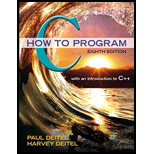
(Recursive Selection Sort) A selection sort searches an array looking for the smallest element in the array. When that element is found, it’s swapped with the first element of the array. The process is then repeated for the subarray, beginning with the second element. Each pass of the array results in one element being placed in its proper location. This sort requires processing capabilities similar to those of the bubble sort—for an array of n elements,

Program Plan-
- Define Header files.
- Define selectionSort()function.
- Define local variables.
- Checking the condition using for() loop till the iteration i is less than 6.
- Display result.
- Checking the condition using if-else() loop.
- Checking the second element with first element.
- Declare main() function.
- Declare local variable.
- Checking the condition using for() loop till the iteration i is less than 6
- Calling selectionSort() function.
- Return successfully to main().
Summary Introduction- (Recursive Selection Sort)A selection sort searches an array looking for the smallest element in the array. When the element is found, it is swapped with the first element of the array. The process is repeated for the sub-array beginning with the second element of the array. Each pass of the array results in one element being placed in its proper location. This sort requires processing capabilities similar to those of bubble sort.
Program Description- The purpose of the program is to implement the recursive selection sort.
Explanation of Solution
Modified program:
/* *This program to implement the recursive selection sort. */ #include<stdio.h> //recursive definition for selcetion sort void selectionSort ( int a[], int size, int i, int j) { //declare local variable int temp; //checking the condtion using if-else loop if(i==size) { //iteration gets executed till value of i is less than 6 for(i=0; i<6; i++) //Displaying result printf("%d ",a[i]); return; //return successfully } else { //checking the condition using if-else() loop if(j<size) { //checking the second element with first //element if(a[i]>a[j]) { temp=a[i]; a[i]=a[j]; a[j]=temp; } //function call //calling function with parameters selectionSort(a,size,i,j+1); } else { //function call selectionSort(a,size,i+1,i+1); } } } //Define main() function int main(int argc, char *argv[]) { //Declare local variables int arr[6],i,min,j,temp; printf("Enter the elements of array\n"); //Asking user to enter elements //Executing for() loop for (i=0; i<6; i++) { printf("Element %d: ",i+1); scanf("%d",&arr[i]); } //sorting the value selectionSort(arr,6,0,1); return 0; //return successfully }
Sample Output-
Sample Output:
Enter the element of array
Element 1:37
Element 2:2
Element 3:6
Element 4:4
Element 5:89
Element 6:8
2 4 6 8 37 89
Want to see more full solutions like this?
Chapter D Solutions
C How To Program Plus Mylab Programming With Pearson Etext -- Access Card Package (8th Edition)
Additional Engineering Textbook Solutions
Starting out with Visual C# (4th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Software Engineering (10th Edition)
Starting Out with Python (4th Edition)
Java How To Program (Early Objects)
- The following recursive function returns the index of the minimum value of an array. Assume variable n is the size of the array. Modify the code so only a single recursive call is made when the second "if" statement is true.arrow_forwardArtificial Intelligence (Part - 1) ==================== The Towers of Hanoi is a famous problem for studying recursion in computer science and searching in artificial intelligence. We start with N discs of varying sizes on a peg (stacked in order according to size), and two empty pegs. We are allowed to move a disc from one peg to another, but we are never allowed to move a larger disc on top of a smaller disc. The goal is to move all the discs to the rightmost peg (see figure). To solve the problem by using search methods, we need first formulate the problem. Supposing there are K pegs and N disk. (1) Propose a state representation for the problem?arrow_forward1. The sorted values array contains 16 integers 5, 7, 10, 13, 13, 20, 21, 25, 30,32, 40, 45, 50, 52, 57, 60. Indicate the sequence of recursive calls that are made tobinaraySearch, given an initial invocation of binarySearch(32, 0, 15).show only the recursive calls. For example, initial invocation is binarySearch(45,0,15)where the target is 45, first is 0 and last is 15.arrow_forward
- There is a close association between pointers and arrays. Recall that an array variable is actually a pointer variable that points to the first indexed variable of the array. Array elements can be accessed using pointer notation as well as array notion. One problem with static arrays is that we must specify the size of the array when we write the program. This may cause two different problems: (1) we may create an array much larger than needed; or (2) we may create one that is smaller than what is needed. In general, this problem is created because we do not know the size of the array until the program is run. This is where dynamic arrays can be used. The new expression can be used to allocate an array on the freestore. Since array variables are pointer variables, you can use the new operator to create dynamic variables that are arrays and treat these dynamic array variables as if they were ordinary arrays. Array elements can also be accessed using pointer notation as well as array…arrow_forwardThere is a close association between pointers and arrays. Recall that an array variable is actually a pointer variable that points to the first indexed variable of the array. Array elements can be accessed using pointer notation as well as array notion. One problem with static arrays is that we must specify the size of the array when we write the program. This may cause two different problems: (1) we may create an array much larger than needed; or (2) we may create one that is smaller than what is needed. In general, this problem is created because we do not know the size of the array until the program is run. This is where dynamic arrays can be used. The new expression can be used to allocate an array on the freestore. Since array variables are pointer variables, you can use the new operator to create dynamic variables that are arrays and treat these dynamic array variables as if they were ordinary arrays. Array elements can also be accessed using pointer notation as well as array…arrow_forwardPointer arithmetic. Implement the following:a. Implement a print function with array and size parameters. This function should print the array using pointer arithmetic.b. Overload the print function to accept array, size and index parameters. If the index is between 1 and size-2 inclusive: Use pointer arithmetic to print: 1. the value at index 2. the value previous to the index 3. the value after the index c. Implement a sum function with array and size parameters. This function should return sum of the array, and use pointer arithmetic.d. Implement a average function with array and size parameters. This function shouldreturn average of the array, and use pointer arithmetic.e. Use provided main function to test your functions. ( Do not change the int main the question has provided. Included a snip of the int main function. Output has to be the same as shown in the snips)arrow_forward
- The following function recursively locates the index of the target value in the array passed in as numbers, returning -1 if the target is not found. Some parts have been removed. fill in the missing code. def find_val(target, numbers, start_index=0): if : _______#base cases return ______ elif :_____ return _____ else:______ #recursive case return ______arrow_forwardWrite a recursive function to sort an array of integers into ascending order using the following idea: the function must place the smallest element in the first position, then sort the rest of the array by a recursive call. This is a recursive version of the selection sort. (Note: You will probably want to call an auxiliary function that finds the index of the smallest item in the array. Make sure that the sorting function itself is recursive. Any auxiliary function that you use may be either recursive or iterative.) Embed your sort function in a driver program to test it. Turn in the entire program and the output.arrow_forward1. Rewrite the Bubble sort to use recursion. 2. Use the time(0) function to determine how many seconds it takes to sort a vector using the recursive method. 3. Use the time(0) function to determine how many seconds it takes to sort a vector using the non-recursive method described in the videos. Run the sort test on vectors containing random integers. You should sort vectors of the following sizes: 100 elements, 1000 elements, 5,000 elements, 10,000 elements and 50,000 elements. Was the recursive method able to work on vectors of each of those sizes? If not, explain why it errored. 4. Create a class that will store a vector of planets (See the Planet assignment). Your class will have functions to add a planet, delete a planet and sort the planets. The class must utilize the insertion sort.arrow_forward
- State whether the statement are true or false. If the answer is false, explain why? " If there are fewer initializers in an initializer list than the number of elements in the array , the remaining elements are initialized to the last value in the initializer list.arrow_forward5. Using c++, Write a function that determines the index of the second to the last occurrence of a target b in an integer array a. Return -1 if b is not in a or there is only 1 occurrence of b in a.arrow_forwardFinish the swap function below using pointers and write the call to swap in the reverse function using the address of the array positions array[i] and array[size-i-1].using pointers. DON'T FORGET TO DECLARE THE PARAMETERS in the function. (Hint: if you can't figure it out with pointers, do it with the references #include <iostream>using namespace std; // Function to swap two ints using pointers// @param pointer to an int// @param pointer to an intvoid swap( ) {// TODO: Add code that swaps two integers using pointers } // Function to reverse arrayvoid reverse(int a[], int size) {for (int i = 0; i < size/2; i++) {// TODO: write the call to swap in the reverse function using the // address of the array positions array[i] and array[size-i-1].arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
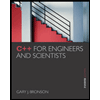