What is meant by guessing the output?
In computer programming languages, guessing the output means to predict the result of a given computer program. It can be predicted by solving the program manually or using trace tables.
By guessing the output, a programmer can understand the algorithm and functioning of the code snippet in a better way. Programmers can determine errors in the code by predicting the output. It also helps in testing an individual’s knowledge of a particular language.
Trace tables
A trace table is an approach used to test an algorithm and predict the stepwise output of the program. In other words, trace tables are used to trace the step-by-step execution of the program to guess the output. Programmers also use trace tables to identify logical errors present in a code.
Consider the following example:
Algorithm 1:
n = 3
print n
for i from 1 to 3
n = n + 5
print n
print “?”
The trace table for the above code will be as follows:
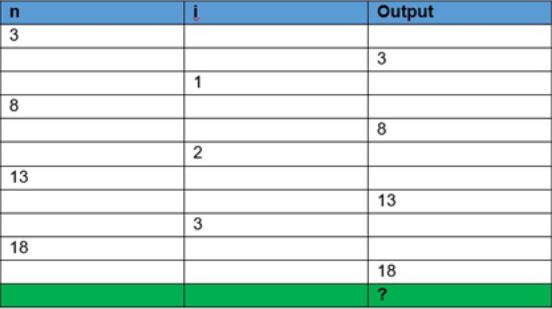
Explanation:
Here, n is initialized to the value 3. So, when the code is executed, the value 3 is printed. Then, the for loop is executed for values i = 1 to 3. The loop begins at i = 1. This line will give the output 3 and as the algorithm proceeds, the value of n will increment to n+5 = 3+5 = 8. So the output will be 8 when the loop is executed for the first time. For the second time, the value of i = 2, n = 8. Therefore, when the loop is executed, the value of n will now be 8+5 = 13. This will print 13 as the output. Again, the for loop will execute with values i = 3, n = 13+5 = 18. This will print the output 13. Finally, the value of i = 4, so, the for loop will terminate and the output “?” will be printed after the last line is executed.
Manually running
Another technique to guess the output for a particular program is to manually run the program. In this approach, the output is predicted by manually tracking the execution of each line of code.
For instance, consider the code snippet below:
sum = 0
for i in range (12, 2, -2);
sum +=1
print sum
Predicting the output:
The syntax of the range () method in Python is range (start, stop, step).
In the above code, the for loop begins at 12 and ends at 2. Each step decrements at -2.
So, by manually testing the code, the output will be:
Sum = 12+10+8+6+4 = 40. (2 is not included)
Tracing the output of Java programs
Since different programming languages follow a different syntax, it is very important to understand the basics of the desired programming language to guess the output of the program written in that language. Some programs related to the Java programming language along with the manual tracing of their outputs are mentioned below.
Example 1:
public class A {
public static void main(String[] args) {
if (true)
break;
}
}
Tracing the output:
- The above program begins by declaring a class A.
- In the main section, the conditional statement if checks if the condition is true.
- If it is true, the code block under if is executed.
- In this case, the if code block consists of the break statement only. As soon as the program encounters this statement, it will return an error because the break statement can only execute with a loop or switch.
- Therefore, this program will generate an error.
Example 2:
public class X{
public static void main(String[] arr){
Integer num1 = 100;
Integer num2 = 100;
Integer num3 = 500;
Integer num4 = 500;
if(num1==num2){
System.out.println("num1 == num2");
}
else{
System.out.println("num1 == num2");
}
if(num3 == num4){
System.out.println("num3 == num4");
}
else{
System.out.println("num3 != num4");
}
}
}
Tracing the output:
- In this Java program, when the program is executed, the third line assigns the value 100 to num1.
- In the next line, num2 is assigned 100, in the fifth line num3 is assigned 500, and in the sixth line, num4 is assigned 500.
- Then, the if condition (num1==num2) is tested. That means two object references num1 and num2 are compared using the == sign and due to integer caching the result of this condition will be true. (IntegerCache is a class in Java that helps in checking object reference values. If the values of the object range between -128 to 127, the object reference for both values will be considered the same.) The same concept applies here. For the value 100, both num1 and num2 give the same reference.
- So the output printed will be num1 == num2.
- The else condition is then skipped.
- Next, the second if condition (num3 == num4) is tested. This condition is false since the value 500 does not fall in the range of -128 to 127. Consequently, num3 and num4 will give different references.
- Therefore, the computer will execute the else part and the output printed will be num3 != num4.
Example 3:
public class C
{
public static void main(String[] args)
{
for (int i = 3; i < 7; i++)
System.out.print("#");
}
}
Tracing the output:
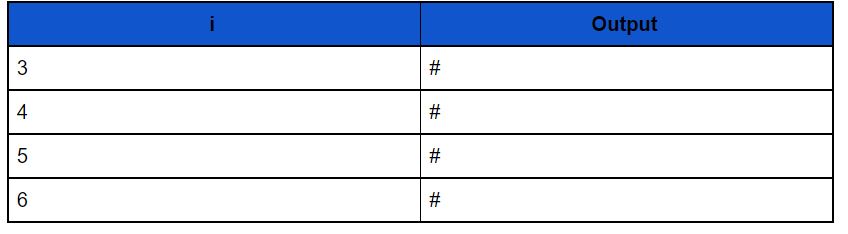
Explanation:
- Here, the value of i is 3 when the program starts executing. So, the for loop checks the condition i < 7. Since 3 is less than 7, the value of i is incremented by 1. So it will be 4 and the for loop is executed to print the output “#”.
- Again, the value of i = 4 which is less than 7, so another “#” is printed. The value of i will then be incremented by 1, so i = 5.
- For i = 5, the for loop will be executed to print another “#” because 5 is less than 7. The value of i will now be 6 after incrementing it by 1.
- Then, for i = 6, which is less than 7, the for loop will be executed. It will again print “#”. Now the value of i will be 7.
- The for loop will now check the condition i<7. Here, it will be 7 < 7, which is false. So, the for loop will terminate.
Output:
Therefore, the final output will be: ####
Context and Applications
Computer programming content is included in all subjects and courses related to computer science. Some of them are listed below:
- Bachelors in Science (Information Technology)
- Bachelors in Science (Computer Science)
- Bachelors in Engineering (Computer Science)
- Masters in Science (Information Technology)
- Masters in Science (Computer Science)
- Masters in Engineering (Computer Science)
Practice Problems
- In the Java program mentioned below, what will be the output?
public class Testing {
public static void main(String args[]){
System.out.print("Z" + "Y" + 'X');
}
}
- Error
- ZY
- ZY12
- ZYX
Answer: Option d
Explanation: When you concatenate any data type (integer, float, character) with a string, the output will be a string. In this code snippet, the character "X" is concatenated with the characters “Z" and "Y”. So, the output will be “ZYX”.
2. What will happen if the following code is compiled?
package com.alex.java2blog;
public class NullCheckMain {
public static void main(String[] args) {
integer i = new integer(null);
String s = new String(null);
}
}
- It will give a runtime error.
- It will give a compile-time error.
- It will return 0.
- It will run perfectly.
Answer: Option b
Explanation: In this code, the String constructor is being assigned a null value. So, the sixth line will generate a compilation error since the string will not be able to recognize, which constructor to call.
3. What will be the correct output of the following Java coding snippet?
public class B {
public static void main(String args[]){
System.out.print('A' + 'B');
}
}
- AB
- 205
- 131
- Runtime error
Answer: Option c
Explanation: In the above program, ‘A’ and ‘B’ are considered as characters and not strings. So, they cannot be concatenated. Hence, the result obtained will be the sum of the Unicode of ‘A’ and ‘B’. The Unicode of ‘A’ is 65 and ‘B’ is 66. So, the output will be 131.
4. What will be the output of the following Java program?
public class A{
public static void main(String[] arr){
}
public static void main(String arr){
}
}
- Infinite error
- Nothing
- Printf Hello
- Binary digits
Answer: Option b
Explanation: Like other methods in Java, the main() method can also be overloaded. However, the Java Virtual Machine will always call the main() method that has String[] argument. So, the above code will return nothing.
5. What will be the output of the following Java code snippet?
public class A {
public static void main(String[] args) {
System.out.print("Hello");
System.out.println("Guys!");
}
}
- HelloGuys!
- Hello Guys!
- Iteration of Hello
- Compile with a warning message
Answer: Option a
Explanation: The System.out.print() function does not print a new line after printing a string. However, the System.out.println() prints a newline after printing a string. Therefore, the above code will return HelloGuys! as the output and a new line will be printed after the statement.
Common Mistakes
Every programming language has different syntaxes and follows a specific set of rules. Students should prepare notes to understand the basics of computer programming languages and remember the rules while guessing the output or executing a program.
Related Concepts
- Basics of C language
- Python programming
- Web programming
- Fundamentals of java programming
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Guessing the output Homework Questions from Fellow Students
Browse our recently answered Guessing the output homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.