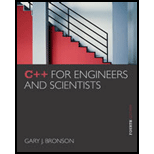
(a)
To write a function that calculate the area of a circle. It call another function to get the radius of the circle. The circumference of the circle is given.
(a)

Explanation of Solution
The name of the function is area().
This function has one parameters.
The parameter will accept afloating number to get the circumference (c) of a circle.
It call another function named radius () to get the radius (r) of the circle.
This function has one parameters.
The parameter will accept a floating number to get the circumference (c) of a circle.
The formula to calculate the radius of the circle is c / 2π.
The formula to calculate the area of the circle is πr2.
The function definition is:
float radius (float circumference) { //variable declaration float r; //calculate radius of the circle r = c / (2 * PI); //return radius return r; } //function to calculate area void area (float c) { //variable declaration float a, r; //get the radius of the circle by calling the function r = radius(c); //calculate area of the circle a = PI * pow (r, 2); //display radius of the circle cout<<"The radius of the circle is: "<< r <<endl; //display area of the circle cout<<"The area of the circle is: "<< a; }
(b)
- c,r,andavariables are used in the program.
- area () function is include in the program to calculate and display the area of the circle.
- radius () function is include in the program tocalculate and return the radius of the circle.
Program Description: The main purpose of the program is to prompt the user to enter the circumference of a circle to calculate the radius and the area of that circle. Include the function named area() in the main program. Call the function from the main () function with the value of the circumference of a circle.
(b)

Explanation of Solution
Program:
//including essential header file #include <iostream> #include <cmath> //define the value of PI (p) #define PI 3.14 //using standard namespace usingnamespace std; //function to calculate radius float radius (float circumference) { //variable declaration float r; //calculate radius of the circle r = circumference / (2 * PI); //return radius return r; } //function to calculate area void area (float c) { //variable declaration float a, r; //get the radius of the circle by calling the function r = radius(c); //calculate area of the circle a = PI * pow (r, 2); //display radius of the circle cout<<"The radius of the circle is: "<< r <<endl; //display area of the circle cout<<"The area of the circle is: "<< a; } //main function intmain() { //variable declaration float c; //prompt the user to enter the circumference of the circle cout<<"Enter the circumference of the circle: "; //get the circumference from the user cin>> c; //call the function area (c); return 0; }
Explanation: In the above code, themain() function is to prompt the user to enter the circumference of a circle. The user-entered circumference is stored in the variable named c. Then the main()function call a function named area()with the value of the circumference to calculate and display the radius and area of the circle. The function named area()has one parameter named c. The parameter named c stores the circumference of a circle passed by the main () function. The function namedarea()called another function to get the radius of the circle. Call the function named radius () with the circumference of a circle. The function named radius ()Calculate the radius of the circle by the given formula and return the radius of the circle.The function namedarea() calculates the area of the circle by the given formula and display the radius and area of the circle.
Sample Output:
Want to see more full solutions like this?
Chapter 6 Solutions
C++ for Engineers and Scientists
- (Practice) Determine the values of the following integer expressions: a.3+46f.202/( 6+3)b.34/6+6g.( 202)/6+3c.23/128/4h.( 202)/( 6+3)d.10( 1+73)i.5020e.202/6+3j.( 10+3)4arrow_forward(General math) The volume of oil stored in an underground 200-foot deep cylindrical tank is determined by measuring the distance from the top of the tank to the surface of the oil. Knowing this distance and the radius of the tank, the volume of oil in the tank can be determined by using this formula: volume=radius2(200distance) Using this information, write, compile, and run a C++ program that accepts the radius and distance measurements, calculates the volume of oil in the tank, and displays the two input values and the calculated volume. Verify the results of your program by doing a hand calculation using the following test data: radius=10feetanddistance=12feet.arrow_forward(Physics) a. The weight of an object on Earth is a measurement of the downward force onth e object caused by Earth’s gravity. The formula for this force is determined by using Newton’s Second Law: F=MAeFistheobjectsweight.Mistheobjectsmass.AeistheaccelerationcausedbyEarthsgravity( 32.2ft/se c 2 =9.82m/ s 2 ). Given this information, design, write, compile, and run a C++ program to calculate the weight in lbf of a person having a mass of 4 lbm. Verify the result produced by your program with a hand calculation. b. After verifying that your program is working correctly, use it to determine the weight, on Earth, of a person having a mass of 3.2 lbm.arrow_forward
- (Practice) Run Program 7.10 to determine the average and standard deviation of the following list of 15 grades: 68, 72, 78, 69, 85, 98, 95, 75, 77, 82, 84, 91, 89, 65, and 74.arrow_forward(Practice) a. To convert inches (in) to feet (ft), the number of inches should be multiplied by which of the following conversion factors? i. 12 in/1 ft ii. 1 ft/12 in b. To convert feet (ft) to meters (m), the number of feet should be multiplied by which of the following conversion factors? i. 1 m/3.28 ft ii. 3.28 ft/1 m c. To convert sq.yd to sq.ft, the number of sq.yd should be multiplied by which of the following conversion factors? i. 1 sq.yd/9 sq.ft ii. 9 sq.ft/1 sq.yd d. To convert meters (m) to kilometers (km), the number of meters should be multiplied by which of the following conversion factors? i. 1000 m/1 km ii. 1 km/1000 m e. To convert sq.in to sq.ft, the number of sq.in should be multiplied by which of the following conversion factors? i. 144 sq.in/1 sq.ft ii. 1 sq.ft/144 sq.in f. To convert minutes (min) to seconds (sec), the number of minutes should be multiplied by which of the following conversion factors? i. 60 sec/1 min ii. 1 min/60 sec g. To convert seconds (sec) to minutes (min), the number of seconds should be multiplied by which of the following conversion factors? i. 60 sec/1 min ii. 1 min/60 secarrow_forward(Practice) The volume, v, and side surface area, s, of a cylinder are given by these formulas: v=r2ls=2rl r is the cylinder’s radius, and l is its length. Using these formulas, write and test a function named cylinder() that accepts a cylinder’s radius and length and returns its volume and side surface area.arrow_forward
- (Physics) a. Design, write, compile, and run a C++ program to calculate the elapsed time it takes to make a 183.67-mile trip. This is the formula for computing elapsed time: elapsedtime=totaldistance/averagespeed The average speed during the trip is 58 mph. b. Manually check the values computed by your program. After verifying that your program is working correctly, modify it to determine the elapsed time it takes to make a 372-mile trip at an average speed of 67 mph.arrow_forward(General math) a. Design, write, compile, and run a C++ program to calculate the volume of a sphere with a radius, r, of 2 in. The volume is given by this formula: Volume=4r33 b. Manually check the values computed by your program. After verifying that your program is working correctly, modify it to determine the volume of a cube with a radius of 1.67 in.arrow_forwardHow should I answer the following regarding the first main function?arrow_forward
- My v and d are incorrect, please write the equations correctly.arrow_forwardAlso test the function in mainarrow_forward(Oceanography) The pressure, P, exerted on an underwater object can be determined by this formula: P=gh is the density of water, which is 1.94slug/ft3 . g is the acceleration caused by Earth’s gravity, which is 32.2ft/sec2. h is the object’s depth in the water in feet. a. Determine the units of P by calculating the units resulting from the right side of the formula. Check that your answer corresponds to the units for pressure listed in Table 1.1. b. Determine the pressure on a submarine operating at a depth of 2500 feet.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
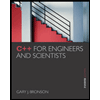