */ int main() { PrintSalutation("Holly", "Welcome"); PrintSalutation("Sanjiv"); return 0;}arrow_forwardPython 3.7.4 Given that add, a function that expects two int parameters and returns their sum, and given that two variables, euro_sales and asia_sales, have already been defined: Write a statement that calls add to compute the sum of euro_sales and asia_sales and that associates this value with a variable named eurasia_sales.arrow_forward10.25 LAB: Quadratic formula Implement the quadratic_formula() function. The function takes 3 arguments, a, b, and c, and computes the two results of the quadratic formula: x1=−b+b2−4ac2a x2=−b−b2−4ac2a The quadratic_formula() function returns the tuple (x1, x2). Ex: When a = 1, b = -5, and c = 6, quadratic_formula() returns (3, 2). Code provided in main.py reads a single input line containing values for a, b, and c, separated by spaces. Each input is converted to a float and passed to the quadratic_formula() function. Ex: If the input is: 2 -3 -77 the output is: Solutions to 2x^2 + -3x + -77 = 0 x1 = 7 x2 = -5.50arrow_forward
- 1.19 Give two examples of semantic errors in a program, using the language of your choice.arrow_forward7. using c++, If c is a character (e.g. char c = ‘a’), then (int) c is a number between 0 and 255 that represents its ascii value of that character (the ascii value of ‘a’ is 97. Appendix B contains the ascii character set; for example, (int) ‘a’ == 97. Write a function that accepts two characters, c1, and c2, determines whether (int) c1 is greater than (int) c2, and then returns the sum of the ascii values of all the characters between c1 and c2.arrow_forward5.28 LAB: Convert to binary - functions Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x / 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110 Your program must define and call the following two functions. The IntegerToReverseBinary() function should return a string of 1's and 0's representing the integer in binary (in reverse). The ReverseString() function should return a string representing the input string in reverse. string IntegerToReverseBinary(int integerValue)string ReverseString(string userString)arrow_forward
- 6.8 In Python, An acronym is a word formed from the initial letters of words in a set phrase. Write a program whose input is a phrase and whose output is an acronym of the input. If a word begins with a lower case letter, don't include that letter in the acronym. Assume there will be at least one upper case letter in the input. Ex: If the input is: Institute of Electrical and Electronics Engineers the output is: IEEEarrow_forwardC++ 10.19 Given a line of text as input, output the number of characters excluding spaces, periods, exclamation points, or commas. Ex: If the input is: Listen, Mr. Jones, calm down. the output is: 21 Note: Account for all characters that aren't spaces, periods, exclamation points, or commas (Ex: "r", "2", "?"). code provided: #include <iostream>#include <string>using namespace std; int main() { string userText; // Add more variables as needed getline(cin, userText); // Gets entire line, including spaces. /* Type your code here. */ return 0;}arrow_forward7.24 LAB: Convert to binary-functions Write a program that takes in a positive integer as input, and outputs a string of 1's and O's representing the integer in binary. For an integer x, the algorithm is As long as x is greater than 0 Output x 2 (remainder is either 0 or 1) x = x // 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string Ex: If the input is the output is: 110 The program must define and call the following two functions. Define a function named int to reverse_binary() that takes an integer as a parameter and returns a string of 1's and O's representing the integer in binary (in reverse). Define a function named string reverse() that takes an input string as a parameter and returns a string representing the input string in reverse. def int_to_reverse_binary(integer_value) def string reverse(input_string) LAB ACTIVITY 7.24.1: LAB. Convert to binary-functions 0/10 1 # Define your functions here. 2…arrow_forward
- 5.17 LAB: Output words in a sentence that are at least 4 characters long The starter code reads in user input (assumed to be one or more sentences), and removes the punctuation. Iterate through the list of words and output the words that are more than 3 characters long. Ex: If the input is: Hello, how are you today? the output is: Hello today #provided code import stringuserInput = input() # this creates a mapping which maps each punctuation character to a null string (None)mapping = str.maketrans('', '', string.punctuation) # this removes puncutation from the stringuserInput = userInput.translate(mapping) print('User input without punctuation:', userInput) # split the string into wordswords = userInput.split()print('User input contains the following words: ')print(words)arrow_forward(7) Implement the ShortenSpace() function. ShortenSpace() has a string parameter and updates the string by replacing all sequences of 2 or more spaces with a single space. ShortenSpace() DOES NOT output the string. Call ShortenSpace() in the PrintMenu() function, and then output the edited string. Ex: Edited text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue!arrow_forward3.27 LAB: Login name Write a program that creates a login name for a user, given the user's first name, last name, and a four-digit integer as input. Output the login name, which is made up of the first six letters of the first name, followed by the first letter of the last name, an underscore (_), and then the last digit of the number (use the % operator). If the first name has less than six letters, then use all letters of the first name. Ex: If the input is: Michael Jordan 1991 the output is: Your login name: MichaeJ_1 Ex: If the input is: Nicole Smith 2024 the output is: Your login name: NicoleS_4arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
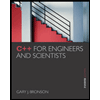