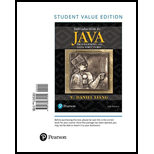
Assign grades
Program Plan:
- Import necessary packages into program.
- Define the class named “Exercise30_01”.
- Define main method.
- Define the “Scanner” object “obj” for input.
- Prompt the user and get the number of students “N” from user.
- Declare the array variable “marks[]” in type of “double”.
- Prompt and get scores using “for” loop.
- Using “DoubleStream” class assign maximum value into “best” variable.
- Declare the “grade” in type of “character”.
- Using “for” loop which is execute from “0” to “marks.length”.
- Using “if..elseif..else” condition, check the score value.
- If the value greater than “best-10”, assign “A” to “grade”.
- If the value greater than “best-20”, assign “B” to “grade”.
- If the value greater than “best-30”, assign “C” to “grade”.
- If the value greater than “best-40”, assign “D” to “grade”.
- Otherwise, assign “E” to “grade”.
- Print appropriate grade with statement on screen.
- Using “if..elseif..else” condition, check the score value.

The following JAVA code is to calculate the grade of student scores using “DoubleStream”.
Explanation of Solution
Program:
/*Include necessary packages*/
import java.util.Scanner;
//Include Stream package
import java.util.stream.DoubleStream;
//Class definition
class Exercise30_01
{
//Main method
public static void main(String[] args)
{
/*Definition of "Scanner" object*/
Scanner obj = new Scanner(System.in);
/*Prompt the user for number of students*/
System.out.print("Enter number of students: ");
//Get input from user
int N = obj.nextInt();
/*Declaration and definition of array variable*/
double[] marks = new double[N];
//Prompt the user for marks
System.out.print("Enter " + N + " scores: ");
//Loop
for (int i = 0; i < marks.length; i++)
{
/*Get scores and store into "marks[]" variable*/
marks[i] = obj.nextDouble();
}
/*Get maximum value using stream*/
double best = DoubleStream.of(marks).max().getAsDouble();
//Declaration of variable
char grade;
//Loop
for (int i = 0; i < marks.length; i++)
{
/*Condition to check marks*/
if (marks[i] >= best - 10)
//Assign grade
grade = 'A';
/*Condition to check marks*/
else if (marks[i] >= best - 20)
//Assign grade
grade = 'B';
/*Condition to check marks*/
else if (marks[i] >= best - 30)
//Assign grade
grade = 'C';
/*Condition to check marks*/
else if (marks[i] >= best - 40)
//Assign grade
grade = 'D';
//Else statement
else
//Assign grade
grade = 'F';
//Print statement
System.out.println("Student " + i + " score is " +marks[i] + " and grade is " + grade);
}
}
}
Enter number of students: 4
Enter 4 scores: 40 55 70 58
Student 0 score is 40.0 and grade is C
Student 1 score is 55.0 and grade is B
Student 2 score is 70.0 and grade is A
Student 3 score is 58.0 and grade is B
Want to see more full solutions like this?
Chapter 30 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version, Student Value Edition (11th Edition)
- has anybody tried to open a file, copy a function inside the program, and use it for a current program? If so could you show an example, nothing too complexarrow_forwardWill this loop run infinitely? Or will it stop? Why?data = [0, 2, 4, 6, 8]for i in range(len(data)):data.append(data[i] + 1)arrow_forwardCould you explain what the R code is doing below? # read in each table read_counts <- lapply(list_of_files, read.table, sep="\t", header = FALSE, skip =2) read_counts <- lapply(read_counts, function(x) x[, c(1,4)]) read_counts <- lapply(read_counts, function(x) x[complete.cases(x),]) # for each dataframe in read_counts transpose and then read_counts <- lapply(read_counts, function(x) t(x[,2]))arrow_forward
- Discuss the use of friend functions, when to use them, and how to specify them at compile and run time.make use of examplesarrow_forwardCan commonly used operators, such the arithmetic and stream operators, be accessed quickly thanks to the usage of enumeration types? Is it conceivable to get an acceptable result by, say, exceeding the capacity of these operators? What are the pluses and minuses of this method?arrow_forward4. What does this function return? Which principle does it illustrate?arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
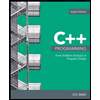