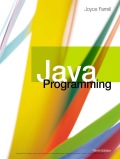
Explanation of Solution
Program:
File name: “Salesperson.java”
//Define a class named Salesperson
public class Salesperson
{
//Declare the private variables
private int id;
private double sales;
/*Define a method named Salesperson
that takes two arguments*/
Salesperson(int idNum, double amt)
{
//Assign the values
id = idNum;
sales = amt;
}
//Define a get method that returns the ID number
public int getId()
{
//Return the value
return id;
}
//Define a get method that returns the sales value
public double getSales()
{
//Return the value
return sales;
}
//Define a set method that takes the ID number
public void setId(int idNum)
{
//Assign the value
id = idNum;
}
//Define a set method that takes the sales value
public void setSales(double amt)
{
//Assign the value
sales = amt;
}
}
File name: “SalespersonSort.java”
//Import necessary header files
import javax.swing.*;
//Define a class named SalespersonSort
public class SalespersonSort
{
//Define a main method
public static void main(String[] args)
{
//Declare an array to store seven Salesperson objects
Salesperson[] salespeople = new Salesperson[7];
//Declare the variables
int x;
int id;
double sales;
String order;
String message = "";
//For loop to be executed until x exceeds 7
for(x = 0; x < salespeople.length; ++x)
{
//Prompt the user to enter an ID number
id = Integer.parseInt(JOptionPane.showInputDialog(null,
"Enter an ID number"));
//Prompt the user to enter the sales value
sales = Double.parseDouble(JOptionPane.showInputDialog(null,
"Enter sales value"));
salespeople[x] = new Salesperson(id, sales);
}
/*Prompt the user to enter the choice of displaying
the objects in order by either ID number or sales value*/
order = JOptionPane.showInputDialog(null,
"By which field do you want to sort?\n" +
"(I)d number or (S)ales");
//If the user enters the choice, ID number
if(order.charAt(0) == 'I')
//Function call
sortById(salespeople);
//Else the user enters the choice, sales value
else
//Function call
sortBySales(salespeople);
//For loop to be executed until x exceeds 7
for(x = 0; x < salespeople...

Trending nowThis is a popular solution!

- This is the question I am stuck on - A. Create a CollegeCourse class. The class contains fields for the course ID (for example, CIS 210), credit hours (for example, 3), and a letter grade (for example, A). Include get and set methods for each field. Create a Student class containing an ID number and an array of five CollegeCourse objects. Create a get and set method for the Student ID number. Also create a get method that returns one of the Student’s CollegeCourses; the method takes an integer argument and returns the CollegeCourse in that position (0 through 4). Next, create a set method that sets the value of one of the Student’s CollegeCourse objects; the method takes two arguments—a CollegeCourse and an integer representing the CollegeCourse’s position (0 through 4). B. Write an application that prompts a professor to enter grades for five different courses each for 10 students. Prompt the professor to enter data for one student at a time, including student ID and course data for…arrow_forwardThis is the question I am stuck on - A. Create a CollegeCourse class. The class contains fields for the course ID (for example, CIS 210), credit hours (for example, 3), and a letter grade (for example, A). Include get and set methods for each field. Create a Student class containing an ID number and an array of five CollegeCourse objects. Create a get and set method for the Student ID number. Also create a get method that returns one of the Student’s CollegeCourses; the method takes an integer argument and returns the CollegeCourse in that position (0 through 4). Next, create a set method that sets the value of one of the Student’s CollegeCourse objects; the method takes two arguments—a CollegeCourse and an integer representing the CollegeCourse’s position (0 through 4). B. Write an application that prompts a professor to enter grades for five different courses each for 10 students. Prompt the professor to enter data for one student at a time, including student ID and course data for…arrow_forwardWrite an application named CollegeList that declares an array of four “regular”CollegeEmployees, three Faculty, and seven Students. Prompt the user tospecify which type of person’s data will be entered (C, F, or S), or allow the userto quit (Q). While the user chooses to continue (that is, does not quit), acceptdata entry for the appropriate type of Person. If the user attempts to enter datafor more than four CollegeEmployees, three Faculty, or seven Students, displayan error message. When the user quits, display a report on the screen listingeach group of Persons under the appropriate heading of “College Employees,”“Faculty,” or “Students.” If the user has not entered data for one or more types ofPersons during a session, display an appropriate message under the appropriateheading. Save the files as Person.java, CollegeEmployee.java, Faculty.java,Student.java, and CollegeList.java.arrow_forward
- Can you make a GUI in java that : 1. (game field) randomizes 9 numbers (form 0 to 9) numbers. randomizes n (rows) and collumns(m) ((from 1 to 4)) The result should be a GUI with a n x m matrix of buttons, each button should be a random number form 1 to 9. The idea is after every compile i should get a random arrangement 2. random target value is displayed above game field(100-300) 3. The current sum of the numbers displayed on the buttons of the game field is printedbelow the game field 4.The number of moves to completion is displayed in the upper right corner above thegame field. 5.current mathematical operation - add a component to the right side of the gamefield that displays the current mathematical operation. The current mathematicaloperation starts at +, after the first move it should randomize. The current mathematical operation only applies for one move, and after the move a new mathematicaloperation is randomly selected that will be evaluated in the next move. Choose fromthe…arrow_forwardThis is the question - The developers of a free online game named Sugar Smash have asked you to develop a class named SugarSmashPlayer that holds data about a single player. The class contains the following fields: idNumber - the player’s ID number (of type int), name - the player's screen name (of type String), scores - an array of integers that stores the highest score achieved in each of 10 game levels Include get and set methods for each field. The get method for scores should require the game level to retrieve the score for. The set method for scores should require two parameters—one that represents the score achieved and one that represents the game level to be retrieved or assigned. Display an error message if the user attempts to assign or retrieve a score from a level that is out of range for the array of scores. Additionally, no level except the first one should be set unless the user has earned at least 100 points at each previous level. If a user tries to set a score for a…arrow_forwardIn main(), prompt the user for two items and create two objects of the ItemToPurchase class. Before prompting for the second item, call scnr.nextLine(); to allow the user to input a new stringarrow_forward
- This is the question that is confusing me - A. Create a CollegeCourse class. The class contains fields for the course ID (for example, CIS 210), credit hours (for example, 3), and a letter grade (for example, A). Include get and set methods for each field. Create a Student class containing an ID number and an array of five CollegeCourse objects. Create a get and set method for the Student ID number. Also create a get method that returns one of the Student’s CollegeCourses; the method takes an integer argument and returns the CollegeCourse in that position (0 through 4). Next, create a set method that sets the value of one of the Student’s CollegeCourse objects; the method takes two arguments—a CollegeCourse and an integer representing the CollegeCourse’s position (0 through 4). B. Write an application that prompts a professor to enter grades for five different courses each for 10 students. Prompt the professor to enter data for one student at a time, including student ID and course…arrow_forwardThis is the question - The developers of a free online game named Sugar Smash have asked you to develop a class named SugarSmashPlayer that holds data about a single player. The class contains the following fields: idNumber - the player’s ID number (of type int) name - the player's screen name (of type String) scores - an array of integers that stores the highest score achieved in each of 10 game levels Include get and set methods for each field. The get method for scores should require the game level to retrieve the score for. The set method for scores should require two parameters—one that represents the score achieved and one that represents the game level to be retrieved or assigned. Display an error message if the user attempts to assign or retrieve a score from a level that is out of range for the array of scores. Additionally, no level except the first one should be set unless the user has earned at least 100 points at each previous level. If a user tries to set a score for a…arrow_forwardThis is the question - The developers of a free online game named Sugar Smash have asked you to develop a class named SugarSmashPlayer that holds data about a single player. The class contains the following fields: idNumber - the player’s ID number (of type int) name - the player's screen name (of type String) scores - an array of integers that stores the highest score achieved in each of 10 game levels Include get and set methods for each field. The get method for scores should require the game level to retrieve the score for. The set method for scores should require two parameters—one that represents the score achieved and one that represents the game level to be retrieved or assigned. Display an error message if the user attempts to assign or retrieve a score from a level that is out of range for the array of scores. Additionally, no level except the first one should be set unless the user has earned at least 100 points at each previous level. If a user tries to set a score for a…arrow_forward
- This is the question - The developers of a free online game named Sugar Smash have asked you to develop a class named SugarSmashPlayer that holds data about a single player. The class contains the following fields: idNumber - the player’s ID number (of type int) name - the player's screen name (of type String) scores - an array of integers that stores the highest score achieved in each of 10 game levels Include get and set methods for each field. The get method for scores should require the game level to retrieve the score for. The set method for scores should require two parameters—one that represents the score achieved and one that represents the game level to be retrieved or assigned. Display an error message if the user attempts to assign or retrieve a score from a level that is out of range for the array of scores. Additionally, no level except the first one should be set unless the user has earned at least 100 points at each previous level. If a user tries to set a score for a…arrow_forwardCreate a class name Taxpayer. Data fields for Taxpayer include yearly gross income and Social Security number (use an int for the type, and do not use dashes within the Social Security number). Methods include a constructor that requires a value for both data fields and two methods that each return one of the data field values. Write an application named USeTaxpayer that declares an array of 10 Taxpayer objects. Set each Social Security number to 999999999 and each gross income to zero. Display the 10 Taxpayer objects. Save the files as TAxpayer.java and USeTAxpayer.javaarrow_forwardCreate a class named Salesperson. Data fields for Salesperson include an integer ID number and a double annual sales amount. Methods include a constructor that requires values for both data fields, as well as get and set methods for each of the data fields. Write an application named DemoSalesperson that declares an array of 10 Salesperson objects. Set each ID number to 9999 and each sales value to zero. Display the 10 Salesperson objects.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
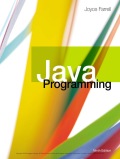
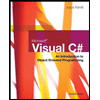
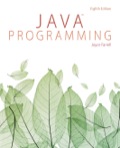