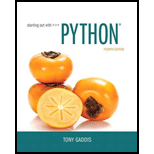
Starting Out with Python (4th Edition)
4th Edition
ISBN: 9780134444321
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 11PE
Program Plan Intro
Most Frequent Character
Program Plan:
- • Declare a function named “findFrequentCharacter()” that accepts a string “my_string” as input. Inside the function,
- ○ Create a list variable “countList” to store the frequency of all alphabets.
- ○ Use the “for” loop to process all the characters in the “my_string” to check for frequency.
- ○ Inside the “for” loop,
- ■ Check if the current character “ch” is the alphabet.
- ■ If so, then find the ASCII value of the character and store it in “n”.
- ■ Check if the ASCII value of the character indicates the uppercase alphabet.
- ■ If the condition is true, then find the index of the character in the “countList” by subtracting “65” from “n”.
- ■ If the condition fails, then ASCII value of the character indicates the lowercase alphabet.
- ■ Thus, find the index of the character in the “countList” by subtracting “97” from “n” value and store it in “n”.
- ■ Using the “n” as the index, increment the value at “n” in the “countList” by “1”.
- ○ After processing all the characters in the “my_string”, declare a variable “maxVal” to hold the highest value of occurrences.
- ○ Also, declare a variable “maxValPos” to hold the position of the “maxVal” in the “countList”.
- ○ Use the “for” loop to find the highest value in the “countList”.
- ○ Inside the “for” loop,
- ■ Check if the current value is higher than “maxVal”.
- ■ If so, then update the “maxVal” with the current value and update the “maxValPos”.
- ○ Convert the “maxVal” into the ASCII value by adding “97” with it.
- ○ After that, convert the “maxVal” into character and return it.
- • Declare a function named “main()”. Inside the “main()” function,
- ○ Get a string from the user and store it in “my_string” variable.
- ○ Call the function “findFrequentCharacter()” and pass “my_string” as input to the function and store the return value in “frequentCharacter”.
- ○ Display the “frequentCharacter” using the “print()” function.
- • In the last, call the function “main()” to execute the program.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Most Frequent Character Create an application that lets the user enter a string and displays the character that appears most frequently in the string.
True or False You cannot store a string in a variable of the char data type.
True or False All variables have a ToString method that you can call to convert the variable’s value to a string.
Chapter 8 Solutions
Starting Out with Python (4th Edition)
Ch. 8.1 - Assume the variable name references a string....Ch. 8.1 - What is the index of the first character in a...Ch. 8.1 - If a string has 10 characters, what is the index...Ch. 8.1 - Prob. 4CPCh. 8.1 - Prob. 5CPCh. 8.1 - Prob. 6CPCh. 8.2 - Prob. 7CPCh. 8.2 - Prob. 8CPCh. 8.2 - Prob. 9CPCh. 8.2 - What will the following code display? mystring =...
Ch. 8.3 - Prob. 11CPCh. 8.3 - Prob. 12CPCh. 8.3 - Write an if statement that displays Digit" if the...Ch. 8.3 - What is the output of the following code? ch = 'a'...Ch. 8.3 - Write a loop that asks the user Do you want to...Ch. 8.3 - Prob. 16CPCh. 8.3 - Write a loop that counts the number of uppercase...Ch. 8.3 - Assume the following statement appears in a...Ch. 8.3 - Assume the following statement appears in a...Ch. 8 - This is the first index in a string. a. 1 b. 1 c....Ch. 8 - This is the last index in a string. a. 1 b. 99 c....Ch. 8 - This will happen if you try to use an index that...Ch. 8 - This function returns the length of a string. a....Ch. 8 - This string method returns a copy of the string...Ch. 8 - This string method returns the lowest index in the...Ch. 8 - This operator determines whether one string is...Ch. 8 - This string method returns true if a string...Ch. 8 - This string method returns true if a string...Ch. 8 - This string method returns a copy of the string...Ch. 8 - Once a string is created, it cannot be changed.Ch. 8 - You can use the for loop to iterate over the...Ch. 8 - The isupper method converts a string to all...Ch. 8 - The repetition operator () works with strings as...Ch. 8 - Prob. 5TFCh. 8 - What does the following code display? mystr =...Ch. 8 - What does the following code display? mystr =...Ch. 8 - What will the following code display? mystring =...Ch. 8 - Prob. 4SACh. 8 - What does the following code display? name = 'joe'...Ch. 8 - Assume choice references a string. The following...Ch. 8 - Write a loop that counts the number of space...Ch. 8 - Write a loop that counts the number of digits that...Ch. 8 - Write a loop that counts the number of lowercase...Ch. 8 - Write a function that accepts a string as an...Ch. 8 - Prob. 6AWCh. 8 - Write a function that accepts a string as an...Ch. 8 - Assume mystrinc references a string. Write a...Ch. 8 - Assume mystring references a string. Write a...Ch. 8 - Look at the following statement: mystring =...Ch. 8 - Initials Write a program that gets a string...Ch. 8 - Sum of Digits in a String Write a program that...Ch. 8 - Date Printer Write a program that reads a string...Ch. 8 - Prob. 4PECh. 8 - Alphabetic Telephone Number Translator Many...Ch. 8 - Average Number of Words If you have downloaded the...Ch. 8 - If you have downloaded the source code you will...Ch. 8 - Sentence Capitalizer Write a program with a...Ch. 8 - Prob. 10PECh. 8 - Prob. 11PECh. 8 - Word Separator Write a program that accepts as...Ch. 8 - Pig Latin Write a program that accepts a sentence...Ch. 8 - PowerBall Lottery To play the PowerBall lottery,...Ch. 8 - Gas Prices In the student sample program files for...
Knowledge Booster
Similar questions
- 4-20) (Process a string) Write a program that prompts the user to enter a string and displays its length and its first character.arrow_forwardTrue or False: The String class’s replace method can replace individual characters, but cannot replace substrings.arrow_forwardT F: The string concatenation operator automatically inserts a space between thejoined strings.arrow_forward
- Sum of Numbers in a String Create an application that lets the user enter a string containing series of numbers separated by commas. Here is an example of valid input: 7,9,10,2,18,6 The program should calculate and display the sum of all the numbers.arrow_forward4-20) (Process a string) Write a program that prompts the user to enter a string and displays its length and its first character. Enter a string The string is of length and the first character isarrow_forwardC# language MORSE CODE CONVERTERDesign a program that asks the user to enter a string and thenconverts that string to Morse code. Morse code is a code whereeach letter of the English alphabet, each digit, and variouspunctuation characters are represented by a series of dots anddashes. Table 8-9 shows part of the code. Needs to be two labels, two text boxes and click button for conversionarrow_forward
- Assume mystring references a string. Write a statement that uses a slicing expression and displays the first 3 characters in the string.arrow_forwardTrue or False: The << insertion operator stops reading a string when it encounters a space..arrow_forwardInitialsWrite a python program that gets a string containing a person’s first, middle, and last names, anddisplays their first, middle, and last initials. For example, if the user enters John William Smith,the program should display J. W. S.arrow_forward
- use C++ Assume letters A, E, I, O, and U as the vowels. Write a program that prompts the user to enter a string and displays the number of vowels and consonants in the string. A sample run is shown below:arrow_forwardC# language MORSE CODE CONVERTER Design a program that asks the user to enter a string and thenconverts that string to Morse code. Morse code is a code whereeach letter of the English alphabet, each digit, and variouspunctuation characters are represented by a series of dots anddashes. Table 8-9 shows part of the code.arrow_forwardUtilizing an instance of a string object may help you locate the substring.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
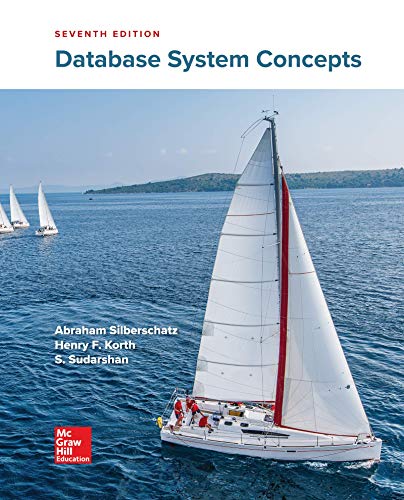
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
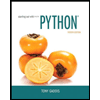
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
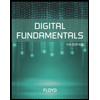
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
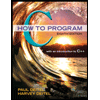
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
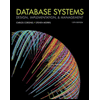
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
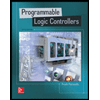
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education