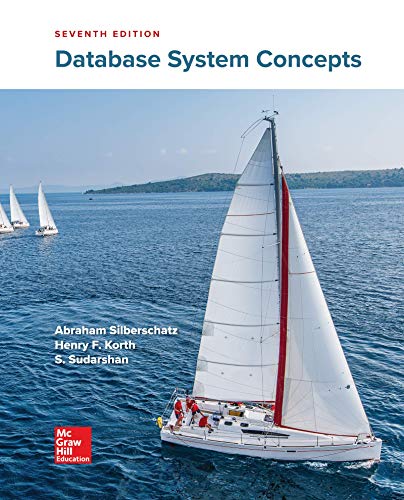
Concept explainers
Write a python code for all.
1. Write a Python program to do the following :
a. Take two numbers from the user in separate inputs. and then perform addition, subtraction, multiplication and division calculations on 2 numbers.
b. Consider the possibility of input errors (i.e. divisor is 0? user entered string instead of numbers? user entered float?) Use try and except to handle the 2 exceptions by outputting statements that indicate related errors.
2. From the two statements given below, using list comprehension, create the list1 (i.e. the characters from v which are in w): list1 = ['e','o'] .
w = 'hello'
v = ('a', 'e', 'i', 'o', 'u')
3. Write a function that returns the mean of a list that is passed as formal argument to a function. Create a list with values [10, 56.7, 56, 89, 100, 99, 87.5, 34, 985, 10] and pass it to the above defined function. Print the mean of the list of values.
4. Add a breakpoint to the mean function (created in question 3) such that the interactive console of pdb can be used to look at the values of mean after each item in the list is calculated.

Step by stepSolved in 3 steps with 4 images

- Use the functions from the previous three problems to implement a pro-gram that computes the sum of the squares of numbers read from a file. Your program should prompt for a file name and print out the sum of thesquares of the values in the file. Hint: Use readlines ()arrow_forwardWrite a C++ program to do the following: Read the attached file ("TextFile.txt") and the count the number of times each alphabetic letter is used. Do not count punctuation, whitespace, or numbers. Only count letters a-z. When counting, treat lower and upper case characters the same. Use the attached CPP program as a guide to manage the counting. Use the structure and array provided to maintain counts. See examples in the program. When the all processing is completed, display the total for all letter counts. Example Output Below.: This numbers below are not intended to be accurate. No special formatting required but should be readable. A: 103 B: 15 C: 22... // remaining lettersZ: 4 cpp code: #include "stdafx.h" #include <iostream> using namespace std; struct LetterCount { char letter; int count; }; // declare an array of structure const int MAXLETTERS = 26; LetterCount myLetterCount[MAXLETTERS]; int main() { // initialize - MOVE THIS TO A METHOD for…arrow_forwardIt is often said that nothing in life is certain but death and taxes. For a programmer or data scientist, however, nothing is certain but encountering errors. In Python, there are two primary types of errors, both of which you are likely familiar with: syntax errors and exceptions. Syntax errors occur when the proper structure of the language is not followed, while exceptions are errors that occur during the execution of a program. These include errors such as ZeroDivision Error, TypeError, NameError, and many more! Under the hood, these errors are based in the concepts of object orientation, and all exceptions are class objects. If you're interested in more detailed explanations of the structure of exceptions as well as how to create your own, check out this article from the Python documentation! In the meantime, we'll implement our own version of an Error class. Complete the Error, SyntaxError, and ZeroDivisionError classes such that they create the correct messages when called. •…arrow_forward
- I am struggling with this types of question because I don't understand it, can you please answer the question and can you be very specfic with it.question: What is the output of the program?arrow_forward2. Find the output of the Python code given below? for i in (1,2,3): print("i")arrow_forwardQ1) Write a python function that will remove a given character from a sentence based on the starting and ending index of the sentence. In the place of the removed characters, add the count of the number of characters removed so far. For this program, you need to take 4 user inputs. Few exceptions to catch: • Sentence . Character to be removed • Starting index (inclusive) • Ending index (inclusive) 2 Example 1: Input: 'my python programming book' 'm' 20 • Name Error if a variable is not found. Print 'Name Error: Variable not defined' • Value Error if the starting index and ending index is not an integer. Print 'Value Error: Wrong input, please enter an integer value. • Type Error when adding count in the new sentence. Print Type Error: Cannot add integer value with string. • Index Error if the starting and ending index is out of range. Print 'Index Error: Index is outside the range of sentence length. For other exceptions, catch Exception. Print 'Some other error occurred. Output: my…arrow_forward
- I find these types of questions challenging due to a lack of understanding. Could you kindly provide a detailed and specific answer to assist me to answer the question below.question:Local variables in different blocks may have the same name. What is the output of the program?arrow_forwardWrite a PYTHON code with at least 2 functions (one should call the other). The function which is called by the first function should also be able to be called directly. Execute these functions with various parameters such that you can demonstrate the following: Each function should have some debug code so you can trace calls to the functions and activity within the functions. Each should handle at least 2 types of exceptions (both shouldn't handle the same exceptions). The function called by the first one should, at some point, generate a division by zero error, but should NOT handle this error. The function that calls the division by zero function should handle a division by zero error. Catch one error and raise a different one. Have some finally code (perhaps with some debugging output) Demonstrate & explain: call the division by zero function both from your main program and from the other function. show that the exception isn't handled by the function itself but by the other…arrow_forward20arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
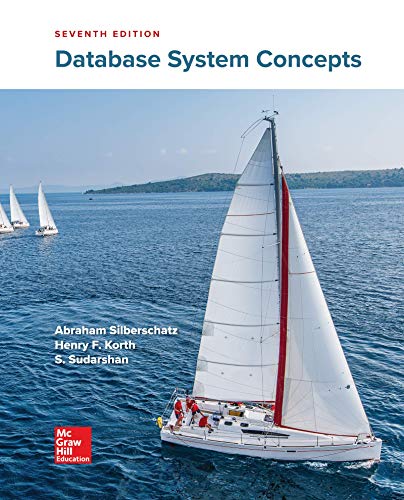
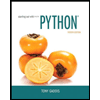
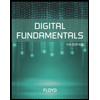
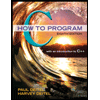
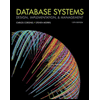
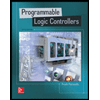