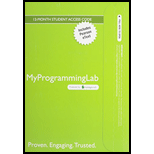
Java : Introduction To Prob...-MyProgrammingLab
15th Edition
ISBN: 9780133860771
Author: SAVITCH
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 7, Problem 11PP
Program Plan Intro
Sudoku puzzle
Program plan:
- Include required header files.
- Define the “SudokuPuzzle” class.
- Declare the two-dimensional array variables.
- In the SudokuPuzzle() constructor,
- Create objects of SudokoPuzzle class.
- Define the main() method.
- Create an object for Scanner class.
- Create an object for SudokoPuzzle class.
- Call initializePuzzle() method to initialize the puzzle.
- Display the puzzle values.
- The while loop executes until the true. If yes,
- Check whether the input is “q”. If yes, exit the program.
- Otherwise, check whether the input is “s”. If yes, set the guess value.
- Otherwise, check whether the input is “g”. If yes, get the allowed values.
- Otherwise, check whether the input is “c”. If yes, clear the puzzles.
- Check whether the puzzle value is not valid. If yes, display the error message.
- Otherwise, check whether the puzzle is full. If yes, display the success message.
- In the toString() method,
- Return the puzzle representation.
- In the addInitial() method,
- Check whether row and col is in between “0” to “9” and value is in between “1” to “9”. If yes,
- Set the puzzle values and the square value cannot be changed by puzzle solver.
- Check whether row and col is in between “0” to “9” and value is in between “1” to “9”. If yes,
- In the addGuess() method,
- Check whether row and col is in between “0” to “9” and value is in between “1” to “9” and start puzzle with “0”. If yes, set the guess value to puzzle.
- In the getValueIn() method,
- Return the value in given square.
- In the reset() method,
- Reset the puzzle value to blank.
- In the isFull() method,
- Check whether the puzzle is full and then set the flag value as “true”.
- In the getAllowedValues() method,
- Display the allowed values for puzzles.
- In the checkPuzzle() method,
- Check whether the values in the squares are legal. If yes, return true.
- In the checkRow() method,
- Check whether value is appears only once in row. If yes, return true.
- In the checkCol() method,
- Check whether value is appears only once in col. If yes, return true.
- In the checkSub() method,
- Check whether value is appears only once in 3 by 3 sub-array. If yes, return true.
- In the initializePuzzle() method,
- Call addInitial() method to initialize the puzzle to the board configuration example in the text.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Sudoku is a popular logic puzzle that uses a 9 by 9 array of squares that are organized into 3 by 3 subarrays. The puzzle solver must fill in the squares with the digits 1 to 9 such that no digit is repeated in any row, any column, or any of the nine 3 by 3 subgroups of squares.
Initially, some squares are filled in already and cannot be changed. For example, the following might be a starting configuration for a Sudoku puzzle:
Create a class SudokuPuzzle.java Download SudokuPuzzle.java that has the attributes
• board—a 9 by 9 array of integers that represents the current state of the puzzle, where 0 indicates a blank square
• start—a 9 by 9 array of boolean values that indicates which squares in board are given values that cannot be changed and the following methods:
• SudokuPuzzle—a constructor that creates an empty puzzle
• toString—returns a string representation of the puzzle that can be printed
• addInitial(row, col, value)—sets the given square to the given value as an…
Knight's Tour: The Knight's Tour is a mathematical problem involving a knight on a chessboard. The knight is placed on the empty board and, moving according to the rules of chess, must visit each square exactly once. There are several billion solutions to the problem, of which about 122,000,000 have the knight finishing on the same square on which it begins. When this occurs the tour is said to be closed. Your assignment is to write a program that gives a solution to the Knight's Tour problem recursively. You must hand in a solution in C++ AND Java. The name of the C++ file should be "main.cc" and the name of the Java file should be "Main.java". Write C++ only with a file name of main.cc Please run in IDE and check to ensure that there are no errors occuring
Output should look similar to:
1 34 3 18 49 32 13 16 4 19 56 33 14 17 50 31 57 2 35 48 55 52 15 12 20 5 60 53 36 47 30 51 41 58 37 46 61 54 11 26 6 21 42 59 38 27 64 29 43 40 23 8 45 62 25 10 22 7 44 39 24 9 28 63
JAVA - Multi Dimensional Arrays
Ask the user for the number of rows and columns of a two-dimensional array and then its integer elements. Print the row number of the row where the sum of all of its elements is even.
For this problem, it is guaranteed that there is one and only one row of such case. Row count starts at 0.
Input
1. One line containing an integer for the number of rows
2. One line containing an integer for the number of columns
3. Multiple lines containing at least one integer each line for the elements of the array
Sample
2 7 4
1 1 2
0 5 0
Output
Note that the row number starts at 0, not 1.
Enter # of rows: 3
Enter # of columns: 3
Enter elements:
2 7 4
1 1 2
0 5 0
Even row: 1
Chapter 7 Solutions
Java : Introduction To Prob...-MyProgrammingLab
Ch. 7.1 - What output will be produced by the following...Ch. 7.1 - What output will be produced by the following...Ch. 7.1 - What output will be produced by the following...Ch. 7.1 - Consider the following array: int [] a = new...Ch. 7.1 - What is wrong with the following code to...Ch. 7.1 - Write a complete Java program that reads 20 values...Ch. 7.2 - Write some Java code that will declare an array...Ch. 7.2 - Rewrite the method displayResults of the program...Ch. 7.2 - What output will be produced by the following...Ch. 7.2 - Give the definition of a static method called...
Ch. 7.2 - Give the definition of a static method called...Ch. 7.2 - Prob. 12STQCh. 7.2 - The following method compiles and executes but...Ch. 7.2 - Suppose that we add the following method to the...Ch. 7.3 - Prob. 15STQCh. 7.3 - Replace the last loop in Listing 7.8 with a loop...Ch. 7.3 - Suppose a is an array of values of type double....Ch. 7.3 - Suppose a is an array of values of type double...Ch. 7.3 - Prob. 19STQCh. 7.3 - Consider the partially filled array a from...Ch. 7.3 - Repeat the previous question, but this time assume...Ch. 7.3 - Write an accessor method getEntryArray for the...Ch. 7.4 - Prob. 23STQCh. 7.4 - Write the invocation of the method selectionSort...Ch. 7.4 - How would you need to change the method...Ch. 7.4 - How would you need to change the method...Ch. 7.4 - Consider an array b of int values in which a value...Ch. 7.5 - What output is produced by the following code?...Ch. 7.5 - Revise the method showTable in Listing 7.13 so...Ch. 7.5 - Write code that will fill the following array a...Ch. 7.5 - Write a void method called display such that the...Ch. 7.6 - Prob. 33STQCh. 7 - Write a program in a class NumberAboveAverage that...Ch. 7 - Write a program in a class CountFamiles that...Ch. 7 - Write a program in a class CountPoor that counts...Ch. 7 - Write a program in a class FlowerCounter that...Ch. 7 - Write a program in a class characterFrequency that...Ch. 7 - Create a class Ledger that will record the sales...Ch. 7 - Define the following methods for the class Ledger,...Ch. 7 - Write a static method isStrictlyIncreasing (double...Ch. 7 - Write a static method removeDuplicates(Character[]...Ch. 7 - Write a static method remove {int v, int [] in}...Ch. 7 - Suppose that we are selling boxes of candy for a...Ch. 7 - Create a class polynomial that is used to evaluate...Ch. 7 - Write a method beyond LastEntry (position) for the...Ch. 7 - Revise the class OneWayNoRepeatsList, as given in...Ch. 7 - Write a static method for selection sort that will...Ch. 7 - Overload the method selectionSort in Listing 7.10...Ch. 7 - Revise the method selectionSort that appears in...Ch. 7 - Prob. 18ECh. 7 - Write a sequential search of an array of integers,...Ch. 7 - Write a static method findFigure (picture,...Ch. 7 - Write a static method blur (double [] [] picture)...Ch. 7 - Write a program that reads integers, one per line,...Ch. 7 - The following code creates a small phone book. An...Ch. 7 - Write the method rotateRight that takes an array...Ch. 7 - The following code creates a ragged 2D array. The...Ch. 7 - Write a program that will read a line of text that...Ch. 7 - Prob. 2PPCh. 7 - Add a method bubbleSort to the class ArraySorter,...Ch. 7 - Add a method insertionSort to the class...Ch. 7 - The class TimeBook in Listing 7.14 is not really...Ch. 7 - Define a class called TicTacToe. An object of type...Ch. 7 - Repeat Programming Project 10 from Chapter 5 but...Ch. 7 - Prob. 8PPCh. 7 - Write a GUI application that displays a picture of...Ch. 7 - ELIZA was a program written in 1966 that parodied...Ch. 7 - Prob. 11PPCh. 7 - Create a GUI application that draws the following...Ch. 7 - Practice Program 2 used two arrays to implement a...Ch. 7 - Practice Program 5.4 asked you to define Trivia...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- A magic square is a square array of non-negative integers where the sums of the numbers on each row,each column, and both main diagonals are the same. An N × N occult square is a magic square with Nrows and N columns with additional constraints:• The integers in the square are between 0 and N, inclusive.• For all 1 ≤ i ≤ N, the number i appears at most i times in the square.• There are at least two distinct positive integers in the square.For example, the following is a 5 × 5 occult square, where the sums of the numbers on each row, eachcolumn, and both main diagonals are 7:0 0 0 3 42 4 0 0 10 0 3 4 05 0 0 0 20 3 4 0 0For a given prime number P, you are asked to construct a P × P occult square, or determine whether nosuch occult square exists.InputInput contains a prime number: P (2 ≤ P ≤ 1000) representing the number of rows and columns in theoccult square.OutputIf there is no P × P occult square, simply output -1 in a line. Otherwise, output P lines, each contains Pintegers…arrow_forwardA popular word game involves finding words from a grid of randomly generatedletters. Words must be at least three letters long and formed from adjoining letters.Letters may not be reused and it is valid to move across diagonals. As an example,consider the following 4 * 4 grid of letters: A B C DE F G HI J K LM N O P The word “FAB” is valid (letters in the upper left corner) and the word “KNIFE”is valid. The word “BABE” is not valid because the “B” may not be reused. Theword “MINE” is not valid because the “E” is not adjacent to the “N”. Write a program that uses a 4 * 4 two-dimensional array to represent the gameboard. The program should randomly select letters for the board. You may wishto select vowels with a higher probability than consonants. You may also wish toalways place a “U” next to a “Q” or to treat “QU” as a single letter. The programshould read the words from the text file words.txt (included on the website withthis book) and then use a recursive algorithm to…arrow_forwardIn C program I'm stuck in a hall of mirrors! I was told that there was only one way for the exit to be revealed to me, and that is if I can determine whether the multidimensional array shown in the mirror looks the same as the one I'm currently holding! You need to help me get out, I'm begging you! Input 1. Number of rows of the multidimensional array Constraints The value is >= 2. 2. Number of columns of the multidimensional array Constraints The value is >= 2. 3. Values of the multidimensional array. Output The first line will contain a message prompt to input the number of rows. The second line will contain a message prompt to input the number of columns. The succeeding lines will prompt to input the values of the multidimensional array. The last line will contain the message "mirror" - if the array is a mirror, and "not" - if the array is not a mirror. Enter the number of rows: 3 Enter the number of columns: 3 4 3 4 3 8 3 10 1 10 mirrorarrow_forward
- Masterchef Daniel recently baked a big pizza that can be represented as a grid of N rows and M columns, each cell can be either empty or contain a jalapeno, Daniel wants to cut out a sub-rectangle from the pizza which contains even number of jalapenos. Before cutting such a sub-rectangle, he is interested in knowing how many sub-rectangles are there which contains even number of jalapenos.First line of input consist of two integers P and Q. Each of the next P lines contains a string of length Q, j-th character of i-th string is 1 if the corresponding cell contains a jalapeno otherwise it's 0.Output a single integer, the number of sub rectangles which contains even number of jalapeno.Sample Input:2 22101Output:5arrow_forwardJavanetbeans Use a one-dimensional array to solve the following problem: Write an application that inputs five numbers each of which is between 10 and 100, inclusive. As each number is read, display it only if it is not a duplicate of a number already read. Provide the “worst case”, in which all five numbers are different. Use the smallest possible array to solve this problem. Display the complete set of unique values input after the user inputs each new value Initialize the integer array numbers to hold five elements. This is the maximum number of values the program must store if all values are input. 2. Validate the input and display an error message if the user inputs invalid data. 3. If the number entered is not unique, display a message to the user; otherwise, store the number in the array and display the list of unique numbers entered so far.arrow_forwardWrite a program and fill a queue with random numbers between 0 and 100. The size of thequeue is assumed to be 15. After filling the array with random numbers, display the elements in the queue and remove the elements of the queue and store these numbers according to the following criteria.If the number in the queue is less than 50, remove it from the queue and store it inside queue 2.Else, remove the number and store these values inside queue 3.Display all three queues on the screen.arrow_forward
- Computer Science Java Program Write a program in Java that takes in a user-submitted file that is a grid of 0s and 1s. Represent the grid as a two-dimensional array. Write a program that: a. computes the size of a group when a square in the group is given b. computes the numbers of different groups c. lists all groups Example: userInput.txt: 0 0 0 0 0 0 0 0 0 10 0 0 1 1 0 0 0 0 10 0 0 0 0 0 0 0 0 00 0 0 0 1 0 0 1 0 00 0 0 1 0 0 0 1 0 00 0 0 0 0 0 0 1 1 00 0 0 0 1 1 0 0 0 00 0 0 0 0 0 0 0 0 00 0 0 0 0 0 0 0 0 00 0 0 0 0 0 0 0 0 0 The program should output three things: The size of the group in which location specified by the user belongs. then if the user enters 1,2 the answer is 0 1,4 the answer is 2 3,4 the answer is 1 4,7 the answer is 4 The number of each size group Size Count 2 3 1 List of each group’s locations 1: (0,9) (1,9) 2: (1,3) (1,4) 3: (3,4) 4: (3,7) (4,7) (5,7) (5,8) 5: (4,3) 6: (6,4) (6,5)arrow_forwardUsing java language In voltTest[10] is an encapsulated array that contains 10 voltages. Display how many are ACTIVE (5V), SATURATED (greater than 5V) and CUTOFF (less than 5V). Use the UML diagram as a reference. setValue(int,int,int) – accepts the value of voltActive, voltSaturated and voltCutoff as a parameter, which is the number of active, saturated and cutoff voltages. displayResult() – displays the number of active, saturated and cutoff voltages.arrow_forwardYou have been hired at an open-air mine. You are to write a program to control a digger. For your task, you have been given a 'map' of all the underground resources in the mine. This map comes as a two-dimensional integer array. The array has n rows and k columns. Each integer is between zero and one hundred (inclusive). This array represents a grid map of the mine – with each row representing a different height level. Each square in the grid can contain valuable resources. The value of these resources can go from zero (no resources) to 100 (max resources). The grid maps the resources just below the surface, and down to the lowest diggable depths. The digger starts at the surface (so, just on top of the topmost row in the grid)—at any horizontal position between 1 and k. The digger cannot dig directly downwards, but it can dig diagonally in either direction, left-down, or right-down. In its first time-step, it will dig onto the first row of the grid. In its second time-step, it'll hit…arrow_forward
- Write a program to place ten queens on 10 x 10 chessboard in such a way that one queen is to bein each row. A program will use 2 DIMENIONAL array x[r][c] to do this configuration. If x[r] hasvalue c, then in row r there is a queen in column c. Write a program that asks a user to enter thecolumns that contain queens in the ten rows. The program then places the queens in thesecolumns (one per row) and prints the board.arrow_forwardYou have been hired at an open-air mine. You are to write a program to control a digger. For your task, you have been given a 'map' of all the underground resources in the mine. This map comes as atwo-dimensional integer array. The array has n rows and k columns. Each integer is between zero and one hundred (inclusive). This array represents a grid map of the mine – with each row representing a different height level. Each square in the grid can contain valuable resources. The value of these resources can go from zero (no resources) to 100 (max resources). The grid maps the resources just below the surface, and down to the lowest diggable depths. The digger starts at the surface (so, just on top of the topmost row in the grid)—at any horizontal position between 1 and k. The digger cannot dig directly downwards, but it can dig diagonally in either direction, left-down, or right-down. In its first time-step, it will dig onto the first row of the grid. In its second time-step, it'll hit…arrow_forwardUse java only. And aswer all tascs Write a program to compute lines equation for a set of lines. The number of lines is read from the user. Each line is computed by generating two points randomly using Point2D API. In addition, distance between two points is computed. The program should display a table which include all information about each line. See sample runs below. Take into consideration: 1. Number of generated lines should be greater than zero. 2. Two point objects are constructed only once. Then just update the location of two points for each line using Point2D API methods. 3. x and y values of each point are generated randomly to be less than 100 and greater than 0. 4. Compute the distance between two points using Point2D API methods. 5. A line has two special cases: horizontal line (m = 0), vertical line( m is undefined).arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
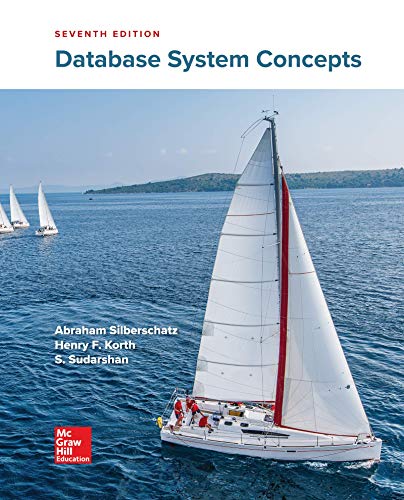
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
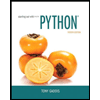
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
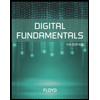
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
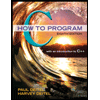
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
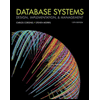
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
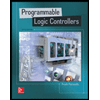
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education