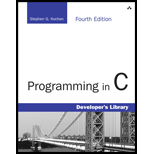
Programming in C
4th Edition
ISBN: 9780321776419
Author: Stephen G. Kochan
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 6, Problem 3E
Program Plan Intro
Program Plan:
- Include required header files.
- Declare the function “main ()”.
- Declare required variables “ratingCounters [11]”, “i”, “j”, “response”.
- Loop from 1 through 10.
- Assign “0” to the array index.
- Do until the “while” condition “response!=999” fails.
- Get the response from the user.
- Check the condition “response < 1 || response > 10”.
- Print the error message.
- Else, increment the counter.
- Increment the variable “i”.
- Loop from 1 through 10
- Print the number of responses.
- Return the statement.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Use C++
Write a program that reads a list of integers, and outputs whether the list contains all even numbers, odd numbers, or neither. The input begins with an integer indicating the number of integers that follow.
Ex: If the input is:
5 2 4 6 8 10
the output is:
all even
Ex: If the input is:
5 1 -3 5 -7 9
the output is:
all odd
Ex: If the input is:
5 1 2 3 4 5
the output is:
not even or odd
Your program must define and call the following two functions. IsVectorEven returns true if all integers in the array are even and false otherwise. IsVectorOdd returns true if all integers in the array are odd and false otherwise.bool IsVectorEven(vector<int> myVec)bool IsVectorOdd(vector<int> myVec)
Loops, C++
Write a program whose input is two integers, and whose output is the first integer and subsequent increments of 5 as long as the value is less than or equal to the second integer.
Ex: If the input is:
-15 10
the output is:
-15 -10 -5 0 5 10
Ex: If the second integer is less than the first as in:
20 5
the output is:
Second integer can't be less than the first.
For coding simplicity, output a space after every integer, including the last.
Use C++
Write a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follow. Then, get the last value from the input, and output all integers less than or equal to that value.
Ex: If the input is:
5 50 60 140 200 75 100
the output is:
50 60 75
The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75. For coding simplicity, follow every output value by a space, including the last one.
Such functionality is common on sites like Amazon, where a user can filter results.
Write your code to define and use two functions:vector<int> GetUserValues(vector<int>& userValues, int numValues)void OutputIntsLessThanOrEqualToThreshold(vector<int> userValues, int upperThreshold)
Utilizing functions helps to make main() very clean and intuitive.
Chapter 6 Solutions
Programming in C
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Python 3.7.4: Write a loop that reads strings from standard input, where the string is either "duck" or "goose". The loop terminates when "goose" is read in. After the loop, your code should print out the number of "duck" strings that were read. ----------------- This is what I tried so far, and it's been marked wrong by MyProgrammingLab: count = 0inputstring=input("Enter goose/duck: ")countducks=0while inputstring!="duck": countducks+=1 inputstring=input("Enter either the word goose or duck: ")print(countducks, "This is the number of times duck was entered:" count)arrow_forwardQuestion 2: Write a program to read student ID and check whether it is odd/even number. Output a 4X4 badge if the student ID is even number and output a 3X3 badge if it is odd number. The badge is built up by the (height X width) of asterisks. (Use for-loop to draw the asterisks, do not hard-coded)arrow_forward(Use Dev C++) Write a program that reads a list of scores between 0 and 100 and finds and prints the smallest and the largest scores. The program needs to ask for the number of the scores from the user; thisnumber must be less than or equal to 10arrow_forward
- Consider again a list of fridge temperatures that should be kept within a certain range, as described in Question 9. Write a program that prints the percentage of readings that are outside the allowed range (0 to 5 degrees Celsius), as shown in the examples below. Note that the percentage is printed as an integer, rounded down, and that there is a space before the percentage sign. You need to decompose this problem into sub-problems and instantiate and combine the corresponding patterns. If you need to compute the length or sum of a list, you may use the list operations len() and sum() instead of programming them from scratch using the counting and aggregator patterns. Use variable names appropriate to the problem at hand instead of the generic names used by the patterns. Use the get_input() operation to get the input list. For example: Input Result [5, 4, 3, 2, -1, 0] 16 % of values are outside the range [4.7, 5.2] 50 % of values are outside the range…arrow_forward6.25 In Python, Write a program that gets a list of integers from input, and outputs non-negative integers in ascending order (lowest to highest). Ex: If the input is: 10 -7 4 39 -6 12 2 the output is: 2 4 10 12 39 For coding simplicity, follow every output value by a space. Do not end with newline.arrow_forwardCan you write a new code in C ++ language with the values I sent you, just like this output? There are two files named group1.txt and group2.txt that contain course information and grades of each student for each class. I will calculate each course average for each group and show in simple bar graph. Use "*" and "#"characters for group1 and group2, respectively. I will see the number -999 at the end of each line in the input files. This value is used for line termination and you can use it to verify that you have arrived at the end of the line. The averages of each group should also be calculated and printed at the end of the file. All of the results will be printed to the file. There will be no screen output. Sample output is shown in Figure 1. Group 1: CSC 80 100 70 80 72 90 89 100 83 70 90 73 85 90 -999ENG 80 90 80 94 90 74 78 63 83 80 90 -999HIS 90 70 80 70 90 50 89 83 90 68 90 60 80 -999MTH 74 80 75 89 90 73 90 82 74 90 84 100 90 79 -999PHY 100 83 93 80 63 78 88…arrow_forward
- A criticism of the break statement and the continue statement is that each is unstructured.Actually, break statements and continue statements can always be replaced by structured statements, although doing so can be awkward. Describe in general how you would remove any breakstatement from a loop in a program and replace that statement with some structured equivalent.[Hint: The break statement leaves a loop from within the body of the loop. The other way to leaveis by failing the loop-continuation test. Consider using in the loop-continuation test a second testthat indicates “early exit because of a ‘break’ condition.”] Use the technique you developed here toremove the break statement from the program of Fig. 4.11arrow_forwardQ19: Write a program to read a set of (5) real no.s and find out thesum and average of them.arrow_forwardRemove the minor errors from this program.pleasearrow_forward
- Write a program to create the following figure by using nested for loops. Please assign the number of lines as a class constant variable in order to change the size of the figure as in the sample figure.arrow_forward4. Write a program that prints all the composers in the list['Rzewski', 'Ruggles', 'Carter', 'Stockhausen','Stravinsky']whose name has at least 10 characters.>>> ========================= RESTART=========================>>>StockhausenStravinskyarrow_forwardmake the given program runarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
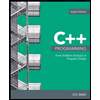
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning