What is race condition?
A race condition occurs within a critical section when two or more tasks try to access and alter a shared resource simultaneously. As a consequence, the process changes to a state of inconsistency.
A race condition is a type of semantic mistake that happens when a fault in the timing or order of events causes a program to misbehave. In a logic circuit that processes boolean values, race circumstances can occur. Multithreaded applications that operate in an unpredictable sequence can also cause race circumstances. In multithreaded programs, race conditions are a common source of worry.
A fan switch is a simple example. A single ceiling fan can be controlled by many switches. The switch position is no longer important when these circuits are used. To turn off a fan that has been switched on, either switch must be moved from its current position. If the fan is turned off, moving any switch from its present state will turn it on. Consider what would happen if two people used two distinct switches to turn on the fan at the same moment. One order may cancel the other, or the circuit breaker may trip as a result of the two actions.
Critical Section
The shared variables in a multithreaded program can be accessed by using a code block called a critical section. Concurrent access to shared resources may cause erroneous simultaneous programming behavior. Because of the sections of the program that access the shared resource, it must be safeguarded in a way that prevents concurrent access. This protected area is the critical portion or critical region that is causing data to change in a contradictory manner.
What causes it to happen?
If requests to read and write large amounts of data occur almost concurrently then a race condition can occur in computer storage. While part of the old data is still being read, the computer tries to overwrite some or all of it. A race condition happens when a shared variable is accessed by two threads simultaneously. Before the second thread overwrites the old data, the first thread reads it. If the program is executed in the wrong order, a race condition might develop. When two or more inputs result in a logic gate clash, a race condition can arise.
Types of race condition
- General race
- Data race
General race - programs that are designed in a deterministic manner but behave in a nondeterministic manner are called general races. They are tough to track as they require analysis of the entire execution to understand how the deterministic behavior deviated.
Data race - Failure to access the critical section for the value causes data race. They are comparatively easy to debug. Any race which is not a data race is known as general race.
Race condition example
Consider the following piece of code in a multithreaded environment.
for(k=0;k<10000000;k++)
{a=a+1}
The code is executed in a multithreaded environment, and when six threads run this code at once, the value of a will not finish being 60,000,000. This is because of the variation in the order of execution of the threads. The following steps must be taken by each line to increase the value of a.
- At first, it retrieves the value of a
- To this value, add 1
- Save this value to a variable.
Here, a is the shared resource. During the execution of the six threads, the status of the variable is altered. During the period when a is read and when it is written back, another thread can change the state of the shared variable. This situation is called a race condition.
How to prevent race condition?
There are two techniques for programmers to avoid race problems in operating systems.
Avoid shared states
This is very useful for analyzing code. If a system uses shared resources, it assures that atomic actions that operate independently of other processes are available. Locking is also used to ensure that key code components operate atomically. It's also possible to employ immutable objects, which can't be modified once they've been created.
Use thread synchronization
Thread synchronization in important parts can help prevent race situations. Thread synchronization can be done in a variety of methods. One method is using a synchronized block of java code or the use of the synchronization method. The synchronization constructs used for achieving thread synchronization are locks or atomic variables. The methods used to address the race condition are of two types.
- Check and act
- Read-modify-update
Networking
A race condition occurs when two members need to open the same channel at the same moment. Before the software allows authorization, neither the machine nor the software receives information that such a channel is utilized. To avoid a race situation, a priority system that gives exclusive access to one user must be designed. When two members try to use the system within a certain amount of time. The member whose login or phone number starts with a lower case letter or a lower number may therefore be granted precedence.
Storage and memory
Race situations can also be avoided by serializing memory or storage access. If users send read and write commands at the same time, the read instruction will be performed and finished first.
Deadlock and Race condition
Deadlock
A deadlock occurs when a group of processes is stalled because each process has a resource it is holding and is waiting for the next process to relinquish it, but that process is not ready to do so since it is waiting for the first process's resource. Both processes are stuck in limbo, waiting for the other to finish. When many activities must utilize a mutually exclusive resource called a soft lock or software, deadlock is a regular phenomenon in multi-processing.
It is a situation that occurs one process P1 demands the resource R1 and when process P2 demands R2 also when P1 demands resource R2 and P2 demands R1.
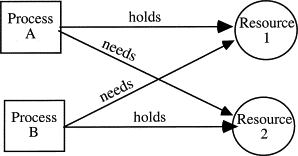
When two or more threads are waiting for an endless length of time for each other to release a resource, a deadlock can result. In addition, the threads are stuck and not executing.
Race condition
A race condition can occur when two or more threads run in parallel but end up giving a result that is wrong and not equivalent if all the operations are done in sequential order. So, deadlock and race conditions are different.
Common Mistakes
People frequently mix up general races and data races, yet race conditions and data races are technically distinct. Many people believe they are the same, yet they are not. A data race happens when two or more threads access a shared variable simultaneously moment. Attempts to alter the variable are made by at least one thread. The data race may be caused by a race condition, but the two are not the same.
Related Topics
- Multi Threading
- Deadlock
- Logic Circuit
- Critical Section
Context and Applications
The content is very useful in the graduation, post-graduation, and competitive exam as well. Especially for-
- Bachelor of technology in Computer Science
- Bachelor of technology in Information Technology
- Master in Computer Science
Practice Problems
Q1. What is race condition?
- When many processes access and modify the same data simultaneously
- A scenario in which a single process accesses and manipulates the same data at the same time.
- A condition in which at no point can two processes access and alter the same data.
- None of the preceding
Correct Ans- 1. When many processes access and modify the same data simultaneously
Explanation: A race condition has occurred in a parallel or multithreaded environment where a shared variable is used. When the ordering or timing of the events is changed unexpected manner, a race condition will occur.
Q2. How can we prevent race condition?
- Proper program command to execute command
- Proper thread synchronization
- Accessing application at the same time
- delaying process execution
Correct Ans- 2. Proper thread synchronization
Explanation- Thread synchronization methods and variables are used for preventing the race condition. The method used in synchronization for race conditions is the use of the lock.
Q3. What is mutual exclusion?
- when two or more processes share the same resource but cannot access the same resource at the same time
- when two or more processes execute the same resource but cannot access the same resource at the same time
- when two or more processes share the same resource but cannot execute the same resource at the same time
- none of the above
Correct Ans- 1. when two or more processes share the same resource but cannot access the same resource at the same time.
Explanation- Mutual exclusion is a technique for preventing racial discrimination. It is a concurrency control characteristic.
Q4. A deadlock occurs when which of the following hold simultaneously?
- Mutual Exclusion
- Hold and wait
- No preemption
- All of the above
Correct Ans- 4. All of the above
Explanation- A deadlock is a situation in the operating system. If a process is in the waiting state and cannot change its state because some other waiting process holds the resources required, the system is said to be a deadlock. There are four conditions necessary for a deadlock namely mutual exclusion, no pre-emption, hold and wait, and circular wait.
Q5. Which one of the below is the program section where the process can modify tables, change common variables, and write to files?
- Data
- Data race
- Instructions
- Critical section
Correct Ans- 4 Critical section
Explanation- A critical section is a code block used in a program. It is used for accessing the shared variables. At any given time, only one process can access the shared variables.
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Race Condition Homework Questions from Fellow Students
Browse our recently answered Race Condition homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.