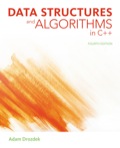
Sequential fit methods using linked list:
Program plan:
- Define a function named “printList()” that displays elements of linked list.
- Define a function named “insert()” that takes an integer value as argument and insert the value into linked list.
- Declare a function named “first_fit()” that allocates the process with memory based on first fit strategy.
- Define a function named “best_fit()” that allocates the process with memory based on best fit strategy.
- Define the function “worst_fit()” that allocates the process with memory based on worst fit strategy.
- Define the function “next_fit()” that allocates the process with memory based on next fit strategy.

/***********************************************************
* This program creates a linked list of memory nodes *
* and allocates memory to new process arrivals based on *
* first fit, next fit, best fit and worst fit strategies *
***********************************************************/
Explanation of Solution
Program:
//Include header files
#include<iostream>
using namespace std;
//Declare variables
int lcount = 0;
//Declare a node "ltemp" and initialize it to NULL
struct lnode *ltemp = NULL;
/*Define a node of the linked list with data and pointer to next node */
struct lnode
{
//Define data of node
int ldata;
//Define pointer to next node
struct lnode *lnext;
};
//Define a node "lnode" and initialize to null
struct lnode *head = NULL;
//The function "printList()" prints all elements of list
void printList()
{
//Set pointer to head of the linked list
struct lnode *ptr = head;
//Loop until the list reaches NULL
while(ptr != NULL)
{
//Display all elements of list
cout<<ptr->ldata;
cout<<"->";
//Iterate to next element of list
ptr = ptr->lnext;
}
}
/* The function "insert()" pushes the element into the linked list, it takes the element to be inserted as argument */
void insert(int ldata)
{
//Allocate memory for the node
struct lnode *link = (struct lnode*)malloc(sizeof(struct lnode));
//Insert data to the node
link->ldata = ldata;
//Set head as link's next
link->lnext = head;
//Set "link" as "head"
head = link;
}
/*The function "first_fit()" allocates the process with memory based on first fit strategy */
void first_fit()
{
//Declare the variables
int id, size;
//Get process id from user
cout<<"Enter process id" ;
//Store the process id
cin>>id;
//Get process size from user
cout<<"Enter process size";
//Store the process size
cin>>size;
//Set pointer to head of the linked list
struct lnode *ptr = head;
//Loop until the list reaches NULL
while(ptr != NULL)
{
/* If the process can be allocated, that is if size is less than or equal to memory size */
if(size <= ptr->ldata)
{
//Allocate the node and set the memory left
ptr->ldata = ptr->ldata - size;
//Traverse to next node of list
ptr = ptr->lnext;
break;
}
else
{
//Move to next node of list
ptr = ptr->lnext;
}
}
}
/*The function "best_fit()" allocates the process with memory based on first fit strategy*/
void best_fit()
{
//Declare the variables
int id, size,f=0, min =5000;
//Get process id from user
cout<<"Enter the process id" ;
//Store the process id
cin>>id;
//Get process size from user
cout<<"Enter the process size";
//Store the process size
cin>>size;
//Set pointer to head of the linked list
struct lnode *ptr = head;
//Loop until the list reaches NULL
while(ptr!=NULL)
{
//store value in node to "b"
int b = ptr->ldata;
/*Take difference of memory present and memory required */
int diff = ptr->ldata - size;
/* If difference has 0 or positive value and also less than or equal to "min" */
if(diff>=0 && diff<=min)
{
//Assign value of minimum difference
min = diff;
/* Store data of node with value of minimum difference */
f = ptr->ldata;
}
//Move to next node
ptr = ptr->lnext;
}
//Set pointer to head of the linked list
struct lnode *ptr1 = head;
//Loop until the list reaches NULL
while (ptr1!=NULL )
{
//check for the node with minimum difference value
if(ptr1->ldata == f )
{
/*Assign the memory left after allocation to node */
ptr1->ldata = min;
/* If there is no space to allocate a process */
if(ptr1->ldata==5000)
//Display error message
cout<<"Allocation not Possible"<<endl;
//Display value of node
ptr1->ldata = 0;
break;
}
//Move to next node of linked list
ptr1= ptr1->lnext;
}
}
/*The function "worst_fit()" allocates the process with memory based on worst fit strategy*/
void worst_fit()
{
//Declare the variables
int id, size;
//Get process id from user
cout<<"Enter the process id" ;
//Store the process id
cin>>id;
//Get process size from user
cout<<"Enter the process size";
//Store the process size
cin>>size;
//Set pointer to head of the linked list
struct lnode *ptr = head;
//Declare the variables
int max = 0, g=0;
//Loop until the list reaches NULL
while(ptr!=NULL)
{
//store value in node to "b"
int b = ptr->ldata;
/*Take difference of memory present and memory required */
int diff = ptr->ldata - size;
/* If difference has 0 or positive value and also less than or equal to "max" */
if(diff>=0 && diff>=max)
{
//Assign the maximum difference value
max = diff;
/* Store data of node with minimum difference value */
g = ptr->ldata;
}
//Move to next node
ptr = ptr->lnext;
}
//Set pointer to head of the linked list
struct lnode *ptr1 = head;
//Declare variables
int lcount = 0;
//Loop until the list reaches NULL
while (ptr1!=NULL)
{
//check for the node with maximum difference value
if(ptr1->ldata == g)
{
/* Assign the memory left after allocation to node */
ptr1->ldata = max;
break;
}
//Move to next node
ptr1= ptr1->lnext;
}
}
/*The function "next_fit()" allocates the process with memory based on next fit strategy*/
void next_fit(struct lnode *ltemp)
{
//Declare variables
int id, size;
//Get process id from user
cout<<"Enter the process id" ;
//Store the process id
cin>>id;
//Get process size from user
cout<<"Enter the process size";
//Store the process size
cin>>size;
//Set pointer to head of the linked list
struct lnode *ptr = ltemp;
//After first iteration of loop allocate to next node
if(lcount>0)
{
//Move to next node of linked list
ptr = ptr->lnext;
}
//Loop until the list reaches NULL
while(ptr != NULL)
{
/* If the process can be allocated, that is if size is less than or equal to memory size */
if(size <= ptr->ldata )
{
//Allocate the node and set the memory left
ptr->ldata = ptr->ldata - size;
//Move to next node
ltemp = ptr->lnext;
//Increment count to represent loop iteration
lcount++;
break;
}
//If size doesn't matches the memory present
else
{
//Move to next node
ptr = ptr->lnext;
}
}
//If the list reaches NULL
if(ptr == NULL)
{
//Set pointer to head of the linked list
struct lnode* ptr1 = head;
//Loop until the list reaches NULL
while(ptr1 != NULL)
{
/*If the process can be allocated, that is if size is less than or equal to memory size */
if(size <= ptr1->ldata )
{
/*Allocate the node and set the memory left */
ptr1->ldata = ptr1->ldata - size;
//Move to next node
ltemp = ptr1->lnext;
/*Increment count to represent loop iteration */
lcount++;
break;
}
//If size doesn't matches the memory present
else
{
//Move to next node
ptr1 = ptr1->lnext;
}
}
}
}
//Define a main function
int main()
{
//Declare variables
int ch;
int c =0;
//Create a linked list, insert the nodes with values
insert(5);
insert(10);
insert(9);
insert(20);
//Display linked list of memory spaces
cout<<"List showing memory layout is "<<endl;
//Call the function "printList()" to display list
printList();
cout<<endl;
//Loop forever until "Exit" is chosen by user
for(;;)
{
//Display the menu
cout<<"1. First Fit"<<endl;
cout<<"2. Best Fit"<<endl;
cout<<"3. Worst Fit"<<endl;
cout<<"4. Next Fit"<<endl;
cout<<"5. Exit"<<endl;
//Get user input
cout<<"Enter your choice"<<endl;
cin>>ch;
/* Define a switch statement to call appropriate function */
switch(ch)
{
/*Call the function "first_fit()" in case of first choice */
case 1:
first_fit();
//Display the list after allocation
cout<<"List after allocation is"<<endl;
/* Call the function "printList()" to display the list */
printList();
break;
/* Call the function "best_fit()" in case of second choice */
case 2:
best_fit();
//Display the list after allocation
cout<<"List after allocation is"<<endl;
/* Call the function "printList()" to display the list */
printList();
break;
/* Call the function "worst_fit()" in case of third choice */
case 3:
worst_fit();
//Display the list after allocation
cout<<"List after allocation is"<<endl;
/* Call the function "printList()" to display the list */
printList();
break;
/* Call the function "next_fit()" in case of fourth choice */
case 4:
//Set "ltemp" as head of linked list
ltemp = head;
next_fit(ltemp);
//Display the list after allocation
cout<<"List after allocation is"<<endl;
/* Call the function "printList()" to display the list */
printList();
break;
//Define case for exit
case 5:
exit(0);
//Default case in switch statement
default:
//Display error message
cout<<"wrong choice";
}
//Pause the console window
system("pause");
}
return 0;
}
List showing memory layout is
20->9->10->5->
1. First Fit
2. Best Fit
3. Worst Fit
4. Next Fit
5. Exit
Enter your choice
1
Enter process id1
Enter process size9
List after allocation is
11->9->10->5->Press any key to continue . . .
1. First Fit
2. Best Fit
3. Worst Fit
4. Next Fit
5. Exit
Enter your choice
2
Enter the process id2
Enter the process size9
List after allocation is
11->0->10->5->Press any key to continue . . .
1. First Fit
2. Best Fit
3. Worst Fit
4. Next Fit
5. Exit
Enter your choice
3
Enter the process id3
Enter the process size1
List after allocation is
10->0->10->5->Press any key to continue . . .
1. First Fit
2. Best Fit
3. Worst Fit
4. Next Fit
5. Exit
Enter your choice
4
Enter the process id5
Enter the process size3
List after allocation is
7->0->10->5->Press any key to continue . . .
1. First Fit
2. Best Fit
3. Worst Fit
4. Next Fit
5. Exit
Enter your choice
Efficiency Comparison of sequential fit Methods
First fit | Best fit | Worst fit | Next first |
Allocates at first possible space | Allocates at best memory space available | Allocates in the largest memory hole present | Allocates first at possible memory space then moves to next node |
Always starts searching from head of list | Always starts searching from head of list | Always starts searching from head of list | Starts searching from memory space allocated before |
It leads to internal fragmentation | It avoids internal fragmentation by chosing best memory space | It reduces the rate of production of small holes | It also leads to internal fragmentation |
It is the fastest | It is slowest of all as it has to consider the best hole | It is less faster than first fit as it has to allocate the largest available space | It is almost as fast as first fit algorithm as it proceeds to next block after assigning first one |
The unused memory after allocation becomes waste | It fills memory with small holes that are useless | A larger process arriving at a later time could not be processed | It reduces the seek time and starts from the previous left off allocated space |
Want to see more full solutions like this?
Chapter 3 Solutions
EBK DATA STRUCTURES AND ALGORITHMS IN C
- Implement a method to remove a node in the middle of a singly linked list (i.e., any node except the first and last node, not necessarily the exact middle), given only access to that node.EXAMPLElnput:the node c from the linked list a->b->c->d->e->fResult: nothing is returned, but the new linked list looks like a ->b->d->e->farrow_forwardImplement a nested class DoubleNode for building doubly-linked lists, whereeach node contains a reference to the item preceding it and the item following it in thelist (null if there is no such item). Then implement static methods for the followingtasks: insert at the beginning, insert at the end, remove from the beginning, removefrom the end, insert before a given node, insert after a given node, and remove a givennode.arrow_forwardImplement a method to remove a node from the centre of a singly linked list—that is, any node other than the first and end nodes—with access to only that node.EXAMPLEThe node C from the linked list a->b->c->d->e->f should be entered.Nothing is returned as a result, although the new linked list has the shape of an a->b->d->e->farrow_forward
- Apply the methods in the ListIterator interface to write a Java program in NetBeans that creates a LinkedList of four elements of type string, namely: Java, C#, PHP and Python.The program should then print out the elements initially in the original order, and then afterwards in reverse order using the ListIterator methods.arrow_forwardLooking at all four list implementations, which actions/methods tend to be less efficient in the Linked List implementation compared to the Array Implementation? Explain why for each action/method you specify.arrow_forwardThe strongest linkedlist is made up of an unknown number of nodes.Is there one in particular that stands out as being particularly lengthy?arrow_forward
- The nodes of the strongest linkedlist are undetermined.Is one especially lengthy?arrow_forward) Implement a self-organizing list by creating a singly linked list of nodes andundertaking frequent retrievals of data in the list repeatedly and at random. Showhow the list restructures itself when (i) count, (ii) move to front and (iii) transposemethods are used for the same set of frequent retrievals.arrow_forwardcan I have the solution for this question in Java coding please? Write an algorithm for the following to swap two adjacent elements by adjusting only the links (and not the data) using: Singly-linked lists. Doubly linked lists.arrow_forward
- Measure the performance of addFirst, remove(Object), and removeLast for each of the three implementations (you may include Vectors, if you wish). Which implementations perform best for small lists? Which implementations perform best for large lists?arrow_forwardImplement program to adds methods to search a linked list for a data item with aspecified key value, and to remove an item with a specified key value. These, along with insertion at the start of the list, are the same operations carried out by the LinkList Workshop applet. Write The complete linkList2.cpp program.arrow_forwardApply the methods in the ListIterator interface to write a Java program in NetBeans that creates a LinkedList of four elements of type string, namely: Java, C#, PHP and Python.The program should then print out the elements initially in the original order, and then afterwards in reverse order using the ListIterator methods. In this case, the following(attached) is the expected output:arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
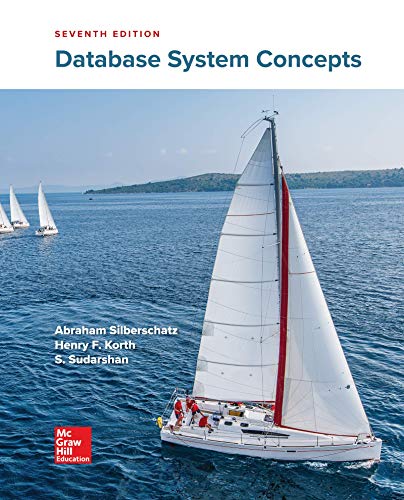
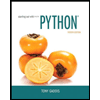
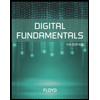
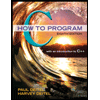
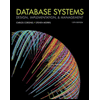
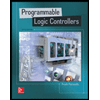