Project_B_Description
pdf
keyboard_arrow_up
School
University of Illinois, Chicago *
*We aren’t endorsed by this school
Course
366
Subject
Electrical Engineering
Date
Apr 3, 2024
Type
Pages
7
Uploaded by SuperHumanBuffalo3416
Project B: Building 32-Bit Adders ECE 366 Computer Organization Spring 2024 Final Submission Due: Friday, Mar. 15 Subject to minor changes Last update: Feb. 18 1.
Overview The purpose of this project is to exercise building digital blocks. You will use Verilog/SystemVerilog to construct 32-bit carry-lookahead adder
and 32-bit prefix adder
. Then, you will use ModelSim to simulate the circuits and verify their functional correctness and timing. The project is group based. The group assignment will be done randomly, unless you and a partner of your choice both request to form a group. Such requests should be sent to the instructor no later than Friday, Feb. 23. There will be three BlackBoard assignments related to this project. You have to make three submissions to get the full credit (see below). 2.
Get Started ModelSim. You will need to install ModelSim 20.1.1 of Intel FPGA Starter Edition on a Windows computer. Use this download link: https://www.intel.com/content/www/us/en/software-
kit/750666/modelsim-intel-fpgas-standard-edition-software-version-20-1-1.html
, choose version 20.1.1 and Windows Software (see below). Run the installer, and choose “ModelSim – Intel FPGA Starter Edition.” License is not required for the starter edition.
2 Create Project and Compile Starter Code
. In ModelSim, create a project named “Project-B”, and then add all the “*.sv” files to the project. Then, in the “Compile” menu, choose “Compile Order” and then select “Auto Generate”. You will see a screenshot like this: Compile all the program files. Verify there is no compiler error. If there is any error, you will see an error message in red color in the Transcript window. Double click on error message, and you will see a pop-up window with detailed error message.
3 Simulate Project and Verify Waveforms. Start
the simulation from the “Simulate” menu. Then, choose tb_ripple_carry_adder_32bit and then select “OK”. Note: If you want, you may simulate any other objects there. Then you will see an “Objects” window. Select all the signals, and then use the right-click menu to add those signals to the “Wave” window (see the screenshot).
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
4 In the Transcript window, type command “run 9.6ns” and then “run 0.2ns” to run the simulation. You will see updates of waveforms in the Wave window. The following are the waveforms from this simulation. They show the inputs and outputs of four instances of the 32-bit ripple-carry adder. Note that the waveforms have been re-organized and formatted – Explore the right-click menu to reproduce the waveforms in the same format. You may save the wave format to a “wave.do” file, and then reload “wave.do” to use the same format after restarting ModelSim. You can see that the outputs are correct in those four test cases, and the worst-case delay is no more than 9.6ns. 3.
Starter Code Review, Coding Requirement, and ModelSim Hints Now read all the starter codes. Make sure you have a good understanding before you proceed. If needed, review Textbook Ch. 5 Hardware Description Languages, and/or ask the professor for any question you may have. In Parts 4 and 5, you must use structural modeling strictly as the starter codes do. In particular, you may not use any assign
statement. The following is an example of structural modeling: // 4-bit ripple-carry adder module ripple_carry_adder_4bit(input logic[3:0] a, input logic[3:0] b, input logic c_in, output logic[3:0] s, output logic c_out); logic[2:0] c; // instantiate four 1-bit full adders, connect their c_out and c_in full_adder_1bit adder0(a[0], b[0], c_in, s[0], c[0]); full_adder_1bit adder1(a[1], b[1], c[0], s[1], c[1]); full_adder_1bit adder2(a[2], b[2], c[1], s[2], c[2]); full_adder_1bit adder3(a[3], b[3], c[2], s[3], c_out); endmodule Additionally, all circuits in Part 4 or Part 5 must be derived from the gates given in “basic_gates.sv”. You may only use those gates and your circuits constructed from those gates.
5 Hardware debugging in ModelSim is very different from program debugging. You may search the Internet for additional help. If you are stuck, please come to the professor’s office hours. You may also discuss with the professor after class meetings or make appointments for help. 4.
Construct the Design of 32-bit Carry-Lookahead Adder In this part, you may only use those gates given in “basic_gates.sv” and your circuits constructed from those gates. Create two new program files, namely “carry_lookahead.sv” and “tb_carry_lookahead.sv”, for your 32-bit carry-lookahead adder design and its testbench. Review “ripple_carry.sv” and “tb_ripple_carry.sv” as samples. In “carry_lookahead.sv”, construct two SystemVerilog modules, one for 4-bit adder with carry-
lookahead
and another one form 32-bit adder with carry-lookahead
. Use the following diagram to guide your design. In “tb_carry_lookahead.sv”, copy the content from “tb_ripple_carry.sv”, and then revise it to test your 32-bit adder with carry-lookahead. You must include all the four test cases, and you’re free to add more. Add the two program files to the ModelSim project. Then, compile, simulate, and observe the waveforms to verify the functional correctness and timing of your design. Note that the delay must be no more than 3.3ns in all test cases. 5.
Construct the Design of 32-bit Prefix Adder In this part, you may only use those gates given in “basic_gates.sv” and your circuits constructed from those gates. Create two new program files, namely “prefix_adder.sv” and “tb_prefix_adder.sv”, for your 32-
bit prefix adder design and its testbench. In “prefix_adder.sv”, construct four SystemVerilog modules for the four red boxes shown in the following diagram. If you want, you should also add more module(s) to simplify the coding for the top box. B
0
+
+
+
+
P
3:0
G
3
P
3
G
2
P
2
G
1
P
1
G
0
P
3
P
2
P
1
P
0
G
3:0
C
in
C
out
A
0
S
0
C
0
B
1
A
1
S
1
C
1
B
2
A
2
S
2
C
2
B
3
A
3
S
3
C
in
A
3:0
B
3:0
S
3:0
4-bit CLA
Block
C
in
A
7:4
B
7:4
S
7:4
4-bit CLA
Block
C
3
C
7
A
27:24
B
27:24
S
27:24
4-bit CLA
Block
C
23
A
31:28
B
31:28
S
31:28
4-bit CLA
Block
C
27
C
out
6 In “tb_carry_lookahead.sv”, copy the content from “tb_ripple_carry.sv”, and then revise it to test your 32-bit prefix adder. You must include all the four test cases, and you are required to add at least four more test cases. Add the two program files to the ModelSim project. Then, compile, simulate and observe the waveforms to verify the functional correctness and timing of your design. Note that the delay must be no more than 1.2ns in all test cases. 6.
GitHub, Project Assignments, and Submissions A GitHub repository will be created for your group. Use the repository for collaboration between you and your partner. Commit and push your assembly codes as frequent as needed. The commit history may also be used as an indication of the contribution of each partner. The following BlackBoard assignments will be created for all groups. a)
Project B Progress Report 1 [10 points, due on Mar. 1]
Use the template provided (Project_A_Report1.docx). Complete the progress report with the following sections. Submit a PDF file with the following sections: 1)
Partner Responsibility 2)
SystemVerilog Modules 3)
Summary of Project Status b)
Project B Progress Report 2 [10 points, due on Mar. 8]
Make a copy of your Project_B_Report1.docx and rename it to Project_B_Report2.docx. Extend the report to have the following sections: 1)
Partner Responsibility 2)
SystemVerilog Modules 3)
Testbench and Test Cases 4)
Summary of Project Status You may revise any existing section as you wish. 0:-1
-1
2:1
1:-1
2:-1
0
1
2
4:3
3
6:5
5:3
6:3
4
5
6
5:-1
6:-1
3:-1
4:-1
8:7
7
10:9
9:7
10:7
8
9
10
12:11
11
14:13
13:11
14:11
12
13
14
13:7
14:7
11:7
12:7
9:-1
10:-1
7:-1
8:-1
13:-1
14:-1
11:-1
12:-1
15
0
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
B
i
A
i
G
i:i
P
i:i
G
k-1:j
P
k-1:j
G
i:k
P
i:k
G
i:j
P
i:j
i
i:j
B
i
A
i
G
i-1:-1
S
i
i
Legend
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
7 c)
Project B Final Report and Submission [80 points, due on Mar. 15] Make a copy Project_B_Report2.docx and rename it to Project_B_Final_Report.docx. Add the following two Appendix sections: •
Appendix 1: Testing Result for Ripple-Carry Adder. Include a screenshot of the waveforms for the eight test cases. Discuss why you think the circuit is functionally correct and meets the timing constraint. •
Appendix 1: Testing Result for Prefix Adder. Include a screenshot of the waveforms for the eight test cases. Discuss why you think the circuit is functionally correct and meets the timing constraint. Push your latest codes into GitHub and the submit the following: 1)
The final report (in PDF format) 2)
All your SystemVerilog files Your codes will be tested for correctness. The testing may use your codes in GitHub, so make sure you push the latest version into GitHub.
Related Documents
Related Questions
Please may you give the solution to this computer science question!
Thank you
arrow_forward
3. What is analogue to digital conversion & explain in detail 2-bit quantization.
arrow_forward
Include circuit diagram
arrow_forward
Write a Verilog code with testbench for 16-bit up/down counter with synchronous reset and synchronous up/down.If up/down is set the counter is up counter and if it is not set, the counter is a down counter.
submit the module code, testbench code, and the simulation results. PLEASE EXECUTE CODE IN VERILOG
arrow_forward
Q1. (a) Design a stick (layout) diagram for the following static CMOS logic gate,
where A, B, C, D are the logic gate inputs and O/P is the output:
VDD
D-d[Q8
A-Q5 B-Q6
C-d [Q7
O/P
CQ3 DQ4
B-Q2
AQ1
Vss
Figure 1
Use dual-well, CMOS technology. Include wells, well-taps, contact cuts,
routing of power and GND in your diagram. Use colour coding and/or clear
and readable detailed annotations to represent the wires in the different
layers.
(b) The logic gate from (a) needs to drive a capacitive load of 150 fF with a
rise-time and fall-time of 1.2 ns. If the length of all transistors is 0.5 μm,
calculate the required widths for all P-type and all N-type MOSFETs in the logic
gate to achieve the required edge-speeds. Clearly show the calculation steps
of your solution.
Assume VDD = 5 V, K'n = 50 μA/V², K'p = 20 μA/V²
arrow_forward
Q1.
(a) Design a stick (layout) diagram for the following static CMOS logic gate,
where A, B, C, D are the logic gate inputs and O/P is the output:
VDD
D-d[Q8
A-Q5 B-Q6
c-d[Q7
O/P
CQ3 DQ4
B-Q2
A-Q1
Vss
Figure 1
Use dual-well, CMOS technology. Include wells, well-taps, contact cuts,
routing of power and GND in your diagram. Use colour coding and/or clear
and readable detailed annotations to represent the wires in the different
layers.
arrow_forward
Can I get the written solution by hand and not ai
arrow_forward
Q2/A) Design 8x1 multiplexer using 2x1 multiplexer?
Q2 B)Simplify the Logic circuit shown below using K-map then draw the
Simplified circuit?
Q2/C) design logic block diagram for adding 12 to 5 using full adder showing
the input for each adder?
arrow_forward
Q2. (a) Figure 2 shows the pinout diagram (and their functional description) of the
2114 Static RAM memory IC. Using a neat sketch show how such IC may be
interfaced with an M68000 microprocessor to realise a system that needs
1K x 16 bits of RAM.
[Note: 2114 is a 4-bit memory IC. You will need multiple ICs to make up the
16-bit address bus of M68000 processor.]
Ao-Ag
Pin Names
Address Inputs
A6 1
A5 2
A4
AO 5
A16
A2 7
CS 8
GND 9
-23456782
18 Vcc
WE
Write Enable
17 A7
CS
Chip Select
16 A8
1/0₁-1/04
Data Input/Output
A3 4
15 A9
VCC
Power (+5V)
GND
Ground
14 I/O 1
13 I/O 2
Truth Table
12 1/03
CS
WE
Comments
11 I/O 4
H
X
Chip Deselected
L
L
Write
10 WE
L
H
Read
Figure 2
(b) Design an address decoder circuit to realise the following memory map
(shown below in hexadecimal notation). The unused address range should
generate an active low signal to be connected to the BERR* input of the
M68000 microprocessor. Use the partial address decoding technique for your
design.
ROM1 00 0000 03 FFFF…
arrow_forward
Q2. (a) Figure 2 shows the pinout diagram (and their functional description) of the
2114 Static RAM memory IC. Using a neat sketch show how such IC may be
interfaced with an M68000 microprocessor to realise a system that needs
1K x 16 bits of RAM.
[Note: 2114 is a 4-bit memory IC. You will need multiple ICs to make up the
16-bit address bus of M68000 processor.]
Ao-Ag
Pin Names
Address Inputs
A6 1
A5 2
A4 3
A3 4
AO 5
A1 6
A2 7
CS 8
GND 9
+23456700
18 Vcc
WE
Write Enable
17 A7
CS
Chip Select
16 A8
1/01-1/04
Data Input/Output
15 A9
Vcc
Power (+5V)
GND
Ground
14 I/O 1
13 I/O 2
Truth Table
12 1/03
CS
WE
Comments
11 1/04
Н
Chip Deselected
L
L
Write
10 WE
L
H
Read
Figure 2
(b) Design an address decoder circuit to realise the following memory map
(shown below in hexadecimal notation). The unused address range should
generate an active low signal to be connected to the BERR* input of the
M68000 microprocessor. Use the partial address decoding technique for your
design.
ROM1 00 0000 03 FFFF…
arrow_forward
Please do part b using the Assumption
arrow_forward
d)
Draw the schematics of 4-bit synchronous and asynchronous MOD-8
counters and comment on their pros and cons.
e)
Calculate the noise margin for a logic gate with the following logic levels:
VIL = 1.1 V, VIH = 3.2 V, VOL = 0.6 V, VOH = 4.0 V.
arrow_forward
3d. will give thumbs up
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
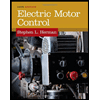
Related Questions
- Write a Verilog code with testbench for 16-bit up/down counter with synchronous reset and synchronous up/down.If up/down is set the counter is up counter and if it is not set, the counter is a down counter. submit the module code, testbench code, and the simulation results. PLEASE EXECUTE CODE IN VERILOGarrow_forwardQ1. (a) Design a stick (layout) diagram for the following static CMOS logic gate, where A, B, C, D are the logic gate inputs and O/P is the output: VDD D-d[Q8 A-Q5 B-Q6 C-d [Q7 O/P CQ3 DQ4 B-Q2 AQ1 Vss Figure 1 Use dual-well, CMOS technology. Include wells, well-taps, contact cuts, routing of power and GND in your diagram. Use colour coding and/or clear and readable detailed annotations to represent the wires in the different layers. (b) The logic gate from (a) needs to drive a capacitive load of 150 fF with a rise-time and fall-time of 1.2 ns. If the length of all transistors is 0.5 μm, calculate the required widths for all P-type and all N-type MOSFETs in the logic gate to achieve the required edge-speeds. Clearly show the calculation steps of your solution. Assume VDD = 5 V, K'n = 50 μA/V², K'p = 20 μA/V²arrow_forwardQ1. (a) Design a stick (layout) diagram for the following static CMOS logic gate, where A, B, C, D are the logic gate inputs and O/P is the output: VDD D-d[Q8 A-Q5 B-Q6 c-d[Q7 O/P CQ3 DQ4 B-Q2 A-Q1 Vss Figure 1 Use dual-well, CMOS technology. Include wells, well-taps, contact cuts, routing of power and GND in your diagram. Use colour coding and/or clear and readable detailed annotations to represent the wires in the different layers.arrow_forward
- Can I get the written solution by hand and not aiarrow_forwardQ2/A) Design 8x1 multiplexer using 2x1 multiplexer? Q2 B)Simplify the Logic circuit shown below using K-map then draw the Simplified circuit? Q2/C) design logic block diagram for adding 12 to 5 using full adder showing the input for each adder?arrow_forwardQ2. (a) Figure 2 shows the pinout diagram (and their functional description) of the 2114 Static RAM memory IC. Using a neat sketch show how such IC may be interfaced with an M68000 microprocessor to realise a system that needs 1K x 16 bits of RAM. [Note: 2114 is a 4-bit memory IC. You will need multiple ICs to make up the 16-bit address bus of M68000 processor.] Ao-Ag Pin Names Address Inputs A6 1 A5 2 A4 AO 5 A16 A2 7 CS 8 GND 9 -23456782 18 Vcc WE Write Enable 17 A7 CS Chip Select 16 A8 1/0₁-1/04 Data Input/Output A3 4 15 A9 VCC Power (+5V) GND Ground 14 I/O 1 13 I/O 2 Truth Table 12 1/03 CS WE Comments 11 I/O 4 H X Chip Deselected L L Write 10 WE L H Read Figure 2 (b) Design an address decoder circuit to realise the following memory map (shown below in hexadecimal notation). The unused address range should generate an active low signal to be connected to the BERR* input of the M68000 microprocessor. Use the partial address decoding technique for your design. ROM1 00 0000 03 FFFF…arrow_forward
- Q2. (a) Figure 2 shows the pinout diagram (and their functional description) of the 2114 Static RAM memory IC. Using a neat sketch show how such IC may be interfaced with an M68000 microprocessor to realise a system that needs 1K x 16 bits of RAM. [Note: 2114 is a 4-bit memory IC. You will need multiple ICs to make up the 16-bit address bus of M68000 processor.] Ao-Ag Pin Names Address Inputs A6 1 A5 2 A4 3 A3 4 AO 5 A1 6 A2 7 CS 8 GND 9 +23456700 18 Vcc WE Write Enable 17 A7 CS Chip Select 16 A8 1/01-1/04 Data Input/Output 15 A9 Vcc Power (+5V) GND Ground 14 I/O 1 13 I/O 2 Truth Table 12 1/03 CS WE Comments 11 1/04 Н Chip Deselected L L Write 10 WE L H Read Figure 2 (b) Design an address decoder circuit to realise the following memory map (shown below in hexadecimal notation). The unused address range should generate an active low signal to be connected to the BERR* input of the M68000 microprocessor. Use the partial address decoding technique for your design. ROM1 00 0000 03 FFFF…arrow_forwardPlease do part b using the Assumptionarrow_forwardd) Draw the schematics of 4-bit synchronous and asynchronous MOD-8 counters and comment on their pros and cons. e) Calculate the noise margin for a logic gate with the following logic levels: VIL = 1.1 V, VIH = 3.2 V, VOL = 0.6 V, VOH = 4.0 V.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
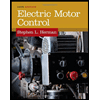