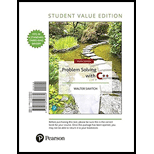
Palindrome testing with pointers
This Practice
bool isPalindrome(char* cstr) { char* front = cstr; char* back = cstr + strlen(cstr)−1; while (front < back) { // Complete code here } return true; } |
Here is a sample main function for quick and dirty testing:
int main() { char s1[50] = "neveroddoreven"; char s2[50] = "not a palindrome"; cout << isPalindrome(s1) << endl; // true cout << isPalindrome(s2) << endl; // false return 0; } |

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Problem Solving with C++, Student Value Edition Plus MyLab Programming with Pearson eText - Access Card Package (10th Edition)
Additional Engineering Textbook Solutions
C Programming Language
Starting Out with C++ from Control Structures to Objects (9th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
C How to Program (8th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- (Pointers + Dynamic 1D Arrays) c++ program please use only pointers and dynamic 1D array and please don't use recursion and vector or otherarrow_forwardSubmarining is a phenomenon that occurs when the pointer disappears when you move it too rapidly.arrow_forwardC++ ---- (the bold is code)------ Consider the function interface below: bool isSame(int x, double y); Write a line of code that declares a pointer named Funprt that points to this function.arrow_forward
- C++ Struct Pointers Help: I have a file called names.txt. Write a program that reads each line and then store the name and nickname under an individual pointer to the class Person. names.txt: Norman, Normie Justine, Jussy Richard, Dick Shelley, Shell class Person { public: string name; string nickname; Person(string name, nickname) { this->name=name; this->nickname = nickname; } }; Print out each pointer's name and nickname to verify that it has been stored.arrow_forward1. Write a C++ function named "extractDebugOption" that accepts arguments similar to argc (an integer for the array size) and argv (an array of pointers to C-string). It will determine whether it has the argument of "/debug yes" or "/debug no". It will return true if the debug option is yes. If "/debug no" or there is no debug option or "/debug" without "yes" or "no" followed, it will return false. Note: command line arguments are simply an array of pointers to C-string. Please test it with the following command line arguments These will return true"/debug yes""/compile /debug yes" These will return false"/debug no""/debug""debug""yes /debug""no /debug"arrow_forwardC++ Pointers must be used to solve the following problem. Write a function createPassword() with no return value to randomly select 8 capital letters from the alphabet. The function receives the address of the first characters of the password string as parameter. Hint: Declare a characters string that contains all the capital letters of the alphabet and use the index values of the characters in the string for random selection of characters, e.g.char alpha [27] = “ABCDEFGHIJKLMNOPQRSTUVWXYZ”;char *pAlpha = alpha; Display the password from the main() function. Write a function replaceVowels() with no return value to replace all the vowels (A, E, I, O, U) that have been selected with a symbol from the list ($ % @ # &). That is, A must be replaced by $, E replaced by %, I mustbe replaced by @, O replaced by #, and U must be replaced by &. The function receives the address of the first characters of the password string as parameter. Display the password agin from the main()…arrow_forward
- 1. Write a function named “getSumBeforeNegative” that takes in a pointer to the array of integers and its size. It will return the sum of all values before it sees a negative number. If there is no negative number in the list, it returns the sum of all numbers in the array.For example,int numList[] = {10,20,-1, 30);// will return 30int priceList[] = {10, 20, 30);// will return 60It must use pointers to integer instead of array index.Write a main program and show how this function is called and used.arrow_forwardIn c++, please and thank you! Write a function that dynamically allocates an array of integers. The function should accept an integer argument indicating the number of elements to allocate. The function should return a pointer to the array.arrow_forwardReverse ArrayWrite a function that accepts an int array and the array’s size as arguments. The function should create a copy of the array, except that the element values should be reversedin the copy. The function should return a pointer to the new array. Demonstrate thefunction in a complete program.arrow_forward
- It's well knowledge that "dangling and wild pointers" are problematic for pointers. Use specific examples to back up your argument.arrow_forward. Storage of strings through pointers saves memory space. Justify your answer with anexample.arrow_forwardC++ Write a function that accepts an array and its size as arguments, creates a new array that is a copy of the argument array, and returns a pointer to the new array. Display the array in main(). The function will work as follows: Accept an array and its size as arguments. Dynamically allocate a new array that is the same size as the argument array. Copy the elements of the argument array to the new array. Return a new pointer to the new array. // This program uses a function to duplicate// an int array of any size.#include <iostream>using namespace std;// Function prototype_________________________________________________int main(){ // Define constants for the array sizes. const int SIZE1 = 5, SIZE2 = 7, SIZE3 = 10; // Define three arrays of different sizes. int array1[SIZE1] = { 100, 200, 300, 400, 500 }; int array2[SIZE2] = { 10, 20, 30, 40, 50, 60, 70 }; int array3[SIZE3] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; // Define three pointers for the duplicate arrays. int *dup1 =…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
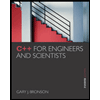
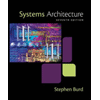