Show the output of the following code:
public class Test {
public static void main(String[] args) {
WeightedGraph<Character> graph = new WeightedGraph<>();
graph.addVertex(‘U’);
graph.addVertex(‘V’);
graph.addVertex(‘X’);
int indexForU = graph.getIndex(‘U’);
int indexForV = graph.getIndex(‘V’);
int indexForX = graph.getIndex(‘X’);
System.out.println(“indexForU is ” + indexForU);
System.out.println(“indexForV is ” + indexForV);
System.out.println(“indexForX is ” + indexForV);
graph.addEdge(indexForU, indexForV, 3.5);
graph.addEdge(indexForV, indexForU, 3.5);
graph.addEdge(indexForU, indexForX, 2.1);
graph.addEdge(indexForX, indexForU, 2.1);
graph.addEdge(indexForV, indexForX, 3.1);
graph.addEdge(indexForX, indexForV, 3.1);
WeightedGraph<Character>.ShortestPathTree tree =
graph.getShortestPath(1);
graph.printWeightedEdges();
tree.printTree();
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 29 Solutions
Instructor Solutions Manual For Introduction To Java Programming And Data Structures, Comprehensive Version, 11th Edition
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (4th Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Database Concepts (7th Edition)
Differential Equations: Computing and Modeling (5th Edition), Edwards, Penney & Calvis
C++ How to Program (10th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
- In C++ class rectangleType { public: void setLengthWidth(double x, double y); //Sets the length = x; width = y; void print() const; //Output length and width double area(); //Calculate and return the area of the rectangle (length*width) double perimeter(); //Calculate and return the perimeter (length of outside boundary of the rectangle) private: double length; double width; }; Set the length of the rectangle to 24 and the width to 30.arrow_forwardIn C++ class rectangleType { public: void setLengthWidth(double x, double y); //Sets the length = x; width = y; void print() const; //Output length and width double area(); //Calculate and return the area of the rectangle (length*width) double perimeter(); //Calculate and return the perimeter (length of outside boundary of the rectangle) private: double length; double width; }; Print out the area of the rectangle.arrow_forwardIn C++ QUESTION 14 class rectangleType { public: void setLengthWidth(double x, double y); //Sets the length = x; width = y; void print() const; //Output length and width double area(); //Calculate and return the area of the rectangle (length*width) double perimeter(); //Calculate and return the perimeter (length of outside boundary of the rectangle) private: double length; double width; }; Write the function definition for the function perimeterarrow_forward
- Trace through the following program and show the output. Show your work for partial credit. public class Employee { private static int empID = 1111l; private String name , position; double salary; public Employee(String name) { empID ++; this.name 3 пате; } public Employee(Employee obj) { empID = obj.empĪD; пате %3D оbj.naте; position = obj.position; %3D public void empPosition(String empPosition) {position = empPosition;} public void empSalary(double empSalary) { salary = empSalary;} public String toString() { return name + " "+empID + " "+ position +" $"+salary; public void setName(String empName){ name = empName;} public static void main(String args[]) { Employee empOne = new Employee("James Smith"), empTwo; %3D empOne.empPosition("Senior Software Engineer"); етрOпе.етpSalary(1000); System.out.println(empOne); еmpTwo empTwo.empPosition("CEO"); System.out.println(empOne); System.out.println(empTwo); %3D етpОпе empOne ;arrow_forwardclass Solution(object): def longestCommonPrefix(self, strs): result ="" for i in strs[0]: for j in strs[1]: for k in strs[2]: if i == j and i == k: result+=i if len(result) >0: return result else: return result IndexError: list index out of range for j in strs[1]: Line 5 in longestCommonPrefix (Solution.py) ret = Solution().longestCommonPrefix(param_1) Line 31 in _driver (Solution.py) _driver() Line 41 in <module> (Solution.py) can someone explain why this is wrong?arrow_forwardclass WaterSort { Character top = null; // create constants for colors static Character red= new Character('r'); static Character blue = new Character('b'); static Character yellow= new Character('g'); // Bottles declaration public static void showAll( StackAsMyArrayList bottles[]) { for (int i = 0; i<=4; i++) { System.out.println("Bottle "+ i+ ": " + bottles[i]); } } public static void main(String args[]) { //part 1 //create the bottle StackAsMyArrayList bottleONE = new StackAsMyArrayList<Character>(); System.out.println("\nbottleONE:"+ bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform());…arrow_forward
- public static void simpleLoop(int total) { String tmp = ""; for (int x = 0; x < total; x++) { tmp + (x + ","); } } } System.out.println(tmp.length()); public static void main(String[] args) { simpleLoop (2);arrow_forwardusing System; class Program { publicstaticvoid Main(string[] args) { int number = 1,i; while (number <= 88) { Console.WriteLine(number); number = number + 2; { Random r = new Random(); int[] randNo=newint[3]; for(i=0;i<3;i++) { randNo[i]= r.Next(1,88) Console.WriteLine("Random Number between 1 and 88 is "+randNo); } } } } } how can this program be fixed to choose three random numbers in C# between 1-88 instead of just one random number?arrow_forwardFor the following four classes, choose the correct relationship between each pair. public class Room ( private String m type; private double m area; // "Bedroom", "Dining room", etc. // in square feet public Room (String type, double area) m type type; m area = area; public class Person { private String m name; private int m age; public Person (String name, int age) m name = name; m age = age; public class LivingSSpace ( private String m address; private Room[] m rooms; private Person[] m occupants; private int m countRooms; private int m countoccupants; public LivingSpace (String address, int numRooms, int numoccupants) m address = address; new int [numRooms]; = new int [numOceupants]; m rooms %3D D occupants m countRooms = m countOccupants = 0; public void addRoom (String type, double area)arrow_forward
- public class GameList {Game head;public GameList() {head = null;}public void addFront(String n, int min) {Game g = new Game(n, min);g.next = head;head = g;}} Add a tail pointerarrow_forwardCourse: Data Structure and Algorithims Language: Java Kindly make the program in 2 hours. Task is well explained. You have to make the proogram properly in Java and attach output screen with program: Restriction: Prototype cannot be change you have to make program by using given prototype. TAsk: classBSTNode { Node left; Node right; int data; publicBSTNode(int _data);// assign data to_data and assign left and right node to null } class BST { BSTNoderoot; public BST();// assign root to null void insert(int data);// this function insert the data in tree which maintain property of BST boolean Search(int key); this function search the data in bst and return true if key is found else return false public void EvenPrint(Node n)// this function only print the data which are even, make this function resursive public void OddPrint(Node n)// this function only print the data which are odd, make this function resursive public void PrimePrint(Node n)// this function only…arrow_forwardclass isinfinite_output { public static void main(String args[]) { Double d = new Double(1 / 0.); boolean x = d.isInfinite(); System.out.print(x); } } What will the given code prints on the output screen.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
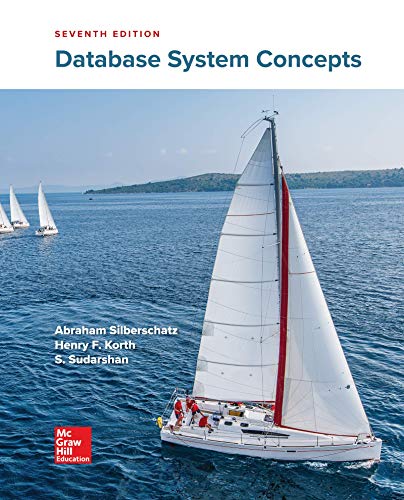
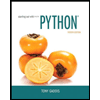
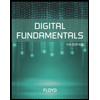
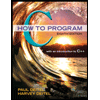
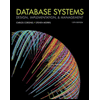
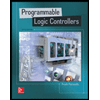