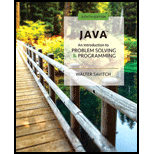
Write a program that will record the votes for one of two candidates by using the class VoteRecorder, which you will design and create. Vote Recorder will have static variables to keep track of the total votes for candidates and instance variables to keep track of the votes made by a single person. It will have the following attributes.
- nameCandidatePresident11—a static String that holds the name of the first candidate for president
- nameCandidatePresident2—a static string that holds the name of the second candidate for president
- nameCandidateVicePresident1 —a static string that holds the name of the first candidate for Vice president
- nameCandidateVicePresident2—a static string that holds the name of the second candidate for Vice president
- votesCondidatePresident1—a static integer that holds the number of votes for the first candidate for president
- votesCandidatePresident2—a static integer that holds the number of voles for the second candidate for president
- votesCandidateVicePresident1—a static integer that holds the number of votes for the first candidate for Vice president
- votesCandidateVicePresident2—a static integer that holds the number of rates for the second candidate for Vice president
- myVoteForPresident—an integer that holds the vote of a single individual for president (0 for no choice, 1 for the first candidate, and 2 for the second candidate)
- myVoteForVicePresident—an integer that holds the vote of a single individual for vice president (0 for no choice, 1 for the first candidate, and 2 for the second candidate)
In addition to appropriate constructors, VotRecorder has the following methods:
- setCandidaterPresident(String name1, String name2)—a static method that sets the names of the names of the two candidates for president
- getCandicateoVicePresident (String name1, string name2)—a static method that sets the names of the two candidates for vice president
- resetVotes—a static method that resets the vote counts to zero
- getCurrentVotePresident—a static method that returns a string with a current total number of votes for both presidential candidates
- getAndConfirmVotes—a non-static method that gets an individual’s votes, confirms then, and then records them
- getAVote(String name1, string name2)—a private method that returns a vote choice for a single race from a individual (0 for no choice, 1 for the first candidate, and 2 for the second candidate)
- getVotes—a private method that returns a vote choice for president and vice president from an individual
- confirmVotes—a private method that displays a person’s vote for president and vice president, asks whether the voter is happy with these choices, and returns true or false according to a yes-or-no response
- recordVotes—a private method that will add an individual’s votes to the appropriate static variables
Create a program that will candidates an election. The candidates for president are Annie and Bob. The candidates for vice president are John and Susan. Use a loop to record the votes of many voters. Create a new Vote Recorder object for each voter. After all the voters are done, present the results.

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Java: An Introduction To Problem Solving And Programming Plus Mylab Programming With Pearson Etext -- Access Card Package (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects
Computer Systems: A Programmer's Perspective (3rd Edition)
Starting out with Visual C# (4th Edition)
Absolute Java (6th Edition)
C How to Program (8th Edition)
Starting Out with Java: Early Objects (6th Edition)
- Given main(), define the Artist class (in file Artist.java) with constructors to initialize an artist's information, get methods, and a printInfo() method. The default constructor should initialize the artist's name to "unknown" and the years of birth and death to -1. printInfo() displays "Artist:", then a space, then the artist's name, then another space, then the birth and death dates in one of three formats: (XXXX to YYYY) if both the birth and death years are nonnegative (XXXX to present) if the birth year is nonnegative and the death year is negative (unknown) otherwise Define the Artwork class (in file Artwork.java) with constructors to initialize an artwork's information, get methods, and a printInfo() method. The default constructor should initialize the title to "unknown", the year created to -1. printInfo() displays an artist's information by calling the printInfo() method in Artist.java, followed by the artwork's title and the year created. Declare a private field of type…arrow_forwardCreate a simple java program that reads all the content in a .txt file, the user will enter the filename to be opened. In this case, the file will contain multiple lines of Car details with each line containing a single car detail. Your task is to then create a Car class that models the Make, Colour, type and year manufactured. The Car class should further be able to assign every car created with a unique serial number using a class variable[format is AAAYYYYCXX, AAA is the first 3 letters of the make, YYYY is the year it was manufactured, C is the first letter of the colour and XX is the value provided by the class variable(in form 01) all in upper caps see samples]. Within the driver class add all the cars read from the file onto a stack following LIFO approach and then print details of 2 cars from the stack that are older then 5 years. You are additionally expected to deal with most common exceptions(See samples below) [HINT: DO NOT PROVIDE A PATH BUT RATHER ONLY OPEN THE FILE WITH…arrow_forwardCreate a simple java program that reads all the content in a .txt file, the user will enter the filename to be opened. In this case, the file will contain multiple lines of Car details with each line containing a single car detail. Your task is to then create a Car class that models the Make, Colour, type and year manufactured. The Car class should further be able to assign every car created with a unique serial number using a class variable[format is AAAYYYYCXX, AAA is the first 3 letters of the make, YYYY is the year it was manufactured, C is the first letter of the colour and XX is the value provided by the class variable(in form 01) all in upper caps see samples]. Within the driver class add all the cars read from the file onto a stack following LIFO approach and then print details of 2 cars from the stack that are older then 5 years. You are additionally expected to deal with most common exceptions(See samples below) [HINT: DO NOT PROVIDE A PATH BUT RATHER ONLY OPEN THE FILE WITH…arrow_forward
- Write a program called “PatternMatcher.java” that may be used to search a dictionaryof words for those that match a given pattern. The program will interact with the user via a graphical user interface that has been suppliedto you in the WordSearch class.Your task is to implement an ActionListener type class designed to deal with the user input.The main program class in the WordSearch class responsible for building the GUIstructure, building a WordList from the file of words, and creating an ActionListener.An ActionListener will be responsible for dealing with the event where the user enters apattern in the JTextField and presses return. It will be responsible for1. when the user presses enter, obtaining the text from the field, and if valid, creating aPattern object,2. building an array of Object from the WordList containing words that match thePattern,3. putting the array of Object in the JList for displayarrow_forwardGiven the code below, write a Circle class (and save it in a file named Circle.java) that inherits from the Shape class. Include in your Circle class, a single private field double radius. Also include a method void setRadius(double r) (which also sets area) and a method double getRadius() (which also returns the current radius). Change the accessibility modifier for area in the Shape class to be more appropriate for a base class. Make sure that ShapeDriver's main() method executes and produces the following output: Shape: area: 78.53981633974483 radius: 5.0arrow_forwardCreate a new Python file in your project named FullName.py. In this module, create a class named FullName. When creating a FullName object, the client will pass a string for first name and a string for last name, which the constructor will assign to two attributes. Your class should have the following methods: __init__, __str__, and __gt__. __gt__ will compare two FullName objects using the following logic: If person A’s last name comes after person B’s last name alphabetically, person A is “greater than” person B. If their last names are equal, check their first names to see which is greater. Hint: The > and < operators are already overloaded for string objects, so your __gt__ method should be relatively simple. In your main.py file, demonstrate creating a few FullName objects and calling these methods on them.arrow_forward
- For the final take on this problem, we will use a class to solve the problem by reading the datafrom a text file. Prepare the text file as you wish if you can read it within your Java project.Name the 4Growth.txt. Don’t forget to close the file after you finish reading it!Create a new Java Project named PopCan04. Include a Jurisdiction class according to this UMLdiagram:You already have several pieces of its solution, the header is the same, the format specifiers arethe same, now, instead of using arrays, use an Array[] or an ArrayList<> of Jurisdictions toimplement the new solution. Be sure to include a method to calculate the population growth asdefines in the class diagram.Since you will be reading from a file, include a FileNotFoundException and catch it by printing amessage to the user: “File not Found.”The rest of the solution needs an enhanced for loop to produce the table. Finish by adding thefooter that you have been using all along. ***Population of provinces and…arrow_forwardWrite a class called DataPoint. A datapoint should have the following two properties: - name - The name should always be exactly 5 characters long. If a name that is too short is given, the name should be padded with additional 'X' characters to make it exactly 5 characters long. If a name is given that is too long the extra characters should be removed. Thus is a user supply 'AB' as the name, it should be padded to become 'ABXXX' whilst if a user gives 'ABCDEFG' as a name, it should be truncated (shortened) to 'ABCDE' - value - The value should always be between 0 and 100. You may assume a numeric value will be supplied. if the value that is given is less than 0, the value should be set to exactly 0 and a message should be printed stating 'The value must be greater than 0'. If a value of more than 100 is given, the value should be set to exactly 100 and a message should be printed stating 'The value must be less than 100' In addition to changing the properties given the above…arrow_forwardYou are contributing to a system that will help an ice cream shop to keep track of their product line and calculate the price of customer orders. In a Python file called icecream.py, write a class called IceCream that matches the following docstring: Part A: '''Class -- IceCreamRepresents a type of ice cream.Attributes:flavor -- The name of the flavor, a string e.g. "chocolate" or "cookies and cream"price_per_scoop -- The price per scoop, a float.Method:...See part B...''' Part B Add a method called get_cost to the IceCream class that will be used to calculate the cost of a customer's order. The method should take a float parameter, the number of scoops the customer wants, and return the total cost for that amount of ice cream. Here are the rules for calculating the ice cream cost: The cost of the ice cream is the number of scoops multiplied by the price per scoop. For less than 2 scoops, the cost is calculated as the price per scoop multiplied by 2. This means that an order for less…arrow_forward
- Write a program in Java to perform the following: Using a BufferedReader class and related objects, read a string object consists of 60 words plus some special characters and numbers. Include double space between some words. Include a few $ and % signs in your text. Store that text into a file using proper classes and methods. Call that file fileon.doc which will be your input file then read the contents of that file and perform the following operations using java available methods: Add a new line at the beginning of the text to include your name and the class name (csc 202) Find the length of you text Change the first character of each line to upper case . Find the number and location of all $ sign and re place it with a space. Delete all double spaces Calculate the number of special characters Find the number of lines in your text. Remember each line is terminated by a “.”, find the number of vowel in the last line Count the number of digits in your text. Append a new line consist…arrow_forwardGiven the class Pet below, write a client program to read data for five pets and display the following data: name of smallest pet, name of largest pet, and average weight of the five pets. Make sure you explain and justify every line of your code using internal documentation to get full mark. /**Class for basic pet data: name, age, and weight. */ public class Pet {private String name;private int age; //in years private double weight;//in kg public Pet(String initialName, int initialAge, double initialWeight) {name = initialName;if ((initialAge < 0) || (initialWeight < 0)) { System.out.println("Error: Negative age or weight."); System.exit(0); } else {age = initialAge; weight = initialWeight; } } public void setPet(String newName, int newAge, double newWeight) { name = newName;if ((newAge < 0) || (newWeight < 0)) {System.out.println("Error: Negative age or weight."); System.exit(0); } else {age = newAge; weight = newWeight; } } public Pet(String initialName) {name =…arrow_forwardWrite a class named DateExtract.cs, which will be used to extract the date of birth from the South African Identity Number. The class will have two methods, one for extracting date and the other one for validating the date.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
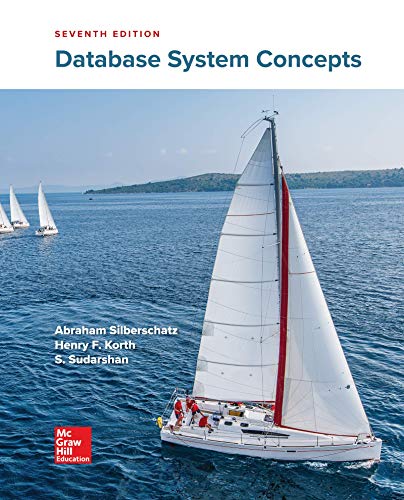
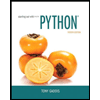
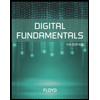
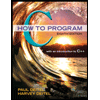
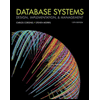
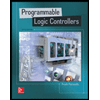