cs180_hw2-final
pdf
keyboard_arrow_up
School
University of California, Los Angeles *
*We aren’t endorsed by this school
Course
180
Subject
Computer Science
Date
Oct 30, 2023
Type
Pages
7
Uploaded by GrandLeopardPerson487
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
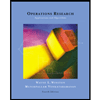
Operations Research : Applications and Algorithms
Computer Science
ISBN:9780534380588
Author:Wayne L. Winston
Publisher:Brooks Cole
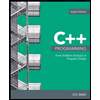
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
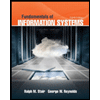
Fundamentals of Information Systems
Computer Science
ISBN:9781305082168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
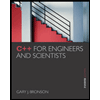
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Recommended textbooks for you
- Operations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks ColeC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningFundamentals of Information SystemsComputer ScienceISBN:9781305082168Author:Ralph Stair, George ReynoldsPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
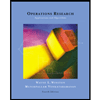
Operations Research : Applications and Algorithms
Computer Science
ISBN:9780534380588
Author:Wayne L. Winston
Publisher:Brooks Cole
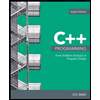
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
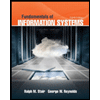
Fundamentals of Information Systems
Computer Science
ISBN:9781305082168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
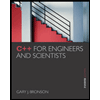
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Browse Popular Homework Q&A
Q: A sheaf of papers in his hand, your friend and colleague, Jason, steps into your office and asked…
Q: (1) in whether lowering the price will yield
higher revenues, and (2) in an estimate of the maximum…
Q: Jennifer Crum has an exercise regimenat the local gym that includes using a treadmill, stair…
Q: 800 9001000
min = Q₁ = Q₂ Q3
(b) Approximately what percenta
Approximately%
(c) Approximately what…
Q: The incomplete ques
Q: The graph of f(x) is shown.
वि
|
45 6
7 X
fl=o at x = ?
DIY'S_
Q: Questions
1. What are two advantages of online ordering in a fast-food restaurant such as Domino's…
Q: A horizontal rifle is fired at a bull's-eye. The muzzle speed of the bullet is 650 m/s. The gun is…
Q: Ginny currently earns a (a. nominal; b. real) wage of $12.00 per hour; in other words, the…
Q: Match the graph to the correct exponential functions. Select all that apply.
-10
-6
□y=-2(3)^…
Q: A row of long cylindrical power cables that are spaced 2 cm evenly apart is placed in parallel with…
Q: Required information
Use the following information for the Quick Studies below. (Algo)
Rafner…
Q: QUESTION 1
You and 3 friends go bowling. You bowl for 2.5 hours. You play 3 games and the total cost…
Q: 1.12 Choose the equation below that can be used to determine the value of "P" for a known
interest…
Q: The first part of the assignment is to open Excel and in column A starting in row 1 and down to row…
Q: Which type of performance evaluation you thinks works best, a criteria based or a competency based…
Q: Below are listed molecules with different chemical characteristics. Knowing that all molecules will…
Q: An ampule of naphthalene in hexane contains 1.00×10-4 naphthalene. The naphthalene is excited with a…
Q: this was completely wrong, and it took me more than hour
Q: Br
H3C CH3
a
b
с
ionization
d
1-propanol
e
Prelab Question #4
Homework Unanswered
H
H
8+
With…
Q: (a) State the null hypothesis H and the alternate hypothesis H₁.
H₁:0
H₁:0
(b) Determine the type of…
Q: O ATOMS, IONS AND MOLECULES
Counting protons and electrons in atoms and atomic ions
Fill in the…
Q: Evaluate
√ √R 6xy5 dydx
R
where the region is bounded by the curves y =
= x²
1 and y = 1-².
Sketch…
Q: A travel magazine conducts an annual survey where readers rate their favorite cruise ship. Ships are…
Q: MEAN=50 STANDARD DEVIATION =7
P(3440)=
*Round to 4 decimal as needed*