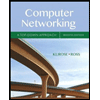
14.8 LAB: Selection sort
Write a
Code I have so far:
def selection_sort_descend_trace(lst):
for i in range(len(lst) - 1):
max_ind = i
for j in range(i + 1, len(lst)):
if lst[j] > lst[max_ind]:
max_ind = j
lst[i], lst[max_ind] = lst[max_ind], lst[i]
print(' '.join([str(x) for x in lst]))
return lst
if __name__ == '__main__':
numbers = [int(x) for x in input().split()]
selection_sort_descend_trace(numbers)
Error I am getting:
How do i get the brackets and comma?
![Expected output
3: Compare output
Your output
88 79 52 45 33
Output differs. See highlights below.
Input
Expected output
[88, 79, 52, 33, 45]
[88, 79, 52, 33, 45]
[88, 79, 52, 33, 45]
[88, 79, 52, 45,
33]
1 2 3 4 5 6 7 8 9
9 2 3 4
9 8 3
9 8 7 4
9 8 7
9 8 7
9 8 7
9 8 7
4
9 8 7
6
6
6
6
5 6
5 6
5
5
5
5
5
6 5
6
4
4
4
4
4
7
4
7
3
3
3
3
8
3
2
2
2
2
2
3 2
2
1
1
1
1
1
1
1
1
[9, 2, 3, 4, 5,
6, 7, 8,
1]
[9, 8, 3,
5,
6, 7, 2, 1]
I
[9, 8, 7, 4, 5, 6, 3, 2, 1]
4,
3, 2,
1]
6, 5,
6, 5,
4, 3, 2, 1]
[9, 8, 7,
[9, 8, 7,
[9, 8, 7,
[9, 8, 7,
6, 5,
6,
HHHHH
4, 3, 2,
1]
5, 4, 3, 2, 1]
0/2](https://content.bartleby.com/qna-images/question/1f559ff2-7390-4b86-9602-ab51f205485c/67cd5453-8fd8-4cf0-a25e-c1835647fe16/8z7ywm_thumbnail.png)

Step by stepSolved in 4 steps with 2 images

- 1.0) head Function Define and implement this function in data_utils.py. Purpose: Produce a new column-based (e.g. dict[str, list[str]1) table with only the first N (a parameter) rows of data for each column. Why: Visualizing a table with hundreds, thousands, or millions of rows in it is overwhelming. You frequently want to just see the first few rows of a table to get a sense you are on the correct path. Function name: head Parameters: 1. dict[str, list[str]]-a column-based table of data that will not be mutated 2. int - The number of "rows" to include in the resulting list • Return type: dict[str, list[str]] Implementation strategy: 1. Establish an empty dictionary that will serve as the returned dictionary this function is building up. 2. Loop through each of the columns in the first row of the table given as a parameter. 1. Inside of the loop, establish an empty list to store each of the first N values in the column. 2. Loop through the first N items of the table's column, 1.…arrow_forward12arrow_forward7) Use a counting sort on a set of keys that fall between (a, b), where a and b are modest positive numbers that the user inputs. 8) For an acceptable selection of the number of buckets, implement bucket sort with an array of lists data structure to sort a list of characters, a list of floating point values, and a list of uniformly distributed integers.arrow_forward
- 4. List comprehension. a) Implement a list comprehension that creates a list of all odd numbers between 1 and 20 inclusive. b) Print the list with the elements separated by a space. Output Example 1 3 5 7 9 11 13 15 17 19 5. List slicing. a) Use a list comprehension to generate a list of all even numbers from 0 to 100 inclusive. Print the list. b) Use slicing to print the first five numbers in the list. c) Use slicing to print the last five numbers in the list. Hint: use len () d) Use slicing to print all list numbers between 25 and 30 inclusive. 2 Output Example [0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24, 26, 28, 30, 32, 34, 36, 38, 40, 42, 44, 46, 48, 50] [0, 2, 4, 6, 8] [32, 34, 36, 38, 40, 42, 44, 46, 48, 50] [20, 22, 24, 26, 28, 30]arrow_forwardI need help on this onearrow_forward6.9 LAB: All permutations of names Write a program that lists all ways people can line up for a photo (all permutations of a list of Strings). The program will read a list of one word names (until -1), and use a recursive method to create and output all possible orderings of those names, one ordering per line. When the input is: Julia Lucas Mia -1 then the output is (must match the below ordering): Julia Lucas Mia Julia Mia Lucas Lucas Julia Mia Lucas Mia Julia Mia Julia Lucas Mia Lucas Julia import java.util.Scanner;import java.util.ArrayList; public class PhotoLineups { // TODO: Write method to create and output all permutations of the list of names.public static void allPermutations(ArrayList<String> permList, ArrayList<String> nameList) {} public static void main(String[] args) {Scanner scnr = new Scanner(System.in);ArrayList<String> nameList = new ArrayList<String>();ArrayList<String> permList = new ArrayList<String>();String name;//…arrow_forward
- 5.19 LAB: Adjust list by normalizing When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by dividing all values by the largest value. The input begins with an integer indicating the number of floating-point values that follow. Assume that the list will always contain less than 20 floating-point values. For coding simplicity, follow every output value by a space, including the last one. And, output each floating-point value with two digits after the decimal point, which can be achieved as follows:printf("%0.2lf ", yourValue); Ex: If the input is: 5 30.0 50.0 10.0 100.0 65.0 the output is: 0.30 0.50 0.10 1.00 0.65 The 5 indicates that there are five floating-point values in the list, namely 30.0, 50.0, 10.0, 100.0, and 65.0. 100.0 is the largest value in the list, so each value is divided by 100.0.…arrow_forwarddef large_rec(m: list[list[int]], r: int, c: int) -> int:"""Do not use any imports, dictionaries, try-except statements or break and continue please you must make use of the helper long here as you loop througheach row of the matrix returns the area of the largest rectangle whose top left corner is atposition r, c in m.>>> case1 = [[1, 0, 1, 0, 0],... [1, 0, 1, 1, 1],... [1, 1, 1, 1, 1],... [1, 0, 0, 1, 0]]>>> largest_at_position(case1, 1, 2)6""" Helper code: def long(lst: list[int]) -> int: count = 0i = 0while i < len(lst) and lst[i] != 0:i = i + 1count = count + 1return countarrow_forwardPlease answer this ques in python with showing the codearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
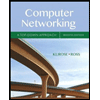
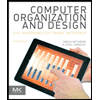
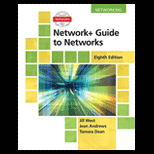
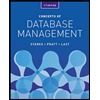
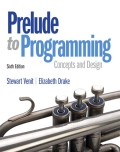
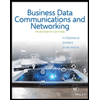