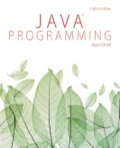
Explanation of Solution
Program:
File name: “Team.java”
//Define a class named Team
public class Team
{
//Declare the private variables
private String name;
private String sport;
private String mascot;
//Initialize a final static variable
public final static String MOTTO = "Sportsmanship!";
//Define a Team method
public Team(String name, String sport, String mascot)
{
//Refers to the instance variables
this.name = name;
this.sport = sport;
this.mascot = mascot;
}
//Define a get method to display the name
public String getName()
{
//Return the value
return name;
}
//Define a get method to display the sport
public String getSport()
{
//Return the value
return sport;
}
//Define a get method to display the mascot
public String getMascot()
{
//Return the value
return mascot;
}
}
File name: “Game.java”
//Define a class named Game
public class Game
{
//Declare the private variables
private Team team1;
private Team team2;
private String time;
//Define a Game method
public Game(Team t1, Team t2, String time)
{
team1 = t1;
team2 = t2;
/*If the two teams in a game have the same value for the sport*/
if(t1.getSport().equals(team2.getSport()))
//Refers to the instance variables
this.time = time;
/*Else if the two teams in a game do not
have the same value for the sport*/
else
//Refers to the instance variables
this...

Trending nowThis is a popular solution!

- In main(), prompt the user for two items and create two objects of the ItemToPurchase class. Before prompting for the second item, call scnr.nextLine(); to allow the user to input a new string.Ex: Item 1 Enter the item name: Chocolate Chips Enter the item price: 3 Enter the item quantity: 1 Item 2 Enter the item name: Bottled Water Enter the item price: 1 Enter the item quantity: 10arrow_forwardPlease provide JAVA source code for following assignment with proper comments. Please use basic While/for/ case/ loop for source code, I just started learning and doesn't know a lot about computer programming. 1. Employee Class Write a class named Employee that has the following fields: name. The name field references a String object that holds the employee’s name. idNumber. The idNumber is an int variable that holds the employee’s ID number. department. The department field references a String object that holds the name of the department where the employee works. position. The position field references a String object that holds the employee’s job title. The class should have the following constructors: A constructor that accepts the following values as arguments and assigns them to the appropriate fields: employee’s name, employee’s ID number, department, and position. A constructor that accepts the following values as arguments and assigns them to the appropriate…arrow_forwardI need to make a Java Application in Apache Netbeans 12.5 , I would like to ask for help please. The question is as follows: To encourage good grades, Hermosa High School has decided to award each student a bookstore credit that is 10 times the student’s grade point average. In other words, a student with a 3.2 grade point average receives a R 32 credit. Create a class that prompts a student for a name and grade point average, and then passes the values to a method that displays a descriptive message. The message uses the student’s name, echoes the grade point average, and computes and displays the credit. Save the application as BookstoreCredit.java.arrow_forward
- The local Driver’s License Office has asked you to write a program that grades the written portion of the driver’s license exam. The exam has 20 multiple choice questions. Here are the correct answers: 1. B 6. A 11. B 16. C 2. D 7. B 12. C 17. C 3. A 8. A 13. D 18. B 4. A 9. C 14. A 19. D 5. C 10. D 15. D 20. A A student must correctly answer 15 of the 20 questions to pass the exam. Write a class named DriverExam that holds the correct answers to the exam in an array field. The class should also have an array field that holds the student’s answers. The class should have the following methods: • passed. Returns true if the student passed the exam, or false if the student failed. • totalCorrect. Returns the total number of correctly answered questions. • totalIncorrect. Returns the total number of incorrectly answered questions. • questionsMissed. An int array containing the question numbers of the questions that the student missed. Demonstrate the class in a complete…arrow_forwardJava question please Create a class called Account that has the following attributes: Account Client - AccountHolder Double - Balance Date - DateCreated Account() Account(Client AccountHolder, Double Balance) getAccountHolder() - Client setAccountHolder(Client) - void getBalance() - Double deposit(Double) - Double withdrawal(Double) - Double getDateCreated() - Date toString() - String compareTo(Account) - Integer equals(Account) - Double Notes: The account holder attribute will link to the Account class that you created in problem 6. Your constructor and overloaded constructor should set the date created to the current date. Your deposit method should add to the balance Your withdrawal method should remove from the balance, as long as there is enough money to withdrawalarrow_forwardCreate class for Electricity for N flats of an apartment. The required details are Flat number, EB meter number, Owner name, Previous month reading( in units), current month reading ( in Units), amount. Except amount and EB meter number remaining values will be accepted from the user. EB meter number could be random number generated between 10000 to 20000. Amount will be calculates as below: 0-100 Minimum charges =100 100-500 2.5 ₹ per unit 500-1000 5.0 ₹ per unit Above 1000 7.5 ₹ per unit Write appropriate functions to perform the following: · Display the flat and owner details who paid maximum and minimum EB charges. · Display the average amount paid by all flats in each floor. Floor will be identified from the first digit of a flat number. For 1001 – first floor , 2005 – second floor, 5010- Fifth floor. Display the floor wise details. · Display the Flat number, EB amount along with the meter number in the ascending order of the meter number. note: java…arrow_forward
- Please create a code that follows the directions below exactly, and have the output look just like the one in the picture provided. If possible, please refrain from using switch statements in the code. Insturctions: This assignment will assess your understanding of void and value returning static methods. Your cousin owns an automotive maintenance shop that performs routine maintenance on cars. He has asked you to write a program that will provide a list of services (and their respective prices) to users and allow them to choose any, or all, services they would like and display the final price, including a 28% labor charge, a 9% markup if the car is an import, and an 8% sales tax. Task To accomplish the above, do the following: Write the two methods outlined below. Test your program and screenshot your successful test. carMaintenance method This method should accept the make of the car as a parameter (e.g., BMW, Ford, Ferrari, etc.). It should display the services and their prices as…arrow_forwardHello, I need help with this: – AssignmentFollowing on the success of homework 2, your mother wants you to augment the tag sale price trackingprogram. In addition to storing the price of each item sold, she wants to store the name of the item sold.Create a class called TagSaleItem, with the following variables (properties) and Get/Set members(functions)Double itemPriceString itemNameAdd a textbox to your form to input the name of the item at the same time as the price.The previous program used an array to store a list of prices. It would be awesome if this program usedan array of objects to store a list of items… but we’ll get to that in a later assignment. For now theassignment is just to create a class and a single object and demonstrate how to use it (using the Get/Setmethods) Code for homework 2 that is referenced: Imports System.IO Public Class FrmMain Dim prices(0) As Double ' Array to store prices of items sold Dim totalSales As Double ' Total amount of money collected…arrow_forwardThe Huntington Boys and Girls Club is conducting a fundraiser by selling chili dinners to go. The price is $7 for an adult meal and $4 for a child’s meal. Write a class that accepts the number of each type of meal ordered and display the total money collected for adult meals, children’s meals, and all meals. Save the class as ChiliToGo.java.arrow_forward
- I need help! Modify the class named FormLetterWriter that includes two overloaded methods named displaySalutation(). The first method takes one String parameter that represents a customer’s last name, and it displays the salutation Dear Mr. or Ms. followed by the last name. The second method accepts two String parameters that represent a first and last name, and it displays the greeting Dear followed by the first name, a space, and the last name. After each salutation, display the rest of a short business letter using the displayLetter method: Thank you for your recent order. public class FormLetterWriter {public static void main(String[] args) {displaySalutation("Kelly");displayLetter();displaySalutation("Christy", "Johnson");displayLetter();} public static void displaySalutation(String lastName) {// Write your code here} public static void displaySalutation(String firstName, String lastName) {// Write your code here}public static void displayLetter() {// Write your code here}}arrow_forwardModify the class named FormLetterWriter that includes two overloaded methods named displaySalutation(). The first method takes one String parameter that represents a customer’s last name, and it displays the salutation Dear Mr. or Ms. followed by the last name. The second method accepts two String parameters that represent a first and last name, and it displays the greeting Dear followed by the first name, a space, and the last name. After each salutation, display the rest of a short business letter using the displayLetter method: Thank you for your recent order. An example of the program is shown below: Dear Mr. or Ms. Kelly, Thank you for your recent order. Dear Christy Johnson, Thank you for your recent order.arrow_forwardModify the class named FormLetterWriter that includes two overloaded methods named displaySalutation(). The first method takes one String parameter that represents a customer’s last name, and it displays the salutation Dear Mr. or Ms. followed by the last name. The second method accepts two String parameters that represent a first and last name, and it displays the greeting Dear followed by the first name, a space, and the last name. After each salutation, display the rest of a short business letter using the displayLetter method: Thank you for your recent order. An example of the program is shown below: Dear Mr. or Ms. Kelly, Thank you for your recent order. Dear Christy Johnson, Thank you for your recent order. public class FormLetterWriter { public static void main(String[] args) { displaySalutation("Kelly"); displayLetter(); displaySalutation("Christy", "Johnson"); displayLetter(); } public static void displaySalutation(String…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
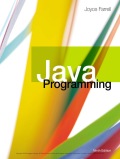
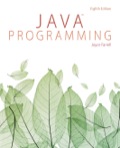