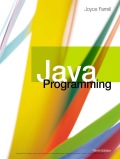
Explanation of Solution
a.
Program code:
Lease.java
//define the class Lease
public class Lease
{
//declare class members
private static final double PET_FEE = 10;
private String tenantName;
private int apartmentNumber;
private double monthlyRent;
private int leasePeriod;
//Default constructor
public Lease()
{
//initialize the class members
tenantName ="XXX";
apartmentNumber =0;
monthlyRent = 1000;
leasePeriod =12;
}
//getters and setters
//define a method getTenantName()
public String getTenantName()
{
//return the variable tenantName
return tenantName;
}
//define a method setTenantName()
public void setTenantName(String tenantName)
{
//set the value of tenantName
this.tenantName = tenantName;
}
//define a method getApartmentNumber()
public int getApartmentNumber()
{
//return the variable apartmentNumber
return apartmentNumber;
}
//define a method setApartmentNumber()
public void setApartmentNumber(int apartmentNumber)
{
//set the value of apartmentNumber
this.apartmentNumber = apartmentNumber;
}
//define a method getMonthlyRent()
public double getMonthlyRent()
{
//return the variable monthlyRent
return monthlyRent;
}
//define a method setMonthlyRent()
public void setMonthlyRent(double monthlyRent)
{
//set the value of monthlyRent
this.monthlyRent = monthlyRent;
}
//define a method getLeasePeriod()
public int getLeasePeriod()
{
//return the variable leasePeriod
return leasePeriod;
}
//define a method setLeasePeriod()
public void setLeasePeriod(int leasePeriod)
{
//set the value of leasePeriod
this.leasePeriod = leasePeriod;
}
//define a method addPetFee()
public void addPetFee()
{
//adds $10
monthlyRent+=PET_FEE;
}
//define a method explainPetPolicy()
public static void explainPetPolicy()
{
//print the statement
System.out.println("Add $10 to rent as pet fee.");
}
}
Explanation:
The above snippet of code is used create a class “Lease”. The class contain different static methods for store the details of a lease. In the code,
- Define a class “Lease”
- Declare the class members.
- Define the constructor “Lease()” method.
- Initialize the class members.
- Define the “getTenantName()” method.
- Return the value of the variable “tenantName”.
- Define the “setTenantName()” method.
- Set the value of the variable “tenantName”.
- Define the “getApartmentNumber()” method.
- Return the value of the variable “apartmentNumber”.
- Define the “setApartmentNumber()” method.
- Set the value of the variable “ApartmentNumber”.
- Define the “getMonthlyRent()” method.
- Return the value of the variable “MonthlyRent”.
- Define the “setMonthlyRent ()” method.
- Set the value of the variable “MonthlyRent”.
- Define the “getLeasePeriod()” method.
- Return the value of the variable “leasePeriod”.
- Define the “setLeasePeriod()” method.
- Set the value of the variable “leasePeriod”.
- Define the “addPetFree()” method.
- Set the value of “monthlyRent”.
- Define the “explainPetPolicy()” method.
- Print the statement.
b.
TestLease.java
//import the packages
import java.text.NumberFormat;
import java.util.Scanner;
//define a class TestLease
public class TestLease
{
//define main() method
public static void main(String[] args)
{
//declare the objects of the class Lease
Lease lease1 = new Lease();
Lease lease2 = new Lease();
Lease lease3 = new Lease();
Lease lease4 = new Lease();
//Call three times getdata()
lease1 = getData();
lease2 = getData();
lease3 = getData();
System.out.print("Display info of tenants\n\n");
//Print info
showValues(lease1);
showValues(lease2);
showValues(lease3);
showValues(lease4);
System...

Trending nowThis is a popular solution!

Chapter 3 Solutions
EBK JAVA PROGRAMMING
- Write a class, AgeMessages , which does the following:a. Ask the user to enter age using a Scannerb. If the age is less than 13, print: “too young to create a Facebook account”c. If the age is less than 16, print: “too young to get a driver's license”d. If the age is less than 18, print: “too young to get a tattoo”e. If the age is less than 21, print: “too young to drink alcohol”f. If the age is less than 35, print: “too young to run for President of the U.S.” and on nextline print: “(How sad!)”g. If the age is greater and equal to 35, print: “able to do anything” and on next line print:“(How happy!)”arrow_forwardWrite a class, AgeMessages , which does the following:a. Ask the user to enter age using a Scannerb. If the age is less than 13, print: “too young to create a Facebook account”c. If the age is less than 16, print: “too young to get a driver's license”d. If the age is less than 18, print: “too young to get a tattoo”e. If the age is less than 21, print: “too young to drink alcohol”f. If the age is less than 35, print: “too young to run for President of the U.S.” and on next line print: “(How sad!)”g. If the age is greater and equal to 35, print: “able to do anything” and on next line print: “(How happy!)”Use an if statementarrow_forwardFor this lab task, you will work with classes and objects. Create a class named text that works similar to the built-in string class. You will need to define a constructor that can be used to initialize objects of this new class with some literal text. Next, define three methods: to_upper() that will convert all characters to uppercase, reverse() that will reverse the text and length() that will return the length of the text. After you have completed this part, copy the following mainfunction to your code and check if the implementation is correct. int main() { text sample = "This is a sample text"; cout << sample.to_upper(); // This should display "THIS IS A SAMPLE TEXT" cout << endl;cout << sample.reverse(); // This should display "txet elpmas a si sihT"cout << endl; cout << sample.length(); // This should display 21 }arrow_forward
- The formula for converting a temperature from Fahrenheit to Celsius is: C = 5/9(F – 32) where F is the temperature in Fahrenheit and C is the temperature in Celsius. Write a class called TemperatureConversion that includes methods that: • Convert Celsius to Fahrenheit. The Celsius temperature is passed as a parameter and it returns the corresponding Fahrenheit temperature. • Convert Fahrenheit to Celsius. The Fahrenheit temperature is passed as a parameter and it returns the corresponding Celsius temperature. Demonstrate the methods by using loops to display the Fahrenheit temperatures 32 through 212 and their Celsius equivalents and the Celsius temperatures between 0 and 100 and their Fahrenheit equivalents. in c#arrow_forwardCreate a class named Checkup with fields that hold a patient number, two blood pressure figures (systolic and diastolic), and two cholesterol figures (LDL and HDL). Include methods to get and set each of the fields. Include a method named computeRatio() that divides LDL cholesterol by HDL cholesterol and displays the result. Include an additional method named explainRatio() that explains that HDL is known as “good cholesterol” and that a ratio of 3.5 or lower is considered optimum. Save the class as CheckupType.cpp. Create a tester program named TestCheckup whose main() method declares four Checkup objects. Call a getData() method four times. Within the method, prompt a user for values for each field for a Checkup, and return a Checkup object to the main() method where it is assigned to one of main()’s Checkup objects. Then, in main(), pass each Checkup object in turn to a showValues()method that displays the data. Blood pressure values are usually displayed with a slash between the…arrow_forwardWrite a class called Pants that contains the following information: 1. Private instance variables for the waist size of the pants (an integer between 20 and 46), and the inseam of the pants (an integer between 24 and 38). 2. A two-argument constructor to set each of the instance variables above. If the waist or inseam is out of range, throw an IllegalArgumentException stating the argument that is not correct. 3. Get and Set methods for each instance variable with the same error detection as the constructor.arrow_forward
- I need to write a jave program to keep track of a team sport. Write a class, Team.java, that has the following: * two private instance variables: a String named color and an int named score. * a default constructor with no parameters that initializes color to "Red" and score to zero. * a constructor that takes two parameters (one for each instance variable) and sets the values of the instance variables. * a public getter and setter method for each instance variable. * a toString method that returns the color and score in the following format: "Red Team with 0 points."arrow_forwardPLEASE FAST Define a class called Country. A Country has populationSize, area, capital, currency, and abbreviation (2-3 letters taken from the country name). Define at least two constructors, at least two getter methods, at least two setter methods, and Override the toString() and the equals() methods. The equals() method must return true if the two countries have the same name and same populationSize and false otherwise. The abbreviation is the first letter and the second letter of the country name and must be generated automatically (e.g. if the country name is Palestine the abbreviation is PA, if the country name is Jordan the abbreviation is JO.) Write a main method, define an array of country objects, read countries data from the user, if two countries have the same abbreviation add the last letter from the country name to the abbreviation of the country inserted last. Display countries whose area is greater than 10000 km2.arrow_forwardCreate a class named Billing that includes three overloaded computeBill()methods for a photo book store.•• When computeBill() receives a single parameter, it represents the price ofone photo book ordered. Add 8% tax, and return the total due.•• When computeBill() receives two parameters, they represent the price ofa photo book and the quantity ordered. Multiply the two values, add 8% tax,and return the total due.•• When computeBill() receives three parameters, they represent the price of aphoto book, the quantity ordered, and a coupon value. Multiply the quantityand price, reduce the result by the coupon value, and then add 8% tax andreturn the total due.Write a main() method that tests all three overloaded methods. Save theapplication as Billing.java.arrow_forward
- Write a class called Investment with fields called principal and interest. The constructor should set the values of those fields. There should be a method called value_after that returns the value of the investment after n years. The formula for this is p(1+i)ⁿ, where p is the principal, and i is the interest rate.arrow_forward1: Based on Program 13-1 in the textbook, create a class name “Circle” with the following declarations by completing missing methods. (Hint: you can use PI=3.14.) //Circle class declaration class Circle { private: double radius; public: void setRadius(double); double getRadius() const; double getArea() const; double getPerimeter() const; };arrow_forwardCreate a class Course, which has one field: String courseName Create the constructor, accessor, and mutator for the class. Then, in the main method of this class, create an instance of the class with the name "CST1201". Write an equivalent while statement to replace the following for statement for (int i=2; i<100; i=i+2) { System.out.println(i); }arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
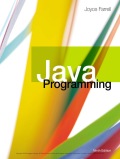
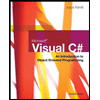