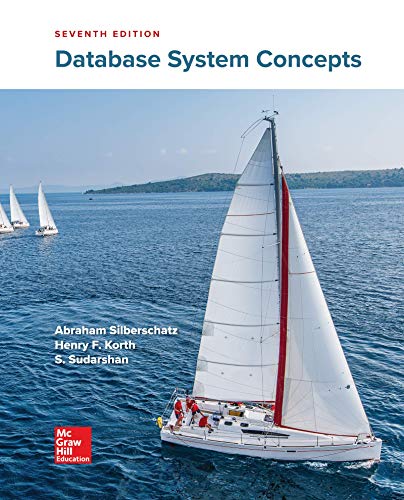
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![12.14 Zylab 3 - Single Procedure Call
Given an array of at least one integer, write a program to create a new array with elements equal to the power of each element in the
original array raised to the index, i.e., P[i] = A[i]^i.
For this, write two functions that will be called in main function independently.
● power
inputs: element (A[i]) and index (1)
• task: returns the value of element raised to index (A[i]^i).
}
O
• newElement
inputs: base address of new array P (*P), current size of P (variable k) and the new element (A[i]^i)
o task: add the new element at the end.
o This function does not return any value (void).
O
Following is a sample C code to perform the required task. You may modify the code for the functions, but the task performed should not be
changed.
int main() {
// Variable Declaration
int *A, *P;
int n, k;
int pow;
// Task of main function
P[0] 1;
for (int j =
k = j;
pow
// Base addresses of A and P
// Lengths of arrays A and B
// Return value from power function
}
k++;
// 0th element = A[0]^0 = 1
1; j < n; j++) {
// Current length of array B
power (A[j], j);
newElement(P, k, pow);
int power(int a, int b) {
int pow= a;](https://content.bartleby.com/qna-images/question/909b55a2-117c-4c26-8933-e5a86ba10ee3/01e10ccc-8b4e-4c44-aa35-c0c254aa1e29/1xz9h8_thumbnail.png)
Transcribed Image Text:12.14 Zylab 3 - Single Procedure Call
Given an array of at least one integer, write a program to create a new array with elements equal to the power of each element in the
original array raised to the index, i.e., P[i] = A[i]^i.
For this, write two functions that will be called in main function independently.
● power
inputs: element (A[i]) and index (1)
• task: returns the value of element raised to index (A[i]^i).
}
O
• newElement
inputs: base address of new array P (*P), current size of P (variable k) and the new element (A[i]^i)
o task: add the new element at the end.
o This function does not return any value (void).
O
Following is a sample C code to perform the required task. You may modify the code for the functions, but the task performed should not be
changed.
int main() {
// Variable Declaration
int *A, *P;
int n, k;
int pow;
// Task of main function
P[0] 1;
for (int j =
k = j;
pow
// Base addresses of A and P
// Lengths of arrays A and B
// Return value from power function
}
k++;
// 0th element = A[0]^0 = 1
1; j < n; j++) {
// Current length of array B
power (A[j], j);
newElement(P, k, pow);
int power(int a, int b) {
int pow= a;
![int main() {
}
}
// Variable Declaration
int *A, *P;
int n, k;
int pow;
}
// Task of main function
P[0]
1;
=
}
k++;
// Base addresses of A and P
// Lengths of arrays A and B
// Return value from power function
// 0th element
for (int j = 1; j < n; j++) {
k = j;
}
return (pow);
=
pow power (A[j], j);
newElement(P, k, pow);
int power(int a, int b) {
int pow= a;
for (int 1 1; 1 < b; 1++) {
pow = pow * a;
// Current length of array B
A[0]^0 = 1
void newElement(int* P, int k, int pow) {
P[k] = pow;
Registers Variables
$50
A
$s1
$s2
$s3
n
P
k](https://content.bartleby.com/qna-images/question/909b55a2-117c-4c26-8933-e5a86ba10ee3/01e10ccc-8b4e-4c44-aa35-c0c254aa1e29/vjmxq0y_thumbnail.png)
Transcribed Image Text:int main() {
}
}
// Variable Declaration
int *A, *P;
int n, k;
int pow;
}
// Task of main function
P[0]
1;
=
}
k++;
// Base addresses of A and P
// Lengths of arrays A and B
// Return value from power function
// 0th element
for (int j = 1; j < n; j++) {
k = j;
}
return (pow);
=
pow power (A[j], j);
newElement(P, k, pow);
int power(int a, int b) {
int pow= a;
for (int 1 1; 1 < b; 1++) {
pow = pow * a;
// Current length of array B
A[0]^0 = 1
void newElement(int* P, int k, int pow) {
P[k] = pow;
Registers Variables
$50
A
$s1
$s2
$s3
n
P
k
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 9. Implement an array with values 1, 5, 14, 23, 45, 52, 58, 81, 82 91. a) Create a getindex( function which does a linear search upon the array for a specific value n. Return the index of n, or -1 ifn does not exist, b) Print the array. c) Search the array for the values 23, 58, 11, rint the reaults. Qutpur Examnle 15 14 23 45 52 58 71 82 91 Number 23 is located at index Number 58 As located at index C Number 11 i looated at index -1arrow_forward4.11 LAB: Number pattern Write a recursive function called print_num_pattern() to output the following number pattern. Given a positive integer as input (Ex: 12), subtract another positive integer (Ex: 3) continually until a negative value is reached, and then continually add the second integer until the first integer is again reached. For this lab, do not end output with a newline. Do not modify the given main program. Ex. If the input is:arrow_forward5.22 LAB: Array palindrome (JAVA) Write a program in java that reads a list of integers from input and determines if the list is a palindrome (values are identical from first to last and last to first). The input begins with an integer indicating the length of the list that follows. Assume that the list will always contain fewer than 20 integers. Output "yes" if the list is a palindrome and "no" otherwise. The output ends with a newline. Ex: If the input is: 6 1 5 9 9 5 1 the output is: yes Ex: If the input is: 5 1 2 3 4 5 the output is: no Code starts here: import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int[] userValues = new int[20]; // List of integers from input /* Type your code here. */ }}arrow_forward
- 7.9 LAB: Sorting TV Shows (dictionaries and lists) Write a program that first reads in the name of an input file and then reads the input file using the file.readlines() method. The input file contains an unsorted list of number of seasons followed by the corresponding TV show. Your program should put the contents of the input file into a dictionary where the number of seasons are the keys, and a list of TV shows are the values (since multiple shows could have the same number of seasons). Sort the dictionary by key (least to greatest) and output the results to a file named output_keys.txt, separating multiple TV shows associated with the same key with a semicolon (;). Next, sort the dictionary by values (alphabetical order), and output the results to a file named output_titles.txt. Ex: If the input is: file1.txt and the contents of file1.txt are: 20 Gunsmoke 30 The Simpsons 10 Will & Grace 14 Dallas 20 Law & Order 12 Murder, She Wrote the file output_keys.txt should…arrow_forward7.19 LAB: Adjust list by normalizing When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by dividing all values by the largest value. The input begins with an integer indicating the number of floating-point values that follow. Assume that the list will always contain less than 20 floating-point values. For coding simplicity, follow every output value by a space, including the last one. And, output each floating-point value with two digits after the decimal point, which can be achieved as follows:printf("%0.2lf ", yourValue); Ex: If the input is: 5 30.0 50.0 10.0 100.0 65.0 the output is: 0.30 0.50 0.10 1.00 0.65 The 5 indicates that there are five floating-point values in the list, namely 30.0, 50.0, 10.0, 100.0, and 65.0. 100.0 is the largest value in the list, so each value is divided by 100.0.…arrow_forward14.13 CIS 2348 LAB: Sorting user IDs Given code that reads user IDs (until -1), complete the quicksort() and partition() functions to sort the IDs in ascending order using the Quicksort algorithm. Increment the global variable num_calls in quicksort() to keep track of how many times quicksort() is called. The given code outputs num_calls followed by the sorted IDs. Ex: If the input is: kaylasimms julia myron1994 kaylajones -1 the output is: 7 julia kaylajones kaylasimms myron1994 Code # Global variable num_calls = 0 # TODO: Write the partitioning algorithm - pick the middle element as the # pivot, compare the values using two index variables l and h (low and high), # initialized to the left and right sides of the current elements being sorted, # and determine if a swap is necessary def partition(user_ids, i, k): # TODO: Write the quicksort algorithm that recursively sorts the low and # high partitions. Add 1 to num_calls each time quisksort() is called def quicksort(user_ids, i,…arrow_forward
- 23.8 LAB: Consecutive heads (Use Python) Given main() and GVCoin class, complete function consecutive_heads() that counts and returns the number of flips taken to achieve a desired number of consecutive heads without a tails. Function consecutive_heads() has a GVCoin object and an integer representing the desired number of consecutive heads without a tails as parameters. Note: For testing purposes, a GVCoin object is created in the main() function using a pseudo-random number generator with a fixed seed value. The program uses a seed value of 15 during development, but when submitted, a different seed value will be used for each test case. Refer to the textbook section on random numbers to learn more about pseudo-random numbers. Ex: If the GVCoin object is created with a seed value of 15 and the desired number of consecutive heads is 5, then the function consecutive_heads() returns 16 and the program outputs: Total number of flips for 5 consecutive heads: 16arrow_forward9.8: Write a program that produces a histogram of student grades for an assignment. The program must allow the user to input each student's grade as an integer until the user inputs -1. The program will store user's input in a vector (covered in Chapter 8). Then the program will allocate the memory for the histogram such that the histogram is able to hold all values from 0 to the maximum grade found in the vector. After this, the program will compute the histogram and print the histogram to standard output. For example, if the user's input was: 20 30 4 20 30 30 -1 Then the output would be: Number of 4's: 1 Number of 20's: 2 Number of 30's: 3 SAMPLE RUN #1: ./histogram Interactive Session Hide Invisibles Enter:20.30.4.20.30.30.-14 the student's.scores, terminating with. -1: - Number of.4's: 14 Number of 20's: 24 Number of 30's::34arrow_forward15.11 LAB: Sorting user IDs C++ Given a main() that reads user IDs (until -1), complete the Quicksort() and Partition() functions to sort the IDs in ascending order using the Quicksort algorithm, and output the sorted IDs one per line. Ex. If the input is: kaylasimms julia myron1994 kaylajones -1 the output is: julia kaylajones kaylasimms myron1994 I can only change the //TODO and please answer in C++ #include <string>#include <vector>#include <iostream> using namespace std; // TODO: Write the partitioning algorithm - pick the middle element as the// pivot, compare the values using two index variables l and h (low and high),// initialized to the left and right sides of the current elements being sorted,// and determine if a swap is necessaryint Partition(vector<string> &userIDs, int i, int k) { } // TODO: Write the quicksort algorithm that recursively sorts the low and// high partitionsvoid Quicksort(vector<string> &userIDs, int i, int k) {…arrow_forward
- 11.17 (Bucket Sort) Use Python. Show the whole code input and output when done. A bucket sort begins with a one-dimensional array of positive integers to be sorted and a two-dimensional array of integers with rows indexed from 0 to 9 and columns indexed from 0 to n – 1, where n is the number of values to be sorted. Each row of the two-dimensional array is referred to as a bucket. Write a class named BucketSort containing a function called sort that operates as follows: a) Place each value of the one-dimensional array into a row of the bucket array, based on the value’s “ones” (rightmost) digit. For example, 97 is placed in row 7, 3 is placed in row 3, and 100 is placed in row 0. This procedure is called a distribution pass. b) Loop through the bucket array row by row, and copy the values back to the original array. This procedure is called a gathering pass. The new order of the preceding values in the one-dimensional array is 100, 3 and 97. c) Repeat this process for each subsequent…arrow_forward8.19 LAB: Flip a coin Define a function named coin_flip that returns "Heads" or "Tails" according to a random value 1 or 0. Assume the value 1 represents "Heads" and 0 represents "Tails". Then, write a main program that reads the desired number of coin flips as an input, calls function coin_flip() repeatedly according to the number of coin flips, and outputs the results. Assume the input is a value greater than 0. Ex: If the random seed value is 5 and the input is: 3 the output is: Heads Heads Tails Note: For testing purposes, a pseudo-random number generator with a fixed seed value is used in the program. The program uses a seed value of 5 during development, but when submitted, a different seed value may be used for each test case. The program must define and call the following function: def coin_flip()arrow_forward24.12 LAB: Dean's list A student makes the Dean's list if their GPA is 3.5 or higher. Complete the Course class by implementing the GetDeansList() member function, which returns a vector of students with a GPA of 3.5 or higher. Given classes: • Class Course represents a course, which contains a vector of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three data members: first name, last name, and GPA. (Hint: getGPA() returns a student's GPA.) ● Note: For testing purposes, different student values will be used. Ex. For the following students: Henry Nguyen 3.5 Brenda Stern 2.0 Lynda Robison 3.2 Sonya King 3.9 the output is: Dean's list: Henry Nguyen (GPA: 3.5) Sonya King (GPA: 3.9)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
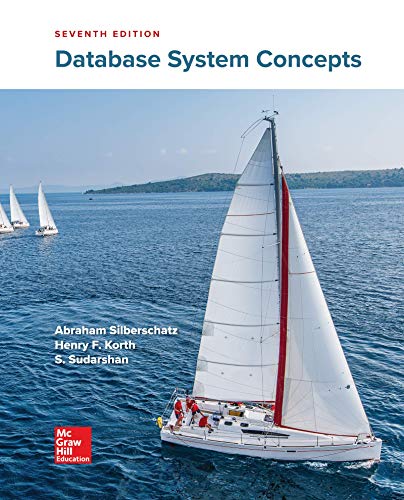
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
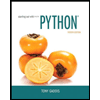
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
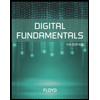
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
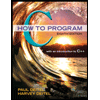
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
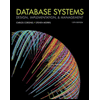
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
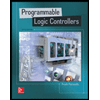
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education