Explanation of Solution
a. Allocate an array of ten integers:
The following declaration statement allocates an array that can hold ten elements of type integers:
//Declare an array of ten integers
int[] values = new int[10];
Explanation:
In the above line,
- “int” refers to the data type of the array.
- “values” refers to the name of the array variable.
- “new” refers to a keyword which allocates a memory space.
- “int[10]” refers to the size of the array “10”.
The above line is used to allocate an integer array named “values” with size “10”
Explanation of Solution
b. The initial element of the array:
The statement to insert the integer value “17” into the initial element of the array values is as follows:
/*Insert the value 17 into the initial element of the array */
values[0] = 17;
Explanation:
The above line assigns the integer value “17” into the initial index (that is, 0th index) of the “values” array.
Explanation of Solution
c. The last element of the array:
The statement to insert the integer value “29” into the last element of the array values is as follows:
/*Insert the value 29 into the last element of the array */
values[9] = 29;
Explanation:
- The above line assigns the integer value “29” into the last index (that is, 9th index) of the “values” array.
Explanation of Solution
d. Fill the remaining elements with –1:
The below “for” loop shows how to fill the remaining elements in an array “values” with “1”:
// for loop to assign -1 to all elements of “values” array
for (int i = 1; i < 9; i++)
{
// Assign the value
values[i] = -1;
}
Explanation:
- At each iteration, the above “for” loop iterates through each element in the “values” array.
- In the “for” loop, initially “i = 0” and the loop will execute until the value of “i” is less than “9”.
- Inside the “for” loop, at each iteration, the value “-1” is stored into “values[i]”.
- At last, the loop will get terminated when it reaches “9 < 9”.
Explanation of Solution
e. Add 1 to each element of the array:
The below “for” loop shows how to add 1 to each element of the array “values” :
/* for loop to compute “values[i] + 1” and store the result into “values[i]” */
for (int i = 0; i < values.length; i++)
{
//calculate the values
values[i] = values[i] + 1
}
Explanation:
- At each iteration, the above “for” loop iterates through each element in the “values” array.
- In the “for” loop, initially “i = 0” and the loop will execute until the value of “i” is less than “values.length”.
- Inside the “for” loop, it calculates “value[i] + 1” and stores the result into “values[i]”.
- At last, the loop will get terminated when it reaches “10 < 10”.
Explanation of Solution
f. Print all elements of the array, one per line:
The below “for” loop shows how to print all elements of the array “values”, one per line :
/* for loop to print all elements of the array */
for (int value : values)
{
/*print the value of all elements in the array */
System.out.println(value);
}
Explanation:
- At each iteration, the given enhanced “for” loop iterates through each “element” in the “values” array.
- The print statement “System.out.println” prints the values of all elements in the “values” array, one per line.
Explanation of Solution
g. Print all elements of the array in a single line, separated by commas:
Consider the below “for” loop to print all the elements in the array “values” in a single line, separated by commas except the last element:
// for loop to print the array values
for (int i = 0; i < values.length; i++)
{
/* checks whether i is less than values.length-1 or not */
if (i < values.length - 1)
/print the values with separator
System.out.print(values[i] + ",");
else
/print the values
System.out.print(values[i]);
}
Explanation:
- In the “for” loop, initially “i = 0” and the loop will execute until “i < values.length”.
- Inside the “for” loop, the conditional statement “if (i < values.length-1))” checks whether “i” value is less than the length of the array or not.
- If the condition is true, then the print statement “System.out.print(values[i] + ",");” prints the values of “values” array separated by commas.
- The else part will print the last element of the array.
- Inside the “for” loop, the conditional statement “if (i < values.length-1))” checks whether “i” value is less than the length of the array or not.
Want to see more full solutions like this?
Chapter 6 Solutions
BIG JAVA, LATE OBJECTS LL W/ EPUB
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
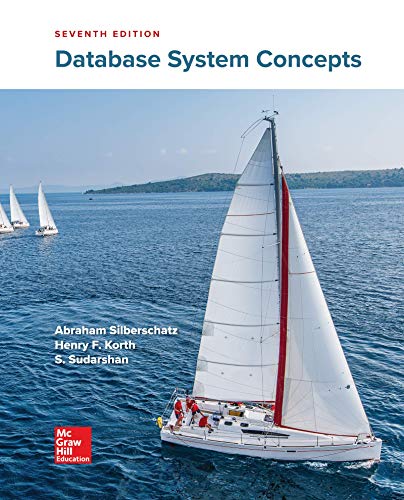
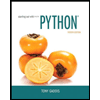
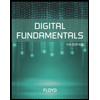
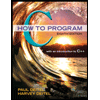
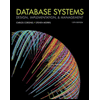
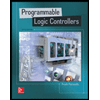