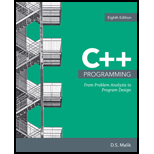
Mark the following statements as true or false.
A double type is an example of a simple data type. (1)
A one-dimensional array is an example of a structured data type. (1)
The size of an array is determined at compile time. (1,6)
Given the declaration:
int list[10];
the statement:
list[5] - list[3] * list[2];
updates the content of the fifth component of the array list. (2)
If an array index goes out of bounds, the
program always terminates in an error. (3)The only aggregate operations allowable on int arrays are the increment and decrement operations. (5)
Arrays can be passed as parameters to a function either by value or by reference. (6)
A function can return a value of type array. (6)
In C++, some aggregate operations are allowed for strings. (11,12,13)
The declaration:
char name [16] = "John K. Miller";
declares name to be an array of 15 characters because the string "John K. Miller" has only 14 characters. (11)
The declaration:
char str = "Sunny Day";
declares str to be a string of an unspecified length. (11)
As parameters, two-dimensional arrays are passed either by value or by reference. (15,16)
a.
A double type is a simple data type. Hence, the given statement is “True”.

A data type is called simple if variables of that type can store only one valueat a time. In contrast, in a structured data type, each data item is a collection of otherdata items. Simple data types are building blocks of structured data types.
A double type is a simple data type. Hence, the given statement is “True”.
Explanation of Solution
Since a double type can store a single value at a time it is a simple data type.
b.
One-dimensional array is an example of a structured data type. Hence, the given statement is “True”.

A data type is called simple if variables of that type can store only one valueat a time. In contrast, in a structured data type, each data item is a collection of otherdata items. Simple data types are building blocks of structured data types.
One-dimensional array is an example of a structured data type. Hence, the given statement is “True”.
Explanation of Solution
One-dimensional array is an example of a structured data type, as it can store a collection of other data items.
c.
The size of an array is determined at compile time. Hence, the given statement is “True”.

An array is a collection of a fixed number of components (also called elements)all of the same data type and in contiguous (that is, adjacent) memory space.
The size of an array is determined at compile time. Hence, the given statement is “True”.
Explanation of Solution
An array is a collection of a fixed number of components hence the size of an array is determined at compile time.
d.
The statement does not update the fifth component. Hence, the given statement is “False”.

In C++[ ] is an operator called the array subscripting operator and the array index starts at 0.
The statement does not update the fifth component. Hence, the given statement is “False”.
Explanation of Solution
The statement updates the sixth component of the array list as the subscript begins with 0.
e.
If an array index goes out of bounds, the program does not necessarily terminatein an error. Hence, the given statement is “False”.

C++ does not check whether the index value is within range. If the index goes out of bounds and the program tries toaccess the component specified by the index, then whatever memory location is indicatedby the index that location is accessed. This can result in altering or accessingthe data of a memory location that was never intended to be modified or accessed. It can also lead to accessing a protected memory that causes the program to instantly halt. Hence, several unknown things can happen if the index goes out of bounds during execution.
If an array index goes out of bounds, the program does not necessarily terminatein an error. Hence, the given statement is “False”.
Explanation of Solution
If the index goes out of bounds and the program tries to access the component specified by the index, then whatever memory location is indicated by the index that location is accessed. This may or may not cause the program to instantly halt or terminate in an error.
f.
C++ does not allow aggregateoperations on an array. Hence, the given statement is “False”.

An aggregate operation on an array is any operation thatmanipulates the entire array as a single unit.
C++ does not allow aggregateoperations on an array. Hence, the given statement is “False”.
Explanation of Solution
C++ does not allow aggregate operations on an array.
g.
Arrays can be passed as parameters to a function never by value but only by reference. Hence, the given statement is “False”.

In C++, arrays are passed by reference only. Because arrays are passed by reference only, the &symbol is never used when declaringan array as a formal parameter.
Arrays can be passed as parameters to a function never by value but only by reference. Hence, the given statement is “False”.
Explanation of Solution
In C++, arrays are passed by reference only and never by value.
h.
A function cannot return a value of type array. Hence, the given statement is “False”.

A function cannot return a value of type array.
A function cannot return a value of type array. Hence, the given statement is “False”.
Explanation of Solution
A function cannot return a value of type array.
i.
In C++, some aggregate operations are allowed for strings. Hence, the given statement is “True”.

Most rules that apply to arrays apply to C-strings as well. Aggregateoperations, such as assignment and comparison, are not allowed on arrays. Also the input/output of arrays is done component-wise. But, C++ allows aggregate operations of the input and output on C-strings which are nothing but character arrays terminated with the null character.
In C++, some aggregate operations are allowed for strings. Hence, the given statement is “True”.
Explanation of Solution
C++ allows aggregate operations of the input and output on C-strings which are nothing but character arrays.
j.
The name array is of size 16 and not 15. Hence, the given statement is “False”.

The name array is of size 16 which is mentioned in the declaration of the variable and not the size of string stored into it. There remains 1 unused component in the name array.
The name array is of size 16 and not 15. Hence, the given statement is “False”.
Explanation of Solution
The name array is of size 16 which is mentioned in the declaration of the variable and not the size of string stored into it. There remains 1 unused component in the name array.
k.
Aggregate operation such as assignment is not allowed on arrays and the statement is illegal. Hence, the given statement is “False”.

Aggregate operation such as assignment is not allowed on arrays and the statement is illegal. For assignment operation on c-strings (char arrays) c-string functions are needed.
Aggregate operation such as assignment is not allowed on arrays and the statement is illegal. Hence, the given statement is “False”.
Explanation of Solution
Aggregate operation such as assignment is not allowed on arrays and the statement is illegal. For assignment operation on c-strings (char arrays) c-string functions are needed.
l.
Two-dimensional arrays can be passed as parameters to a function, and they arepassed by reference only. Hence, the given statement is “False”.

Two-dimensional arrays can be passed as parameters to a function, and they arepassed by reference.
Two-dimensional arrays can be passed as parameters to a function, and they arepassed by reference only. Hence, the given statement is “False”.
Explanation of Solution
Two-dimensional arrays can be passed as parameters to a function, and they arepassed by reference.
Want to see more full solutions like this?
Chapter 8 Solutions
C++ PROGRAMMING:FROM...(LL) >CUSTOM<
- When using functions in python, it allows us tto create procedural abstractioons in our programs. What are 5 major benefits of using a procedural abstraction in python?arrow_forwardFind the error, assume data is a string and all variables have been declared. for ch in data: if ch.isupper: num_upper = num_upper + 1 if ch.islower: num_lower = num_lower + 1 if ch.isdigit: num_digits = num_digits + 1 if ch.isspace: num_space = num_space + 1arrow_forwardFind the Error: date_string = input('Enter a date in the format mm/dd/yyyy: ') date_list = date_string.split('-') month_num = int(date_list[0]) day = date_list[1] year = date_list[2] month_name = month_list[month_num - 1] long_date = month_name + ' ' + day + ', ' + year print(long_date)arrow_forward
- Find the Error: full_name = input ('Enter your full name: ') name = split(full_name) for string in name: print(string[0].upper(), sep='', end='') print('.', sep=' ', end='')arrow_forwardPlease show the code for the Tikz figure of the complex plane and the curve C. Also, mark all singularities of the integrand.arrow_forward11. Go to the Webinars worksheet. DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: a. Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. b. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. c. Determine and enter the constraints based on the information provided in Table 3. d. Use Simplex LP as the solving method to find a global optimal solution. e. Save the Solver model below the Maximum weekly profit model label. f. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and…arrow_forward
- Go to the Webinars DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. Determine and enter the constraints based on the information provided in Table 3. Use Simplex LP as the solving method to find a global optimal solution. Save the Solver model below the Maximum weekly profit model label. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and Thursday…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)? When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene. Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency. To elaborate,…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…arrow_forward
- Male comedians were typically the main/dominant star of television sitcoms made during the FCC licensing freeze. Question 19 options: True False In the episode of The Honeymooners that you watched this week, why did Alice decide to get a job outside of the home? Question 1 options: to earn enough money to buy a mink coat to have something to do while the kids were at school to pay the bills after her husband got laid offarrow_forwardAfter the FCC licensing freeze was lifted, sitcoms featuring urban settings and working class characters became far less common. Question 14 options: True Falsearrow_forwardsolve this questions for me .arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
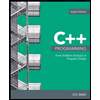
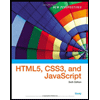
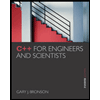
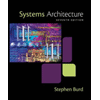
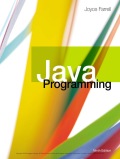