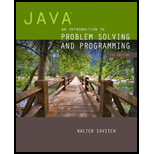
Write a

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Java: Introduction to Problem Solving and Programming
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Starting Out with Python (4th Edition)
Software Engineering (10th Edition)
Starting Out with C++: Early Objects (9th Edition)
Starting Out With Visual Basic (7th Edition)
Concepts of Programming Languages (11th Edition)
- Write a program to input a list of numbers in an array and then rearrange this array in such a waythat all the odd numbers appear in the beginning of the array and all the even numbers appear in theend of the array. E.g., if the input list is 1,4,2,7,6,5,9, then after rearrangement the list should be:1,7,5,9,4,2,6.arrow_forwardWrite an c progra, that takes an array of N integers (N will be specified by the user at run time). It then finds how many numbers in the array are divisible by 3 and how many numbers are divisible by 7. the it copies, from the input array above, the numbers that are divisible by 3 into a new array and those divisible by 7 into a different new array. Once copied, the algorithm should compute the average of each of these two new arrays and print the averages on the screen with suitable message to let the user know which average is being printed Note: an integer number is divisible by 3 (respectively 7) if the remaining of its division by 3 (respectively 7) is 0. You are allowed to use the modulus operator %.arrow_forwardWrite a program to Build a 100-element array with the integers 1 through 100 for which shellsort utilises as many comparisons as it can discover, using increments of 1 4 13 40.arrow_forward
- Write a program that prompts the user to enter an integerm and find the smallest integer n such that m * n is a perfect square. (Hint:Store all smallest factors of m into an array list. n is the product of the factors thatappear an odd number of times in the array list. For example, consider m = 90,store the factors 2, 3, 3, and 5 in an array list. 2 and 5 appear an odd number oftimes in the array list. Thus, n is 10.) Here are some sample run: Enter an integer m: 1500 ↵EnterThe smallest number n for m * n to be a perfect square is 15m * n is 22500arrow_forwardImplement the buildDistributionArray function to take an array of scores, built by parseScores, as an argument. A grade distribution array of length 5 is returned. Loop through the scores array and tally up the number of A, B, C, D, and F scores using the standard scoring system (90 and above = A, 80‐89 = B, 70‐79 = C, etc.). Store these totals in a distribution array where the number of As is the first number, number of Bs is the second number, etc. Ex: ["45","78","98","83","86","99","59"] → [2, 2, 1, 0, 2] buildDistributionArray returns [0, 0, 0, 0, 0] when the scoresArray argument is empty.arrow_forwardPROGRAM in JAVA Write a program that defines two arrays - one of strings and one of integers, both of size 10. Your program should then ask the user to enter the a string representing a persons name (just one name - their family name - not their given name as well), and an integer representing their age. It should continue to do this until either the user enters ‘done’ instead of a name, or until the array is full (that is, 10 pairs of names and ages have been entered). It should then print out the names and ages as well as the names of the youngest and oldest. Hints: One tricky (deliberately) part is making sure that once you’ve typed ‘done’ to finish entering names, your program does not then ask you for the age of the person with name ‘done’ - be careful about this. This is one of the few cases where, if you are careful, you can sensibly use a ‘break’ statement outside of a switch statement Stage 6: Assessed Task Redo Stage 5 above but replace the arrays with ArrayLists.arrow_forward
- Write a Java program to find continuous sub array of the given array whose sum is equal to a given number. For example, If {12, 5, 31, 9, 21, 8} is the given array and 45 is the given number, then you have to find continuous sub array in this array such that whose elements add up to 45. In this case, {5, 31, 9} is such sub array whose elements add up to 45.arrow_forwardWrite a program that reads from the user an array with one dimension n and then find the largest even and smallest negative element numbers.arrow_forwardWrite a program that reads an array A of N elements containing only 0's and 1's. Your program should find the position of a 0 and replace it with a 1 to get the longest continuous sequence of 1's. Let this position of 0 be called P. Print P if such a 0 exists and print -1 if the original array A contains only 1's. Assume the array indexing starts from 0.arrow_forward
- Write a program to take input of 100 integers in a dynamically allocated array using malloc() function, then find the average of numbers that are in between 20 and 80. For example if an array contains [10, 40, 15, 20, 30, 90] then the average will be (40+20+30) / 3 = 30.arrow_forwardWrite a Java class that creates a 3x4 array of 12 random ints between 0 and 100. Write a method to return the sum of the elements in the last column of each row in the two-dimensional array.arrow_forwardWrite a program that creates an array of size 6 and input values to it through user input. It turnsout that these values that the user inputs were biased, and had an offset relative to the index ofthe array (for example, the first value had no bias since index = 0, the second value had a bias of 1since index = 1, and so on...).Pass this array to a function, that should remove the bias from each value by subtracting the biasfrom the original value. You need to subtract values using a for loop.Print the updated values of the array in the main function after the call to this bias removerfunction.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
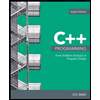