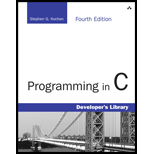
Programming in C
4th Edition
ISBN: 9780321776419
Author: Stephen G. Kochan
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Question
Chapter 7, Problem 14E
7.10
Program Plan Intro
Modified version of the exercise 7.10 with the global variables:
Program Plan:
- Include required header files.
- Give the function prototype.
- Declare the variable “n” and “val” as global variable
- Define the main function,
- Get the number from the user.
- Call the function “prime()”.
- Check whether “val” equals to “1”.
- Print the corresponding statement.
- Otherwise, if the condition fails,
- Print the corresponding statement.
- Definition for the function “prime()”,
- Declare the variable “i”.
- Use for loop to check the condition.
- Check whether the remainder equals to 0.
- Return the value “0”.
- Return the value “1”.
- Check whether the remainder equals to 0.
- Use for loop to check the condition.
- Declare the variable “i”.
a)
Program Plan Intro
Modified version of the exercise 7.12(a) with the global variables:
Program Plan:
- Include required header files
- Declare and initialize the array values globally
- Declare the array “Arr_2” globally.
- Definition for the function “isTrans(int A[4][5], int B[5][4])”
- Declare the variable “k” and “l”.
- For loop to check whether “k” is less than “4”
- For loop to check whether “j” is less than “5”
- Condition to check if both the elements in the array are not equal.
- Return the value “0”.
- Return the value “1”.
- Condition to check if both the elements in the array are not equal.
- Definition for the function “transposeMatrix(int A[4][5], int B[5][4])”
- Declare the variable “k” and “l”.
- For loop to check whether “k” is less than “4”
- For loop to check whether “j” is less than “5”
- Condition to check if both the elements in the array are equal.
- Return the value “0”.
- Condition to check if both the elements in the array are equal.
- Check whether “isTrans(A,B)” equals to 1.
- For loop to check whether “k” is less than “5”
- For loop to check whether “l” is less than “4”
- Print the element.
- Print new line.
- For loop to check whether “l” is less than “4”
- Otherwise, print the corresponding statement inside “else” clause.
- For loop to check whether “k” is less than “5”
- Define the main function
- Call the function “transpose()” by passing the array.
- Return the value “0”.
b)
Program Plan Intro
Modified version of the exercise 7.12(b) with the global variables:
Program Plan:
- Include required header files
- Definition for the function “isTrans(int A[row][col], int B[row][col])”
- Declare the variable “k” and “l”.
- For loop to check whether “k” is less than “row”
- For loop to check whether “j” is less than “col”
- Condition to check if both the elements in the array are not equal.
- Return the value “0”.
- Return the value “1”.
- Condition to check if both the elements in the array are not equal.
- Definition for the function “t(int A[row][col], int B[row][col])”
- Declare the variable “k” and “l”.
- For loop to check whether “k” is less than “row”
- For loop to check whether “j” is less than “col”
- Condition to check if both the elements in the array are equal.
- Return the value “0”.
- Condition to check if both the elements in the array are equal.
- Check whether “isTrans(A,B)” equals to 1.
- For loop to check whether “k” is less than “col”
- For loop to check whether “l” is less than “row”
- Print the element.
- Print new line.
- For loop to check whether “l” is less than “row”
- Otherwise, print the corresponding statement inside “else” clause.
- For loop to check whether “k” is less than “col”
- Define the main function
- Declare and initialize the array values.
- Declare the array “Arr_2”.
- Call the function “transpose()” by passing the array.
- Return the value “0”.
7.13
Program Plan Intro
Modified version of the exercise 7.13 with the global variables:
Program Plan:
- Include required header files.
- Declare and initialize the array as global.
- Declare the variable “n” as global variable.
- Definition for the function “sort()”.
- Declare the variables.
- Condition to check the “i<n-1”.
- Condition to check the “j<n”.
- Check whether to sort in increasing order.
- Assign “a[i] ” to “temp”.
- Assign “a[j] ” to “a[i]”.
- Assign “a[j]” to “temp”.
- Check whether to sort in decreasing order.
- Assign “a[i]” to “temp”.
- Assign “a[j] ” to “a[i]”.
- Assign “a[j]” to “temp”
- Check whether to sort in increasing order.
- Condition to check the “j<n”.
- Define the main function
- Print the statement.
- Loop to traverse the array.
- Print the element.
- Call the function “sort()”.
- Loop to traverse the array.
- Print the element.
- Print new line
- Return the value “0”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
What is pass a 2D array to a functions?
Modify the selection sort function developed in this chapter so that it accepts a second optional argument, which may be either 'up' or 'down'. If the argu-ment is 'up', sort the data in ascending order. If the argument is 'down', sort the data in descending order. If the argument is missing, the default case is to sort the data in ascending order. (Be sure to handle the case of invalid arguments, and be sure to include the proper help information in your function.)
Here is the given function which need to be mofified:
function out = ssort(a)
nvals = length(a);
iptr = ii;
for jj = ii+1:nvals
if a(jj) < a(iptr)
iptr = jj;
end
end
if ii ~= iptr
temp = a(ii);
a(ii) = a(iptr);
a(iptr) = temp;
end
end
out = a;
# Write a function to copy one array to another by using pointers. Comment/Discussion on the obtained results and discrepancies (if any).
Chapter 7 Solutions
Programming in C
Ch. 7 - Type in and run the 17 programs presented in this...Ch. 7 - Prob. 2ECh. 7 - Modify Program 7.8 so that the value of e is...Ch. 7 - Modify Program 7.8 so that the value of g is...Ch. 7 - Prob. 6ECh. 7 - Write a function that raises an integer to a...Ch. 7 - Prob. 8ECh. 7 - The least common multiple (1cm) of two positive...Ch. 7 - Prob. 10ECh. 7 - Write a function called a that takes two...
Knowledge Booster
Similar questions
- Create the function that takes integer target and the array as the parameters and returns the length of minimum subarray. Check the function on example 1 in the main function. In C++, O(n) complexity.arrow_forwardWhen arrays are supplied to functions as parameters: A They are never passed by reference.B. They are usually passed by reference.C. Arrays cannot be sent as arguments to functions.D. There is no need to supply arrays to functions because they are global by default.arrow_forward(Modify) Modify the program written for Exercise 9 by adding an eighth column to the array. The grade in the eighth column should be calculated by computing the average of the top three grades only.arrow_forward
- 2/ Repeat question 2 by passing 2-D array to a function called column_sum.arrow_forwardWhich mechanism do we employ to return multiple values from a function? Give an example to back up your answer.arrow_forwardWrite the programming language function that calls the below function appropriately, while adjusting the variables top, bottom, left and right accordingly; these 4 variables and the 2-D array should be global variables. The end result should be the array (matrix) filled in a spiral manner.arrow_forward
- 2.) Revise the function quickSort so that it always chooses the last item in the array as the pivot. Add a counter to the function partition that counts the number of comparisons that are made. Compare the behavior of the revised function with the original one (pivot should be selected using sortFirstMiddleLast algorithm), using arrays of various sizes. At what size array does the difference in the number of comparisons become significant? For which pivot selection strategy does the difference in the number of comparisons become significant? Compare your analysis with the actual running times and counter as a function of the input size n = 2, 4, 8, 16, 32, 64, 128, 256, 512, 1024, 2048, 8192, 16384 <time.h> and clock() function. For comparison, you need to create two tables for execution times and a counter for both algorithmsarrow_forwardAn array with an odd number of elements is said to be balanced if all elements (except the middle one) are strictly greater than the value of the middle element. Note that only arrays with an odd number of elements have a middle element. Write a C++ function that accepts an integer array and returns 1 or “Balanced” if it is a balanced array, otherwise it returns 0 or “Not Balanced”.arrow_forwardComplete the swift function given below and test it for the array [14, 67, 4, 16]arrow_forward
- Write a function set_zero() that takes a numpy array and a limit number as parameters. Set the items greater than the limit number to 0. Return the new numpy array.arrow_forwardPlease provide the solution/code for the following screenshot. The language is used is Racket and please make sure to provide the definitions of all the functions and the function itself. Please use recursion and set operation.arrow_forwardWrite a function named find that takes a pointer to the beginning and a pointer to the end (1 element past the last) of an array, as well as a value. The function should search for the given value and return a pointer to the first element with that value, or the end pointer if no element was found.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
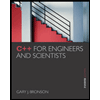
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
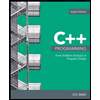
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning