Lab9
.docx
keyboard_arrow_up
School
Georgia Southern University *
*We aren’t endorsed by this school
Course
1301
Subject
Computer Science
Date
Dec 6, 2023
Type
docx
Pages
2
Uploaded by BaronBoulderSeahorse44
CSCI 1301 Programming Principles Lab 9
Due date: November 3, 2023 (Friday), 5:00pm
2D Array of Objects
Create a 2-D array of MyPoint objects.
Design a class named MyPoint to represent a point.
The class contains:
Two double private data fields named x and y that specify the coordinates of a
point.
A pubic no-arg constructor that creates a default point (0, 0).
A pubic constructor that creates a point with specified x and y coordinates.
Two sets of pubic get and set methods for the two private data fields.
Write a test class named Lab9 with a main method to create a 2 by 3 array filled with
points
1
as follows:
(0.5, 1.2)
(0.0, 3.14)
(15.0, 27.5)
(6.6, 7.7)
(1.2, 2.1)
(12.0, 127.0)
In your main method, use a pair of nested for-loops to print the contents of the array. Your
output should appear as below:
Array[0][0] = (0.5, 1.2)
Array[0][1] = (0.0, 3.14)
Array[0][2] = (15.0, 27.5)
Array[1][0] = (6.6, 7.7)
Array[1][1] = (1.2, 2.1)
Array[1][2] = (12.0, 127.0)
Requirements
: (1) Draw the UML of MyPoint class; (2) implement MyPoint class; (3)
implement Lab9 class with a main method
.
Save the two program files as MyPoint.java
and Lab9.java.
Grading:
This lab is worth 100 points.
Out of 100 maximum points, the UML will
count for 20 points, the two source codes will count for 80 points
.
Submission:
For this assignment, please name your class file as “MyPoint”
1
(3.5, 2.7) is a notation that describes a point with x=3.5 and y=2.7.
package
homework;
public
class
MyPoint
{
//Please write your code here
}
Please name your test program as “Lab9”
package
homework;
public
class
Lab9 {
// Main method
public
static
void
main(String[]
args
) {
//Please write your code here
}
}
How to hand in the assignment
Follow the steps below:
1.
Complete the UML in a Microsoft Word document or other document file format,
and the programs in .java files. Once you are done,
convert your UML file to a
PDF (.pdf)
.
2.
Upload the .java files as well as the PDF document UML into Gradescope.
Do
not
submit a .docx file for the UML, first convert your file to PDF (.pdf)
.
3.
Click “Upload”.
I remind you that if you do not submit the assignment before the due date, you will not be
able to submit it.
Note
: Your output should look
exactly
like the output below and should be able to run
test cases.
Array[0][0] = (0.5, 1.2)
Array[0][1] = (0.0, 3.14)
Array[0][2] = (15.0, 27.5)
Array[1][0] = (6.6, 7.7)
Array[1][1] = (1.2, 2.1)
Array[1][2] = (12.0, 127.0)
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Rectangle Object Monitoring
Create a Rectangle class that can compute the total area of all the created rectangle objects using static fields (variables). Remember that a Rectangle has two attributes: Length and Width. Implement the class by creating a computer program that will ask the user about three rectangle dimensions. The program should be able to display the total area of the three rectangle objects. For this exercise, you are required to apply all OOP concepts that you learned in class.
Sample output:
Enter Length R1: 1
Enter Width R1: 1
Enter Length R2: 2
Enter Width R2: 2
Enter Length R3: 3
Enter Width R3: 3
The total area of the rectangles is 14.00
Note: All characters in boldface are user inputs.
arrow_forward
Book Donation App
Create a book-app directory. The app can be used to manage book donations and track donors and books. The catalog is implemented using the following classes:
1. The app should have donors-repo.js to maintain the list of donors and allow adding, updating, and deleting donors. The donor object has donorID, firstName, lastName, and email properties. This module should implement the following functions:
• getDonor(donorId): returns a donor by id.
• addDonor(donor): adds a donor to the list of donors; donorID should be autoassigned a random number.
• updateDonor(donor): updates the donor having the matching donorID.
• deleteDonor(donorID): delete the donor with donorID from the list of donors, only if they are not associated with any books.
2. The app should have books-repo.js to maintain the list of donated books and allow adding, updating, and deleting books. The book object has bookID, title, authors, and donorID properties.
• donorID references the book’s donor. This…
arrow_forward
The main advantage of the encapsulation is that, it provides abstraction between an object and its clients.
Select one:
O True
OFalse
Encapsulation is the process of grouping the attributes and method of an object together in a single data structure known as a class
Select one:
O True
O False
A constructor is a member variable that is called automatically when an object is created.
Select one:
O True
O False
We cannot declare more than one constructor per class.
Select one:
O True
O False
on
arrow_forward
True/ False
1- Class objects can be defined prior to the class declaration.
2- You must use the private access specification for all data members of a class.
3- If an array is partially initialized, the uninitialized elements will be set to zero.
4- A vector object automatically expands in size to accommodate the items stored in it.
5- With pointer variables, you can access but not modify data in other variables.
6- Assuming myValues is an array of int values and index is an int variable, both of the
following statements do the same thing.
1.
cout header file.
15- To solve a problem recursively, you must identify at least one case in which the problem can be
solved without recursion.
arrow_forward
Class Student
__init__(self, id: int, fist_name: str, last_name: str, town:str):
""" This creates a student object with the specified ID first and last name and home town. This constructor should also create data structure for holding the students grades for all of there assignments. Additionally it should create a variable that holds the student's energy level which will be a number between 0 and 1. :param id: The student's identifiaction number :param fist_name: The student's first name :param last_name: The student's last name :param town: The student's home town """
get_id(self)->int:
""" Returns the ID of the student as specified in the constructor. :return: The student's ID """
get_first_name(self) -> str:
""" Returns the first name of the student. :return: The student's first name """
set_first_name(self, name:str):
""" Changes the student first name to the specified value of the name parameter. :param name: The value that the first name of the student will equal. """…
arrow_forward
Colorful bubbles
In this problem, you will use the Bubble class to make images of colorful bubbles. You will use your knowledge of classes and objects to make an instance of the Bubble class (aka instantiate a Bubble), set its member variables, and use its member functions to draw a Bubble into an image.
Every Bubble object has the following member variables:
X coordinate
Y coordinate
Size (i.e. its radius)
Color
Complete main.cc
Your task is to complete main.cc to build and draw Bubble objects based on user input. main.cc already does the work to draw the Bubble as an image saved in bubble.bmp. You should follow these steps:
First, you will need to create a Bubble object from the Bubble class.
Next, you must prompt the user to provide the following: an int for the X coordinate, an int for the Y coordinate, an int for the Bubble's size, and a std::string for the Bubble's color.
Next, you must use the user's input to set the new Bubble object's x and y coordinates, the size, and the…
arrow_forward
StockList Class
• This class will contain the methods necessary to display various information about the stock data.
• This class shall be a subclass of ArrayList.
• This class shall not contain any data fields. (You don't need them for this class if done correctly).
• This class shall have a default constructor with no parameters.
o No other constructors are allowed.
• This class shall have the following public, non-static, methods:
• printAllStocks:
This method shall take no parameters and return nothing.
▪ This method should print each stock in the StockList to an output file.
▪ NOTE: This method shall NOT print the data to the console.
The output file shall be called all_stock_data.txt.
The data should be printed in a nice and easy to read format.
o displayFirstTenStocks:
This method shall take no parameters and return nothing.
▪ This method should display only the first 10 stocks in the StockList in a nice and easy to read format.
▪ This method should display the data in the…
arrow_forward
True or false: The default constructor is the only constructor that may be called for objects in an array of objects.
arrow_forward
PyCharm Programming!
A Game object knows its name and the number of avators is currently has. It is initialized without Avatars objects. It has a method startGame, which initializes an internal empty list of Avator objects. It has a method to add one Avator object at a time to the Game. It also has a method to remove an Avatar object by the Avatar’s name. A Game object also has a method to animate all its current Avatar objects. Furthermore, it has a method to stopGame, which destroys all current Avator objects in its internal list.
The Avatar class creates simple human-type Avatars, which have attributes such as name, hair color, height, superpower. An Avatar object can change its hair color and superpower. It also has a method that “animates” the avatar. The animate() method should simply be a print message such as “Hello! I am …! I have…. hair and my super power is….!”, using the Avatar’s object variables.
In main program, create 3 different Avatar objects that resemble 3…
arrow_forward
2. The MyInteger Class
Problem Description:
Design a class named MyInteger. The class contains:
n
[]
[]
A private int data field named value that stores the int
value represented by this object.
A constructor that creates a MyInteger object for the
specified int value.
A get method that returns the int value.
Methods isEven () and isOdd () that return true if the value
is even or odd respectively.
Static methods isEven (int) and isOdd (int) that return true
if the specified value is even or odd respectively.
Static methods isEven (MyInteger) and isOdd (MyInteger) that
return true if the specified value is even or odd
respectively.
Methods equals (int) and equals (MyInteger) that return true
if the value in the object is equal to the specified value.
Implement the class. Write a client program that tests all
methods in the class.
arrow_forward
Java Code
Write a program in which you create a class called TextFileReader. The TextFileReader class must contain an instance variable consisting of an array of Strings 100 elements long.
TextFileReader should have two constructors: a default constructor and a constructor that takes a String argument, which represents the name of a text file to be opened and read into the array of Strings. The first (default) constructor does not really do anything. If the second constructor is used, it will open the file and read the contents of the file into the array of Strings as follows: fill the array by having your program read one line of the file into each string until you have filled the array. Once the array is full, you may stop reading the input file.
Add a member function contents(), which will convert the array of Strings into a single StringBuffer, which is then returned to the calling program so that it may be displayed.
Add a second member function display(), which prints the array…
arrow_forward
Chapter 15 Customer Employee Creator
Create an object-oriented program that allows you to enter data for customers and employees. Each class will be stored in its own python file.
Console
Specifications
Create a Person class that provides attributes for first name, last name, and email address. Include a parameter for each attribute in the constructor method. This class will provide a property or method that returns the person’s full name.
Create a Customer class that inherits the Person class. This class must add an attribute for a customer
Create an Employee class that inherits the Person class. This class must add an attribute for a social security number (SSN).
Put the above three classes in a module named ‘company_objects’, separate from the application module.
The program will create a Customer or Employee object from the data entered by the user and store the object in a single variable.
Create a function that will display the Customer or Employee. The function will have a…
arrow_forward
1. Employee Class
Write a class named Employee that has the following fields:
name. The name field references a String object that holds the employee's name.
•idNumber. The idNumber is an int variable that holds the employee's ID number.
• department. The department field references a string object that holds the name of
the department where the employee works.
• position. The position field references a string object that holds the employee's
job title.
The class should have the following constructors:
• A constructor that accepts the following values as arguments and assigns them to the
appropriate fields: employee's name, employee's ID number, department, and position.
• A constructor that accepts the following values as arguments and assigns them to the
appropriate fields: employee's name and ID number. The department and position
fields should be assigned an empty string ("*).
• A no-arg constructor that assigns empty strings ("") to the name, department, and
position fields, and…
arrow_forward
Problem 2: Route Planning You are on the development team for a Route Planning application. The team has already developed a class named NavigatorApp for storing and displaying the graph representing the road network. This class has a void displayShortestPath() function which takes the source and destination vertices of the trip and displays the shortest route between them. Using the strategy pattern, draw a class diagram of the system which will allow for the NavigatorApp to display the shortest driving, walking, or cycling route when the displayShortestPath() function is called. You can assume that another developer is in charge of making sure the appropriate strategy is correctly set and for calling the displayShortestPath() function
Starter code
/* Modify NavigatorApp (if necessary) */
class NavigatorApp {
private:
Graph map;
public:
void displayMap(Window * window);
void displayShortestPath(Vertex source, Vertex
destination, Window * window);
/* write pseudo
code for this function…
arrow_forward
In java language
arrow_forward
Create a BowlingTeam class
The class has 2 fields: a field for the team name and an array that holds the team members’ names.
Create get and set methods for the teamName field.
Add a setMember method that sets a team member’s name. The method requires a position and a name, and it uses the position as a subscript to the members array.
Add a getMember method that returns a team member’s name. The method requires a value used as a subscript that determines which member’s name to return.
Create a BowlingTeamDemo class. In the main method include the following:
Declare 2 variables: name and a constant NUM_TEAMS that holds 4 Bowling Team objects.
Declare and instantiate an array teams of BowlingTeam objects.
Using nested for loops, prompt the user to enter the 4 team names and enter the team members’ names.
Using another nested for loop, output each team’s name and their team members’.
Output should look like:
Members of team The Lucky Strikes
Carlos
Diego
Rose
Lynn
Members of team I…
arrow_forward
An n-sided regular polygon’s sides all have the same length and all of its angles have the same degree (i.e., the polygon is both equilateral and equiangular). Design a class named RegularPolygon thatcontains:■ A private int data field named n that defines the number of sides in the polygon.■ A private float data field named side that stores the length of the side.■ A private float data field named x that defines the x-coordinate of the center of the polygon with default value 0.
■ A private float data field named y that defines the y-coordinate of the center of the polygon with default value 0.■ A constructor that creates a regular polygon with the specified n (default 3),side (default 1), x (default 0), and y (default 0).■ The accessor and mutator methods for all data fields.■ The method getPerimeter() that returns the perimeter of the polygon.■ The method getArea() that returns the area of the polygon. The formula for computing the area of a regular polygon is Area = (n * s2) / (4 *…
arrow_forward
JAVA -
arrow_forward
Objects and Pointers
Write code to instantiate/create two Bicycle objects: a default object (my_bike) and a non-default object (sister_bike). For the non-default object you may hard-code (or make up) the required parameters (brand, price and wheel size). No console input is needed.
arrow_forward
1. Dummy GUI Application
by Codechum Admin
A GUI Application is an application that has a user interface that the user can interact with. For this program, we will be simulating this behavior.
First, implement another class called Checkbox which implements the Clickable interface which has only one method: public void click(). The Checkbox will have the following properties:
private boolean isChecked (defaults to false upon the creation of object)
private String text
Additionally, it should have the following methods:
the implementation of the click() method
If the isChecked is currently false, this will set the isChecked to true and will then print the message "Checkbox is checked". If it is currently true, this will set the isChecked to false and will then print the message "Checkbox is unchecked". Note that the messages to be printed should have also print a new line at the end.
an override of the toString() method which returns the message: "Checkbox ({text} - Clicked…
arrow_forward
17. Phone Book ArrayList
Write a class named PhoneBookEntry that has fields for a person's name and phone number.
The class should have a constructor and appropriate accessor and mutator methods. Then
write a program that creates at least five PhoneBookEntry objects and stores them in an
ArrayList. Use a loop to display the contents of each object in the ArrayList.
arrow_forward
RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should
store the following data in attributes: item description, units in inventory, and price.
Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them:
Description Units in Inventory Price
Item #1 Jacket 12 59.95
Item #2 Designer Jeans 40 34.95
Item #3 Shirt 20 24.95
arrow_forward
Write a program that will contain an array of person class objects.
The program should include two classes:
1) One class will be the person class.
2) The second class will be the team class, which contain the main method and the array or array list.
The person class should include the following data fields;
Name
Phone number
Birth Date
Jersey Number
Be sure to include get and set methods for each data field.The team class should contain the data fields for the team, such as:
Team name
Coach name
Conference name
The program should include the following functionality.
Add person objects to the array;
Find a specific person object in the array; (find a person using any data field you choose such as name or jersey number)
Output the contents of the array, including all data fields of each person object. (display roster)
arrow_forward
1. Employee Class
Write a class named Employee that has the following fields:
• name. The name field references a String object that holds the employee's name.
• idNumber. The idNumber is an int variable that holds the employee's ID number
• department. The department field references a String object that holds the no
of the department where the employee works.
• position. The position field references a String object that holds the employee's
job title.
The class should have the following constructors:
• A constructor that accepts the following values as arguments and assigns them to the
appropriate fields: employee's name, employee's ID number, department, and
position.
• A constructor that accepts the following values as arguments and assigns them to the
appropriate fields: employee's name and ID number. The department and position
fields should be assigned an empty string ("").
• A no-arg constructor that assigns empty strings ("") to the name, department, and
position fields, and…
arrow_forward
JAVA Programming Problem 3 - Book
A Book has such properties as title, author, and numberOfPages. A Volume will have properties such as volumeName, numberOfBooks, and an array of book objects (Book [ ]). You are required to develop the Book and Volume classes, then write an application (DemoVolume) to test your classes. The directions below will give assistance.
Create a class called Book with the following properties using appropriate data types: Title, Author, numberOfPages, Create a second class called Volume with the following properties using appropriate data types: volumeName, numberOfBooks and Book [ ]. The Book [ ] contains an array of book objects.
Directions
Create a class called Book with the following properties using appropriate data types: Title, Author, numberOfPages,
The only methods necessary in the Book class, for this exercise, are the constructor and a toString().
Create a second class called Volume with the following properties using appropriate data types:…
arrow_forward
11:02
all 10%
+
Create 1 1000.01
Create 2 2000.02
Create 3 3000.03
Deposit 111.11
Deposit 2 22.22
Withdraw 4 5000.00
Create 4 4000.04
Withdraw 1 0.10
Balance 2
Withdraw 2 0.20
Deposit 3 33.33
Withdraw 4 0.40
Bad Command 65
Balance 1
Balance 2
Balance 3
Balance 4
arrow_forward
Computer programming
arrow_forward
Q1: Class called (num) and an objeet of this class called (numl).This class
contains two data members of type int ( a and b) with private access and two
member funetions with public access: Constructor to give value to variables
and show_number() to display the sum and multiply of variables. Write a
program to set and display the object fields.
arrow_forward
Code. 1
arrow_forward
Creational Pattern
arrow_forward
1.
Part 1: A point in the x-y plane is represented by its x-coordinate and y-coordinate.
Design the class Point that can store and process a point in the x-y plane.
Your class should contains the following methods.
Default constructor without parameters that initializes the private data member to
the suitable values
Constructor with parameters sets the values of the instance variables to the
values of the parameter.
Accessor methods that return the values of the x-axis and y-axis.
setPoint method which set the values of x-axis and y-axis
makeCopy method that make a copy of a received object of type Point.
getCopy method that returns a copy of the current object
toString method that returns the object string
isEqual that returns true if the current object is equal to the received object of type
point
distance, which receives two objects of type Point and returns the distance
arrow_forward
Appointment Class Requirements
The appointment object shall have a required unique appointment ID string that cannot be longer than 10 characters. The appointment ID shall not be null and shall not be updatable.
The appointment object shall have a required appointment Date field. The appointment Date field cannot be in the past. The appointment Date field shall not be null.Note: Use java.util.Date for the appointmentDate field and use before(new Date()) to check if the date is in the past.
The appointment object shall have a required description String field that cannot be longer than 50 characters. The description field shall not be null.
Appointment Service Requirements
The appointment service shall be able to add appointments with a unique appointment ID.
The appointment service shall be able to delete appointments per appointment ID.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
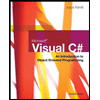
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
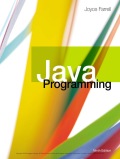
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Related Questions
- Rectangle Object Monitoring Create a Rectangle class that can compute the total area of all the created rectangle objects using static fields (variables). Remember that a Rectangle has two attributes: Length and Width. Implement the class by creating a computer program that will ask the user about three rectangle dimensions. The program should be able to display the total area of the three rectangle objects. For this exercise, you are required to apply all OOP concepts that you learned in class. Sample output: Enter Length R1: 1 Enter Width R1: 1 Enter Length R2: 2 Enter Width R2: 2 Enter Length R3: 3 Enter Width R3: 3 The total area of the rectangles is 14.00 Note: All characters in boldface are user inputs.arrow_forwardBook Donation App Create a book-app directory. The app can be used to manage book donations and track donors and books. The catalog is implemented using the following classes: 1. The app should have donors-repo.js to maintain the list of donors and allow adding, updating, and deleting donors. The donor object has donorID, firstName, lastName, and email properties. This module should implement the following functions: • getDonor(donorId): returns a donor by id. • addDonor(donor): adds a donor to the list of donors; donorID should be autoassigned a random number. • updateDonor(donor): updates the donor having the matching donorID. • deleteDonor(donorID): delete the donor with donorID from the list of donors, only if they are not associated with any books. 2. The app should have books-repo.js to maintain the list of donated books and allow adding, updating, and deleting books. The book object has bookID, title, authors, and donorID properties. • donorID references the book’s donor. This…arrow_forwardThe main advantage of the encapsulation is that, it provides abstraction between an object and its clients. Select one: O True OFalse Encapsulation is the process of grouping the attributes and method of an object together in a single data structure known as a class Select one: O True O False A constructor is a member variable that is called automatically when an object is created. Select one: O True O False We cannot declare more than one constructor per class. Select one: O True O False onarrow_forward
- True/ False 1- Class objects can be defined prior to the class declaration. 2- You must use the private access specification for all data members of a class. 3- If an array is partially initialized, the uninitialized elements will be set to zero. 4- A vector object automatically expands in size to accommodate the items stored in it. 5- With pointer variables, you can access but not modify data in other variables. 6- Assuming myValues is an array of int values and index is an int variable, both of the following statements do the same thing. 1. cout header file. 15- To solve a problem recursively, you must identify at least one case in which the problem can be solved without recursion.arrow_forwardClass Student __init__(self, id: int, fist_name: str, last_name: str, town:str): """ This creates a student object with the specified ID first and last name and home town. This constructor should also create data structure for holding the students grades for all of there assignments. Additionally it should create a variable that holds the student's energy level which will be a number between 0 and 1. :param id: The student's identifiaction number :param fist_name: The student's first name :param last_name: The student's last name :param town: The student's home town """ get_id(self)->int: """ Returns the ID of the student as specified in the constructor. :return: The student's ID """ get_first_name(self) -> str: """ Returns the first name of the student. :return: The student's first name """ set_first_name(self, name:str): """ Changes the student first name to the specified value of the name parameter. :param name: The value that the first name of the student will equal. """…arrow_forwardColorful bubbles In this problem, you will use the Bubble class to make images of colorful bubbles. You will use your knowledge of classes and objects to make an instance of the Bubble class (aka instantiate a Bubble), set its member variables, and use its member functions to draw a Bubble into an image. Every Bubble object has the following member variables: X coordinate Y coordinate Size (i.e. its radius) Color Complete main.cc Your task is to complete main.cc to build and draw Bubble objects based on user input. main.cc already does the work to draw the Bubble as an image saved in bubble.bmp. You should follow these steps: First, you will need to create a Bubble object from the Bubble class. Next, you must prompt the user to provide the following: an int for the X coordinate, an int for the Y coordinate, an int for the Bubble's size, and a std::string for the Bubble's color. Next, you must use the user's input to set the new Bubble object's x and y coordinates, the size, and the…arrow_forward
- StockList Class • This class will contain the methods necessary to display various information about the stock data. • This class shall be a subclass of ArrayList. • This class shall not contain any data fields. (You don't need them for this class if done correctly). • This class shall have a default constructor with no parameters. o No other constructors are allowed. • This class shall have the following public, non-static, methods: • printAllStocks: This method shall take no parameters and return nothing. ▪ This method should print each stock in the StockList to an output file. ▪ NOTE: This method shall NOT print the data to the console. The output file shall be called all_stock_data.txt. The data should be printed in a nice and easy to read format. o displayFirstTenStocks: This method shall take no parameters and return nothing. ▪ This method should display only the first 10 stocks in the StockList in a nice and easy to read format. ▪ This method should display the data in the…arrow_forwardTrue or false: The default constructor is the only constructor that may be called for objects in an array of objects.arrow_forwardPyCharm Programming! A Game object knows its name and the number of avators is currently has. It is initialized without Avatars objects. It has a method startGame, which initializes an internal empty list of Avator objects. It has a method to add one Avator object at a time to the Game. It also has a method to remove an Avatar object by the Avatar’s name. A Game object also has a method to animate all its current Avatar objects. Furthermore, it has a method to stopGame, which destroys all current Avator objects in its internal list. The Avatar class creates simple human-type Avatars, which have attributes such as name, hair color, height, superpower. An Avatar object can change its hair color and superpower. It also has a method that “animates” the avatar. The animate() method should simply be a print message such as “Hello! I am …! I have…. hair and my super power is….!”, using the Avatar’s object variables. In main program, create 3 different Avatar objects that resemble 3…arrow_forward
- 2. The MyInteger Class Problem Description: Design a class named MyInteger. The class contains: n [] [] A private int data field named value that stores the int value represented by this object. A constructor that creates a MyInteger object for the specified int value. A get method that returns the int value. Methods isEven () and isOdd () that return true if the value is even or odd respectively. Static methods isEven (int) and isOdd (int) that return true if the specified value is even or odd respectively. Static methods isEven (MyInteger) and isOdd (MyInteger) that return true if the specified value is even or odd respectively. Methods equals (int) and equals (MyInteger) that return true if the value in the object is equal to the specified value. Implement the class. Write a client program that tests all methods in the class.arrow_forwardJava Code Write a program in which you create a class called TextFileReader. The TextFileReader class must contain an instance variable consisting of an array of Strings 100 elements long. TextFileReader should have two constructors: a default constructor and a constructor that takes a String argument, which represents the name of a text file to be opened and read into the array of Strings. The first (default) constructor does not really do anything. If the second constructor is used, it will open the file and read the contents of the file into the array of Strings as follows: fill the array by having your program read one line of the file into each string until you have filled the array. Once the array is full, you may stop reading the input file. Add a member function contents(), which will convert the array of Strings into a single StringBuffer, which is then returned to the calling program so that it may be displayed. Add a second member function display(), which prints the array…arrow_forwardChapter 15 Customer Employee Creator Create an object-oriented program that allows you to enter data for customers and employees. Each class will be stored in its own python file. Console Specifications Create a Person class that provides attributes for first name, last name, and email address. Include a parameter for each attribute in the constructor method. This class will provide a property or method that returns the person’s full name. Create a Customer class that inherits the Person class. This class must add an attribute for a customer Create an Employee class that inherits the Person class. This class must add an attribute for a social security number (SSN). Put the above three classes in a module named ‘company_objects’, separate from the application module. The program will create a Customer or Employee object from the data entered by the user and store the object in a single variable. Create a function that will display the Customer or Employee. The function will have a…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
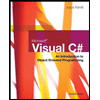
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
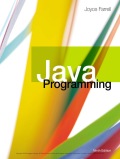
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT