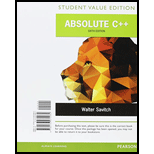
Concept explainers
Define a class called Month that is an abstract data type for a month. Your class will have one member variable of type int to represent a month (1 for January, 2 for February, and so forth). Include all the following member functions: a constructor to set the month using the first three letters in the name of the month as three arguments, a constructor to set the month using an integer as an argument (1 for January, 2 for February, and so forth), a default constructor, an input function that reads the month as an integer, an input function that reads the month as the first three letters in the name of the month, an output function that outputs the month as an integer, an output function that outputs the month as the first three letters in the name of the month, and a member function that returns the next month as a value of type Month. Embed your class definition in a test

Program plan:
1 . The following variables are used in the program:
- monthvariable of integer data type is used to store the month.
2. The following methods are used in the program:
- Default constructor.
- Constructor having one integer argument.
- Constructor having three characters arguments.
- input() function to take the month number from the user.
- inputMonthLetter() function to take the month's first three letters from the user.
- getMonth() function to return the month number.
- outputMonthLetter() function to display the month first three-letter.
- nextMonth() function to return the next month.
Program description:
The main purpose of the program is to create the constructor to initialize the class member variable using the integer argument and the first three letters of the month. Also, display the next month. Test all the class member functions in a test program.
Explanation of Solution
Program:
//including essential header file #include <iostream> //using standard namespace using namespace std; //Month class class Month { public: int month; //default constructor Month() { //initialize the month to 1 month = 1; } //constructor to set the month by number Month(int monthNumber) { //set the month to the monthNumber month = monthNumber; } //constructor to set the month number using the Month letters Month (char monthLetter1, char monthLetter2, char monthLetter3) { //when the monthLetter is jan if ((monthLetter1 == 'j') && (monthLetter2 == 'a') && (monthLetter3 == 'n')) { //set the month to 1 month = 1; } //when the monthLetter is feb if ((monthLetter1 == 'f') && (monthLetter2 == 'e') && (monthLetter3 == 'b')) { //set the month to 2 month = 2; } //when the monthLetter is mar if ((monthLetter1 == 'm') && (monthLetter2 == 'a') && (monthLetter3 == 'r')) { //set the month to 3 month = 3; } //when the monthLetter is apr if ((monthLetter1 == 'a') && (monthLetter2 == 'p') && (monthLetter3 == 'r')) { //set the month to 4 month = 4; Month(month); getMonth(); } //when the monthLetter is may if ((monthLetter1 == 'm') && (monthLetter2 == 'a') && (monthLetter3 == 'y')) { //set the month to 5 month = 5; } //when the monthLetter is jun if ((monthLetter1 == 'j') && (monthLetter2 == 'u') && (monthLetter3 == 'n')) { //set the month to 6 month = 6; } //when the monthLetter is jul if ((monthLetter1 == 'j') && (monthLetter2 == 'u') && (monthLetter3 == 'l')) { //set the month to 7 month = 7; } //when the monthLetter is aug if ((monthLetter1 == 'a') && (monthLetter2 == 'u') && (monthLetter3 == 'g')) { //set the month to 8 month = 8; } //when the monthLetter is sep if ((monthLetter1 == 's') && (monthLetter2 == 'e') && (monthLetter3 == 'p')) { //set the month to 9 month = 9; } //when the monthLetter is oct if ((monthLetter1 == 'o') && (monthLetter2 == 'c') && (monthLetter3 == 't')) { //set the month to 10 month = 10; } //when the monthLetter is nov if ((monthLetter1 == 'n') && (monthLetter2 == 'o') && (monthLetter3 == 'v')) { //set the month to 11 month = 11; } //when the monthLetter is dec if ((monthLetter1 == 'd') && (monthLetter2 == 'e') && (monthLetter3 == 'c')) { //set the month to 12 month = 12; } } void input() { cout<<endl<< "Enter the month number: "; cin>> month; while (month < 1 || month > 12) { cout<< "Invalid month number. Month number must be between 1 to 12." <<endl; cout<< "Enter the month number again: "; cin>> month; } } void inputMonthLetter() { //char letter1, letter2, letter3; char letter[4]; cout<<endl<< "Enter the first three letters of the month: "; cin>> letter; Month (letter[0], letter[1], letter[2]); } int getMonth() { return month; } void outputMonthLetter() { if (month == 1) { cout<< "The month is Jan"; } if (month == 2) { cout<< "The month is Feb"; } if (month == 3) { cout<< "The month is Mar"; } if (month == 4) { cout<< "The month is April"; } if (month == 5) { cout<< "The month is May"; } if (month == 6) { cout<< "The month is June"; } if (month == 7) { cout<< "The month is July"; } if (month == 8) { cout<< "The month is August"; } if (month == 9) { cout<< "The month is Sep"; } if (month == 10) { cout<< "The month is Oct"; } if (month == 11) { cout<< "The month is Nov"; } if (month == 12) { cout<< "The month is Dec"; } } //member function to return the next month as Month type Month nextMonth() { int m = ((month % 12 ) + 1 ); return Month(m); } }; int main() { //create objects of the class Month m1, m3; m1.input(); m1.outputMonthLetter(); m3=m1.nextMonth(); cout<<endl<< "The next month is: "<<m3.getMonth(); Month m2, m4; m2.inputMonthLetter(); cout<<endl<< "month is: " << m2.getMonth(); m4 = m2.nextMonth(); cout<<endl<< "The next month is: " << m4.getMonth(); return 0; }
Explanation:
In the above program, a default constructor is created which initializes the variable month. Another constructor that takes an integer parameter also initializes the variable month. A third constructor takes three-character arguments. This constructor matches these arguments with the first three letters of each month and then initializes the variable month accordingly.
Inside the main() method, objects of the class Month are created. The input() method is used to take user input for the month in the form of an integer. The outputMonthLetter() is used to output the first three letters of the month corresponding to the user input. The nextMonth() function returns the month in the form of the object of the class Month. The inputMonthLetter() method is used to take user input for the first three letters of the name of the month. This method calls the parameterized constructor that takes three-character arguments.The getMonth() method returns the variable month. The nextMonth() function returns the month in the form of the object of the class Month.
Sample output:
Want to see more full solutions like this?
Chapter 7 Solutions
Absolute C++,NO CODE INCLUDED (6th Edition)
Additional Engineering Textbook Solutions
Computer Science: An Overview (12th Edition)
C Programming Language
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Absolute Java (6th Edition)
Starting Out with Python (4th Edition)
Modern Database Management (12th Edition)
- Write a program to model a student class with roll number and name as the sensitive information. Initialize default values for the sensitive information using a default constructor, use a copy constructor to copy from another object, use a member function to take user input and update the name and roll number and display the same using another member function. b.Add constructor overloading to the above code and enable initialization of name, roll number via constructor arguments. NAME ROHI ROLLNO 18arrow_forwardConsider a class named Calculator with typical four specific functionalities i.e. addition, subtraction, multiplication, and division. Implement these functionalities as four functions with two parameters. It is also required to overload all these functions for int and double data types. In the main function, create an object of class Calculator and invoke its member functions while passing parameters of int and double type.arrow_forwardDefine a class for complex numbers. A complex number is a number of the form a + b*i where for our purposes, a and b are numbers of type double, and i is a number that represents the quantity √-1. Represent a complex number as two values of type double. Name the member variables real and imaginary. (The variable for the number that is multiplied by i is the one called imaginary.) Call the class Complex. Include a constructor with two parameters of type double that can be used to set the member variables of an object to any values. Include a constructor that has only a single parameter of type double; call this parameter realPart and define the constructor so that the object will be initialized to realPart + 0*i.Include a default constructor that initializes an object to 0 (that is, to 0 + 0*i).Overload all the following operators so that they correctly apply to the type Complex: ==, +, −, *, >>, and <<. You should also write a test program to test your class. Hints: To add…arrow_forward
- Create a base class named Point consisting of x and y data members representing point coordinates. From this class, derive a class named Circle with another data member named radius. For this derived class, the x and y data members represent a circle’s center coordinates. The member functions of the Point class should consist of a constructor, an area() function that returns 0, and a distance() function that returns the distance between two points,(x1,y1) and (x2,y2), where Additionally, the derived class should have a constructor and an override function named area() (PI*pow(radius,2)) that returns a circle’s area. Task: 1-Include the classes constructed in a working C++ program. 2-Have your program call all the member functions in each class. 3- In addition, call the base class’s distance() function with two circle objects and explain the results this function returns.arrow_forwardwrite a class Point with parametrized constructor. This class have four member variables, a,b,c,d. Write the following member functions a. drawTriangle(int x, int y, int z ) b. drawRectangle(int x, int y, int z, int a). Each function should display the length of lines for each of the shape (triangle, rectangle). For example triangle should calculate length of its three lines by following methods as shown in code. Note: the number of lines depends on the name of shape. void drawTriangle(int x, int y, int z ) { int line 1 = x - y; // convert to positive value if line length is negative int line 2 = y -z; int line 3 = z -x; cout<< The length of each lines is: << //// here display length of each line with proper formatting. } Write similar code for drawRectangle(int x, int y, int z, int a). Wtite main function to call these three functionarrow_forwardConsider the following declarations: class xClass{public:void func();void print() const ; xClass ();xClass (int, double); private:int u;double w;};Sample Output: Program 2 and assume that the following statement is in a user program: xClass x;a. How many constructors does class xClass have?b. Write the definition of the default constructor of the class xClass so that the private member variables are initialized to 0.c. Write the definition of the constructor of the class xClass so that the private member variables u and w are initialized to the values passed in the functions.arrow_forward
- Using classes and arrays, the team will develop a set of functions for an online shopping system. The system is represented by the following structure:1- Class Item having the following private attributes: (ID, name, quantity, price) and the following public methods:- Constructors (default, parameterized, and copy)- Setters & Getters- Operator overloading for the ==, +=,-=, >> and << operators Note that the ID member variable is not entered or read from the user. It is automatically set by the class as a serial ID starting with the first item of ID 1 and incrementing with every new object.2- Class Seller having the following private attributes: (name, email, items,maxItems), where items is a dynamic array of objects of type Item with the size maxItems. The class has the following public methods:- Constructor (parameterized)- Operator overloading for the >> and << operators- Add An Item.- This will take an Item object as a parameter:- If the item already…arrow_forwardUsing classes and arrays, the team will develop a set of functions for an online shopping system. The system is represented by the following structure: 1- Class Item having the following private attributes: (ID, name, quantity, price) and the following public methods:- Constructors (default, parameterized, and copy)- Setters & Getters- Operator overloading for the ==, +=,-=, >> and << operatorsNote that the ID member variable is not entered or read from the user. It is automatically set by the class as a serial ID starting with the first item of ID 1 and incrementing with every new object.2- Class Seller having the following private attributes: (name, email, items,maxItems), where items is a dynamic array of objects of type Item with the size maxItems. The class has the following public methods:- Constructor (parameterized)- Operator overloading for the insertion << operators- Add An Item.- This will take an Item object as a parameter:- If the item already exists…arrow_forwardWrite a class definition that creates a class called leverage with one private data member, crowbar, of type int and one public function whose declaration is void pry().arrow_forward
- Assume the definition of class foodType as given in Exercise 6. Answer the following questions? (1, 2, 3, 5, 6) Write the definition of the member function set so that private members are set according to the parameters. The values of the int and double instance variables must be nonnegative. Write the definition of the member function print that prints the values of the data members. Write the definitions of the member functions getName, getcalories, getFat, getSugar, getCarbohydrate, getPotassium to return the values of the instance variable. Write the definition of the default constructor of the class foodType so that the private member variables are initialized to 0, 0. 0, 0, 0. 0, 0. 0, respectively. Write the definition of the constructor with parameters of the class foodType so that the private member variables are initialized according to the parameters. The values of the int and double instance variables must be nonnegative. Write a C + + statement that prints the value of the object fruit 2. Write a C++ statement that declares an object my Fruit of type foodType, and initializes the member variables of myFruit to Apple, 52, 0. 2, 10,13.8, and 148.0, respectively.arrow_forwardIn Chapter 10, the class clockType was designed to implement the time of day in a program. Certain applications, in addition to hours, minutes, and seconds, might require you to store the time zone. Derive the class extclockType from the class clockType by adding a member variable to store the time zone. Add the necessary member functions and constructors to make the class functional. Also, write the definitions of the member functions and the constructors. Finally, write a test program to test your class.arrow_forwardC++ QUESTION 2. Give a definition for a class InternationalStudent that is a derived class of the base class Student given in the following. Do not bother with #include directives or namespace details.class Student{public:Student( );void printInfo( ) const;protected:int id;string name;};This class InternationalStudent should have an additional data field nation of type string ; one additional member function isFunded that takes no arguments and returns a value of type bool ; and suitable constructors.You do not need to give any implementations, just the class definition.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
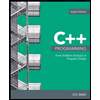
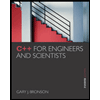