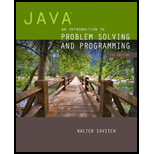
Explanation of Solution
Creating “Main.java”:
- Create a class named “Main”.
- Define the “main ()” method.
- Declare required variables.
- Do till the user enters “n” using “while” condition.
- Get the string from the user.
- Check if the length of the string is greater than or equal to 20.
- Create an object “e” for the class. Here the exception is thrown using default constructor.
- Get and print the message using the method “e.getMessage()”.
- Else,
- Print the number of characters.
- Ask whether the user wants to continue or not and store the response in a variable “response”.
- Check if the response is equal to “n”.
- Break the loop.
- Define the “main ()” method.
Creating “MessageTooLongException.java”:
- Create a class named “MessageTooLongException” that extends “Exception”.
- Define a default constructor that calls the parent class’s method using “super ()” by passing a message.
- Define a parameterized constructor that calls the parent class’s method using “super ()” by passing a message that is given as the argument.
Program:
MessageTooLongException.java:
//Define a class
public class MessageTooLongException extends Exception
{
//Default constructor
public MessageTooLongException()
{
//Call the parent class by passing the message
super("Message Too Long!");
}
//Parameterized constructor
public MessageTooLongException(String message)
{
//Call the parent class by passing the message
super(message);
}
}
Main.java:
//import the package
import java.util.Scanner;
//Main class
class Main
{
//Define main method
public static void main(String[] args)
{
//Create an object for the scanner class
Scanner sc = new Scanner(System.in);
//Declare required variables
String str, response = "y";
//Do till the user enters 'n'
while(response...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: Introduction to Problem Solving and Programming
- Write a class TimeOfDay that uses the exception classes defined in the previous exercise. Give it a method setTimeTo(timeString) that changes the time if timeString corresponds to a valid time of day. If not, it should throw an exception of the appropriate type. [Java]arrow_forwardWrite a program that prompts the user to enter a length in feet and then enter a length in inches and outputs the equivalent length in centimeters. If the user enters a negative number or a non-digit number, throw and handle an appropriate exception and prompt the user to enter another set of numbers. Your error message should read A non positive number is entered and allow the user to try again. Format your output with setprecision(2) to ensure the proper number of decimals for testing!arrow_forwardWrite a JAVA program to calculate percentage of a student for 3 different subjects. Create an exception if the marks entered greater than 100.arrow_forward
- C++Write a program SEGMENT that does the following: Asks the user for his/her birthday using 2 integer values, i.e., int month, int year. Using a try/catch block with two catch blocks, check for the following exceptions: Invalid month (i.e., a month less than or equal to 0 or greater than 12). Throw a string exception to be caught by a catch block accepting a string. Output the message thrown by the exception indicating the month is an invalid month. Year less than 1900 or greater than 2020. Throw an integer exception to be caught by a catch block accepting an integer. In the catch block, if the integer thrown is less than 1900, output the message “You are very old”. If the integer exception is greater than 2020, output the message “You have not been born yet!” If neither exception occurs, simply output (cout) a message with the user’s birth month and year, for example, “You were born in “ << month << “ and “ << year. Declare any variables needed.…arrow_forwardOnly new java code can be added after the code given: The while loop makes multiple attempts to read a nonnegative integer from input into userAge. Use multiple exception handlers to: Catch an InputMismatchException, output "Unexpected input: The UserAge program quits", and assign retry with false. Catch an Exception and output the message of the Exception. End each output with a newline. Ex: If the input is 44, then the output is: Valid input: User's age is 44 Ex: If the input is L, then the output is: Unexpected input: The UserAge program quits Ex: If the input is -65 44, then the output is: User's age must be nonnegative Valid input: User's age is 44 import java.util.Scanner;import java.util.InputMismatchException; public class UserAge { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int userAge; boolean retry = true; while (retry) { try { userAge = scnr.nextInt(); if (userAge < 0) {…arrow_forwardWrite a program that calls a method that throws an exception of type ArithmeticExcepton at arandom iteration in a for loop. Catch the exception in the method and pass the iteration countwhen the exception occurred to the calling method by using an object of an exception class youdefinein java codearrow_forward
- Programme Leader of ITMB wants to check whether the student number and GSM number entered by the student for the programming contest is valid or not. Write a Java program to read the Student ID and GSM Number of a student. Use a method called ValidityDetails () for checking the validity of details entered. If the Student ID doesn’t start with the characters ST and contains more than 6 letters or if the Mobile Number does not contain exactly 8 digits, throw a user defined exception InvalidDetailsException. If the details entered are valid, display the message ‘”correct details are entered!!!” otherwise display “Entered invalid details!!!!”arrow_forwardباستخدام جافا Write a program that allows the user to compute the subtracted value afterthe subtraction of two integer values. The subtraction value of x - y isstored in z. Catch any exception thrown and allow the user to enter newvalues.arrow_forwardWrite a C++ program by using Exception(throw, try, catch), validate a grade. ask the user to enter a grade and then if the grade is less than zero or more than 100 then throw an exception. you have to ask user to enter 3 grades. if all grades are valid you calculate the average and print it, if any of the 3 grades is invalid, throw an exception.arrow_forward
- Write a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError and output an exception message. Use another except block to catch any ValueError caused by invalid input and output an exception message. Note: ZeroDivisionError is thrown when a division by zero happens. ValueError is thrown when a user enters a value of different data type than what is defined in the program. Do not include code to throw any exception in the program. Ex: If the input of the program is: 15 3 the output of the program is: 5 Ex: If the input of the program is: 10 0 the output of the program is: Zero Division Exception: integer division or modulo by zero Ex: If the input of the program is: 15.5 5 the output of the program is: Input Exception: invalid literal for int() with base 10: '15.5' My code: # Type your code here.user_num = input()div_num =…arrow_forwardWrite a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError and output an exception message. Use another except block to catch any ValueError caused by invalid input and output an exception message. Note: ZeroDivisionError is thrown when a division by zero happens. ValueError is thrown when a user enters a value of different data type than what is defined in the program. Do not include code to throw any exception in the program. Ex: If the input of the program is: 15 3 the output of the program is: 5 Ex: If the input of the program is: 10 0 the output of the program is: Zero Division Exception: integer division or modulo by zero Ex: If the input of the program is: 15.5 5 the output of the program is: Input Exception: invalid literal for int() with base 10: '15.5' My code: # Type your code here.user_num = int(input())div_num =…arrow_forwardWrite an exception class that is appropriate for indicating that a time enteredby a user is not valid. The time will be in the format hour:minute followed by“am” or “pm.”arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
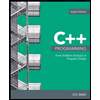