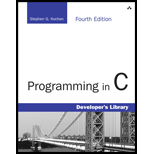
Concept explainers
Write a
C F

Explanation of Solution
Program:
The following program demonstrate the conversion of Fahrenheit into Celsius.
//include the header file
#include <stdio.h>
//definition of main method
int main (void)
{
//declare the required variables
float F, C;
//get the input from the user
printf (" Enter the temperature value in Fahrenheit: ");
scanf("%f", &F);
//convert Fahrenheit into Celsius
C = (F - 32) / 1.8;
//display the result
printf (" The Celsius: %f\n", C);
//return statement
return 0;
}
Explanation:
In the above program, declare the required header file. Inside the main method, get the temperature from the user then convert Fahrenheit into Celsius using given formula. Finally display the output.
Enter the temperature value in Fahrenheit: 27
The Celsius: -2.777778
Want to see more full solutions like this?
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Using MIS (10th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Starting out with Visual C# (4th Edition)
- Write a program named FahrenheitToCelsius that accepts a temperature in Fahrenheit from a user and converts it to Celsius by subtracting 32 from the Fahrenheit value and multiplying the result by 5/9. Display both values to one decimal place.arrow_forwardWrite a program to calculate velocity of a car when distance and time are given by the user.arrow_forwardThe following equation estimates the average calories burned for a person when exercising, which is based on a scientific journal article (source): Calories = ( (Age x 0.2757) + (Weight x 0.03295) + (Heart Rate x 1.0781) — 75.4991 ) x Time / 8.368 Write a program using inputs age (years), weight (pounds), heart rate (beats per minute), and time (minutes), respectively. Output the average calories burned for a person. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:print('Calories: {:.2f} calories'.format(calories)) Ex: If the input is: 49 155 148 60 then the output is: Calories: 736.21 calories ''' Calories = ((Age x 0.2757) + (Weight x 0.03295) + (Heart Rate x 1.0781) — 75.4991) x Time / 8.368 ''' ''' Type your code here. '''arrow_forward
- The following equation estimates the average calories burned for a person when exercising, which is based on a scientific journal article (source): Calories = ( (Age x 0.2757) + (Weight x 0.03295) + (Heart Rate x 1.0781) — 75.4991 ) x Time / 8.368 Write a program using inputs age (years), weight (pounds), heart rate (beats per minute), and time (minutes), respectively. Output the average calories burned for a person. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:print(f'calories: {calories:.2f} calories') Ex: If the input is: 49 155 148 60 then the output is: Calories: 736.21 caloriesarrow_forwardThe following equation estimates the average calories burned for a person when exercising, which is based on a scientific journal article (source): Calories = ( (Age x 0.2757) + (Weight x 0.03295) + (Heart Rate x 1.0781) — 75.4991 ) x Time / 8.368 Write a program using inputs age (years), weight (pounds), heart rate (beats per minute), and time (minutes), respectively. Output the average calories burned for a person. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:print(f'Calories: {calories:.2f} calories')arrow_forwardWrite a program accepts numerical value x from 0 to 100 as input and computes and displays the corresponding letter grade given by the following tablearrow_forward
- Translate the statement into equation: When three is multiplied by one less than a number x, the result is 10 less than 5 times the number x.arrow_forwardWrite a program named FahrenheitToCelsius that accepts a temperature in Fahrenheit from a user and converts it to Celsius by subtracting 32 from the Fahrenheit value and multiplying the result by the double 5.0/9.0. Display both values to one decimal place.In C#arrow_forwardWrite a program that prompts the user to input three (3) integers: MM, DD, and YYYY. For example, 07 26 1998. The program will print the number of days elapsed from 01 January of that year, i.e. January 1st is always 1 and December 31 is either 365 or 366, depending on if leap year or not.arrow_forward
- Write a program that converts Celsius temperatures to Fahrenheit temperatures. The formula isarrow_forwardWrite a program that converts U.S. dollars to Canadian dollars, German marks, and British pounds, as shown in Figure 36.18. The user enters the U.S. dollar amount and the conversion rate, and clicks the Convert button to display the converted amount.arrow_forwardUsing Python Write a program that displays all numbers divisible by 3 in the range 1 – 100arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
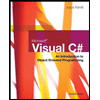
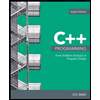
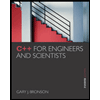
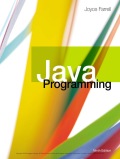