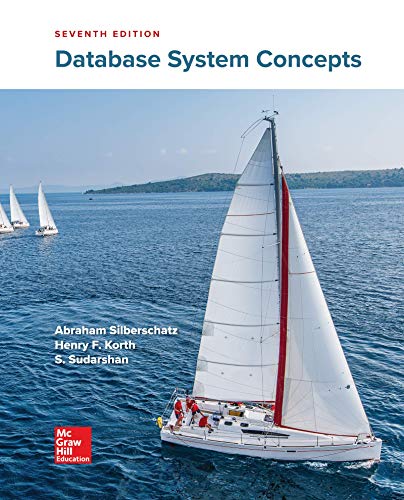
Concept explainers
1. Write a Python program to do the following :
a. Take two numbers from the user in separate inputs. and then perform addition, subtraction, multiplication and division calculations on 2 numbers.
b. Consider the possibility of input errors (i.e. divisor is 0? user entered string instead of numbers? user entered float?) Use try and except to handle the 2 exceptions by outputting statements that indicate related errors.
2. From the two statements given below, using list comprehension, create the list1 (i.e. the characters from v which are in w): list1 = ['e','o'] .
w = 'hello' v = ('a', 'e', 'i', 'o', 'u')
3. Write a function that returns the mean of a list that is passed as formal argument to a function. Create a list with values [10, 56.7, 56, 89, 100, 99, 87.5, 34, 985, 10] and pass it to the above defined function. Print the mean of the list of values.
4. Add a breakpoint to the mean function (created in question 3) such that the interactive console of pdb can be used to look at the values of mean after each item in the list is calculated.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Hello I need help with a couple of things in my python code. I'm working on files and exceptions 1. Line 15: There's a type error and I don't know how to change "numbers" from an str to an int 2. I have to execute two examples of this, one with the file_name golf.txt and the other without the file_name. But should I use FileNotFoundError, as it's typically used for files that don't exist and golf.txt does exist, or should I purposefully make a mistake writing the file_name and then the except will print? Or is there a different error I should be using?arrow_forwardIn Python: What gets printed by executing the following code?arrow_forwardWrite a PYTHON code with at least 2 functions (one should call the other). The function which is called by the first function should also be able to be called directly. Execute these functions with various parameters such that you can demonstrate the following: Each function should have some debug code so you can trace calls to the functions and activity within the functions. Each should handle at least 2 types of exceptions (both shouldn't handle the same exceptions). The function called by the first one should, at some point, generate a division by zero error, but should NOT handle this error. The function that calls the division by zero function should handle a division by zero error. Catch one error and raise a different one. Have some finally code (perhaps with some debugging output) Demonstrate & explain: call the division by zero function both from your main program and from the other function. show that the exception isn't handled by the function itself but by the other…arrow_forward
- I need help with fixing this java program as described in the image below:arrow_forwardWrite in C++. Need help matching the output belowarrow_forwardUse Standard Exceptions to write a program with a function called safe_divide that takes two double arguments and returns a double corresponding to the result of dividing the first argument by the second argument. Before performing the division, the function should check to determine if division by zero would occur. If division by zero would occur, instead of performing the division, the function should throw an exception of type std::invalid_argument. pls help answer this code in c++arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
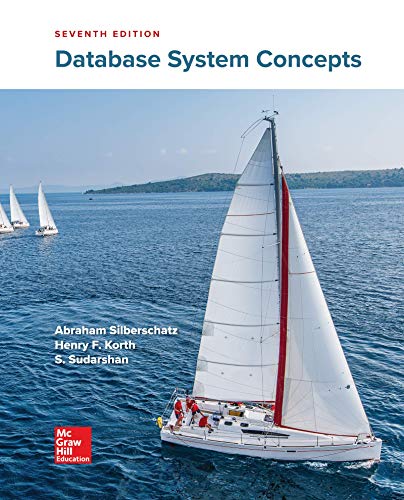
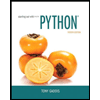
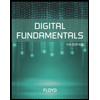
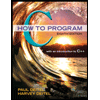
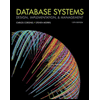
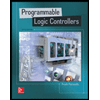