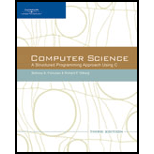
Concept explainers
Write a
To determine the day of the week, you will first need to calculate the day of the week for December 31 of the previous year. To calculate the day for December 31, use the following formula.
The formula determines the day based on the values as shown below.
Once you know the day for December 31, you simply calculate the days in the year before the month in question. Use a switch statement to make this calculation. If the desired month is 12, add the number of days for November (30). If it is 11, add the number of days for October (31). If it is 3, add the number of days for February (28). If it is 2, add the number of days for January (31). If you do not use a break between the months, the switch will add the days in each month before the current month.
To this figure, add the day in the current month and then add the result to the day code for December 31. This number modulo seven is the day of the week.
There is one more refinement. If the current year is a leap year, and if the desired date is after February, you need to add 1 to the day code. The following formula can be used to determine if the year is a leap year.
Your program should have a function to get data from the user, another to calculate the day of the week, and a third to print the result.
To test your program, run it with the following dates:

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Computer Science: A Structured Programming Approach Using C, Third Edition
- Write a program named GuessingGame that generates a random number between 1 and 10. (In other words, in the example above, min is 1 and max is 11.) Ask a user to guess the random number, then display the random number and a message indicating whether the users guess was too high, too low, or correct.arrow_forwardWrite a program named FahrenheitToCelsius that accepts a temperature in Fahrenheit from a user and converts it to Celsius by subtracting 32 from the Fahrenheit value and multiplying the result by 5/9. Display both values to one decimal place.arrow_forward(Program) Write a program to simulate the rolling of two dice. If the total of the two dice is 7 or 11, you win; otherwise, you lose. Embellish this program as much as you like with betting, different odds, different combinations for win or lose, stopping play when you have no money left or reach the house limit, displaying the dice, and so forth. (Hint: Calculate the dots showing on each die with theexpressiondots=(int)(6.0randomnumber+1), where random number is between 0 and 1.)arrow_forward
- Write a program that prompts the user to enter theminutes (e.g., 1 billion), and displays the number of years and remaining days forthe minutes. For simplicity, assume that a year has 365 days. Here is a sample run: Enter the number of minutes: 10000000001000000000 minutes is approximately 1902 years and 214 daysarrow_forwardWrite a program that asks the user how many credit units they have taken. If they have taken 23 or less, print that the student is a freshman. If they have taken between 24 and 53, print that they are a sophomore. The range for juniors is 54 to 83, and for seniors it is 84 and over.arrow_forwardWrite a program that checks to see if the user won the lottery. Assume the winning lottery numbers are 8, 13, 27, 53, and 54. Ask the user for five numbers and compare those numbers to the winning numbers to determine how many matches the user got. In a lottery, the order of the numbers doesn't matter.arrow_forward
- Write a program that inputs a number and checks whether it is a perfect number or not. A perfect number is the number that is numerically equal to the sum of its divisors. For example, 6 is a perfect number because the divisors of 6 are 1, 2, 3 and 1+2+3=6arrow_forwardWrite a program that reads three numbers and prints "increasing" if they are in increasing order, "decreasing" if they are in decreasing order, and "neither" otherwise. Here, "increasing" means "strictly increasing", where each value is less than the next. The sequence 3 4 4 would not be considered increasing, for example. PYTHONarrow_forwardWrite a program that can be used to calculate the federal tax. The tax is calculated as follows: For single people, the standard exemption is $4,000; for married people, the standard exemption is $7,000. A person can also put up to 6% of his or her gross income in a pension plan. The tax rates are as follows: If the taxable income is: Between $0 and $15,000, the tax rate is 15%. Between $15,001 and $40,000, the tax is $2,250 plus 25% of the taxable income over $15,000. Over $40,000, the tax is $8,460 plus 35% of the taxable income over $40,000. Prompt the user to enter the following information: Marital status If the marital status is “married,” ask for the number of children under the age of 14 Gross salary (If the marital status is “married” and both spouses have income, enter the combined salary.) Percentage of gross income contributed to a pension fund Your program must consist of at least the following functions: Function getData: This function asks the user to enter the…arrow_forward
- Write a program that can be used to calculate the federal tax. The tax is calculated as follows: For single people, the standard exemption is $4,000; for married people, the standard exemption is $7,000. A person can also put up to 6% of his or her gross income in a pension plan. The tax rates are as follows: If the taxable income is: Between $0 and $15,000, the tax rate is 15%. Between $15,001 and $40,000, the tax is $2,250 plus 25% of the taxable income over $15,000. Over $40,000, the tax is $8,460 plus 35% of the taxable income over $40,000. Prompt the user to enter the following information: Marital status If the marital status is “married,” ask for the number of children under the age of 14 Gross salary (If the marital status is “married” and both spouses have income, enter the combined salary.) Percentage of gross income contributed to a pension fundarrow_forwardWrite a program that can be used to calculate the federal tax. The tax is calculated as follows: For single people, the standard exemption is $4,000; for married people, the standard exemption is $7,000. A person can also put up to 6% of his or her gross income in a pension plan. The tax rates are as follows: If the taxable income is: Between $0 and $15,000, the tax rate is 15%. Between $15,001 and $40,000, the tax is $2,250 plus 25% of the taxable income over $15,000. Over $40,000, the tax is $8,460 plus 35% of the taxable income over $40,000. Prompt the user to enter the following information: Marital status If the marital status is “married,” ask for the number of children under the age of 14 Gross salary (If the marital status is “married” and both spouses have income, enter the combined salary.) Percentage of gross income contributed to a pension fund Your program must consist of at least the following functions: Function getData: This function asks the user to…arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
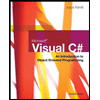
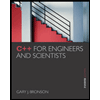
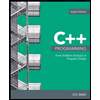