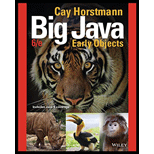
Big Java, Binder Ready Version: Early Objects
6th Edition
ISBN: 9781119056447
Author: Cay S. Horstmann
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 25, Problem 4PP
Program Plan Intro
Invoice Builder
Program plan:
Filename: “LineItem.java”
This program file is used to define a class “LineItem”. In the code,
- Define a class “LineItem”.
- Declare the class members “quantity” and “theProduct”.
- Define the constructor “LineItem()”.
- Initialize “quantity”.
- Initialize “theProduct”.
- Define a method “getTotalPrice()”,
- Return the price.
- Define a method “format()”,
- Return the value in string format
- Define a method “getQuantity()”,
- Return the value of “quantity”.
- Define a method “getProduct()”,
- Return the value of “theProduct”.
Filename: “Product.java”
This program file is used to define a class “Product”. In the code,
- Define a class “Product”.
- Declare the class members “description” and “price”.
- Define the constructor “Product()”.
- Initialize “description”.
- Initialize “price”.
- Define a method “getDescription()”,
- Return the “description”.
- Define a method “getPrice()”,
- Return the “price”.
Filename: “Address.java”
This program file is used to define a class “Address”. In the code,
- Define a class “Address”.
- Declare the class members “name”, “street”, “city”, “state” and “zip”.
- Define the constructor “Address()”.
- Initialize “name”.
- Initialize “street”.
- Initialize “city”.
- Initialize “state”.
- Initialize “zip”.
- Define a method “format()”,
- Return the value in string format
- Define a method “getName()”,
- Return the value of “name”.
- Define a method “getStreet()”,
- Return the value of “street”.
- Define a method “getCity()”,
- Return the value of “city”.
- Define a method “getState()”,
- Return the value of “state”.
- Define a method “getZip()”,
- Return the value of “zip”.
Filename: “Invoice.java”
This program file is used to define a class “Invoice”. In the code,
- Define a class “Invoice”.
- Declare the class members “billingAddress” and “items”.
- Define the constructor “Invoice()”.
- Initialize “billingAddress”.
- Initialize “items”.
- Define a method “add()”,
- Define the object of “LineItem” called “anItem”.
- Add item using “add()” method.
- Define a method “getBillingAddress()”,
- Return the value of “billingAddress”.
- Define a method “getItems()”,
- Return the value of “items”.
- Define a method “format()”,
- Declare a string variable “r” and set its value.
- Iterate a “for” loop,
- set the value of “r”.
- Set the value of “r” to a string format.
- Return the value of “r”.
- set the value of “r”.
- Define a method “getAmountDue()”,
- Declare a variable “amountDue”.
- Iterate a “for” loop,
- Set the value of “amountDue”.
- Return the value of “amountDue”.
- Set the value of “amountDue”.
Filename: “InvoiceBuilder.java”
This program file is used to define a class “InvoiceBuilder”. In the code,
- Define a class “InvoiceBuilder”.
- Declare the class members “builder” and “doc”.
- Define the constructor “InvoiceBuilder()”.
- Create an object “factory” of class “DocumentBuilderFactory”.
- Set “builder”.
- Initialize “items”.
- Define a method “build()”,
- Set “doc”.
- Create an object “root” of “Element”.
- Add “root” to “doc”.
- Return the “doc”.
- Define a method “createInvoice()”,
- Create an element “invoiceElement” for invoice.
- Create an element “addressElement”.
- Create an element “itemsElement”.
- Append “addressElement” to “invoiceElement”.
- Append “itemsElement” to “invoiceElement”.
- Define a method “createInvoice()”,
- Create an element “addressElement” for address.
- Create a text node “nameText”.
- Create an element “nameElement” for name.
- Append “nameText” to “nameElement”.
- Create a text node “streetText”.
- Create an element “streetElement” for street.
- Append “streetText” to “streetElement”.
- Create a text node “cityText”.
- Create an element “cityElement” for city.
- Append “cityText” to “cityElement”.
- Create a text node “stateText”.
- Create an element “stateElement” for state.
- Append “stateText” to “stateElement”.
- Create a text node “zipText”.
- Create an element “zipElement” for zip.
- Append “zipText” to “zipElement”.
- Append “nameElement” to “addressElement”.
- Append “streetElement” to “addressElement”.
- Append “cityElement” to “addressElement”.
- Append “stateElement” to “addressElement”.
- Append “zipElement” to “addressElement”.
- Return the value of “addressElement”.
- Define a method “createItemList()”,
- Create an element “itemsElement” for items.
- Iterate a “for” loop,
- Create an object “itemElement”.
- Append “itemElement” to “itemsElement”.
- Return the value of “itemsElement”.
- Define a method “createItem()”,
- Create an element “itemElement” for item.
- Create an element “productElement”.
- Create a text node “quantityText”.
- Create an element “quantityElement”.
- Append “quantityElement” to “quantityText”.
- Append “itemElement” to “productElement”.
- Append “itemElement” to “quantityElement”.
- Define a method “createProduct()”,
- Create a text node “descriptionText”.
- Create a text node “priceText”.
- Create an element “descriptionElement” for description.
- Create an element “priceElement” for price.
- Append “descriptionText” to “descriptionElement”.
- Append “priceText” to “priceElement”.
- Create an element “productElement” for product.
- Append “descriptionElement” to “productElement”.
- Append “priceElement” to “productElement”.
- Return “productElement”.
Filename: “InvoiceBuilderDemo.java”
This program file is used to define a class “InvoiceBuilderDemo”. In the code,
- Import the required packages.
- Define a class “InvoiceBuilderDemo”.
- Define the method “main()”.
- Define the object “samsAddress” of class “Address”.
- Define the object “samsInvoice” of class “Invoice”.
- Add the products using “add()” method.
- Define the object “builder” of class “InvoiceBuilder”.
- Define the DOM elements.
- Define a variable “out”.
- Print the variable “out”.
- Define the method “main()”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Chapter 25 Solutions
Big Java, Binder Ready Version: Early Objects
Ch. 25.1 - Prob. 1SCCh. 25.1 - Prob. 2SCCh. 25.1 - Prob. 3SCCh. 25.2 - Prob. 4SCCh. 25.2 - Prob. 5SCCh. 25.3 - Prob. 6SCCh. 25.3 - Prob. 7SCCh. 25.4 - Prob. 8SCCh. 25.4 - Prob. 9SCCh. 25.4 - Prob. 10SC
Ch. 25 - Prob. 1RECh. 25 - Prob. 2RECh. 25 - Prob. 3RECh. 25 - Prob. 4RECh. 25 - Prob. 5RECh. 25 - Prob. 6RECh. 25 - Prob. 7RECh. 25 - Prob. 8RECh. 25 - Prob. 9RECh. 25 - Prob. 10RECh. 25 - Prob. 11RECh. 25 - Prob. 12RECh. 25 - Prob. 13RECh. 25 - Prob. 14RECh. 25 - Prob. 15RECh. 25 - Prob. 16RECh. 25 - Prob. 17RECh. 25 - Prob. 18RECh. 25 - Prob. 1PECh. 25 - Prob. 2PECh. 25 - Prob. 3PECh. 25 - Prob. 4PECh. 25 - Prob. 5PECh. 25 - Prob. 6PECh. 25 - Prob. 1PPCh. 25 - Prob. 2PPCh. 25 - Prob. 3PPCh. 25 - Prob. 4PPCh. 25 - Prob. 5PPCh. 25 - Prob. 6PPCh. 25 - Prob. 7PPCh. 25 - Prob. 8PPCh. 25 - Prob. 9PPCh. 25 - Prob. 10PPCh. 25 - Prob. 11PPCh. 25 - Prob. 12PP
Knowledge Booster
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
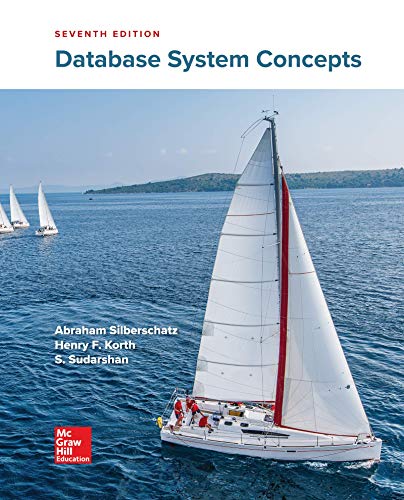
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
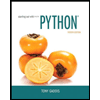
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
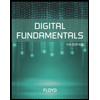
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
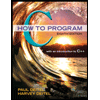
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
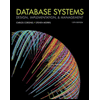
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
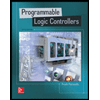
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education