Week 8 Discussion
.docx
keyboard_arrow_up
School
University of Maryland Global Campus (UMGC) *
*We aren’t endorsed by this school
Course
617
Subject
Biology
Date
Apr 3, 2024
Type
docx
Pages
3
Uploaded by DeanGullMaster970
Write a program that uses a regular expression to extracts out and prints to the screen the accession number of a sequence in a FASTA file.
So for instance if the sequence file contains:
>AF145348.1 Glycine max peroxidase (Prx2b) mRNA, complete cds
ATATTTTATTTGCACTACTACTACTACTTTATTTAAGCAGAAGCAGGCGAGTGAGG
AAGAGCGAAGAGTG
AAGAGAATGGCTCCCAAGGGTTTAACCTTTCTGGCTGTGTTAATATGCGTTTCAGC
ACTGTCACTGAGTC
CTTCTGTTGCGGGGGAAGGGCAAAATAATGGCCTTGTTATGAACTTCTACAAGGAA
TCATGCCCTCAGGC
TGAAGACATCATCACAGAACAAGTCAAGCTTCTCTACAAGCGCCACAAGAACACT
GCTTTCTCCTGGCTC
AGGAACATCTTCCATGACTGTGCTGTTCAGAGTTGTGATGCTTCACTGTTGCTGGA
CTCCACAAGAAGGA
GCTTGTCTGAGAAGGAAACAGATAGAAGCTTTGGGTTGAGAAATTTCAGGTACAT
TGAGACCATCAAAGA
The output should be: AF145348.1
import re
def extract_accession_number(fasta_file):
with open(fasta_file, 'r') as file:
data = file.read()
match = re.search(r'^>(\S+)', data, re.MULTILINE)
if match:
accession_number = match.group(1)
print(accession_number)
else:
print("No accession number found in the FASTA file.")
fasta_file = "fasta_file.txt"
extract_accession_number(fasta_file)
Write a program that produces the following output when searching for the word RNA in the paragraph below:
Output:
(RNAi) ends at position 49
(dsRNA) ends at position 211
(ssRNAs) ends at position 374
mRNAs. ends at position 431
Search Text (you can assign this to a string in your program):
"Several rapidly developing RNA interference (RNAi)
methodologies hold the promise to selectively inhibit gene expression in
mammals. RNAi is an innate cellular process activated when a
double-stranded RNA (dsRNA) molecule of greater than 19 duplex
nucleotides enters the cell, causing the degradation of not only the
invading dsRNA molecule, but also single-stranded (ssRNAs) RNAs of
identical sequences, including endogenous mRNAs."
text = """Several rapidly developing RNA interference (RNAi)
methodologies hold the promise to selectively inhibit gene expression in
mammals. RNAi is an innate cellular process activated when a
double-stranded RNA (dsRNA) molecule of greater than 19 duplex
nucleotides enters the cell, causing the degradation of not only the
invading dsRNA molecule, but also single-stranded (ssRNAs) RNAs of
identical sequences, including endogenous mRNAs."""
def find_rna_variants(text):
variants = ["RNAi", "dsRNA", "ssRNAs", "mRNAs"]
for variant in variants:
index = text.find(variant)
if index != -1:
end_index = index + len(variant)
print(f"{variant} ends at position {end_index}")
find_rna_variants(text)
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Consider the following coding 71 nucleotide DNA template sequence (It does not contain a translational start):
5’- GTTTCCCCTATGCTTCATCACGAGGGCACTGACATGTGTAAACGAAATTCCAACCTGAGCGGCGT GTTGAG-3’
By in vitro translating the mRNA, you determined that the translated peptide is 15 amino acids long. What is the expected peptide sequence in single letter abbreviations?
arrow_forward
A portion of the sequence from the DNA coding strand of the chick ovalbumin gene is shown. Determine the partial amino acid
sequence of the encoded protein.
CTCAGAGTTCACCATGGGCTCCATCGGTGCAGCAAGCATGGAA-(1104
bp)-TTCTTTGGCAGATGTGTTTCCCCTTAAAAAGAA
Enter the 3-letter abbreviation for each amino acid in sequence, separated with dashes, and no spaces (example: xxx-xxx-XXX-XXX...)
The amino acid sequence is
.1104bp..…........
arrow_forward
The bacterial gene shorty (sh) encodes for a small protein. The DNA sequence of the sh gene is shown below. The ORF is in CAPITAL LETTERS
5’-tataatgggcttaacaATGAGTAAAAGAGGTCCTTTACTCCGGTATCACCAATAGaaatattatttaa-3’ 3’-atattacccgaattgtTACTCATTTTCTCCAGGAAATGAGGCCATAGTGGTTATCtttataataaatt-5’
Answer the following questions:
Q1. Which is the coding strand? Which is the template strand? [10%]
Top-bottom.
Bottom-Top.
Both can be used as either coding or template for this gene.
arrow_forward
The bacterial gene shorty (sh) encodes for a small protein. The DNA sequence of the sh gene is shown below. The ORF is in CAPITAL LETTERS
5’-tataatgggcttaacaATGAGTAAAAGAGGTCCTTTACTCCGGTATCACCAATAGaaatattatttaa-3’ 3’-atattacccgaattgtTACTCATTTTCTCCAGGAAATGAGGCCATAGTGGTTATCtttataataaatt
What is the length in AA’s of the Sh protein? Assume fMet is NOT CLEAVED
[10%]
39 AA
13 AA
12 AA
21 AA
arrow_forward
Using the genetic code table provided below, identify the open reading frame in this mRNA
sequence, and write out the encoded 9 amino acid long peptide sequence:
5'- CGACAUGCCUAAAAUCAUGCCAUGGAGGGGGUAACCUUUU
C
A
G
U
UUU Phe UCU Ser
UUC Phe UCC Ser UAC
UCA Ser UAA
UCG Ser
UAG
UUA Leu
Leu
G
C
CUU Leu
CUC Leu CCC
CUA Leu
CUG Leu
AUU lle
AUC lle
AUA lle
AUG Met ACG
ACU
Thr
ACC
Thr
ACA Thr
Thr
A
UAU Tyr
UGU Cys
Tyr UGC Cys
CCU Pro CAU His CGU Arg
Pro CAC His
Pro CAA Gln
CGC Arg
CGA
Arg
CCA
CCG Pro
CAG Gln CGG
Arg
GUU Val GCU
Ala
GAU
GUC Val GCC
Ala
GAC
GUA Val GCA Ala
GAA
GUG Val GCG Ala GAG
Stop UGA
Stop UGG
AAU Asn
AAC
AAA
AAG
AGU
Asn AGC
G
Lys
Lys
Asp
Asp
Glu
Glu
Stop A
Trp
Ser
Ser
AGA Arg
AGG Arg
GGU Gly
GGC Gly
UCAG
GGA Gly
GGG Gly
с
U
C
A
G
U
C
A
G
U
C
A
G
arrow_forward
Briefly discuss (referring to the images provided) why mutant 2 fails to produce functional protein. Note that none of the mRNA transcribed from this gene is of the expected size; some of the mRNA molecules produced are 223 nucleotides shorter than expected, whilst others are 47 nucleotides longer than expected.
arrow_forward
Using the genetic code table provided below, write out the sequence of three different possible
mRNA sequences that could encode the following sequence of amino acids:
Met-Phe-Cys-Trp-Glu
C
A
G
U
C
UUU Phe UCU Ser
UUC Phe UCC Ser
UCA Ser
UUA Leu
UUG Leu UCG Ser
CUU Leu CCU Pro
CUC Leu
CUA Leu
CUG Leu CCG
A
CAU His CGU Arg
CCC Pro CAC His CGC Arg
UAU Tyr UGU Cys U
UAC Tyr UGC Cys C
Stop UGA Stop
Stop UGG
Trp
UAA
A
UAG
G
CCA Pro CAA Gln
Pro CAG
AUU lle
AUC lle
AUA lle
AUG Met ACG
G
등등
Gln
CGA Arg
CGG Arg
ACU Thr AAU Asn AGU Ser
ACC
AAC Asn
AGC Ser
Thr
ACA Thr
Thr
AAA Lys AGA Arg
AAG
Lys
AGG Arg
GUU Val GCU
Ala
GAU Asp
GGU Gly
GGC Gly
GUC Val GCC
Ala
GAC
Asp
GUA Val
GCA
Ala
GAA
Glu GGA Gly
GUG Val
GCG Ala GAG
Glu GGG Gly
U
C
A
G
U
C
A
G
SUAU
arrow_forward
The amino acids, in one-letter symbols and no spaces, coded by the following mRNA sequence is 5’ AAUGGAACGUCGGUACUGCCAUCGCAUUAGUACCAUGGCAAGCUGAAGC 3’
arrow_forward
Using the genetic code below, decipher the following mRNA sequence. 5'CCCGGGAUGCGGUGUUGGUUUUAACCCGGG3' (show all work)
arrow_forward
c) A gene in a bacteria has the following DNA sequences (the promoter sequence is
positioned to the left but is not shown):
5'-CAATCATGGAATGCCATGCTTCATATGAATAGTTGACAT-3'
3'-GTTAGTACCT TACGGTACGAAGTATACTTATCAACTGTA-5'
i) By referring to the codon table below, write the corresponding mRNA transcript and
polypeptide translated from this DNA strand.
2
Second letter
с
A
UUUPhe
UAU
Tyr
UAC.
UGU
UGCJ
UCU)
UCC
UCA
UUG Leu UCG
Cys
UUC
UUA
Ser
UAA Stop UGA Stop A
UAG Stop UGG Trp G
CUU
CÚC
CCU
ССС
CAU
CGU
His
САC
Pro
CC
CỦA
Leu
ССА
CAA
Arg
CGA
CUG J
CCG)
CAG Gin
CGG
AUU
ACU
AAU
Asn
AGU
Ser
AUC le
АСC
АCА
AAC
AAA
AGC.
Thr
JArg
AUA
AGA
AUG Met ACG
AAG Lys
AGG.
GAU
Asp
GUU)
GCU
GCC
GCA
GCG
GGU"
GGC
GGA
GGG
GUC
Val
GUA
GAC
Ala
Gly
GAA
Glu
GAGJ
GUG
ii) If the nucleotide indicated by the highlighted bold letter undergoes a mutation that
resulted in deletion of the C:G base pair, what will be the resulting amino acid sequence
following transcription and translation?
Third letter
DUAG
DUAG
DUAG
A.
First…
arrow_forward
draw mRNA sequence for the following sequence
ATGGCCCTGTGGATGCGCCTCCTGCCCCTGCTGGCGCTGCTGGCCCTCTGGGGACCTGACCCAGCCGCAGCCTTTGTGAACCAACACCTGTGCGGCTCACACCTGGTGGAAGCTCTCTACCTAGTGTGCGGGGAACGAGGCTTCTTCTACACACCCAAGACCCGCCGGGAGGCAGAGGACCTGCAGGTGGGGCAGGTGGAGCTGGGCGGGGGCCCTGGTGCAGGCAGCCTGCAGCCCTTGGCCCTGGAGGGGTCCCTGCAGAAGCGTGGCATTGTGGAACAATGCTGTACCAGCATCTGCTCCCTCTACCAGCTGGAGAACTACTGCAACTAG
arrow_forward
Write down the corresponding amino acids sequence for each mRNA sequence. Use the codon chart.
arrow_forward
You may wish to consult the genetic code above to answer the following question. A mutation has
changed a portion of a protein coding gene that encodes a messenger RNA sequence.
The original messenger RNA sequence is 5-AUGCCCAGAGCU-3'
Which mutation is a nonsynonymous (missense) mutation that changes a single amino acid in the
encoded protein?
O 5-AUGCCCAGGGCC-3'
O 5'-AUGCCCUGAGCU-3'
O 5'-AUGCCCACAGCU-3
5'-AUGCCCCAGAGCU-3
arrow_forward
For the messenger RNA sequence below, find the beginning of the amino acid coding sequence and translate the sequence using the
genetic code provided below.
5' - AAUUAUGGGCAAUAUGCCGGGCcGGUUAAGCG - 3'
Second Letter
A
UGU cys u
UGC
Phe UCU
UU
U UUC
UUA
UAU
Tyr
Ser UAC
UAA
UAG
Leu
UCA
Stop UGA Stop
UUG
UCG
Stop UGG
Trp
CUU
CU
CAU
His
CGU
c cuc
Leu ccc
ССА
CCG
Pro
CÁC
CAA
CAG
CGC
CGA
CGG
Arg
CUA
CUG
Gin
1st
3rd
letter
Ser u letter
AUU
ACU
AAU
AAC
AAA
AAG
Asn
A AUC
AUA
AUG
lle ACC
ACA
AGU
AGC
AGA
AGG
Thr
Lys
Arg
Met ACG
GUU
G GUC
GUA
GUG
GCU
GAU
GAC
GAA
Asp
GGU
Val
GCC
Ala
GGC
Gly
GCA
GGA
Glu
GCG
GAG
GGG
G
arrow_forward
In the copies of each sequence below, divide the sequences into codons (triplets) by putting a slash between each group of three bases.
Sequence ATCTTCCCTCCTAAACGTTCAACCGGTTCTTAATCCGCCGCCAGGGCCCCGCCCCTCAGAAGTTGGTSequence BTCAGACGTTTTTGCCCCGTAACAACTTGTTACAACATGGTCATAAACGTCAGAGATGGTCAATCTCTTAATGACTSequence CTACAAACATGTAAACACACCCTCAGTGGACCAACTCCGCAACATAAACCAAACACCGCTCGCGCCGAAAAAGATATGG
arrow_forward
A protein has the following amino acid sequence: Met-Tyr-Asn-Val-Arg-Val-Tyr-Lys-Ala-Lys-Trp-Leu-Ile-His-Thr-Pro You wish to make a set of probes to screen a cDNA library for the sequence that encodes this protein. Your probes should be at least 18 nucleotides in length. a. Which amino acids in the protein should be used to construct the probes so that the least degeneracy results? b. How many different probes must be synthesized to be certain that you will find the cDNA sequence that specifies the protein?
arrow_forward
The double stranded DNA sequence shown contains the promoter for the transcription of a
bacterial gene.
GGCACCTGCGATGCATGAATATATCGATCGGGAATCGCTATGTCAAGCCATGGCTAGATTA
CCGTGGACGCTACGTACTTATATAGCTAGCCCTTAGCGATACAGTTCGGTACCGATCTAAT
Draw a box around each of the promoter elements and identify each.
Identify which strand will be used as the template strand by putting a vertical line between the -1/+1 start
site nucleotides and underlining in the direction of transcription on the template strand as the example
below indicates.
ATCGG\GAATCGC
TAGCCCTTAGCG
Give the sequence of the RNA created
arrow_forward
A segment of a polypeptide chain is Arg-Gly-Ser-Phe-Val-Asp-Arg. It is encoded by the following segment of DNA:
GGCTAGCTGCTTCCTTGGGGA
CCGATCGACGAAGGAACCCCT
Note out the mRNA sequence generated by the template strant to produce that polypeptide chain
Label each stran with its correct polarity (5' and 3' ends on each strand)
arrow_forward
The DNA sequence of a short gene from a sea slug, and the mature RNA synthesized from this gene, are shown below.
DNA sequence:
5’ - AGCATCTCATGTGCGAGTCCTGACGCTGACTAGC – 3’
3’ - TCGTAGAGTACACGCTCAGGACTGCGACTGATCG – 5’
mature mRNA:
5’ – cap-AUCUCAUGUGCGAACGCUGACUAGAAAAAAAAAA- 3’
Draw boxes around any structures or bases that were added to the RNA during processing.
arrow_forward
The DNA sequence of a short gene from a sea slug, and the mature RNA synthesized from this gene, are shown below.
DNA sequence:
5’ - AGCATCTCATGTGCGAGTCCTGACGCTGACTAGC – 3’
3’ - TCGTAGAGTACACGCTCAGGACTGCGACTGATCG – 5’
mature mRNA:
5’ – cap-AUCUCAUGUGCGAACGCUGACUAGAAAAAAAAAA- 3’
In the DNA sequence, draw boxes around two exons in the gene. The splicing machinery does NOT pay attention to codons. The splicing of this DNA demonstrates that. How?
arrow_forward
The DNA sequence of a short gene from a sea slug, and the mature RNA synthesized from this gene, are shown below.
DNA sequence:
5’ - AGCATCTCATGTGCGAGTCCTGACGCTGACTAGC – 3’
3’ - TCGTAGAGTACACGCTCAGGACTGCGACTGATCG – 5’
mature mRNA:
5’ – cap-AUCUCAUGUGCGAACGCUGACUAGAAAAAAAAAA- 3’
How many amino acids are in the peptide encoded by the gene? _____________
arrow_forward
Consider the following DNA sequence, which codes for a short polypeptide:
5'-ATGGGCTTAGCGTAGGTTAGT-3'
Determine the mRNA transcript of this sequence. You have to write these sequences from the 5' end to the 3' end and indicate those ends as shown in the original sequence in order to get the full mark.
How many amino acids will make up this polypeptide?
Determine the first four anticodons that will be used in order to translate this sequence.
arrow_forward
Given the DNA sequence below:
5’-ACATGTGTACAGGCTTTGTCTGAATGGCTT-3’
3’-TGTACACATGTCCGAAACAGACTTACCGAA-5’
Transcribe the gene. (Write the primary structure of the mRNA that will be produced.)
arrow_forward
A protein has the following amino acid sequence:
Met-Tyr-Asn-Val-Arg-Val-Tyr-Lys-Ala-Lys-Trp-Leu-Ile-His-Thr-Pro
You wish to make a set of probes to screen a cDNA library for the sequence that encodes this protein. Your probes should be at least 18 nucleotides in length.
Q. Which amino acids in the protein should be used to construct the probes so that the least degeneracy results?
arrow_forward
Please do not copy paste from web I will report if you do it.
arrow_forward
NASA has identified a new microbe present on Mars and requests that you determine the genetic code of this organism. To accomplish this goal, you isolate an extract from this microbe that contains all the components necessary for protein synthesis except mRNA. Synthetic mRNAs are added to this extract and the resulting polypeptides are analyzed:
Synthetic mRNA
Resulting Polypeptides
AAAAAAAAAAAAAAAA
Lysine-Lysine-Lysine etc.
CACACACACACACACA
Threonine-Histidine-Threonine-Histidine etc.
AACAACAACAACAACA
Threonine-Threonine-Threonine etc.
Glutamine-Glutamine-Glutamine etc.
Asparagine-Asparagine-Asparagine etc.
From these data, what specifics can you conclude about the microbe’s genetic code?
What is the sequence of the anticodon loop of a tRNA carrying a threonine?
If you found that this microbe contained 61 different tRNAs, what could you speculate about the fidelity of translation in this organism?
arrow_forward
Write out the sequence of the polypeptide in AA: use the three letter notation, e.g. Met-Ser-Pro-
arrow_forward
How many amino acid are in each of the two polypeptides produced? And how many nucleotides long would the final processed mRNA made from the gene be?
arrow_forward
Please draw the pre-mRNA that would be produced from this gene.Gray nucleotides indicate noncoding sequences. Label template strand:
5’-ACTGTGACCTTGACTGTCGATACGATG-3’
3’-TGACACTGGAACTGACACGTATGCTAC-5’
arrow_forward
Based on sequences A,B,C. Provide an anticodon sequence that would build this protein.
Sequence ATCTTCCCTCCTAAACGTTCAACCGGTTCTTAATCCGCCGCCAGGGCCCCGCCCCTCAGAAGTTGGTSequence BTCAGACGTTTTTGCCCCGTAACAACTTGTTACAACATGGTCATAAACGTCAGAGATGGTCAATCTCTTAATGACTSequence CTACAAACATGTAAACACACCCTCAGTGGACCAACTCCGCAACATAAACCAAACACCGCTCGCGCCGAAAAAGATATGG
arrow_forward
Please help me find the protein sequence in reference to the text below.
"mRNA Sequence Translate the mRNA sequence of HbS.
5'-AUG GUG CAC CUG ACU CCU GUG GAG AAG UCU GCC GUU ACU - 3¹
Protein Sequence 5'-"
arrow_forward
Please find the mRNA codons for the following missing items
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
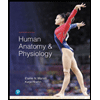
Human Anatomy & Physiology (11th Edition)
Biology
ISBN:9780134580999
Author:Elaine N. Marieb, Katja N. Hoehn
Publisher:PEARSON
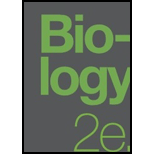
Biology 2e
Biology
ISBN:9781947172517
Author:Matthew Douglas, Jung Choi, Mary Ann Clark
Publisher:OpenStax
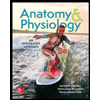
Anatomy & Physiology
Biology
ISBN:9781259398629
Author:McKinley, Michael P., O'loughlin, Valerie Dean, Bidle, Theresa Stouter
Publisher:Mcgraw Hill Education,
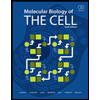
Molecular Biology of the Cell (Sixth Edition)
Biology
ISBN:9780815344322
Author:Bruce Alberts, Alexander D. Johnson, Julian Lewis, David Morgan, Martin Raff, Keith Roberts, Peter Walter
Publisher:W. W. Norton & Company
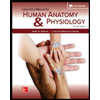
Laboratory Manual For Human Anatomy & Physiology
Biology
ISBN:9781260159363
Author:Martin, Terry R., Prentice-craver, Cynthia
Publisher:McGraw-Hill Publishing Co.
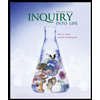
Inquiry Into Life (16th Edition)
Biology
ISBN:9781260231700
Author:Sylvia S. Mader, Michael Windelspecht
Publisher:McGraw Hill Education
Related Questions
- Consider the following coding 71 nucleotide DNA template sequence (It does not contain a translational start): 5’- GTTTCCCCTATGCTTCATCACGAGGGCACTGACATGTGTAAACGAAATTCCAACCTGAGCGGCGT GTTGAG-3’ By in vitro translating the mRNA, you determined that the translated peptide is 15 amino acids long. What is the expected peptide sequence in single letter abbreviations?arrow_forwardA portion of the sequence from the DNA coding strand of the chick ovalbumin gene is shown. Determine the partial amino acid sequence of the encoded protein. CTCAGAGTTCACCATGGGCTCCATCGGTGCAGCAAGCATGGAA-(1104 bp)-TTCTTTGGCAGATGTGTTTCCCCTTAAAAAGAA Enter the 3-letter abbreviation for each amino acid in sequence, separated with dashes, and no spaces (example: xxx-xxx-XXX-XXX...) The amino acid sequence is .1104bp..…........arrow_forwardThe bacterial gene shorty (sh) encodes for a small protein. The DNA sequence of the sh gene is shown below. The ORF is in CAPITAL LETTERS 5’-tataatgggcttaacaATGAGTAAAAGAGGTCCTTTACTCCGGTATCACCAATAGaaatattatttaa-3’ 3’-atattacccgaattgtTACTCATTTTCTCCAGGAAATGAGGCCATAGTGGTTATCtttataataaatt-5’ Answer the following questions: Q1. Which is the coding strand? Which is the template strand? [10%] Top-bottom. Bottom-Top. Both can be used as either coding or template for this gene.arrow_forward
- The bacterial gene shorty (sh) encodes for a small protein. The DNA sequence of the sh gene is shown below. The ORF is in CAPITAL LETTERS 5’-tataatgggcttaacaATGAGTAAAAGAGGTCCTTTACTCCGGTATCACCAATAGaaatattatttaa-3’ 3’-atattacccgaattgtTACTCATTTTCTCCAGGAAATGAGGCCATAGTGGTTATCtttataataaatt What is the length in AA’s of the Sh protein? Assume fMet is NOT CLEAVED [10%] 39 AA 13 AA 12 AA 21 AAarrow_forwardUsing the genetic code table provided below, identify the open reading frame in this mRNA sequence, and write out the encoded 9 amino acid long peptide sequence: 5'- CGACAUGCCUAAAAUCAUGCCAUGGAGGGGGUAACCUUUU C A G U UUU Phe UCU Ser UUC Phe UCC Ser UAC UCA Ser UAA UCG Ser UAG UUA Leu Leu G C CUU Leu CUC Leu CCC CUA Leu CUG Leu AUU lle AUC lle AUA lle AUG Met ACG ACU Thr ACC Thr ACA Thr Thr A UAU Tyr UGU Cys Tyr UGC Cys CCU Pro CAU His CGU Arg Pro CAC His Pro CAA Gln CGC Arg CGA Arg CCA CCG Pro CAG Gln CGG Arg GUU Val GCU Ala GAU GUC Val GCC Ala GAC GUA Val GCA Ala GAA GUG Val GCG Ala GAG Stop UGA Stop UGG AAU Asn AAC AAA AAG AGU Asn AGC G Lys Lys Asp Asp Glu Glu Stop A Trp Ser Ser AGA Arg AGG Arg GGU Gly GGC Gly UCAG GGA Gly GGG Gly с U C A G U C A G U C A Garrow_forwardBriefly discuss (referring to the images provided) why mutant 2 fails to produce functional protein. Note that none of the mRNA transcribed from this gene is of the expected size; some of the mRNA molecules produced are 223 nucleotides shorter than expected, whilst others are 47 nucleotides longer than expected.arrow_forward
- Using the genetic code table provided below, write out the sequence of three different possible mRNA sequences that could encode the following sequence of amino acids: Met-Phe-Cys-Trp-Glu C A G U C UUU Phe UCU Ser UUC Phe UCC Ser UCA Ser UUA Leu UUG Leu UCG Ser CUU Leu CCU Pro CUC Leu CUA Leu CUG Leu CCG A CAU His CGU Arg CCC Pro CAC His CGC Arg UAU Tyr UGU Cys U UAC Tyr UGC Cys C Stop UGA Stop Stop UGG Trp UAA A UAG G CCA Pro CAA Gln Pro CAG AUU lle AUC lle AUA lle AUG Met ACG G 등등 Gln CGA Arg CGG Arg ACU Thr AAU Asn AGU Ser ACC AAC Asn AGC Ser Thr ACA Thr Thr AAA Lys AGA Arg AAG Lys AGG Arg GUU Val GCU Ala GAU Asp GGU Gly GGC Gly GUC Val GCC Ala GAC Asp GUA Val GCA Ala GAA Glu GGA Gly GUG Val GCG Ala GAG Glu GGG Gly U C A G U C A G SUAUarrow_forwardThe amino acids, in one-letter symbols and no spaces, coded by the following mRNA sequence is 5’ AAUGGAACGUCGGUACUGCCAUCGCAUUAGUACCAUGGCAAGCUGAAGC 3’arrow_forwardUsing the genetic code below, decipher the following mRNA sequence. 5'CCCGGGAUGCGGUGUUGGUUUUAACCCGGG3' (show all work)arrow_forward
- c) A gene in a bacteria has the following DNA sequences (the promoter sequence is positioned to the left but is not shown): 5'-CAATCATGGAATGCCATGCTTCATATGAATAGTTGACAT-3' 3'-GTTAGTACCT TACGGTACGAAGTATACTTATCAACTGTA-5' i) By referring to the codon table below, write the corresponding mRNA transcript and polypeptide translated from this DNA strand. 2 Second letter с A UUUPhe UAU Tyr UAC. UGU UGCJ UCU) UCC UCA UUG Leu UCG Cys UUC UUA Ser UAA Stop UGA Stop A UAG Stop UGG Trp G CUU CÚC CCU ССС CAU CGU His САC Pro CC CỦA Leu ССА CAA Arg CGA CUG J CCG) CAG Gin CGG AUU ACU AAU Asn AGU Ser AUC le АСC АCА AAC AAA AGC. Thr JArg AUA AGA AUG Met ACG AAG Lys AGG. GAU Asp GUU) GCU GCC GCA GCG GGU" GGC GGA GGG GUC Val GUA GAC Ala Gly GAA Glu GAGJ GUG ii) If the nucleotide indicated by the highlighted bold letter undergoes a mutation that resulted in deletion of the C:G base pair, what will be the resulting amino acid sequence following transcription and translation? Third letter DUAG DUAG DUAG A. First…arrow_forwarddraw mRNA sequence for the following sequence ATGGCCCTGTGGATGCGCCTCCTGCCCCTGCTGGCGCTGCTGGCCCTCTGGGGACCTGACCCAGCCGCAGCCTTTGTGAACCAACACCTGTGCGGCTCACACCTGGTGGAAGCTCTCTACCTAGTGTGCGGGGAACGAGGCTTCTTCTACACACCCAAGACCCGCCGGGAGGCAGAGGACCTGCAGGTGGGGCAGGTGGAGCTGGGCGGGGGCCCTGGTGCAGGCAGCCTGCAGCCCTTGGCCCTGGAGGGGTCCCTGCAGAAGCGTGGCATTGTGGAACAATGCTGTACCAGCATCTGCTCCCTCTACCAGCTGGAGAACTACTGCAACTAGarrow_forwardWrite down the corresponding amino acids sequence for each mRNA sequence. Use the codon chart.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Human Anatomy & Physiology (11th Edition)BiologyISBN:9780134580999Author:Elaine N. Marieb, Katja N. HoehnPublisher:PEARSONBiology 2eBiologyISBN:9781947172517Author:Matthew Douglas, Jung Choi, Mary Ann ClarkPublisher:OpenStaxAnatomy & PhysiologyBiologyISBN:9781259398629Author:McKinley, Michael P., O'loughlin, Valerie Dean, Bidle, Theresa StouterPublisher:Mcgraw Hill Education,
- Molecular Biology of the Cell (Sixth Edition)BiologyISBN:9780815344322Author:Bruce Alberts, Alexander D. Johnson, Julian Lewis, David Morgan, Martin Raff, Keith Roberts, Peter WalterPublisher:W. W. Norton & CompanyLaboratory Manual For Human Anatomy & PhysiologyBiologyISBN:9781260159363Author:Martin, Terry R., Prentice-craver, CynthiaPublisher:McGraw-Hill Publishing Co.Inquiry Into Life (16th Edition)BiologyISBN:9781260231700Author:Sylvia S. Mader, Michael WindelspechtPublisher:McGraw Hill Education
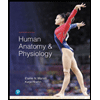
Human Anatomy & Physiology (11th Edition)
Biology
ISBN:9780134580999
Author:Elaine N. Marieb, Katja N. Hoehn
Publisher:PEARSON
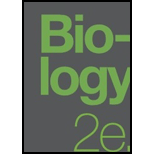
Biology 2e
Biology
ISBN:9781947172517
Author:Matthew Douglas, Jung Choi, Mary Ann Clark
Publisher:OpenStax
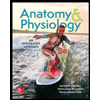
Anatomy & Physiology
Biology
ISBN:9781259398629
Author:McKinley, Michael P., O'loughlin, Valerie Dean, Bidle, Theresa Stouter
Publisher:Mcgraw Hill Education,
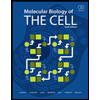
Molecular Biology of the Cell (Sixth Edition)
Biology
ISBN:9780815344322
Author:Bruce Alberts, Alexander D. Johnson, Julian Lewis, David Morgan, Martin Raff, Keith Roberts, Peter Walter
Publisher:W. W. Norton & Company
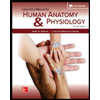
Laboratory Manual For Human Anatomy & Physiology
Biology
ISBN:9781260159363
Author:Martin, Terry R., Prentice-craver, Cynthia
Publisher:McGraw-Hill Publishing Co.
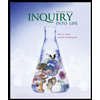
Inquiry Into Life (16th Edition)
Biology
ISBN:9781260231700
Author:Sylvia S. Mader, Michael Windelspecht
Publisher:McGraw Hill Education