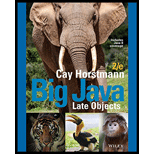
Big Java Late Objects
2nd Edition
ISBN: 9781119330455
Author: Horstmann
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 5, Problem 14PP
Program Plan Intro
Print calendar
Program plan:
- Import necessary package.
- Create a class “Calendar”,
- Define the function “is_LeapYear()”,
- Check whether the year is multiple of “400”,
- If it is true, return “true”.
- Check whether the year is not multiple of “100”,
- If it is true, return “false”.
- Check whether the year is multiple of “4”,
- If it is true, return “true”.
- Otherwise, return “false”.
- Check whether the year is multiple of “400”,
- Define the function “get_NDays()”,
- Check whether the month is “2”February,
- If it is “true”, Call the function to check whether the year is leap year,
- Return “29” as number of days.
-
- Otherwise,
- Return “28” as number of days.
- Otherwise,
- If it is “true”, Call the function to check whether the year is leap year,
- Check whether the month is either “4”(April) or “6”(June) or “9”(September) or “11”(November),
- Return “30” as number of days.
- Otherwise,
- Return “31” as number of days.
- Check whether the month is “2”February,
- Define “main()” function,
- Declare the necessary variables.
- Create “Scanner” object.
- Prompt the user to enter month and year.
- Get the month and year from the user.
- Assign the value return from “get_NDays()”.
- Print the heading.
- Create for loop to iterate over all days,
- Get the first day of month.
- Check whether day is “1”(Monday),
- Create “for” loop to iterate till the first day of month,
-
- Print space.
- Print the formatted output.
- Check whether the first day of month is “6”(Saturday),
- Print new line.
- Define the function “is_LeapYear()”,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a program that does two string functions: capitalize the first word of a phrase, and reverse the letters of words in odd positions within the phrase. For this program it is acceptable to let punctuation be part of the word. Write a capitalizeWords() and a reverseOddWords() method and call them in a test program. Make certain to properly handle empty phrases, phrases with one and two words, and phrases with greater than two words.
For example, if the original phrase were:
Learning Java is fun! Java is powerful and easy to use
The capitalized version would be:
Learning Java Is Fun! Java Is Powerful And Easy To Use
And with reversed odd words would be:
gninraeL Java sI Fun! avaJ Is lufrewoP And ysaE To esU
Write a program that reads HAND_SIZE cards from the user, then analyzes the cards and prints out the type of poker hand that they represent. (HAND_SIZE will be a global constant, typically 5, but your program must still work if it is set to something other than 5.)
Poker hands are categorized according to the following hand-types: Straight flush, four of a kind, full house, straight, flush, three of a kind, two pairs, pair, high card.
To simplify the program we will ignore card suits, and face cards. The values that the user inputs will be integer values from LOWEST_NUM to HIGHEST_NUM. (These will be global constants. We'll use LOWEST_NUM = 2 and HIGHEST_NUM = 9, but your program must work if these are set to something different.) When your program runs it should start by collecting HAND_SIZE integer values from the user and placing the integers into an array that has HAND_SIZE elements. It might look like this:
Enter 5 numeric cards, no face cards. Use 2 - 9.
Card 1: 8
Card 2: 7
Card…
Write a program that reads HAND_SIZE cards from the user, then analyzes the cards and prints out the type of poker hand that they represent. (HAND_SIZE will be a global constant, typically 5, but your program must still work if it is set to something other than 5.)
Poker hands are categorized according to the following hand-types: Straight flush, four of a kind, full house, straight, flush, three of a kind, two pairs, pair, high card.
To simplify the program we will ignore card suits, and face cards. The values that the user inputs will be integer values from LOWEST_NUM to HIGHEST_NUM. (These will be global constants. We'll use LOWEST_NUM = 2 and HIGHEST_NUM = 9, but your program must work if these are set to something different.) When your program runs it should start by collecting HAND_SIZE integer values from the user and placing the integers into an array that has HAND_SIZE elements. It might look like this:
Enter 5 numeric cards, no face cards. Use 2 - 9. Card 1: 8 Card 2: 7 Card…
Chapter 5 Solutions
Big Java Late Objects
Ch. 5.1 - Consider the method call Math.pow(3, 2). What are...Ch. 5.1 - What is the return value of the method call...Ch. 5.1 - The Math.ceil method in the Java standard library...Ch. 5.1 - It is possible to determine the answer to Self...Ch. 5.2 - What is the value of cubeVolume(3)?Ch. 5.2 - Prob. 6SCCh. 5.2 - Provide an alternate implementation of the body of...Ch. 5.2 - Declare a method squareArea that computes the area...Ch. 5.2 - Consider this method: public static int...Ch. 5.3 - What does this program print? Use a diagram like...
Ch. 5.3 - Prob. 11SCCh. 5.3 - What does this program print? Use a diagram like...Ch. 5.4 - Prob. 13SCCh. 5.4 - What does this method do? public static boolean...Ch. 5.4 - Implement the mystery method of Self Check 14 with...Ch. 5.5 - How do you generate the following printout, using...Ch. 5.5 - Prob. 17SCCh. 5.5 - Prob. 18SCCh. 5.5 - Prob. 19SCCh. 5.5 - The boxString method contains the code for...Ch. 5.6 - Consider the following statements: int...Ch. 5.6 - Consider this method that prints a page number on...Ch. 5.6 - Consider the following method that computes...Ch. 5.6 - The comment explains what the following loop does....Ch. 5.6 - In Self Check 24, you were asked to implement a...Ch. 5.7 - Explain how you can improve the intName method so...Ch. 5.7 - Prob. 27SCCh. 5.7 - What happens when you call intName(0)? How can you...Ch. 5.7 - Trace the method call intName(72), as described in...Ch. 5.7 - Prob. 30SCCh. 5.8 - Which lines are in the scope of the variable i...Ch. 5.8 - Which lines are in the scope of the parameter...Ch. 5.8 - The program declares two local variables with the...Ch. 5.8 - There is a scope error in the mystery method. How...Ch. 5.8 - Prob. 35SCCh. 5.9 - Consider this slight modification of the...Ch. 5.9 - Consider this recursive method: public static int...Ch. 5.9 - Consider this recursive method: public static int...Ch. 5.9 - Prob. 39SCCh. 5.9 - The intName method in Section 5.7 accepted...Ch. 5 - In which sequence are the lines of the Cubes.java...Ch. 5 - Write method headers for methods with the...Ch. 5 - Give examples of the following methods from the...Ch. 5 - Prob. 4RECh. 5 - Consider these methods: public static double...Ch. 5 - Prob. 6RECh. 5 - Design a method that prints a floating-point...Ch. 5 - Write pseudocode for a method that translates a...Ch. 5 - Describe the scope error in the following program...Ch. 5 - For each of the variables in the following...Ch. 5 - Prob. 11RECh. 5 - Perform a walkthrough of the intName method with...Ch. 5 - Consider the following method: public static int...Ch. 5 - Consider the following method that is intended to...Ch. 5 - Suppose an ancient civilization had constructed...Ch. 5 - Give pseudocode for a recursive method for...Ch. 5 - Give pseudocode for a recursive method that sorts...Ch. 5 - Write the following methods and provide a program...Ch. 5 - Write the following methods and provide a program...Ch. 5 - Prob. 4PECh. 5 - Prob. 5PECh. 5 - Prob. 6PECh. 5 - Prob. 7PECh. 5 - Prob. 8PECh. 5 - Write methods public static double...Ch. 5 - Write a recursive method public static String...Ch. 5 - Write a recursive method public static boolean...Ch. 5 - Use recursion to implement a method public static...Ch. 5 - Use recursion to determine the number of digits in...Ch. 5 - Write a method that computes the balance of a bank...Ch. 5 - Write a method that tests whether a file name...Ch. 5 - It is a well-known phenomenon that most people are...Ch. 5 - Prob. 3PPCh. 5 - Use recursion to compute an, where n is a positive...Ch. 5 - Leap years. Write a method public static boolean...Ch. 5 - In Exercise P3.13 you were asked to write a...Ch. 5 - Prob. 10PPCh. 5 - Write a program that reads two strings containing...Ch. 5 - Prob. 12PPCh. 5 - Write a program that reads words and arranges them...Ch. 5 - Prob. 14PPCh. 5 - Write a program that reads two fractions, adds...Ch. 5 - Write a program that prints the decimal expansion...Ch. 5 - Write a program that reads a decimal expansion...Ch. 5 - Write two methods public static void...Ch. 5 - Write a program that reads in the width and height...Ch. 5 - Repeat Exercise P5.19 with hexagonal circle...Ch. 5 - Postal bar codes. For faster sorting of letters,...Ch. 5 - Write a program that reads in a bar code (with :...Ch. 5 - Write a program that converts a Roman number such...Ch. 5 - A non-governmental organization needs a program to...Ch. 5 - Having a secure password is a very important...Ch. 5 - Prob. 30PPCh. 5 - Prob. 31PPCh. 5 - Electric wire, like that in the photo, is a...Ch. 5 - The drag force on a car is given by FD=12v2ACD...
Knowledge Booster
Similar questions
- Write a program in Java that creates a login name for a user, given the user's first name, last name, and a four-digit integer as input. Output the login name, which is made up of the first six letters of the first name, followed by the first letter of the last name, an underscore (_), and then the last digit of the number (use the % operator). If the first name has less than six letters, then use all letters of the first name. Ex: If the input is: Michael Jordan 1991 the output is: Your login name: MichaeJ_1 Ex: If the input is: Nicole Smith 2024 the output is: Your login name: NicoleS_4arrow_forwardWrite a Java program that would resemble a payroll system. An input window which will accept the employee number and the number of days of rendered work. A 2d-arraylist that contains the employee number and the rate per day is to be searched and used to compute for the gross salary. Gross salary is computed by multiplying the number of days of rendered work by rate per day of the particular employee. If the gross salary computed is greater than 100,000 the tax is 20% of the gross salary, otherwise, the tax is 10%. Deduct the tax from the gross salary to get the net pay. Display the employee number, gross salary, tax deduction, and the net pay in an output window. Design your own input/output windows. ( USE OOP CONCEPT AND JOPTION)arrow_forwardImplement the vacuum cleaning agent function in Python/Java for the scenario described below: Consider two-dimensional Boolean array of size m x n (m and n can each be equal to 4) where m is the number of rows, and n is the number of columns. Obtain numerical positive integer values for m and n as inputs from the user. Generate random numbers and scale them down to either a “0” or a “1” according to whether they represent “Clean” or “Dirty”, respectively. After filling the array with 0s or 1s, start at some random array position indicating the initial position of the vacuum cleaner. First check if at that current position, there is the presence of a “Dirt” or not determined by whether 0 or 1 is filled in at that initial position of the vacuum cleaner. If there is a “Dirt”, clean it, and if there is no “Dirt” do a No-operation. After this is done, generate random numbers modulo 4 to obtain random numbers 0, 1, 2, 3 indicating whether to take a left, right, up or down correspondingly. If…arrow_forward
- Write a program that prompts the user to enter adecimal number and displays the number in a fraction. (Hint: read the decimalnumber as a string, extract the integer part and fractional part from the string,and use the BigInteger implementation of the Rational class in Programming to obtain a rational number for the decimal number.) Here are somesample runs: Enter a decimal number: 3.25 ↵EnterThe fraction number is 13/4 Enter a decimal number: –0.45452 ↵EnterThe fraction number is –11363/25000arrow_forwardImplement a function alterCase in Python that converts a word to "alterCase". Given a word, using any mix of upper and lower case letters, the function then returns the same word, except that the first letter is upper case, the second is lower case, and then cases of letters alternate throughout the rest of the word. >>> alterCase('apple')'ApPlE'arrow_forwardWrite a Java program that asks the user to enter positive numbers, and store it into (myArray) of type int. Use any letter as a sentinel. Show the sum of all the numbers, total number of numbers and their average.arrow_forward
- Write a program that reads an integer age of kids, finds the maximum of them, and counts its occurances, finds the minimum of them, and counts its occurances. And Find the average ages. Assum that the input ends with number -1.arrow_forwardWrite a program that takes the details of a person (name, age, and city) and search for a specific name using linear search in java with outputarrow_forwardPlease implement in Java implement a keyed bag in which the items to be stored are strings (perhaps people’s names) and the keys are numbers (perhaps Social Security or other identification numbers). So, the in- sertion method has this specification: public void insert(String entry, int key);// Precondition: size( ) < CAPACITY, and the // bag does not yet contain any item// with the given key.// Postcondition: A new copy of entry has// been added to the bag, with the given key. When the programmer wants to remove or retrieve an item from a keyed bag, the key of the item must be specified rather than the item itself. The keyed bag should also have a boolean method that can be used to determine whether the bag has an item with a specified key. In a keyed bag, the pro- grammer using the class specifies a particular key when an item is inserted. Here’s an implementation idea: A keyed bag can have two private arrays, one that holds the string data and one that holds the corresponding…arrow_forward
- Write a program RandomWalker.java that takes an integer command-line argument r and simulates the motion of a random walk until the random walker is at Manhattan distance r from the starting point. Print the coordinates at each step of the walk (including the starting and ending points), treating the starting point as (0, 0). Also, print the total number of steps taken.arrow_forwardWrite a program that reads a number and finds it is a prime or not. 0. A prime number is a number such that 1 and itself are the only numbers that evenly divide it. For example: 3, 5,7, 11, 13, 17. 2.arrow_forwardIs there a way to create a java program on Branching and selecting method on problems like this? Suppose that we are working for an online service that provides a bulletin board for its users. We would like to give our users the option of filtering out profanity. Suppose that we consider the words cat dog and rabbit to be profane. Write a program that reads a string from the keyboard and test whether the string contain any one of these words. Your program should find words like cAt that differ only in case. Have your program reject only lines that contain one or more of the three words exactly. For example, concatenation is a small category should not be considered profane. This problem can be solved easily. If you add a space to the beginning of the string and a space at the end of the string you only need to check if space cat space or space dog space or space rabbit space is in the string. Use three if statements; one for each word. If one or more of the words are there set…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
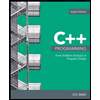
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning