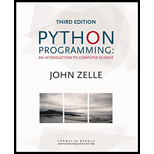
Program to displays the score with each click and the total score in series
Program plan:
- Import the required packages
- In the “main()” function,
- Create the object of “GraphWin()”.
- Set the coordinates by calling the function “setCoords()”
- Create an object named “c” and store the points
- The outline of the circle is set to “green4” color.
- The circle is filled with “white” color.
- The width of the circle is set with the use of function “setWidth()”.
- Draw the circle with the use of function “draw()”.
- Create an object named “c2” and store the points
- The outline of the circle is set to “green4” color.
- The circle is filled with “red” color.
- The width of the circle is set with the use of function “setWidth()”.
- Draw the circle with the use of function “draw()”.
- Create an object named “c3” and store the points
- The outline of the circle is set to “green4” color.
- The circle is filled with “blue” color.
- The width of the circle is set with the use of function “setWidth()”.
- Draw the circle with the use of function “draw()”.
- Create an object named “c4” and store the points
- The outline of the circle is set to “green4” color.
- The circle is filled with “black” color.
- The width of the circle is set with the use of function “setWidth()”.
- Draw the circle with the use of function “draw()”.
- Create an object named “c5” and store the points
- The outline of the circle is set to “green4” color.
- The circle is filled with “white” color.
- The width of the circle is set with the use of function “setWidth()”.
- Draw the circle with the use of function “draw()”.
- Initialize a for loop to get the value of the points.
- Get the points where the mouse is clicked and store it in variable “arrow”.
- Derive the x-coordinate with the use of “getX()” function.
- Derive the x-coordinate with the use of “getY()” function.
- Calculate the value derived out of the equation and store in “z”.
- If the z-value is less than or equal to 5 and greater than 4 then,
- “y” is assigned with “1”
- “sum” is added with the value of “y”.
- If the z-value is less than or equal to 4 and greater than 3 then,
- “y” is assigned with “3”
- “sum” is added with the value of “y”.
- If the z-value is less than or equal to 3 and greater than 2 then,
- “y” is assigned with “5”
- “sum” is added with the value of “y”.
- If the z-value is less than or equal to 2 and greater than 1 then,
- “y” is assigned with “7”
- “sum” is added with the value of “y”.
- If the z-value is less than 1 then,
- “y” is assigned with “9”
- “sum” is added with the value of “y”.
- otherwise,
- “y” is assigned with “0”
- print the output statement.
- Print the value stored in “y” and “sum”.
- Call the function “main()”.

This program displays score achieved with each click in an archery board and also calculates and displays the sum of the entire series of outputs.
Explanation of Solution
Program:
#import the required packages
from graphics import *
import math as m
#define the main() function
def main():
#declare the required variables
win = GraphWin()
#set the coordinates
win.setCoords(-5, -5, 5, 5)
#draw the circle with specified points
c = Circle(Point(0,0), 5)
#set the outline of the circle
c.setOutline("green4")
#fill the circle with the colour
c.setFill("white")
#set the width of the circle
c.setWidth(1)
#draw the circle
c.draw(win)
#draw the circle with specified points
c2 = Circle(Point(0,0), 4)
#set the outline of the circle
c2.setOutline("green4")
#fill the circle with the colour
c2.setFill("red")
#set the width of the circle
c2.setWidth(1)
#draw the circle
c2.draw(win)
#draw the circle with specified points
c3 = Circle(Point(0,0), 3)
#set the outline of the circle
c3.setOutline("green4")
#fill the circle with the colour
c3.setFill("blue")
#set the width of the circle
c3.setWidth(1)
#draw the circle
c3.draw(win)
#draw the circle with specified points
c4 = Circle(Point(0,0), 2)
#set the outline of the circle
c4.setOutline("green4")
#fill the circle with the colour
c4.setFill("black")
#set the width of the circle
c4.setWidth(1)
#draw the circle
c4.draw(win)
#draw the circle with specified points
c5 = Circle(Point(0,0), 1)
#set the outline of the circle
c5.setOutline("green4")
#fill the circle with the colour
c5.setFill("white")
#set the width of the circle
c5.setWidth(1)
#draw the circle
c5.draw(win)
#declare and initialize the variable
sum = 0
#initialize the loop for x less than 5
for x in range (5):
#get the locations where mouse is clicked
arrow = win.getMouse()
#stores the X coordinate
x = arrow.getX()
#stores the Y coordinate
y = arrow.getY()
#calculate and store the value
z = m.sqrt(x ** 2 + y ** 2)
#condition for z to be less than or equal to 5 and greater than 4
if 5 >= z > 4:
#declare the variable
y = 1
#calculate the value of sum
sum = y + sum
#condition for z to be less than or equal to 4 and greater than 3
elif 4 >= z > 3:
#declare the variable
y = 3
#calculate the value of sum
sum = y + sum
#condition for z to be less than or equal to 3 and greater than 2
elif 3 >= z > 2:
#declare the variable
y = 5
#calculate the value of sum
sum = y + sum
#condition for z to be less than or equal to 2 and greater than 1
elif 2 >= z > 1:
#declare the variable
y = 7
#calculate the value of sum
sum = y + sum
#condition for z to be less than 1
elif 1 > z:
#declare the variable
y = 9
#calculate the value of sum
sum = y + sum
#else statement
else:
#declare the variable
y = 0
#print the statement
print("You missed!")
#print the statement
print("Point: {0} Total: {1}".format(y, sum))
#call the main() function
main()
Output:
Screenshot of output
Want to see more full solutions like this?
Chapter 7 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- Programming Exercise 10 in Chapter 6 asks you find the mean and standard deviation of five numbers. Extend this programming exercise to find the mean and standard deviation of up to 100 numbers. Suppose that the mean (average) of n numbers x1, x2, . . ., xn is x. Then the standard deviation of these numbers is:S=sqrt(((x1-x)^2+(x2-x)^2+...+(xi-x)^2+...+(xn-x)^2)/n)arrow_forwardA robot is initially located at position (0, 0) in a grid [−5, 5] × [−5, 5]. The robot can move randomly in any of the directions: up, down, left, right. The robot can only move one step at a time. For each move, print the direction of the move and the current position of the robot. If the robot makes a circle, which means it moves back to the original place, print “Back to the origin!” to the console and stop the program. If it reaches the boundary of the grid, print “Hit the boundary!” to the console and stop the program. A successful run of your code may look like: Down (0,-1) Down (0,-2) Up (0,-1) Left (-1,-1) Left (-2,-1) Up (-2,0) Left (-3,0) Left (-4,0) Left (-5,0) Hit the boundary! or Left (-1,0) Down (-1,-1) Right (0,-1) Up (0,0) Back to the origin! Instructions: This program is to give you practice using the control flow, the random number generator, and output formatting. You may not use stdafx.h. Include header comments. Include <iomanip> to format your output. Name…arrow_forwardcompare loans with various interest rates) Write a programthat lets the user enter the loan amount and loan period in number of years anddisplays the monthly and total payments for each interest rate starting from 5% to10%, with an increment of 1/4. Here is a sample run: Loan Amount: 10000Number of Years: 5Interest Rate Monthly Payment Total Payment5.000% 188.71 11322.745.250% 189.86 11391.595.500% 191.01 11460.70...9.750% 211.24 12674.5510.000% 212.47 12748.23arrow_forward
- One interesting application of computers is drawing graphs and bar charts.Write a program that prompts the user to enter 5 integers, and then outputs abar chart consisting of asterisks. The number of asterisks in each line of thebar chart must be equal to the number the user entered. You may assume theuser will enter only positive integers or 0. C++ please.arrow_forwardIn the Raptor program Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows:1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don’t display the computer’s choice yet.)2. The user enters his or her choice of “rock,” “paper,” or “scissors” at the keyboard.3. The computer’s choice is displayed.4. A winner is selected according to the following rules:• If one player chooses rock and the other player chooses scissors, then rock wins. (Rock smashes scissors.)• If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.)• If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps rock.)• If both players make the same choice, the…arrow_forwardIn the Raptor program Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows:1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don’t display the computer’s choice yet.)2. The user enters his or her choice of “rock,” “paper,” or “scissors” at the keyboard.3. The computer’s choice is displayed.4. A winner is selected according to the following rules:• If one player chooses rock and the other player chooses scissors, then rock wins. (Rock smashes scissors.)• If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.)• If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps rock.)• If both players make the same choice, the…arrow_forward
- In the Raptor program Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows:1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don’t display the computer’s choice yet.)2. The user enters his or her choice of “rock,” “paper,” or “scissors” at the keyboard.3. The computer’s choice is displayed.4. A winner is selected according to the following rules:• If one player chooses rock and the other player chooses scissors, then rock wins. (Rock smashes scissors.)• If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.)• If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps rock.)• If both players make the same choice, the…arrow_forwardA word is called as a good word if all the letters of the word are distinct. That is, all the letters of the word are different from each other letter. Else, the word is called as a bad word. This can be continued based on the user input is 'Y'.arrow_forwardQuestion # 8: Write a program that inputs a number and checks whether it is a perfect number or not. A perfect number is the number that is numerically equal to the sum of its divisors. For example, 6 is a perfect number because the divisors of 6 are 1, 2, 3 and 1+2+3=6arrow_forward
- French Articles: French country names are feminine when they end with the letter e, masculine otherwise, except for the following which are masculine even though they end with e: le Belize le Cambodge le Mexique le Mozambique le Zaïre le Zimbabwe Write a program that reads the French name of a country and adds the article: le for masculine or la for feminine, such as le Canada or la Belgique. However, if the country name starts with a vowel, use l’; for example, l’Afghanistan. For the following plural country names, use les: les Etats-Unis les Pays-Basarrow_forwardIN JAVA Alice and Bob are playing a board game with a deck of nine cards. For each digit between 1 to 9, there is one card with that digit on it. Alice and Bob each draw two cards after shuffling the cards, and see the digits on their own cards without revealing the digits to each other. Then Alice gives her two cards to Bob. Bob sees the digits on Alice’s cards and lays all the four cards on the table in increasing order by the digits. Cards are laid facing down. Bob tells Alice the positions of her two cards. The goal of Alice is to guess the digits on Bob’s two cards. Can Alice uniquely determine these two digits and guess them correctly? Input The input has two integers p,q (1≤p<q≤9) on the first line, giving the digits on Alice’s cards. The next line has a string containing two ‘A’s and two ‘B’s, giving the positions of Alice’s and Bob’s cards on the table. It is guaranteed that Bob correctly sorts the cards and gives the correct positions of Alice’s cards. Output If Alice can…arrow_forwardA Python Programming in Physics and Materials Science. In physics, chemistry and materials science, percolation refers to the movement and filtering of fluids through porous materials.(Wikipedia.org). Consider a simplified model below. Imagine, this is a filter or maybe some layers of stones such that when a certain amount of fluid is put on top, it will reach the bottom only if there is a direct passage. In this case, the blue squares serve as the porous materials or the holes where fluid may pass. Your task is to simulate this simple percolation by representing them in a 2-dimensional array. The input would be in a string, while the output would either be yes or no to indicate whether the fluid can pass through the filter or not. Example: Input: “1,0,0,0,0;0,1,0,0,0;0,1,0,0,0;0,0,1,0,0;0,0,0,1,0” Output: Yes Note: It should also work with these two inputs: “1” -> Yes “0” -> Noarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
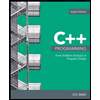
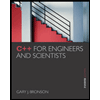
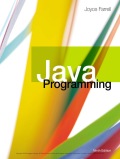