hw02_revised
.pdf
keyboard_arrow_up
School
University of North Georgia, Dahlonega *
*We aren’t endorsed by this school
Course
1001
Subject
Computer Science
Date
Dec 6, 2023
Type
Pages
18
Uploaded by ProfessorIron11938
hw02_revised
September 15, 2023
1
Homework 2: Arrays, Table Manipulation, and Visualization
[4]:
# Don't change this cell; just run it.
# When you log-in please hit return (not shift + return) after typing in your
␣
,
→
email
import
numpy
as
np
from
datascience
import
*
# These lines do some fancy plotting magic.\n",
import
matplotlib
%
matplotlib
inline
import
matplotlib.pyplot
as
plots
plots
.
style
.
use(
'fivethirtyeight'
)
Recommended Reading
: *
Data Types
*
Sequences
*
Tables
Please complete this notebook by filling in the cells provided. Throughout this homework and all
future ones, please be sure to not re-assign variables throughout the notebook! For example, if you
use
max_temperature
in your answer to one question, do not reassign it later on.
Before continuing the assignment, select “Save and Checkpoint” in the File menu.
1.1
1. Creating Arrays
Question 1.
Make an array called
weird_numbers
containing the following numbers (in the given
order):
1. -2
2. the sine of 1.2
3. 3
4. 5 to the power of the cosine of 1.2
Hint:
sin
and
cos
are functions in the
math
module.
Note:
Python lists are different/behave differently than numpy arrays. In Data 8, we use numpy
arrays, so please make an
array
, not a python list.
[5]:
# Our solution involved one extra line of code before creating
# weird_numbers.
...
1
weird_numbers
=
make_array(
-2
, np
.
sin(
1.2
),
3
,
5**
np
.
cos(
1.2
))
weird_numbers
[5]:
array([-2.
,
0.93203909,
3.
,
1.79174913])
Question 2.
Make an array called
numbers_in_order
using the
np.sort
function.
[6]:
numbers_in_order
=
make_array(np
.
sort(weird_numbers))
numbers_in_order
[6]:
array([[-2.
,
0.93203909,
1.79174913,
3.
]])
Question 3.
Find the mean and median of
weird_numbers
using the
np.mean
and
np.median
functions.
[7]:
weird_mean
=
np
.
mean(weird_numbers)
weird_median
=
np
.
median(weird_numbers)
# These lines are provided just to print out your answers.
print
(
'weird_mean:'
, weird_mean)
print
(
'weird_median:'
, weird_median)
weird_mean: 0.930947052910613
weird_median: 1.361894105821226
1.2
2. Indexing Arrays
These exercises give you practice accessing individual elements of arrays. In Python (and in many
programming languages), elements are accessed by
index
, so the first element is the element at
index 0.
Note:
Please don’t use bracket notation when indexing (i.e.
arr[0]
), as this can yield different
data type outputs than what we will be expecting.
Question 1.
The cell below creates an array of some numbers. Set
third_element
to the third
element of
some_numbers
.
[8]:
some_numbers
=
make_array(
-1
,
-3
,
-6
,
-10
,
-15
)
third_element
=
some_numbers
.
item(
2
)
third_element
[8]:
-6
Question 2.
The next cell creates a table that displays some information about the elements of
some_numbers
and their order. Run the cell to see the partially-completed table, then fill in the
missing information (the cells that say “Ellipsis”) by assigning
blank_a
,
blank_b
,
blank_c
, and
blank_d
to the correct elements in the table.
2
[9]:
blank_a
=
"third"
blank_b
=
"fourth"
blank_c
= 0
blank_d
= 3
elements_of_some_numbers
=
Table()
.
with_columns(
"English name for position"
, make_array(
"first"
,
"second"
, blank_a,
␣
,
→
blank_b,
"fifth"
),
"Index"
,
make_array(blank_c,
1
,
2
, blank_d,
4
),
"Element"
,
some_numbers)
elements_of_some_numbers
[9]:
English name for position | Index | Element
first
| 0
| -1
second
| 1
| -3
third
| 2
| -6
fourth
| 3
| -10
fifth
| 4
| -15
Question 3.
You’ll sometimes want to find the
last
element of an array. Suppose an array has
142 elements. What is the index of its last element?
[10]:
index_of_last_element
= 141
index_of_last_element
[10]:
141
More often, you don’t know the number of elements in an array, its
length
. (For example, it might
be a large dataset you found on the Internet.) The function
len
takes a single argument, an array,
and returns the
len
gth of that array (an integer).
Question 4.
The cell below loads an array called
president_birth_years
. Calling
.column(...)
on a table returns an array of the column specified, in this case the
Birth Year
column of the
president_births
table.
The last element in that array is the most recent birth year of any
deceased president. Assign that year to
most_recent_birth_year
.
[11]:
president_birth_years
=
Table
.
read_table(
"president_births.csv"
)
.
column(
'Birth
␣
,
→
Year'
)
most_recent_birth_year
=
president_birth_years
.
item(
37
)
most_recent_birth_year
[11]:
1917
[12]:
president_birth_years
=
Table
.
read_table(
"president_births.csv"
)
.
column(
'Birth
␣
,
→
Year'
)
president_birth_years
3
[12]:
array([1732, 1735, 1743, 1751, 1758, 1767, 1767, 1773, 1782, 1784, 1790,
1791, 1795, 1800, 1804, 1808, 1809, 1822, 1822, 1829, 1831, 1833,
1837, 1843, 1856, 1857, 1858, 1865, 1872, 1874, 1882, 1884, 1890,
1908, 1911, 1913, 1913, 1917])
Question 5.
Finally, assign
sum_of_birth_years
to the sum of the first, tenth, and last birth
year in
president_birth_years
.
[13]:
sum_of_birth_years
=
president_birth_years
.
item(
0
)
+
president_birth_years
.
,
→
item(
9
)
+
president_birth_years
.
item(
37
)
sum_of_birth_years
[13]:
5433
1.3
3. Basic Array Arithmetic
Question 1.
Multiply the numbers 42, 4224, 42422424, and -250 by 157.
Assign each variable
below such that
first_product
is assigned to the result of
42
∗
157
,
second_product
is assigned
to the result of
4224
∗
157
, and so on.
For this question,
don’t
use arrays.
[14]:
first_product
= 42*157
second_product
= 4224*157
third_product
= 42422424*157
fourth_product
= -250*157
print
(first_product, second_product, third_product, fourth_product)
6594 663168 6660320568 -39250
Question 2.
Now, do the same calculation, but using an array called
numbers
and only a single
multiplication (
*
) operator. Store the 4 results in an array named
products
.
[15]:
numbers
=
make_array(
42
,
4224
,
42422424
,
-250
)
products
=
numbers
*157
products
[15]:
array([
6594,
663168, 6660320568,
-39250])
Question 3.
Oops, we made a typo! Instead of 157, we wanted to multiply each number by 1577.
Compute the correct products in the cell below using array arithmetic.
Notice that your job is
really easy if you previously defined an array containing the 4 numbers.
[16]:
correct_products
=
numbers
*1577
correct_products
[16]:
array([
66234,
6661248, 66900162648,
-394250])
Question 4.
We’ve loaded an array of temperatures in the next cell. Each number is the highest
temperature observed on a day at a climate observation station, mostly from the US. Since they’re
4
from the US government agency
NOAA
, all the temperatures are in Fahrenheit.
Convert them
all to Celsius by first subtracting 32 from them, then multiplying the results by
5
9
. Make sure to
ROUND
the final result after converting to Celsius to the nearest integer using the
np.round
function.
[17]:
max_temperatures
=
Table
.
read_table(
"temperatures.csv"
)
.
column(
"Daily Max
␣
,
→
Temperature"
)
celsius_max_temperatures
=
np
.
round((max_temperatures)
-32
)
*5/9
celsius_max_temperatures
[17]:
array([-3.88888889, 30.55555556, 31.66666667, …, 16.66666667,
22.77777778, 16.11111111])
Question 5.
The cell below loads all the
lowest
temperatures from each day (in Fahrenheit).
Compute the size of the daily temperature range for each day.
That is, compute the difference
between each daily maximum temperature and the corresponding daily minimum temperature.
Pay attention to the units, give your answer in Celsius!
Make sure
NOT
to round your
answer for this question!
[18]:
min_temperatures
=
Table
.
read_table(
"temperatures.csv"
)
.
column(
"Daily Min
␣
,
→
Temperature"
)
celsius_temperature_ranges
=
((max_temperatures)
-32
)
*5/9 -
␣
,
→
((min_temperatures)
-32
)
*5/9
celsius_temperature_ranges
[18]:
array([ 6.66666667, 10.
, 12.22222222, …, 17.22222222,
11.66666667, 11.11111111])
1.4
4. World Population
The cell below loads a table of estimates of the world population for different years, starting in
1950. The estimates come from the
US Census Bureau website
.
[19]:
world
=
Table
.
read_table(
"world_population.csv"
)
.
select(
'Year'
,
'Population'
)
world
.
show(
4
)
<IPython.core.display.HTML object>
The name
population
is assigned to an array of population estimates.
[20]:
population
=
world
.
column(
1
)
population
[20]:
array([2557628654, 2594939877, 2636772306, 2682053389, 2730228104,
2782098943, 2835299673, 2891349717, 2948137248, 3000716593,
3043001508, 3083966929, 3140093217, 3209827882, 3281201306,
5
3350425793, 3420677923, 3490333715, 3562313822, 3637159050,
3712697742, 3790326948, 3866568653, 3942096442, 4016608813,
4089083233, 4160185010, 4232084578, 4304105753, 4379013942,
4451362735, 4534410125, 4614566561, 4695736743, 4774569391,
4856462699, 4940571232, 5027200492, 5114557167, 5201440110,
5288955934, 5371585922, 5456136278, 5538268316, 5618682132,
5699202985, 5779440593, 5857972543, 5935213248, 6012074922,
6088571383, 6165219247, 6242016348, 6318590956, 6395699509,
6473044732, 6551263534, 6629913759, 6709049780, 6788214394,
6866332358, 6944055583, 7022349283, 7101027895, 7178722893,
7256490011])
In this question, you will apply some built-in Numpy functions to this array. Numpy is a module
that is often used in Data Science!
The difference function
np.diff
subtracts each element in an array from the element after it within
the array. As a result, the length of the array
np.diff
returns will always be one less than the
length of the input array.
The cumulative sum function
np.cumsum
outputs an array of partial sums. For example, the third
element in the output array corresponds to the sum of the first, second, and third elements.
Question 1.
Very often in data science, we are interested understanding how values change with
time. Use
np.diff
and
np.max
(or just
max
) to calculate the largest annual change in population
between any two consecutive years.
[21]:
largest_population_change
=
np
.
max(np
.
diff(population))
largest_population_change
[21]:
87515824
Question 2.
What do the values in the resulting array represent (choose one)?
[22]:
np
.
cumsum(np
.
diff(population))
[22]:
array([
37311223,
79143652,
124424735,
172599450,
224470289,
277671019,
333721063,
390508594,
443087939,
485372854,
526338275,
582464563,
652199228,
723572652,
792797139,
863049269,
932705061, 1004685168, 1079530396, 1155069088,
1232698294, 1308939999, 1384467788, 1458980159, 1531454579,
1602556356, 1674455924, 1746477099, 1821385288, 1893734081,
1976781471, 2056937907, 2138108089, 2216940737, 2298834045,
2382942578, 2469571838, 2556928513, 2643811456, 2731327280,
2813957268, 2898507624, 2980639662, 3061053478, 3141574331,
3221811939, 3300343889, 3377584594, 3454446268, 3530942729,
3607590593, 3684387694, 3760962302, 3838070855, 3915416078,
3993634880, 4072285105, 4151421126, 4230585740, 4308703704,
4386426929, 4464720629, 4543399241, 4621094239, 4698861357])
1) The total population change between consecutive years, starting at 1951.
6
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Type Casting & Inspection - How do you do this with Swift code?
arrow_forward
Q1. The last post didnt include the items in the database. Need a different function for the python program display_ticket below:
def display_tickets():t = Texttable()tickets=cur.execute('''SELECT * FROM tickets''')for i in tickets:t.add_rows([['tid', 'actual_speed','posted_speed','age','violator_sex'],list(i)])print()print(t.draw())print()
Q2. Also need a different function for the python program add_ticket below:
def add_tickets():actual_speed=int(input("enter actual speed:"))posted_speed=int(input("enter posted speed:"))age=int(input("enter age:"))sex=input("Male or Female:")cur.execute('''INSERT INTO tickets(actual_speed,posted_speed,age,violator_sex) VALUES(?,?,?,?)''',(actual_speed,posted_speed,age,sex))con.commit()
If you'd like to see what thousands of traffic tickets look like, check out this link: https://www.twincities.com/2017/08/11/we-analyzed-224915-minnesota-speeding-tickets-see-what-we-learned/
arrow_forward
Campground reservations, part 3: Available campsites
Excellent job! This next one's a little more challenging.
Which campsites are available to reserve?
We want to be able to quickly find which campsites are available. Can you help us?
Given a campgrounds array, return an array with the campsite numbers of all currently unreserved (isReserved === false) campsites. Call the function availableCampsites.
Dataset
As a reminder, our data looks like this. It's an array with a bunch of campsite objects in it. Here is a small subset of the data to give you an idea:
const campgrounds = [ { number: 1, view: "ocean", partySize: 8, isReserved: false }, { number: 5, view: "ocean", partySize: 4, isReserved: false }, { number: 12, view: "ocean", partySize: 4, isReserved: true }, { number: 18, view: "forest", partySize: 4, isReserved: false }, { number: 23, view: "forest", partySize: 4, isReserved: true }, ];
arrow_forward
Output and writer please.
arrow_forward
In cell C18 type a VLOOKUP function to find the corresponding letter grade (from column D) for the name in A18. The table array parameter is the same as in B18. Type FALSE for the range lookup parameter. Copy the formula in C18 to C19:C22Notice this formula works correctly for all cells. Range lookup of FALSE means do an exact match on the lookup value whether or not the table array is sorted by its first column.
In cell E11, type an IF function that compares the score in B11 with the minimum score to pass in A5. If the comparison value is true, display Pass. Otherwise, display Fail. Copy the formula in E11 to E12:E15. Did you use appropriate absolute and relative references so the formula copied properly?
In cell F11, type an IF function that compares the grade in D11 with the letter F (type F). If these two are not equal, display Pass. Otherwise, display Fail. Copy the formula in F11 to F12:F15
arrow_forward
Objective:
This activity has the purpose of helping students to design proper hashing strategies. (Objective 3).
Students Instructions:
After studying this module, write the answer to the following programming assignments in a text file and
submit them as an attached Word doc or PDF through the provided link in Blackboard. The deadline for
submitting this assignment is indicated on "Tools", which is located in "Calendar" in the "Blackboard"
platform. You will have two (2) attempt to send your answers by Assignment link before the deadline and
the last submission would be considered as your final solution. This exercise has
Implement a dictionary by using hashing and separate chaining. Question:
arrow_forward
C# Programming
See attached photo for the problem needed to be fixed.
Please make my code correct without changing the IEnumerable<string> in the function because it is crucial to my code. I need this code to be corrected and if you do, I owe you a big thank you and good rating. Thank you so much for your help in advance!
arrow_forward
Please help me write the code to produce the outcome scenarios below. Thank you
1. A Grave is a dictionary with two keys:
'name': A string value with the grave's occupant's name
'message': A string value with the grave's message
Define a function named count_shouters that consumes a list of graves and produces an integer representing the number of graves that had their messages in all capital letters. Hint: use the .upper() method.
You are not required to document of unit test the function.
2.
A Media is a dictionary with the following keys:
"name": The name of this media
"kind": Either "movie", "song", or "game"
"duration": The length of this media in minutes
Define the function longest_kind that consumes a list of Media and produces a string value representing the kind that had the highest duration. If the list is empty, return the value None.
You are not required to document of unit test the function.
arrow_forward
Write a program that generates a square table that contains randomly generated integer values that are between 0 and10.
- include a fixed seed value by using the function random.seed
arrow_forward
Array can store values of same datatypes.
Select one:
True
False
arrow_forward
JQuery loading
Consider following code
$(function(){
// jQuery methods go here...
});
What is the purpose of this code with respect to JQuery ?
O This is to prevent any jQuery code from running before the document is finished loading (is ready).
O This is to load the jQuery and to run this as soon as page start before loading.
This is to load the function so we can run jQuery code
arrow_forward
Here is the code I have written in Python but I need to make DRY using one single get_array function pulling in 5 times
def display_common_items(plant_xml): plant_root = plant_xml.getroot() common_array = [] for item in plant_root.findall("PLANT"): common_array.append(item.find("COMMON").text) return common_array
def display_botanical_items(plant_xml): plant_root = plant_xml.getroot() botanical_array = [] for item in plant_root.findall("PLANT"): botanical_array.append(item.find("BOTANICAL").text) return botanical_array
def display_zone_items(plant_xml): plant_root = plant_xml.getroot() zone_array = [] for item in plant_root.findall("PLANT"): zone_array.append(item.find("ZONE").text) return zone_array
def display_light_items(plant_xml): plant_root = plant_xml.getroot() light_array = [] for item in plant_root.findall("PLANT"): light_array.append(item.find("LIGHT").text) return light_array
def…
arrow_forward
This code gets an error for going out of range in the last for loop. What can I do to fix this?
# Lucas Conklin# 5772707import csvimport statistics
def readCSVIntoDictionary(f_name): data = [] with open(f_name) as f: reader = csv.reader(f) for row in reader: if not data: for index in range(len(row)): data.append([]) for index in range(len(row)): data[index].append(float(row[index])) f.close() return data
features = readCSVIntoDictionary("C:\\Users\\lucas\\Downloads\\pima.csv")print(features)
def find_median_and_SD(data, feature): med = statistics.median(data[feature]) rounded_med = round(med, 4) st_dev = statistics.stdev(data[feature]) rounded_st_dev = round(st_dev, 5) return rounded_med, rounded_st_dev
for i in range(0, len(features)): (median, st_dev) = find_median_and_SD(features, i) print(f'Feature {i} Median: {median} Standard Deviation: {st_dev}')…
arrow_forward
def get_legal_word_scores(letters, dictionary, value_dict):
"""
Given a str of letters that we can use, and a dictionary
that contains all legal words allowed in scrabble game,
and a value_dict that maps each letter to a score,
Returns a dictionary that contains all possible scores
earned by any legal word as the key, and for each score,
the score/key maps to a list of words that are from the
dictionary made from the given letters and computes to that
score. The list should be in alphabetical order.
"""
arrow_forward
python please
arrow_forward
Provide full code in terminawith code
arrow_forward
File names: color_square.py, chessboard.py, test_squares.py
Positions on a chess board are identified by a letter and a number. The letter identifies the column; the number identifies the row. See the image below.
You will provide the functions that allow us to determine:
if a chessboard square is either black or white, given its coordinates.
If the row/column is valid or not for the standard chessboard
For example, if we pass in “d” for the column and 4 for the row, your function that determines color should return the string: “BLACK”. If we pass in "k" for the column to your function that validates columns, that function should return the boolean False.
Requirements:
Your function for validating the column should be able to accept upper or lowercase strings as parameters.
Your functions for validating the row should be able to accept integers or strings as parameters.
Your function that determines black-or-white may assume that the input has been validated prior to us calling…
arrow_forward
Fix an error for me please?
arrow_forward
Task 7
Suppose you are given two dictionaries.
Now create a new dictionary "marks", merging the two dictionaries, so that the original two dictionaries remain unchanged.
Note: You can use dictionary functions.
Given:
{'Harry':15, 'Draco':8, 'Nevil":19}
{'Ginie':18, 'Luna': 14}
Output:
{'Harry': 15, 'Draco': 8, 'Nevil": 19, 'Ginie": 18, 'Luna': 14}
arrow_forward
Question 19 a
Consider the following statement, which is intended to create an ArrayList named years that can be used to store elements both of type Integer and of type String.
/* missing code */ = new ArrayList();
Which of the following can be used to replace /* missing code */ so that the statement compiles without error?
ArrayList years
B
ArrayList years()
ArrayList years[]
D
ArrayList years
E
ArrayList years
arrow_forward
Fix an error and show it please?
arrow_forward
Give the index of the pivot value after the whole list below has been partitioned using the median-of-3 value as the pivot value.
[93, 49, 60, 65, 40, 74, 50, 69, 58, 97, 64]
Notes:
• Your answer should be a single valid, non-negative, literal Python int value. For example, 123 is a valid int literal.
• This is not asking for the complete quicksort.
• Hint: you don't have to get everything in the exactly the right place, just the pivot value. You can do a rough partition, ensuring each value is on
the appropriate side of the pivot value.
• You can pre-check your answer (to check it's a valid, non-negative, literal int).
Answer: (penalty regime: 10, 20, ... %)
Precheck
Check
arrow_forward
Mr. Patrick is fond of reading books he is looking for a computerized way to keep record of hisbook collection, you have been given the responsibility to meet up his following requirements.(Use appropriate data structure to accomplish your task), develop following methods:1. Add(): adds a new book to the list ( A book contains name of the book, author name)NOTE: every new book will have access to its neighboring books (previous and next)2. Remove():in case any book is removed from the pile update your list3. countBooks(): displays the total number of books 4. search(): returns true if the given book is present in the list else returns false 5. display(): shows the book collection
arrow_forward
Javascript:
Using the data set as a pre-defined variable in your program, write code that uses the dataset to print the FIRST NAMES ONLY of people who have BOTH above average English grades AND below average age from the dataset.
var dataSet = [
{
"name":"Maura Glass",
"age":60,
"math":97,
"english":63,
"yearsOfEducation":4
},
{
"name":"James Gates",
"age":55,
"math":72,
"english":96,
"yearsOfEducation":10
},
{
"name":"Mills Morris",
"age":26,
"math":83,
"english":77,
"yearsOfEducation":10
},
{
"name":"Deena Morton",
"age":57,
"math":63,
"english":63,
"yearsOfEducation":10
},
{
"name":"Edith Roth",
"age":38,
"math":79,
"english":94,
"yearsOfEducation":10
},
{
"name":"Marva Morse",
"age":31,
"math":93,
"english":78,
"yearsOfEducation":9
},
{
"name":"Etta Potts",
"age":48,
"math":57,
"english":93,
"yearsOfEducation":7
},
{
"name":"Tate Moss",
"age":22,
"math":83,
"english":64,
"yearsOfEducation":8
},
{
"name":"Sanders Burris",
"age":27,
"math":65,
"english":66,
"yearsOfEducation":5…
arrow_forward
1. Declare a reference variable to an array of integer elements. The name of the variable
is listl.
Remember that the syntax for declaring a reference variable is:
elementType [] refVariable;
2. Print the reference variable to the console, as in the statement below, and observe and
understand what happens. Then, remove the print statement.
System.out.println(list1);
3. Create an array of 50 integer values, and assign its reference to the variable list1.
Remember that the syntax for creating an array is:
new elementType[arraySize];
In order to assign the returned reference to a reference variable, we put the new
statement to the right of an assignment statement to a reference variable.
refVariable = new elementType[arraySize]
%3D
4. Combine array declaration and creation such that they are on the same line as in the
following syntax:
elementType [] refVariable= new elementType[arraySize];
5. Try to print the value of the reference variable to the console again, and observe the
output.…
arrow_forward
individual characters using their 0-based index. Hint: You will need to do this for checking all the rules. However, when you access a character .
arrow_forward
*Reasking a question to make request clearer
Looking to code in python the following:
Suppose the weekly hours for all employees are stored in a table.
Each row records an employee’s seven-day work hours with seven columns. For example, the following table stores the work hours for eight employees.
Su M T W Th F Sa Employee 0 2 4 3 4 5 8 8 Employee 1 7 3 4 3 3 4 4 Employee 2 3 3 4 3 3 2 2 Employee 3 9 3 4 7 3 4 1 Employee 4 3 5 4 3 6 3 8 Employee 5 3 4 4 6 3 4 4 Employee 6 3 7 4 8 3 8 4 Employee 7 6 3 5 9 2 7 9
I need a program that inputs the hours of all employees by the user and displays employees and their total hours in decreasing order of the total hours.
Thanks
arrow_forward
Analyze the following code:int matrix[5][5];for (int column = 0; column < 5; column++);matrix[4][column] = 10;
Select the correct option:
After the loop, the last row of matrix 10, 10, 10, 10, 10.
After the loop, the last row of matrix 0, 0, 0, 0, 0.
A syntax error, because column is not defined.
After the loop, the last column of matrix 10, 10, 10, 10, 10.
After the loop, the last row of matrix 0, 0, 0, 0, 10.
arrow_forward
pizzaStats Assignment Description
For this assignment, name your R file pizzaStats.R
For all questions you should load tidyverse and lm.beta. You should not need to use any other libraries.
Load tidyverse with
suppressPackageStartupMessages(library(tidyverse))
Load lm.beta withsuppressPackageStartupMessages(library(lm.beta))
Run a regression predicting whether or not wine was ordered from temperature, bill, and pizza.
Assign the coefficients of the summary of the model to Q1. The output should look something like this:
Estimate Std. Error z value Pr(>|z|)(Intercept) -1.09 1.03 -1.06 0.29temperature -0.04 0.01 -3.20 0.00bill 0.03 0.01 3.75 0.00pizzas 0.19 0.06 3.27 0.00
Here is the link of the pizza.csv https://drive.google.com/file/d/1_m2TjPCkPpMo7Z2Vkp32NiuZrXBxeRn6/view?usp=sharing
arrow_forward
Create a Java array FirstArray, type int, length 10. Initialize it with the multiple of 2.
Print the array using comma separators. (Hint: Use Arrays.toSring method. You need to import java.util.Arrays package)
Print the array with vertical line (|) separator. DO NOT put the vertical line after last element.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
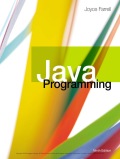
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
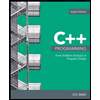
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Related Questions
- Type Casting & Inspection - How do you do this with Swift code?arrow_forwardQ1. The last post didnt include the items in the database. Need a different function for the python program display_ticket below: def display_tickets():t = Texttable()tickets=cur.execute('''SELECT * FROM tickets''')for i in tickets:t.add_rows([['tid', 'actual_speed','posted_speed','age','violator_sex'],list(i)])print()print(t.draw())print() Q2. Also need a different function for the python program add_ticket below: def add_tickets():actual_speed=int(input("enter actual speed:"))posted_speed=int(input("enter posted speed:"))age=int(input("enter age:"))sex=input("Male or Female:")cur.execute('''INSERT INTO tickets(actual_speed,posted_speed,age,violator_sex) VALUES(?,?,?,?)''',(actual_speed,posted_speed,age,sex))con.commit() If you'd like to see what thousands of traffic tickets look like, check out this link: https://www.twincities.com/2017/08/11/we-analyzed-224915-minnesota-speeding-tickets-see-what-we-learned/arrow_forwardCampground reservations, part 3: Available campsites Excellent job! This next one's a little more challenging. Which campsites are available to reserve? We want to be able to quickly find which campsites are available. Can you help us? Given a campgrounds array, return an array with the campsite numbers of all currently unreserved (isReserved === false) campsites. Call the function availableCampsites. Dataset As a reminder, our data looks like this. It's an array with a bunch of campsite objects in it. Here is a small subset of the data to give you an idea: const campgrounds = [ { number: 1, view: "ocean", partySize: 8, isReserved: false }, { number: 5, view: "ocean", partySize: 4, isReserved: false }, { number: 12, view: "ocean", partySize: 4, isReserved: true }, { number: 18, view: "forest", partySize: 4, isReserved: false }, { number: 23, view: "forest", partySize: 4, isReserved: true }, ];arrow_forward
- Output and writer please.arrow_forwardIn cell C18 type a VLOOKUP function to find the corresponding letter grade (from column D) for the name in A18. The table array parameter is the same as in B18. Type FALSE for the range lookup parameter. Copy the formula in C18 to C19:C22Notice this formula works correctly for all cells. Range lookup of FALSE means do an exact match on the lookup value whether or not the table array is sorted by its first column. In cell E11, type an IF function that compares the score in B11 with the minimum score to pass in A5. If the comparison value is true, display Pass. Otherwise, display Fail. Copy the formula in E11 to E12:E15. Did you use appropriate absolute and relative references so the formula copied properly? In cell F11, type an IF function that compares the grade in D11 with the letter F (type F). If these two are not equal, display Pass. Otherwise, display Fail. Copy the formula in F11 to F12:F15arrow_forwardObjective: This activity has the purpose of helping students to design proper hashing strategies. (Objective 3). Students Instructions: After studying this module, write the answer to the following programming assignments in a text file and submit them as an attached Word doc or PDF through the provided link in Blackboard. The deadline for submitting this assignment is indicated on "Tools", which is located in "Calendar" in the "Blackboard" platform. You will have two (2) attempt to send your answers by Assignment link before the deadline and the last submission would be considered as your final solution. This exercise has Implement a dictionary by using hashing and separate chaining. Question:arrow_forward
- C# Programming See attached photo for the problem needed to be fixed. Please make my code correct without changing the IEnumerable<string> in the function because it is crucial to my code. I need this code to be corrected and if you do, I owe you a big thank you and good rating. Thank you so much for your help in advance!arrow_forwardPlease help me write the code to produce the outcome scenarios below. Thank you 1. A Grave is a dictionary with two keys: 'name': A string value with the grave's occupant's name 'message': A string value with the grave's message Define a function named count_shouters that consumes a list of graves and produces an integer representing the number of graves that had their messages in all capital letters. Hint: use the .upper() method. You are not required to document of unit test the function. 2. A Media is a dictionary with the following keys: "name": The name of this media "kind": Either "movie", "song", or "game" "duration": The length of this media in minutes Define the function longest_kind that consumes a list of Media and produces a string value representing the kind that had the highest duration. If the list is empty, return the value None. You are not required to document of unit test the function.arrow_forwardWrite a program that generates a square table that contains randomly generated integer values that are between 0 and10. - include a fixed seed value by using the function random.seedarrow_forward
- Array can store values of same datatypes. Select one: True Falsearrow_forwardJQuery loading Consider following code $(function(){ // jQuery methods go here... }); What is the purpose of this code with respect to JQuery ? O This is to prevent any jQuery code from running before the document is finished loading (is ready). O This is to load the jQuery and to run this as soon as page start before loading. This is to load the function so we can run jQuery codearrow_forwardHere is the code I have written in Python but I need to make DRY using one single get_array function pulling in 5 times def display_common_items(plant_xml): plant_root = plant_xml.getroot() common_array = [] for item in plant_root.findall("PLANT"): common_array.append(item.find("COMMON").text) return common_array def display_botanical_items(plant_xml): plant_root = plant_xml.getroot() botanical_array = [] for item in plant_root.findall("PLANT"): botanical_array.append(item.find("BOTANICAL").text) return botanical_array def display_zone_items(plant_xml): plant_root = plant_xml.getroot() zone_array = [] for item in plant_root.findall("PLANT"): zone_array.append(item.find("ZONE").text) return zone_array def display_light_items(plant_xml): plant_root = plant_xml.getroot() light_array = [] for item in plant_root.findall("PLANT"): light_array.append(item.find("LIGHT").text) return light_array def…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
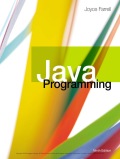
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
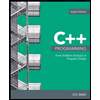
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning