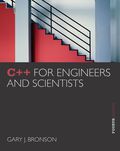
(Statistics) a. Write a C++
b. Extend the program written for Exercise 1a to display each grade and its letter equivalent, using the following scale:
(a)

Program Plan:
- Declaremax and count variables of int data type.
- Declare sumand average variables of double data type
- Declare an array grade[100] of double data type.
- Use for loop to read all the array elements from the user.
- Use if statementto check the negative value.
- Calculatethe sum and average of all the user entered score.
- Display the calculated results to the user.
- Use for loop to calculate grades below the average.
- Use if condition to find the grades below the average.
- Display the grades below average by using the asterisk in the front of the grade(*).
- int main() method function is used to perform all the tasks.
Program Description: The main purpose of the program is to read all scores from the user, storing the scores in the grade[] array, calculating the sum and average of all the entered elements and to display the grades below average by using the asterisk in the front of grade(*).
Explanation of Solution
Program code:
//including required header files #include<iostream> usingnamespacestd; //main method int main() { //declaring required variables int max = 100, count =0; double sum=0, average=0; //Declaring the array to store value of grades double grade[max]; cout<<"Enter scores and any negative number to terminate"<<endl; //This loop will execute maximum of 100 //while loop while(count<max){ //Reading inputs from user cin>>grade[count]; //if the number is negative then loop is terminated if(grade[count]<0) break; //adding the entered sum sum = sum + grade[count]; //increnting loop variable count++; }//end of while loop //Calculating average average = sum/count; //displaying Calculated results to the user cout<<"Total of the scores: "<<sum<<endl; cout<<"Average of the scores: "<<average<<endl; cout<<"Scores below average: "; //for loop to find the score below average for(int i=0; i<count; i++) { //if the score is below average if(grade[i]<average) { cout<<"\n * "<<grade[i]; } } return0; }//end of the main method
Sample output:
(b)

Program Plan:
- Declaremax and count variables of int data type.
- Declare sumand average variables of double data type
- Declare an array grade[100] of double data type.
- Use for loop to read all the array elements from the user.
- Use if statement to check the negative value.
- Calculate the sum and average of all the user entered score.
- Display the calculated results to the user.
- Use for loop to calculate grades below the average.
- Use if condition to find the grades below the average.
- Display the grades below average by using the asterisk in the front of grade(*).
- Use if condition to find the grade.
- int main() method function is used to perform all the task.
Program Description: The main purpose of the program is to modify the program code given in part (a) so that the code will also display the grade letters to the user.
Explanation of Solution
Program code:
//including required header files #include<iostream> usingnamespacestd; //main method int main() { //declaring required variables int max = 100, count =0; double sum=0, average=0; //Declaring the array to store value of grades double grade[max]; cout<<"Enter scores and any negative number to terminate"<<endl; //This loop will execute maximum of 100 //while loop while(count<max){ //Reading inputs from user cin>>grade[count]; //if the number is negative then loop is terminated if(grade[count]<0) break; //adding the entered sum sum = sum + grade[count]; //increnting loop variable count++; }//end of while loop //Calculating average average = sum/count; //displaying Calculated results to the user cout<<"Total of the scores: "<<sum<<endl; cout<<"Average of the scores: "<<average<<endl; cout<<"Scores below average: "; //for loop to find the score below average for(int i=0; i<count; i++) { //if the score is below average if(grade[i]<average) { cout<<"\n * "<<grade[i]; } } //displaying message to the user cout<<"\n Grades and equivalent letters are: "; //for loop for(int i=0; i<count; i++) { //if grades are between 90 and 100 if(grade[i]<=100&& grade[i]>=90){ cout<<" \n"<<grade[i]<<": A"<<endl; } /*if grades are greater than or equal to 80 and less than 90*/ elseif(grade[i]<90&& grade[i]>=80){ cout<<" \n"<<grade[i]<<": B"<<endl; } /*if grades are greater than or equal to 70 and less than 80*/ elseif(grade[i]<80&& grade[i]>=70){ cout<<" \n"<<grade[i]<<": C"<<endl; } /*if grades are greater than or equal to 60 and less than 70*/ elseif(grade[i]<70&& grade[i]>=60) { cout<<" \n"<<grade[i]<<": D"<<endl; } //otherwise else{ cout<<" \n"<<grade[i]<<": F"<<endl; } } return0; }//end of the main method
Sample output:
Want to see more full solutions like this?
Chapter 7 Solutions
EBK C++ FOR ENGINEERS AND SCIENTISTS
- How should I answer the following regarding the first main function?arrow_forwardImplement the following function: *Code should be in pythonarrow_forward(General math) The volume of oil stored in an underground 200-foot deep cylindrical tank is determined by measuring the distance from the top of the tank to the surface of the oil. Knowing this distance and the radius of the tank, the volume of oil in the tank can be determined by using this formula: volume=radius2(200distance) Using this information, write, compile, and run a C++ program that accepts the radius and distance measurements, calculates the volume of oil in the tank, and displays the two input values and the calculated volume. Verify the results of your program by doing a hand calculation using the following test data: radius=10feetanddistance=12feet.arrow_forward
- (General math) a. Write a C++ program to calculate and display the value of the slope of the line connecting two points with the coordinates (3,7) and (8,12). Use the fact that the slope between two points with the coordinates (x1,y1)and(x2,y2)is(y2y1)/(x2x1). b. How do you know the result your program produced is correct? c. After verifying the output your program produces, modify it to determine the slope of the line connecting the points (2,10) and (12,6). d. What do you think will happen if you use the points (2,3) and (2,4), which results in a division by zero? How do you think this situation can be handled? e. If your program doesn’t already do so, change its output to this: The value of the slope is xxx.xx The xxx.xx denotes placing the calculated value in a field wide enough for three places to the left of the decimal point and two places to the right of it.arrow_forward(Physics) a. The weight of an object on Earth is a measurement of the downward force onth e object caused by Earth’s gravity. The formula for this force is determined by using Newton’s Second Law: F=MAeFistheobjectsweight.Mistheobjectsmass.AeistheaccelerationcausedbyEarthsgravity( 32.2ft/se c 2 =9.82m/ s 2 ). Given this information, design, write, compile, and run a C++ program to calculate the weight in lbf of a person having a mass of 4 lbm. Verify the result produced by your program with a hand calculation. b. After verifying that your program is working correctly, use it to determine the weight, on Earth, of a person having a mass of 3.2 lbm.arrow_forwardState whether each of the following is true or false. If false, explain why g) Definitions can appear anywhere in the body of a function.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
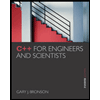