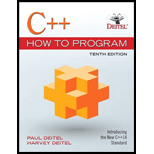
(Knight's Tour) One of the more interesting puzzlers for chess buffs is the Knight's Tour
problem. The question is this: Can the chess piece called the knight move around an empty chess-
board and touch each of the 64 squares once and only once? We study this intriguing problem in
depth in this exercise.
The knight makes L-shaped moves (over two in one direction then over one in a perpendicular
direction). Thus, from a square in the middle of an empty chessboard. the knight can make
eight different moves (numbered 0 through 7) as shown in Fig. 7.25.
- Draw an 8-by-8 chessboard on a sheet of paper and attempt a Knight's Tour by hand' Put a 1 in the first square you move to. a 2 in the second square, A 3 in the third,
- Now let's develop a
program that will move the knight around a chessboard. The board Is represented by an 8-by-8 two-dimensional array board. Each of the squares is initialized
Before starting the tour, estimate how far you think you'll get, remembering that a full
tour consists of 64 moves. How Ear did you get? Was this close to your estimate?
zero. We describe each of the eight possible moves in terms of both their horizontal
and vertical components. For example, a move of type 0. as shown in Fig, 7.25,
consists of moving two squares horizontally to the right and one square vertically up-
ward. Move 2 consists of moving one square horizontally to the left and squares
0 1 2 3 4 5 6 7 0 1 2 1 2 3 0 3 K 4 4 7 5 5 6 6 7
Fig.7.25 The eight possible moves of the knight
Vertically upward. Horizontal moves to the left and vertical moves upward are indicated with negative numbers. The eight moves may be described by two one-dimensional arrays, horizontal and vertical, as follows:
Horizontal [0] =2 vertical [0] = 1 Horizontal [1] = 1 vertical [1] = 2 Horizontal [2] =-1 vertical [2] = -2 Horizontal [3] = -2 vertical [3] = -1 Horizontal [4] = -2 vertical [4] = 1 Horizontal [5] = -1 vertical [5] = 2 Horizontal [6] = 1 vertical [6] = 2 Horizontal [7]= 2 vertical [7] = 1
Let the variables and currentCoIumn indicate the row and column of
the knight's current position. To make a move of type moveNumber, where is moveNumber is
between 0 and 7. your program uses the statements
CurrentRow += vertical [moveNumber];
currentColumn += horizontal [moveNumber];
Keep a counter that varies I to 64. Record latest in each square the knight moves to. Remember to test cach potential move to see if the knight has already visited that square, and of course, test every potential move to make sure that the knight does not land off the chessboard. Now "Tite a program to move the knight around the chessboard. Run the program. How many moves did the knight make?
c) After attempting to write and run a Knight's Tour program. you've probablv developed some value Insights. We'll use these develop a heuristic (or strategy) for moving the knight. Heuristics do not guarantee success, but a carefully developed heuristic greatly improves the chance of success. You may have observed that the outer squares are more troublesome than the squares nearer the center of the board. In fact, the most troublesome, or inaccessible, squares arc the four corners.
Intuition may suggest that you should attempt to move the knight to the most troublesome squares first and leave open those that easiest to get to, so when the board gets congested near the end of the tour. there will be a greater chance of success.
We may may develop an "accessibility heuristic" by classifying each square according to how accessible it's then always moving the knight to the square (within the knight's L shaped moves, of course) that's least accessible. We label a two-dimensional array accessibility with numbers indicating from how many squares each particular square is accessible. On a blank chessboard, each center square is rated as 8, each corner square is rated as 2 and the other squares have accessibility numbers of 3,4 or 6 as follows
-
2 3 4 4 4 4 3 2
3 4 6 6 6 6 4 3
4 6 8 8 8 8 6 4
4 6 8 8 8 8 6 4
4 6 8 8 8 8 6 4
4 6 8 8 8 8 6 4
3 4 6 6 6 6 4 3
2 3 4 4 4 4 3 2
Now write a version of the Knight's Tour program using the accessibility heuristic.
At any time, the knight should move to the square with the lowest accessibility num ber. In case of a tie, the knight may move to any of the tied squares. Therefore, the tour may begin in any of the four corners. [Note: As the knight moves around the chess- board, your program should reduce the accessibility numbers as more and more Squares become occupied. In this way, at any given time during the tour, each available square's accessibility number will remain equal to precisely the number of squares from which that square may be reached.] Run this version of your program. Did you get a full tour? Now modify the program to run 64 tours, one starting from each square of the chessboard. How many full tours did you get?
d.Write a version of the Knight's Tour program Which, When encountering a tie between two or more Squares, decides what square to choose by looking ahead to those squares reachable from the "tied" squares. Your program should move to the square for which the next move would arrive at a square with the lowest accessibility number.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
C++ How to Program (10th Edition)
- Python: A ball falls freely from a height of 100 meters, and bounces back to half of its original height after each landing; again, how many meters does it travel when it hits the ground for the 10th time? How high is the 10th rally?arrow_forward8. using c++, Write a function method that determines the mean of all the values in an array of integers. Your mean function should call a separate function that you write that determines the sum. Don’t use built-in sum or mean gadgets, but roll your own.arrow_forwardis it possible to change this function from recursive to iterative one? Any help would be greatly appreciated.arrow_forward
- TROUBLESHOOTING: Fix the errors in the code below and run the script with your modified code function [x,numIter,omega] = gaussSeidel(func,x,maxIter,epsilon) % Solves Ax = b by Gauss-Seidel method with relaxation. % USAGE: [x,numIter,omega] = gaussSeidel(func,x,maxIter,epsilon) % INPUT: % func = handle of function that returns improved x using % x = starting solution vector % maxIter = allowable number of iterations (default is 500) % epsilon = error tolerance (default is 1.0e-9) % OUTPUT: % x = solution vector % numIter = number of iterations carried out % omega = computed relaxation factor if nargin < 4; epsilon = 1.0e-9; end if nargin < 3; maxIter = 500; end k = 10; p = 1; omega = 1; for numIter = 1:maxIter xOld = x; x = feval(func,x,omega); dx = sqrt(dot(x - xOld,x - xOld)); if dx < epsilon; return; end if numIter == k; dx1 = dx; end if numIter == k + p omega = 2/(1 + sqrt(1 - (dx/dx1)ˆ(1/p))); end end error(’Too many iterations’)arrow_forwardNeed answer of this code. Waiting for responsearrow_forwarddo the followinfarrow_forward
- SUCCESSIVE-OVER-RELAXATION Method TROUBLESHOOTING: Fix the errors in the code below and run the script with your modified code and screenshot your output and codes with answer function [x,numIter,omega] = gaussSeidel(func,x,maxIter,epsilon) % Solves Ax = b by Gauss-Seidel method with relaxation. % USAGE: [x,numIter,omega] = gaussSeidel(func,x,maxIter,epsilon) % INPUT: % func = handle of function that returns improved x using % x = starting solution vector % maxIter = allowable number of iterations (default is 500) % epsilon = error tolerance (default is 1.0e-9) % OUTPUT: % x = solution vector % numIter = number of iterations carried out % omega = computed relaxation factor if nargin < 4; epsilon = 1.0e-9; end if nargin < 3; maxIter = 500; end k = 10; p = 1; omega = 1; for numIter = 1:maxIter xOld = x; x = feval(func,x,omega); dx = sqrt(dot(x - xOld,x - xOld)); if dx < epsilon; return; end if numIter == k; dx1 = dx; end if numIter == k + p omega = 2/(1 + sqrt(1 -…arrow_forwardProgram this questionarrow_forwardCan anyone please help me to write this code using recursion function?arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
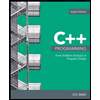